In this article, we’ll write a program to find largest number in an array using programming languages such as C#, C++, Python, and Java.
Table of Contents
Definition of Array:
An array is a data structure that allows the storage of a collection of elements of the same data type under a single identifier. Array elements are stored in contiguous memory and are accessed using numeric indexes starting at 0.
Arrays provide a structured way to organize and manage data and allow developers to work with multiple values of the same data type within a single variable.
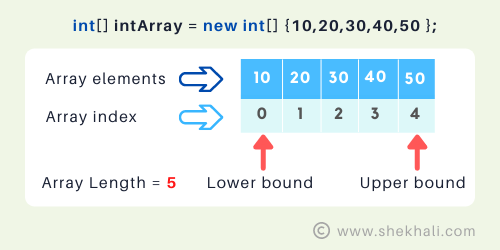
C#
Example 1: C# program to find largest number in an array using LINQ.
using System;
using System.Linq;
class Program
{
static void Main()
{
// Sample array
int[] numbers = { 12, 45, 78, 23, 56, 89, 34, 67 };
// Find the largest number using LINQ
int largestNumber = numbers.Max();
// Display the result
Console.WriteLine("The largest number in the array is: " + largestNumber);
}
}
Output:
The largest number in the array is: 89
Example 2: C# program to Iterate through the array and identify the largest number.
using System;
class Program
{
static void Main()
{
// Sample array
int[] numbers = { 12, 45, 78, 23, 56, 89, 34, 67 };
// Find the largest number
int largestNumber = FindLargestNumber(numbers);
// Display the result
Console.WriteLine("The largest number in the array is: " + largestNumber);
}
static int FindLargestNumber(int[] array)
{
// Initialize the largest number with the first element
int maxNumber = array[0];
// Iterate through the array to find the largest number
foreach (int num in array)
{
if (num > maxNumber)
{
maxNumber = num;
}
}
// Return the largest number
return maxNumber;
}
}
Output:
The largest number in the array is: 89
Code Explanation:
- We start by defining an array of integers named
numbers
. - The
FindLargestNumber()
method is then created to iterate through the array and identify the largest number. - The
Main
method callsFindLargestNumber
and displays the result.
C++
C++ program to find largest number in an array.
#include <iostream>
using namespace std;
int findLargestNumber(int array[], int size) {
// Initialize the largest number with the first element
int maxNumber = array[0];
// Iterate through the array to find the largest number
for (int i = 1; i < size; i++) {
if (array[i] > maxNumber) {
maxNumber = array[i];
}
}
// Return the largest number
return maxNumber;
}
int main() {
// Sample array
int numbers[] = { 12, 45, 78, 23, 56, 89, 34, 67 };
int size = sizeof(numbers) / sizeof(numbers[0]);
// Find the largest number
int largestNumber = findLargestNumber(numbers, size);
// Display the result
cout << "The largest number in the array is: " << largestNumber << endl;
return 0;
}
Output:
The largest number in the array is: 89
Python
Python program to find largest number in an array.
def find_largest_number(numbers):
# Initialize the largest number with the first element
max_number = numbers[0]
# Iterate through the array to find the largest number
for num in numbers[1:]:
if num > max_number:
max_number = num
# Return the largest number
return max_number
# Sample array
numbers = [12, 45, 78, 23, 56, 89, 34, 67]
# Find the largest number
largest_number = find_largest_number(numbers)
# Display the result
print("The largest number in the array is:", largest_number)
Output:
The largest number in the array is: 89
JAVA
JAVA program to find largest number in an array.
public class FindLargestNumber {
public static void main(String[] args) {
// Sample array
int[] numbers = {12, 45, 78, 23, 56, 89, 34, 67};
// Find the largest number
int largestNumber = findLargestNumber(numbers);
// Display the result
System.out.println("The largest number in the array is: " + largestNumber);
}
static int findLargestNumber(int[] array) {
// Initialize the largest number with the first element
int maxNumber = array[0];
// Iterate through the array to find the largest number
for (int num : array) {
if (num > maxNumber) {
maxNumber = num;
}
}
// Return the largest number
return maxNumber;
}
}
Output:
The largest number in the array is: 89
Conclusion
In this article, we’ve explored how to find the largest number in an array using C#, C++, Python, and Java. Understanding this fundamental task provides a solid foundation for working with arrays in various programming languages. Happy coding!
Recommended Articles:
- Program to copy all elements of an array into another array
- C# Program to Convert Decimal to Binary with Examples
- C# Program to Convert Binary to Decimal with Examples
- C# Program to Convert Hexadecimal to Decimal
- How to remove duplicate characters from a String in C#
- C# program to count the occurrences of each character in a String
- C# Program to Print Multiplication Table of a Given Number
- Program to print prime numbers from 1 to N
- C# Program to Check if a Given Number is Even or Odd
- Program to copy all elements of an array into another array
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- C# Programs asked in Interviews
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024