C# Arrays: An Array is used to store multiple values in a single variable, rather than declaring a separate variable for each element. It is a fixed-sized sequential collection of elements of the same type.
The data types of the elements in an array can be int
, char
, float
, string
, and so on, and they are stored in a contiguous location.
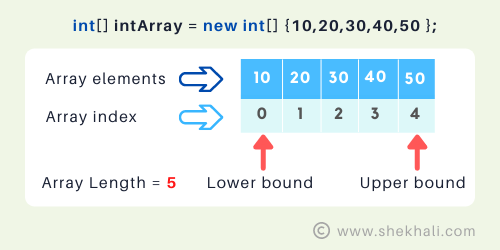
Array elements are accessed using numeric indexes and start at 0. The default value for numeric array items is zero, while the default value for reference array elements is null.
The array is available within the System.Array
namespace. It must be specified in brackets [], along with the type of variables it will carry and, therefore, the array’s name.
Table of Contents
Create an Array
In C#, arrays can be defined as fixed or dynamic lengths. Fixed-length arrays can only store a specified number of elements.
Dynamic arrays, on the other hand, have no predefined size. A dynamic array expands in size as new elements are added.
You can also transform the dynamic array into a static array after defining it.
Let’s look at an example of a single-dimensional array of a fixed size of integer type.
To create a single-dimensional array in C#, you must use square brackets [] after the type.
// Declare a single-dimensional array having a fixed length of 5
int[] intArray1 = new int[5];
// Declare and set the values of array elements.
int[] intArray2 = new int[] {10, 20, 30, 40, 50 }
// Alternative syntax.
int[] intArray3 = { 10, 20, 30, 40, 50 };
In the above code snippet, we have declared and initialized an integer-type array that can hold up to five elements. Since an array is a reference type, a new keyword is needed to create an instance of the array.
If you want an array to hold data of any type than its type must be specified as an object. Because All predefined, user-defined, reference, and value types in the C# type system are derived directly or indirectly from Object.
Arrays in C#: What You Should Know
- The Array in C# is basically a reference type that is available in
System.Array
namespace. - The Array class is an
abstract
base class, which means we can’t actually create an instance of it. - The default value for numeric array elements is zero, while reference elements are set to null.
- All arrays implement the IList, and IEnumerable interfaces, which allows you to iterate over an array using the foreach statement.
- Arrays in C# are actually objects, so you can use the array’s properties and other methods. An example of this is using the Length property to get the array’s length.
- A C# array is an object of the base type
System.Array
. - In an array, the index position starts at 0.
- The
Array
class is not part of theSystem.Collections
namespace. However, because it is based on the IList interface, it is considered a collection. - The default maximum size of an Array in the.net framework is 2 gigabytes (GB).
Access the elements of an array
The index number is used to access an array element. This statement retrieves the value of the first and second elements in the array called numbers.
using System;
namespace TestConsoleApp
{
internal class Program
{
static void Main(string[] args)
{
// Declare and set array element values.
int[] numbers = { 10, 20, 30, 40, 50 };
// Access first and second elements of an array
Console.WriteLine(numbers[0]);
Console.WriteLine(numbers[1]);
Console.ReadLine();
}
}
}
// Output:
// 10
// 20
You will get an error if you try to pass an item index that is greater than the number of items in the array.
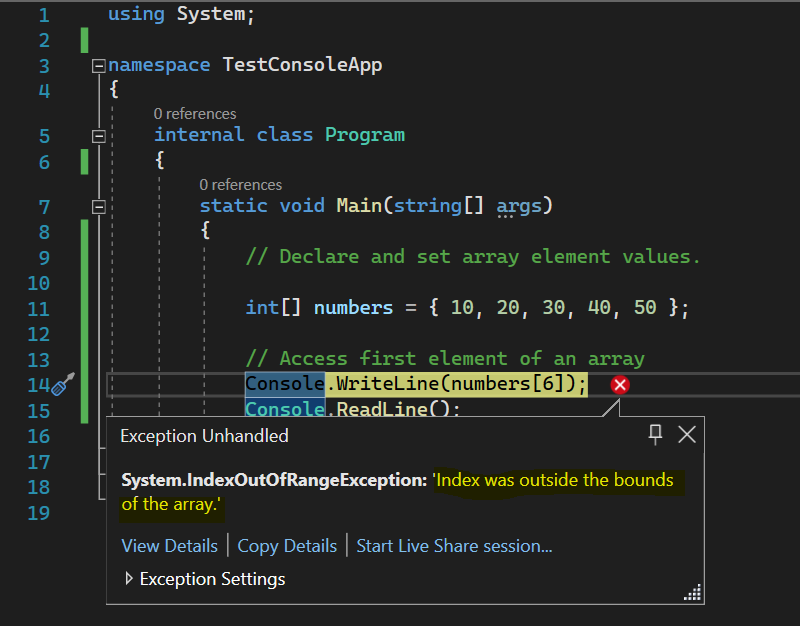
Accessing items in a C# array using a foreach loop
You can iterate over the array using either a for loop or a foreach statement. For example, the following code uses a foreach statement to read all the elements from an array of strings.
using System;
namespace CSharpArrayExample
{
public class CSharpArrayExample
{
static void Main(string[] args)
{
// Declare an array
string[] fruitsName = { "Apple", "Orange", "Banana", "Grapes" };
// Access the elements using foreach (loop) statement
foreach (string fruit in fruitsName)
{
Console.WriteLine(fruit );
}
Console.ReadKey();
}
//Output:
// Apple
// Orange
// Banana
// Grapes
}
}
When comparing the for loop and the foreach loop, you’ll notice that the foreach method is easier to code, requires no counter (since it uses the Length property), and is more readable.
// Access the ayyay's elements using for loop statement
for (int i = 0; i < fruitsName.Length; i++)
{
Console.WriteLine(fruitsName[i]);
}
In the above line of code, the Length
property of the array provides the size of the array.
C# Array Operations using the Linq methods
The System.Linq namespace in C# contains several methods for performing various operations on an array. For example, Max()
, Min()
, Sum()
, reverse()
, Count()
, Average()
, and so on.
using System;
using System.Linq;
namespace CSharpArrayExample
{
public class CSharpArrayLinqExample
{
static void Main(string[] args)
{
// Declaration and initialization of an Array.
int[] numbersArray = new int[5] { 10, 20, 30, 40, 50 };
Console.WriteLine($" Min: { numbersArray.Min()}");
Console.WriteLine($" Max: { numbersArray.Max()}");
Console.WriteLine($" Sum: { numbersArray.Sum()}");
Console.WriteLine($" Count: {numbersArray.Count()}");
Array.Reverse(numbersArray);
Console.WriteLine($" Reverse: {String.Join(',', numbersArray)}");
Console.ReadKey();
}
// Array Result:
// Min: 10
// Max: 50
// Sum: 150
// Count: 5
// Reverse: 50,40,30,20,10
}
}
C# Array Types
The following are the three types of arrays that you can use in C# programming.
- Single Dimensional Array
- Multidimensional Array
- Jagged Array
Single Dimensional Array
A one-dimensional array, also known as a single-dimensional array in C#, is the most basic type of array, with only one row for data storage. It has only a single pair of square brackets (“[]”). The elements of a One-Dimensional Array are stored linearly and can be accessed individually by providing the index value of each array element.
The following code can be used to declare a single-dimensional array of 5 integers.
// Declaration and initialization of a single dimensional array.
int[] integerArray = new int[5] { 10, 20, 30, 40, 50 };
// Access first element of the array
Console.WriteLine(integerArray[0]);
The array above is single-dimensional, with 5 elements in a single row.
Multidimensional Array
In C#, a multi-dimensional array is an array with multiple rows for storing data. The multi-dimensional array is also a rectangular array because each row has the same length. It can be a 2D array, a 3D array, or a multi-dimensional array. it has more than one comma (,) Within single rectangular brackets [,,]
. You must use a nested loop in the program to store and access the items of a multidimensional array.
The following example will demonstrate the concept of a multidimensional array.
// Example of multi-dimensional Array.
int[,] 2dArray; // two-dimensional array
int[, ,] 3dArray; // three-dimensional array
int[, , ,] 4dArrray ; // four-dimensional array
Note: Each element of a multidimensional array is an array itself.
// An example of a two-dimensional array with two rows and three columns.
int[,] array2d = new int[2,3]{
{10, 20 ,30},
{40, 50, 60}
};
// or
int[,] array2d = {
{10, 20 ,30},
{40, 50, 60}
};
array2d[0, 0]; //returns 10
array2d[0, 1]; //returns 20
array2d[0, 2]; //returns 30
array2d[1, 0]; //returns 40
array2d[1, 1]; //returns 50
array2d[1, 2]; //returns 60
In the above code example, the array2d
is a multidimensional array [2, 3]
defines the no of rows and columns. It has two elements: {10, 20 ,30}
and {40, 50,60}
.
The first rank indicates the number of rows, while the second rank indicates the number of columns. Here, each element of the array is also an array with three elements.
in the example above, The value of a two-dimensional array can be retrieved by row and column index numbers as [row index, column index]
. As a result, [0, 0]
returns the first row and first column values, while [1, 1]
returns the second row and second column value.
Print a multidimensional array using loop in C#
The for and foreach statement provides a way to iterate over the elements of a multidimensional array. The following example shows how to use for and foreach statement to print the multidimensional arrays in C#.
using System;
using System.Linq;
namespace CSharpArrayExample
{
public class CSharp2DArrayExample
{
static void Main(string[] args)
{
// Example: 2D array
int[,] array2d = new int[2, 3]{
{10, 20 ,30},
{40, 50, 60}
};
// Print 2D array's elements using nested loop
Console.WriteLine(" Print 2D array's elements using nested for loop");
for (int i = 0; i < array2d.GetLength(0); i++)
for (int j = 0; j < array2d.GetLength(1); j++)
// or
// for (int i = 0; i < 2; i++)
// for (int j = 0; j < 3; j++)
Console.Write(array2d[i, j] + " ");
Console.WriteLine("\n Print 2D array's elements using foreach loop");
foreach (int i in array2d)
{
Console.Write($" {i}");
}
Console.ReadKey();
}
// Output:
// Print 2D array's elements using nested for loop
// 10 20 30 40 50 60
// Print 2D array's elements using foreach loop
// 10 20 30 40 50 60
}
}
Jagged Arrays
In C#, a jagged array is also called an “array of arrays” because its elements are arrays.
A jagged array consists of multiple arrays as its elements. However, unlike multidimensional arrays, each array within a jagged array can have a different size.
Let’s look at an example of declaring a jagged array that has three elements.
using System;
using System.Linq;
namespace CSharpArrayExample
{
public class CSharpJaggedArrayExample
{
static void Main(string[] args)
{
// declaring jagged array of the integer type
int[][] jaggedArray = new int[3][];
// initializing each array
jaggedArray[0] = new int[] { 5, 10, 15 };
jaggedArray[1] = new int[] { 20, 25 };
jaggedArray[2] = new int[] { 3, 9 };
// print elements of jagged array
Console.WriteLine($"jaggedArray[0][0]: {jaggedArray[0][0]}");
Console.WriteLine($"jaggedArray[0][1]: {jaggedArray[0][1]}");
Console.WriteLine($"jaggedArray[0][2]: {jaggedArray[0][2]}");
Console.WriteLine($"jaggedArray[1][0]: {jaggedArray[1][0]}");
Console.WriteLine($"jaggedArray[1][1]: {jaggedArray[1][1]}");
Console.WriteLine($"jaggedArray[2][0]: {jaggedArray[2][0]}");
Console.WriteLine($"jaggedArray[2][1]: {jaggedArray[2][1]}");
Console.ReadKey();
}
// Jagged Arrays result:
// jaggedArray[0][0]: 5
// jaggedArray[0][1]: 10
// jaggedArray[0][2]: 15
// jaggedArray[1][0]: 20
// jaggedArray[1][1]: 25
// jaggedArray[2][0]: 3
// jaggedArray[2][1]: 9
}
}
FAQ
Q: What’s the difference between a list and an array?
Ans: Array can store elements of only one data type but a List can store the elements of different data types too. Hence, the Array stores homogeneous data values, and the list can store heterogeneous data values.
Q: Why are arrays more performant than collections?
Ans: Arrays run faster, which consumes more memory and improves performance. Collections, on the other hand, consume less memory but have lower performance than arrays.
Q: What are the advantages of using arrays?
Ans: In an array, elements can be randomly accessed via index number. The array allocates storage in contiguous locations for all its elements. Therefore, the array is not likely to allocate additional memory. This avoids array memory overflows and memory shortages.
Summary:
In this article, we covered the basics of arrays and how to use arrays in C# such as storing and retrieving the values ​​from an array. At the beginning of this article, we discussed the Array class, and then we went through the different types of arrays, such as single-dimension, multi-dimension, and jagged arrays. We also learn how to work with arrays using different methods and properties of the Array class.
Thank you for reading the blog; if you enjoyed it, please like, comment, and share it with others.
Articles to Check Out:
- C# Array vs List: When should you use an array or a List?
- C# Hashtable Vs Dictionary
- C# Abstract class Vs Interface
- WCF vs WEB API
- Serialization and Deserialization in C# with Example
- Top 50 C# Interview Questions And Answers For Freshers And Experienced
- Collections in C# .NET
- Abstraction vs Encapsulation in C#: Difference between abstraction and encapsulation
- C# Struct vs Class
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025