In this post, we’ll look at a simple but difficult interview question in C#: Call By Value vs Call By Reference.
This is a question that both fresher and experienced developers are asked in interviews. It’s one of the most frequently asked questions by technical interviewers.
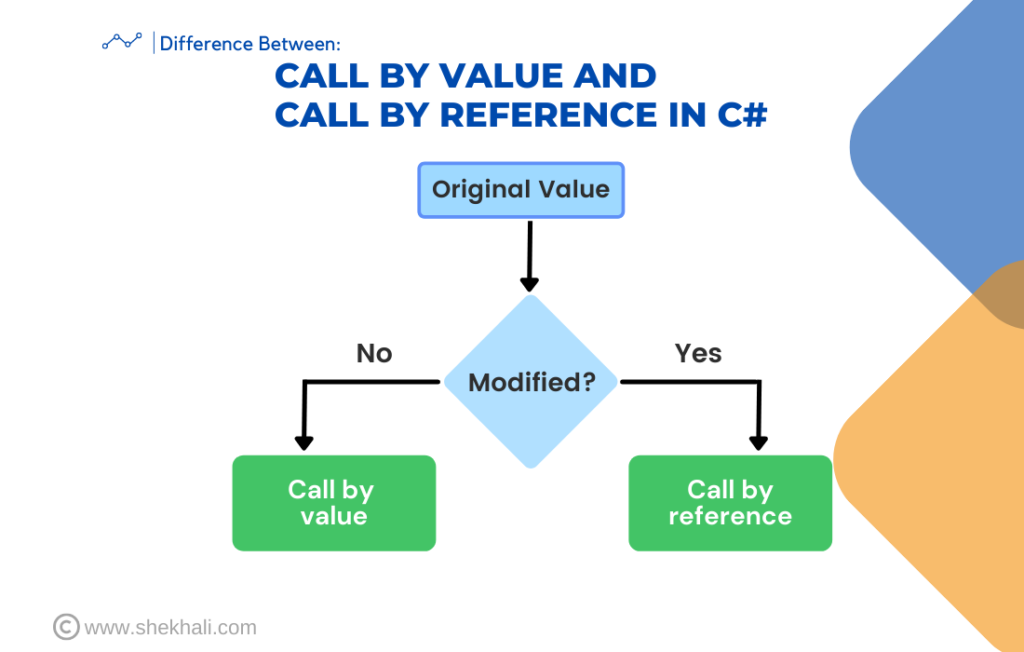
In C#, arguments can be passed to the method parameters either by value or by reference. It’s important to remember that C# types can be reference types (like classes, string, interface) or value types (like structs, int).
Pass by Value means sending a copy of the variable’s value to the method.
This means that any modifications made to the parameter within the method won’t affect the original variable outside of it.
Value types, like int, float, and structs, are typically passed by value.
Pass by Reference means you’re providing the method with direct access to the original variable.
This means that any changes made to the parameter within the method will directly impact the original variable outside of it.
Reference types, such as classes, are commonly passed by reference.
In C#, you explicitly specify whether you’re passing an argument by reference using the ref
or out
keywords. This can be helpful when you want to modify the original value within a method and have those changes reflected outside the method.
It’s important to remember that in C#, all objects are passed by value by default, not by reference, regardless of whether they’re Value Types or Reference Types.
Table of Contents
- 1 What is call by Reference in C#?
- 2 What is call by Value in C#?
- 3 Call by Value VS Call by Reference
- 4 What is the Difference between Call by Value and Call by Reference in C#?
- 5 FAQs
- 5.1 What is the meaning of the term “call by value” in C#?
- 5.2 Can you explain what the term “call by reference” means in C#?
- 5.3 What is the difference between call by value and call by reference?
- 5.4 Q: What are the advantages of using Call by Reference in C#?
- 5.5 Q: When is Call by Value used in C#?
- 5.6 Related
What is call by Reference in C#?
In the call-by-reference mechanism, instead of passing a copy of the actual value the address or reference of a variable is passed as a function parameter.
This means any changes made to the passed values are permanent and directly impact the original variable.
In C#, to specify a reference type parameter, you must use the ref
keyword during both the parameter declaration and the method call.
The ref
keyword is useful for passing the function a reference to the arguments rather than duplicating the actual value.
This approach is particularly useful when you want changes made within a function to reflect in the original variable outside the function.
C# Pass By Reference Example:
The following is the C# code example demonstrating call-by-reference using ref keyword.
using System;
namespace CallByReferenceExample
{
class Program
{
// Define a method that takes an integer by reference using ref keyword
static void ModifyValue(ref int number)
{
// Multiply the original value by 2
number *= 2;
}
static void Main(string[] args)
{
// The variable must be initialized first in case of ref keyword.
int originalValue = 10;
// Display the original value
Console.WriteLine("Original Value: " + originalValue);
// Call the ModifyValue method by reference
ModifyValue(ref originalValue);
// Display the modified value
Console.WriteLine("Modified Value: " + originalValue);
// Pause execution to see the result
Console.ReadLine();
}
}
}
Output:
Original Value: 10
Modified Value: 20
Code Explanation:
- We define a
ModifyValue
method that takes an integer argument by reference using theref
keyword. This means any changes made to thenumber
parameter inside the method will directly affect the original variable passed to it. - In the
Main
method, we initialize an integer variableoriginalValue
with the value10
. - We display the original value using
Console.WriteLine
. - We call the
ModifyValue
method and passoriginalValue
as an argument by reference usingref originalValue
. This allows the method to modify the original value directly. - After calling the method, we display the modified value, which is now doubled.
Note: To use the ref
argument in a C# program, it is important to explicitly include the ref
keyword in both the method declaration and the method call.
What is call by Value in C#?
Call by value in C# is a parameter-passing mechanism where the values of the arguments or parameters are copied and passed to a method or function.
In call by value, any changes made to the parameter values within the method do not affect the original values of the variables used as arguments.
It means that modifications inside the method are isolated and don’t impact the external variables.
Note: The Value-Type variables will store the value of the variable directly in memory, whereas Reference-Type variables will save a reference to the data.
C# Call By Value Example:
using System;
namespace CallByValueExample
{
class Program
{
// Pass by value example
public static void IncrementValue(int i)
{
i ++;
}
static void Main(string[] args)
{
int x = 100;
IncrementValue(x);
Console.WriteLine(x);
Console.ReadKey();
// Output: 100
}
}
}
As shown in the above result, the changes made inside of the method have not affected the original value of the variable.
Note: When you intend to use pass-by-reference, you must use either the
out
orref
keyword, irrespective of whether the argument type is a value type or a reference type.In such cases, because the parameter shares the same storage location as the argument, any modifications to the parameter within the method will directly affect the original variable.
Call by Value VS Call by Reference
here’s a comparison table with your provided information, rephrased for clarity:
Aspect | Call By Value | Call By Reference |
---|---|---|
1. Mechanism: | Call by value copies the variable value to the method. | Call by reference passes the variable reference address to the method. |
2. Behavior: | Call by value copies parameter value into the function, no impact on the parameter. | Argument and parameter share the same address, changes affect the parameter. |
3. Original Value: | In Call by value the original value remains unchanged. | In Call by reference the original value is modified. |
4. Variable Change: | It doesn’t allow altering actual variables through function calls. | It allows altering actual variables via function calls. |
5. Memory Location: | It has separate memory locations for parameters and arguments. | It has shared memory location for parameters and arguments. |
6. Keyword Usage: | Call by value doesn’t require ref or out keyword. | Call by reference requires ref or out keyword for call-by-reference. |
Read more about ref and out keyword in C#.
What is the Difference between Call by Value and Call by Reference in C#?
In C#, the key difference between Call by Value and Call by Reference lies in how method arguments are passed:
- Call by Value:
- In Call by value, the values of arguments are copied and passed to the method.
- Any changes made to these copies within the method do not affect the original variables used as arguments.
- It’s the default method of passing parameters in C#.
- Call by Reference:
- In Call by reference, the actual variables or their reference addresses are passed to the method.
- Any modifications made to these arguments within the method directly affect the original variables outside the method.
- Call by Reference requires the use of
ref
orout
keywords to indicate that the method will operate on the actual variables rather than copies.
FAQs
What is the meaning of the term “call by value” in C#?
In the call-by-value method, the function receives copies of variables as its formal parameters. Therefore, any modifications made to these parameters within the function do not impact the values of the original arguments or actual variables outside the function.
Can you explain what the term “call by reference” means in C#?
When passing arguments to a function by reference, the function receives the actual address of the argument in its formal parameter.
This address is used within the function to access the original parameter used in the call. Therefore, any modifications to the parameter within the function directly affect the passed argument or the actual variable’s value outside of the function.
What is the difference between call by value and call by reference?
The main difference between Call By Value and Call By Reference is that, in Call By Reference, the address or reference of an argument is copied into the function’s formal parameter, while in Call By Value, separate copies of the variable’s value are passed to the formal parameter of the function.
Q: What are the advantages of using Call by Reference in C#?
Call by Reference allows methods to directly manipulate original variables and reducing memory usage as no additional copies are created. It is useful for functions that need to return multiple values or modify input variables.
Q: When is Call by Value used in C#?
Call by Value is used by default in C# when passing arguments to methods. It is suitable when you want to work with copies of values and prevent changes to the original variables.
References: MSDN-Passing Parameters (C# Programming Guide) | Call by Value and Call by Reference
Thank you for taking the time to read the blog; if you found it useful, please leave a comment and share it with others.
Articles to Check Out:
- Difference between Ref and Out keywords in C#
- C# Abstract class Vs Interface
- C# Struct vs Class
- Static vs Singleton in C#: Understanding the Key Differences
- Top 50 C# Interview Questions And Answers For Freshers And Experienced
- C# 10 new features
- Understanding Covariance And Contravariance in C#
- C# StreamReader and StreamWriter Example
- C# Tutorial
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024