Dependency Injection (DI) is a software design pattern that helps developers to build high-quality software.
It reduces tight coupling between software components and allows us to develop loosely-coupled code, which is easier to maintain and offers greater flexibility and testability.
The fundamental principle behind Dependency Injection is to minimize hard-coded dependencies among classes by dynamically injecting them during runtime instead of design time.
This approach ensures that the dependencies of a class are provided from external sources, typically through constructor parameters or property setters, rather than being directly instantiated within the class itself.
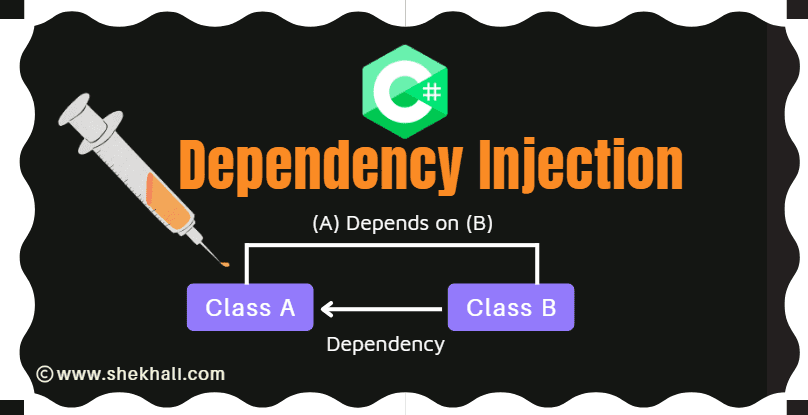
A dependency is an object that another object depends on.
Table of Contents
- 1 Understanding the Problem:
- 2 Different ways to achieve Dependency Injection in C#
- 3 Constructor Injection in C#
- 4 Property Injection in C#
- 5 Method Injection in C#
- 6 FAQs
- 6.1 Q: What is dependency injection in C#?
- 6.2 Q: What are the different types of dependency injection in C#?
- 6.3 Q: What is constructor injection in C#?
- 6.4 Q: What is property injection in C#?
- 6.5 Q: What is method injection in C#?
- 6.6 Q: What are the advantages of using dependency injection in C#?
- 6.7 Q: What are some common dependency injection frameworks in C#?
- 6.8 Related
Understanding the Problem:
Let’s imagine a situation where we have two classes, ClassA
and ClassB
. ClassA
relies on ClassB
to carry out specific tasks.
In the traditional approach, ClassA
would create an instance of ClassB
internally, leading to tight coupling between the two classes. This tight coupling causes various problems, such as making it challenging to modify or test ClassA
independently.
Code Example:
Let’s illustrate the traditional approach with a code example:
public class ClassA
{
private ClassB dependency; // ClassA has a dependency on ClassB
public ClassA()
{
dependency = new ClassB(); // ClassA creates an instance of ClassB internally
}
// ClassA methods and operations
}
public class ClassB
{
// ClassB methods and operations
}
In the above example, ClassA
creates an instance of ClassB
internally using the new
keyword. This approach makes ClassA
tightly coupled with ClassB
, making it challenging to modify or test ClassA
independently.
Different ways to achieve Dependency Injection in C#
There are three different ways to achieve dependency injection in C#:
- Constructor injection.
- Method injection.
- Property injection.
Constructor Injection in C#
Constructor injection is a technique in software development where dependencies are provided to a class through its constructor. It involves passing the required dependencies as parameters when creating an instance of the class. It enables loose coupling and makes the class more flexible and testable.
The following is an example of Constructor Injection in C#:
using System;
// Interface for the dependency
public interface IMessageService
{
void SendMessage(string message);
}
// Implementation of the dependency
public class EmailService : IMessageService
{
public void SendMessage(string message)
{
Console.WriteLine($"Sending email: {message}");
}
}
// Class that requires the dependency
public class NotificationService
{
private readonly IMessageService messageService;
// Constructor injection
public NotificationService(IMessageService messageService)
{
this.messageService = messageService;
}
// Method that uses the dependency
public void NotifyUser(string userName)
{
string message = $"Hello, {userName}! You have a new notification.";
messageService.SendMessage(message);
}
}
// Main class to run the code
class Program
{
static void Main(string[] args)
{
// Create an instance of the dependency
IMessageService emailService = new EmailService();
// Create an instance of the class with the dependency injected
NotificationService notificationService = new NotificationService(emailService);
// Use the class with the injected dependency
notificationService.NotifyUser("Roman");
}
}
Output:
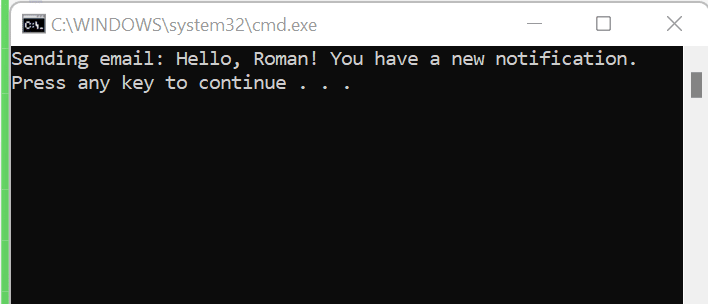
Property Injection in C#
Property injection in C# is a technique where public properties provide dependencies to a class. Instead of passing dependencies through the constructor, the class exposes properties that can be set with the required dependencies from an external source.
It allows for flexibility in injecting dependencies after the object is created. Property injection promotes loose coupling and enhances the testability and modifiability of the code.
The following is an example of Property Injection in C#:
using System;
// Interface for the dependency
public interface IMessageService
{
void SendMessage(string message);
}
// Implementation of the dependency
public class EmailService : IMessageService
{
public void SendMessage(string message)
{
Console.WriteLine($"Sending email: {message}");
}
}
// Class that requires the dependency
public class NotificationService
{
// Property to inject the dependency
public IMessageService MessageService { get; set; }
// Method that uses the dependency
public void NotifyUser(string userName)
{
string message = $"Hello, {userName}! You have a new notification.";
MessageService.SendMessage(message);
}
}
// Main class to run the code
class Program
{
static void Main(string[] args)
{
// Create an instance of the dependency
IMessageService emailService = new EmailService();
// Create an instance of the class
NotificationService notificationService = new NotificationService();
// Inject the dependency through the property
notificationService.MessageService = emailService;
// Use the class with the injected dependency
notificationService.NotifyUser("Roman");
}
}
Output:
Sending email: Hello, Roman! You have a new notification.
Method Injection in C#
Method injection in C# is a technique that provides a method with a dependency as a parameter. Instead of injecting the dependency through the constructor or as a property, it is passed explicitly when calling the method.
This allows for flexibility in injecting different dependencies for specific method invocations, promoting loose coupling and enhancing the modifiability and testability of the code.
The following is an example of Method Injection in C#:
using System;
// Interface for the dependency
public interface ILogger
{
void Log(string message);
}
// Implementation of the dependency
public class ConsoleLogger : ILogger
{
public void Log(string message)
{
Console.WriteLine($"Logging: {message}");
}
}
// Class that requires the dependency
public class PaymentService
{
// Method with the dependency parameter
public void ProcessPayment(decimal amount, ILogger logger)
{
// Perform payment processing logic
// ...
// Log the payment transaction
logger.Log($"Payment processed: {amount}$");
}
}
// Main class to run the code
class Program
{
static void Main(string[] args)
{
// Create an instance of the dependency
ILogger consoleLogger = new ConsoleLogger();
// Create an instance of the class
PaymentService paymentService = new PaymentService();
// Use the class with the injected dependency through method injection
paymentService.ProcessPayment(100.50m, consoleLogger);
}
}
Output:
Logging: Payment processed: 100.50$
FAQs
Q: What is dependency injection in C#?
Dependency injection is a design pattern that reduces dependencies between classes and components in a software system. It allows for creating loosely-coupled code that is easier to maintain and test.
Q: What are the different types of dependency injection in C#?
There are three types of dependency injection in C#:
Constructor injection, property injection, and method injection.
Q: What is constructor injection in C#?
Constructor injection is a type of dependency injection where dependencies are passed to a class through its constructor. Constructor injection is the most common type of dependency injection used in C#.
Q: What is property injection in C#?
Property injection is a type of dependency injection where dependencies are set through public properties of a class. This type of injection is less common than constructor injection and can lead to more complex code.
Q: What is method injection in C#?
Method injection is a type of dependency injection where dependencies are passed to a method as parameters. This type of injection is useful for methods that need to use a dependency only occasionally or in specific scenarios.
Q: What are the advantages of using dependency injection in C#?
Dependency injection can make code more modular and easier to test and maintain. It can also reduce dependencies between classes and improve overall code quality.
Q: What are some common dependency injection frameworks in C#?
Some popular dependency injection frameworks in C# include Autofac, Ninject, and Unity. These frameworks provide tools and utilities to manage and inject dependencies in a software system.
References: MSDN-DI in .NET
Recommended Articles:
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- Difference between interface and abstract class In C#
- C# Struct vs Class
- Constructors in C# with Examples
- C++ vs C#: Difference Between C# and C++
- C# Regex: Introduction to Regular Expressions in C# with Examples
- C# String Interpolation – Syntax, Examples, and Performance
- C# Stopwatch Explained – How to Measure Code Execution Time?
- C# String Vs StringBuilder
- C# Queue with examples
- Web API vs web service: Top 10+ Differences between web API and web service in C#
- C# Polymorphism: Different types of polymorphism in C# with examples
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024