Here, we have a list of 50 + commonly asked JavaScript interview questions, simplified answers, and code examples. It will help you prepare for your interview. Let’s get started:
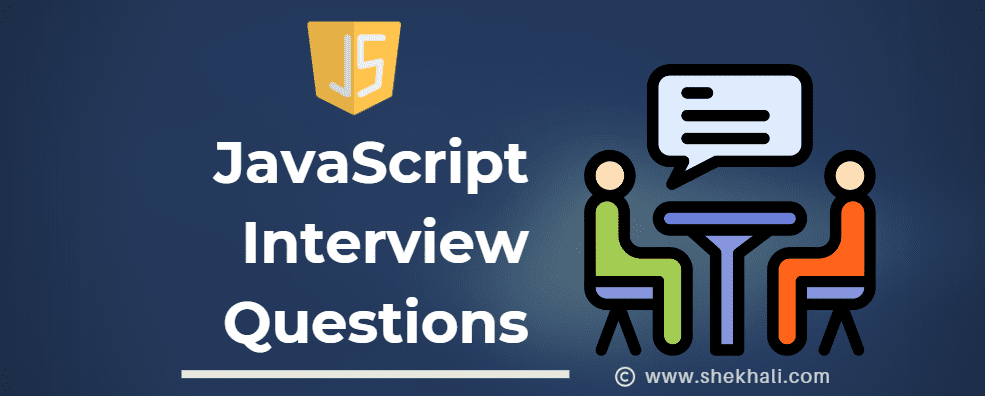
Table of Contents
- 0.1 1. What is JavaScript?
- 0.2 2. What are the data types in JavaScript?
- 0.3 3. What are the differences between let, const, and var in JavaScript?
- 0.4 4. What is the difference between null and undefined?
- 0.5 5. Explain the concept of hoisting in JavaScript.
- 0.6 6. What is a closure in JavaScript?
- 0.7 7. How does JavaScript handle asynchronous programming?
- 0.8 8. Explain the difference between synchronous and asynchronous code execution.
- 0.9 9. What is a callback function in JavaScript?
- 0.10 10. Explain Promises in JavaScript.
- 0.11 11. What is the purpose of the async keyword in JavaScript?
- 0.12 12. Explain await in JavaScript.
- 0.13 13. How do you handle errors in asynchronous code using Promises?
- 0.14 14. Explain the concept of prototypal inheritance in JavaScript.
- 0.15 15. How do you create an object in JavaScript?
- 0.16 16. How do you check if an object has a specific property?
- 0.17 17. What is the purpose of the this keyword in JavaScript?
- 0.18 18. Explain the difference between == and === in JavaScript.
- 0.19 19. What is DOM? What is the use of a document object?
- 0.20 20. Explain the purpose of the map() method in JavaScript.
- 0.21 21. How do you remove an item from an array in JavaScript?
- 0.22 22. Explain the concept of event delegation in JavaScript.
- 0.23 23. How can you prevent the default behavior of an event in JavaScript?
- 0.24 24. What does the isNaN() function?
- 0.25 25. How do you check if a variable is an array in JavaScript?
- 0.26 26. Explain the purpose of the reduce() method in JavaScript.
- 0.27 27. Explain the concept of the “event loop” in JavaScript.
- 0.28 28. How do you convert a string to a number in JavaScript?
- 0.29 29. Explain the purpose of the filter() method in JavaScript.
- 0.30 30. How do you reverse a string in JavaScript?
- 0.31 31. What are arrow functions in JavaScript?
- 0.32 32. Explain Implicit Type Coercion in JavaScript.
- 0.33 33. How do you clone an object in JavaScript?
- 0.34 34. Explain the purpose of the forEach() method in JavaScript.
- 0.35 35. How do you check if a variable is an object in JavaScript?
- 0.36 36. Explain the purpose of the push() method in JavaScript.
- 0.37 37. Explain the purpose of the charAt() method in JavaScript.
- 0.38 38. What is the difference between localStorage and sessionStorage in JavaScript?
- 0.39 39. Explain the purpose of the findIndex() method in JavaScript.
- 0.40 40. How do you convert a number to a string with a specific number of decimal places in JavaScript?
- 0.41 41. Explain the purpose of the Object.assign() method in JavaScript.
- 0.42 42. How do you check if an object is empty in JavaScript?
- 0.43 43. How do you convert a string to uppercase or lowercase in JavaScript?
- 0.44 44. What is the purpose of the concat() method in JavaScript?
- 0.45 45. How do you remove a property from an object in JavaScript?
- 0.46 46. Explain call(), apply() and, bind() methods.
- 0.47 47. What is currying in JavaScript?
- 0.48 48. What are the different pop-up boxes available in JavaScript?
- 0.49 49. How to change the background color of HTML document using JavaScript?
- 0.50 50. How you can validate a form in JavaScript?
- 0.51 51. What are the advantages of JavaScript?
- 1 52. What is Object Destructuring in JavaScript?
- 2 Summary
1. What is JavaScript?
JavaScript is a widely used scripting language for building interactive and dynamic web applications. It facilitates interaction with visitors and executes complex actions, updating content, controlling multimedia, animated graphics, and much more.
2. What are the data types in JavaScript?
In JavaScript, there are several data types including:
- Number: Represents numeric values, like integers or floating-point numbers. Example:
age
. - String: Represents textual data enclosed in single or double quotes. Example:
name
. - Boolean: Represents true or false values. Example:
isStudent
. - Object: Represents a collection of key-value pairs, where the keys are strings (properties) and the values can be of any data type. Example:
person
. - Array: Represents an ordered collection of elements, which can be of mixed data types. Example:
fruits
. - Undefined: Represents a variable that has been declared but hasn’t been assigned a value. Example:
x
. - Null: Represents the intentional absence of value. Example:
y
. - Symbol: Represents a unique and immutable value often used as an object property identifier. Example:
uniqueSymbol
.
Each data type serves a specific purpose and is used to store and manipulate different kinds of information in JavaScript.
// Number
let age = 25;
// String
let name = "John";
// Boolean
let isStudent = true;
// Object
let person = {
firstName: "Shekh",
lastName: "Ali",
age: 25
};
// Array
let fruits = ["apple", "banana", "orange", "mango"];
// Undefined
let x;
// Null
let y = null;
// Symbol
const uniqueSymbol = Symbol("unique");
3. What are the differences between let, const, and var in JavaScript?
var
has function scope, while let
and const have block scope. var
is hoisted to the top of its function scope, while let
and const
are hoisted to the top of their block scope. const
is used for variables that should not be reassigned, while let
allows reassignment.
function exampleScope() {
if (true) {
var x = "var"; // Function-scoped
let y = "let"; // Block-scoped
const z = "const"; // Block-scoped
}
console.log(x); // Outputs: "var"
console.log(y); // Error: y is not defined
console.log(z); // Error: z is not defined
}
exampleScope();
4. What is the difference between null
and undefined
?
null
is an intentional absence of any object value, while undefined
indicates a variable has been declared but has not yet been assigned a value.
let x;
console.log(x); // Outputs: undefined
const y = null;
console.log(y); // Outputs: null
5. Explain the concept of hoisting in JavaScript.
Hoisting is a JavaScript behavior where variable and function declarations are moved to the top of their scope during compilation, irrespective of where the functions and variables are declared.
hoistingVariable = 100;
console.log(hoistingVariable); // output: 100
var hoistingVariable;
6. What is a closure in JavaScript?
A closure is a function that has access to its own scope, the scope of the outer function, and the global scope. It is an ability of a function to remember the variables and functions that are declared in its outer or global scope.
Let’s understand closures by the following code example:
var x = 10;
function outer() {
function inner() {
console.log(x);
}
return inner;
}
var closureFunc = outer();
closureFunc(); // Outputs '10'
7. How does JavaScript handle asynchronous programming?
JavaScript uses callbacks, Promises, and async/await to manage asynchronous operations.
8. Explain the difference between synchronous and asynchronous code execution.
Synchronous code is executed line by line, blocking further execution until a task is complete. Asynchronous code allows other tasks to run while waiting for a non-blocking operation to finish.
9. What is a callback function in JavaScript?
A callback function is a function passed as an argument to another function to be executed later. They can be used as an argument inside another function, can be returned by the function, and can even be used as a property of an object.
Here is an example of a callback function:
function fetchData(callback) {
// Simulate fetching data
setTimeout(function() {
const data = "Some data";
callback(data);
}, 1000);
}
function processData(data) {
console.log("Processed data:", data);
}
// Passing one function as an argument to another function.
fetchData(processData);// Output: Processed data: Some data
10. Explain Promises in JavaScript.
Promises are used to handle asynchronous operations like a server request in a more organized and readable way. They provide a structure to manage callbacks, making it easier to handle success and error scenarios in asynchronous tasks.
To instantiate a promise, the Promise constructor is used, which accepts a callback function with two parameters: resolve and reject respectively.
Promise Object has four states:
- Pending: Initial state when the Promise is created.
- Fulfilled: The state when the asynchronous operation is completed successfully.
- Rejected: The state when the asynchronous operation encounters an error.
- Settled: The Promise is either fulfilled or rejected, but not pending anymore.
Resolve and Reject:
- Resolve: Resolve is a function used inside a Promise to indicate successful completion of the asynchronous task.
- Reject: Reject is a function used inside a Promise that indicates an error or failure in the asynchronous task.
// Creating a Promise
const promise = new Promise(function(resolve, reject) {
// Simulate an asynchronous operation
setTimeout(function() {
const success = true;
const data = { id: 1, name: 'Shekh Ali' };
if (success) {
resolve(data);
} else {
reject("Error occurred");
}
}, 2000);
});
// Consuming the Promise
promise
.then(function(data) {
console.log("Success:", data);
})
.catch(function(error) {
console.log("Error:", error);
});
// Output after 2 seconds (if resolved):
// Success: { id: 1, name: 'Shekh Ali' }
11. What is the purpose of the async keyword in JavaScript?
The async keyword is used to define an asynchronous function, which always returns a Promise.
async function fetchData(){
const response = await getData();
const data = response;//response.json();
return data;
};
function getData()
{
return {"id":1,"name":"shekh ali","age":29};
}
fetchData().then(function(data){
console.log("fetchedData : ",data);
});
// Output:
// fetchedData : { id: 1, name: 'shekh ali', age: 29 }
12. Explain await in JavaScript.
The await keyword is used to pause the execution of an async function until the Promise is resolved, and it returns the resolved value.
13. How do you handle errors in asynchronous code using Promises?
You can use the catch()
method or try/catch
inside an async
function to handle errors in asynchronous code.
async function fetchData() {
try {
const response = await fetch("https://api.example.com/data");
const data = await response.json();
return data;
} catch (error) {
console.log("Error fetching data:", error);
throw error;
}
}
fetchData()
.then(function(data) {
console.log("Fetched data:", data);
})
.catch(function(error) {
console.log("Error:", error);
});
14. Explain the concept of prototypal inheritance in JavaScript.
Prototypal inheritance is a mechanism where an object inherits properties and methods from another object.
function Person(name) {
this.name = name;
}
Person.prototype.greet = function() {
console.log(`Hello, my name is ${this.name}`);
};
const person = new Person("Shekh Ali");
person.greet(); // Outputs: "Hello, my name is Shekh Ali"
15. How do you create an object in JavaScript?
Objects can be created using object literals, constructor functions, or the class
syntax (ES6).
// Object literal
const person = {
name: "John",
age: 30,
};
// Constructor function
function Person(name, age) {
this.name = name;
this.age = age;
}
const person1 = new Person("Amit", 30);
// ES6 class
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
}
const person2 = new Person("John", 30);
16. How do you check if an object has a specific property?
You can use the hasOwnProperty()
method to check if an object has a specific property.
const person = {
name: "Lisa",
age: 20,
};
console.log(person.hasOwnProperty("name")); // Outputs: true
console.log(person.hasOwnProperty("gender")); // Outputs: false
17. What is the purpose of the this
keyword in JavaScript?
The this
keyword refers to the current object, which can vary depending on how a function is called.
const person = {
name: "Shekh Ali",
greet: function() {
console.log(`Hello, my name is ${this.name}`);
},
};
person.greet(); // Outputs: "Hello, my name is Shekh Ali"
18. Explain the difference between ==
and ===
in JavaScript.
==
is a loose equality operator that checks for equality after converting operands to the same type. ===
is a strict equality operator that checks for equality without type conversion.
console.log(5 == "5"); // Outputs: true
console.log(5 === "5"); // Outputs: false
19. What is DOM? What is the use of a document object?
DOM stands for Document Object Model. It’s a programming interface provided by web browsers that represent the structure of an HTML or XML document. It creates a structured representation of the document’s elements, allowing scripts to manipulate and interact with the content and structure of a web page.
Use of Document Object: The document object is a core part of the DOM. It represents the entire web page and provides methods and properties to access and modify the content and structure of the document.
Example: Suppose you have an HTML document like this:
// Accessing an element by its ID and changing its content
const headingElement = document.getElementById("myHeading");
headingElement.textContent = "Hello, DOM!";
// Creating a new element and adding it to the document
const newParagraph = document.createElement("p");
newParagraph.textContent = "This is a new paragraph.";
document.body.appendChild(newParagraph);
// Modifying CSS styles of an element
const styledElement = document.querySelector(".styled");
styledElement.style.color = "blue";
// Adding an event listener to an element
const buttonElement = document.getElementById("myButton");
buttonElement.addEventListener("click", () => {
alert("Button clicked!");
});
20. Explain the purpose of the map()
method in JavaScript.
The map()
method is used to create a new array by applying a function to each element of the original array.
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map(function(num) {
return num * 2;
});
console.log(doubledNumbers); // Outputs: [2, 4, 6, 8, 10]
21. How do you remove an item from an array in JavaScript?
You can use methods like splice()
, filter()
, or pop()
to remove items from an array.
const numbers = [1, 2, 3, 4, 5];
// Using splice
numbers.splice(2, 1); // Removes the element at index 2
console.log(numbers); // Outputs: [1, 2, 4, 5]
// Using filter
const filteredNumbers = numbers.filter(function(num) {
return num !== 2;
});
console.log(filteredNumbers); // Outputs: [1, 4, 5]
// Using pop
numbers.pop(); // Removes the last element
console.log(numbers); // Outputs: [1, 2, 4]
22. Explain the concept of event delegation in JavaScript.
Event delegation is a technique where you attach a single event listener to a parent element to handle events for all its child elements.
23. How can you prevent the default behavior of an event in JavaScript?
You can use the preventDefault()
method to prevent the default behavior of an event, such as preventing a link from navigating to a new page.
document.getElementById("myLink").addEventListener("click", function(event) {
event.preventDefault();
console.log("Link clicked, but default behavior prevented");
});
24. What does the isNaN() function?
The isNaN()
function in JavaScript is used to determine whether a given value is “Not-A-Number” (NaN) or not. It returns true
if the value is NaN, and false
if the value is a valid number or can be converted to one.
console.log(isNaN(42)); // Output: false (42 is a valid number)
console.log(isNaN("Hello")); // Output: true ("Hello" can't be converted to a number)
console.log(isNaN(NaN)); // Output: true (NaN is not a valid number)
console.log(isNaN(undefined)); // Output: true (undefined can't be converted to a number)
console.log(isNaN(null)); // Output: false (null is treated as 0 when converting to a number)
25. How do you check if a variable is an array in JavaScript?
You can use the Array.isArray()
method to check if a variable is an array.
const arr = [1, 2, 3];
console.log(Array.isArray(arr)); // Outputs: true
const notArr = "Not an array";
console.log(Array.isArray(notArr)); // Outputs: false
26. Explain the purpose of the reduce()
method in JavaScript.
The reduce()
method is used to reduce an array to a single value by applying a function to each element, accumulating the result.
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce(function(acc, num) {
return acc + num;
}, 0);
console.log(sum); // Outputs: 15
27. Explain the concept of the “event loop” in JavaScript.
The event loop is a core concept in JavaScript that handles asynchronous operations by executing callback functions in a non-blocking way.
console.log("Start");
setTimeout(function() {
console.log("Async operation done");
}, 1000);
console.log("End");
28. How do you convert a string to a number in JavaScript?
You can use the parseInt()
or parseFloat()
functions to convert a string to a number.
const str = "42";
const num = parseInt(str);
console.log(num); // Outputs: 42
29. Explain the purpose of the filter()
method in JavaScript.
The filter()
method is used to create a new array by filtering elements from the original array that match a specified condition.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(function(num) {
return num % 2 === 0;
});
console.log(evenNumbers); // Outputs: [2, 4]
30. How do you reverse a string in JavaScript?
- You can use the
split()
,reverse()
, andjoin()
methods to reverse a string.
const str = "Hello, World!";
const reversedStr = str.split("").reverse().join("");
console.log(reversedStr); // Outputs: "!dlroW ,olleH"
- Reverse a String With a Decrementing For Loop.
function reverseString(str) {
var newString = "";
for (var i = str.length - 1; i >= 0; i--) {
newString += str[i];
}
return newString;
}
console.log("Reverse String: ",reverseString('hello'));
// Reverse String: olleh
31. What are arrow functions in JavaScript?
Arrow functions are a concise way to write anonymous functions in JavaScript, with a simpler syntax and a lexical this
binding.
const add = (a, b) => a + b;
console.log(add(5, 3)); // Outputs: 8
32. Explain Implicit Type Coercion in JavaScript.
Implicit type coercion in JavaScript refers to automatically converting one data type to another during operations without the programmer specifying the conversion explicitly.
This can lead to unexpected behavior if not understood properly.
let num = 42; // num is a number
let str = "3"; // str is a string
let result = num + str; // JavaScript implicitly converts num to a string and performs string concatenation
console.log(result); // Output: "423" (num was coerced to a string and concatenated with str)
To return the correct result, we need to explicitly convert the “3” to a number using the Number() method:
let num = 42; // num is a number
let str = "3"; // str is a string
result = num + Number(str);
console.log(result); // Output: 45
33. How do you clone an object in JavaScript?
You can clone an object using techniques like the spread operator or Object.assign()
.
const original = { a: 1, b: 2 };
const clone = { ...original };
console.log(clone); // Outputs: { a: 1, b: 2 }
34. Explain the purpose of the forEach()
method in JavaScript.
The forEach()
method is used to iterate over the elements of an array and execute a function for each element.
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(num) {
console.log(num);
});
35. How do you check if a variable is an object in JavaScript?
You can use the typeof
operator to check if a variable is an object, but it doesn’t differentiate between arrays and objects.
const obj = { a: 1, b: 2 };
console.log(typeof obj === "object"); // Outputs: true
const arr = [1, 2, 3];
console.log(typeof arr === "object"); // Outputs: true
const num = 10;
console.log(typeof num === "object"); // Outputs: false
36. Explain the purpose of the push()
method in JavaScript.
The push()
method is used to add one or more elements to the end of an array and returns the new length of the array.
const numbers = [1, 2, 3];
numbers.push(4);
console.log(numbers); // Outputs: [1, 2, 3, 4]
37. Explain the purpose of the charAt()
method in JavaScript.
The charAt()
method is used to retrieve the character at a specified index in a string.
const str = "Hello, World!";
const char = str.charAt(7);
console.log(char); // Outputs: "W"
38. What is the difference between localStorage and sessionStorage in JavaScript?
Both localStorage and sessionStorage are Web Storage APIs used to store key-value pairs in a web browser, but they have different lifetimes:
- Data stored in localStorage persists even after the browser is closed and is available across different tabs/windows.
- Data stored in sessionStorage is limited to the current session and is not shared between tabs/windows.
// Using localStorage
localStorage.setItem("name", "Shekh");
console.log(localStorage.getItem("name")); // Outputs: "Shekh"
// Using sessionStorage
sessionStorage.setItem("name", "Ram");
console.log(sessionStorage.getItem("name")); // Outputs: "Ram"
39. Explain the purpose of the findIndex()
method in JavaScript.
The findIndex()
method is used to find the index of the first element in an array that satisfies a provided testing function.
const numbers = [10, 20, 30, 40, 50];
const index = numbers.findIndex(function(num) {
return num > 25;
});
console.log(index); // Outputs: 2 (index of 30)
40. How do you convert a number to a string with a specific number of decimal places in JavaScript?
You can use the toFixed()
method to convert a number to a string with a specified number of decimal places.
const num = 3.14159;
const str = num.toFixed(2);
console.log(str); // Outputs: "3.14"
41. Explain the purpose of the Object.assign()
method in JavaScript.
The Object.assign()
method is used to copy the values of all enumerable properties from one or more source objects to a target object.
const target = {};
const source = { a: 1, b: 2 };
Object.assign(target, source);
console.log(target); // Outputs: { a: 1, b: 2 }
42. How do you check if an object is empty in JavaScript?
You can use the Object.keys()
method to check if an object has any keys.
const obj = {};
// Using Object.keys
console.log(Object.keys(obj).length === 0); // Outputs: true
// Using JSON.stringify
console.log(JSON.stringify(obj) === '{}'); // Outputs: true
43. How do you convert a string to uppercase or lowercase in JavaScript?
You can use the toUpperCase()
method to convert a string to uppercase and the toLowerCase()
method to convert it to lowercase.
const str = "Hello, World!";
const upperCaseStr = str.toUpperCase();
const lowerCaseStr = str.toLowerCase();
console.log(upperCaseStr); // Outputs: "HELLO, WORLD!"
console.log(lowerCaseStr); // Outputs: "hello, world!"
44. What is the purpose of the concat()
method in JavaScript?
The concat()
method is used to concatenate arrays and create a new array.
const arr1 = [1, 2, 3];
const arr2 = [4, 5, 6];
const combinedArray = arr1.concat(arr2);
console.log(combinedArray); // Outputs: [1, 2, 3, 4, 5, 6]
45. How do you remove a property from an object in JavaScript?
To remove a property from an object in JavaScript, you can use the delete
keyword followed by the object’s property you want to remove.
Here is an example to illustrate how to remove a property from an object:
// Create an object
const person = {
firstName: "Shekh",
lastName: "Ali",
age: 25
};
console.log("Before:", person); // Output: { firstName: 'Shekh', lastName: 'Ali', age: 25 }
// Remove a property using the delete keyword
delete person.age;
console.log("After:", person); // Output: { firstName: 'Shekh', lastName: 'Ali' }
46. Explain call(), apply() and, bind() methods.
1. call(): The call() method invokes a function immediately with a specified this value and arguments provided individually.
2. apply(): The apply() method is similar to call(), but it accepts arguments as an array or an array-like object.
3. bind(): The bind() method creates a new function with a specified this value and possibly some initial arguments. Unlike call() and apply(), it doesn’t execute the function immediately; it returns a new function that can be called later.
Example:
Suppose you have a simple person
object and a function introduce
:
const person = {
firstName: "Shekh",
lastName: "Ali"
};
function introduce(greeting) {
console.log(`${greeting}, I'm ${this.firstName} ${this.lastName}.`);
}
Using call()
:
introduce.call(person, "Hello"); // Output: Hello, I'm Shekh Ali.
Using apply()
:
introduce.apply(person, ["Hi"]); // Output: Hi, I'm Shekh Ali.
Using bind()
:
const boundIntroduce = introduce.bind(person, "Hey");
boundIntroduce(); // Output: Hey, I'm Shekh Ali.
47. What is currying in JavaScript?
Currying is a technique in JavaScript where a function that takes multiple arguments is transformed into a series of functions that take one argument each. It allows you to partially apply a function by fixing a certain number of arguments, producing a new function that accepts the remaining arguments.
Example of Currying:
// Normal function that takes three arguments
function add(a, b, c) {
return a + b + c;
}
// Curried version of the add function
function curriedAdd(a) {
return function(b) {
return function(c) {
return a + b + c;
};
};
}
// Using the curried function
const result = curriedAdd(1)(2)(3);
console.log(result); // Output: 6
48. What are the different pop-up boxes available in JavaScript?
Alert Box:
- Alert Box displays a message and an “OK” button.
- Used to provide information or notifications.
Example:
alert("This is an alert message.");
Confirm Box:
- Displays a message with “OK” and “Cancel” buttons.
- Used to ask for user confirmation before proceeding.
Example:
const confirmed = confirm("Do you want to continue?");
if (confirmed) {
console.log("User confirmed.");
} else {
console.log("User canceled.");
}
Prompt Box:
- Displays a message with an input field and “OK” and “Cancel” buttons.
- Used to get user input or information.
Example:
const userInput = prompt("Please enter your name:");
if (userInput !== null) {
console.log("User entered:", userInput);
} else {
console.log("User canceled.");
}
49. How to change the background color of HTML document using JavaScript?
To change the background color of an HTML document using JavaScript, you can access the document
object and modify the style
property of the body
element. Here is how you can do it:
// Get the body element
const body = document.body;
// Change the background color
body.style.backgroundColor = "blue"; // You can replace "blue" with any valid color value
50. How you can validate a form in JavaScript?
Here is an example of validating a simple form using JavaScript. In this example, we’ll validate a form with two fields: name and email.
HTML:
<form id="myForm">
<label for="name">Name:</label>
<input type="text" id="name" required>
<label for="email">Email:</label>
<input type="email" id="email" required>
<button type="submit">Submit</button>
</form>
JavaScript:
const form = document.getElementById("myForm");
form.addEventListener("submit", function(event) {
const nameInput = document.getElementById("name");
const emailInput = document.getElementById("email");
if (nameInput.value.trim() === "") {
alert("Please enter your name.");
event.preventDefault(); // Prevent form submission
}
if (emailInput.value.trim() === "") {
alert("Please enter your email.");
event.preventDefault(); // Prevent form submission
}
});
In this example:
- The form element is accessed using
getElementById
. - An event listener is attached to the form’s
submit
event. - Inside the event listener, the values of the name and email fields are checked. If they are empty, an alert is shown, and
event.preventDefault()
is used to prevent the form from being submitted.
51. What are the advantages of JavaScript?
Here are some advantages of JavaScript:
- Easy to Learn: JavaScript has a simple syntax, making it beginner-friendly.
- Runs in Browsers: JavaScript works directly in web browsers, making websites interactive.
- Speed: JavaScript works fast because it’s an “interpreted” language, saving time compared to languages like Java that need compiling.
- Versatile: It can be used for web development, server-side with Node.js, and even mobile apps.
- Dynamic Web Pages: Enables real-time updates without refreshing the whole page.
- Async Programming: Handles multiple tasks at once for a smoother user experience.
- Cross-Platform: JavaScript can run on various operating systems and devices.
- Open Source: Code is freely available, encouraging collaboration and innovation.
- Large Community: Plenty of resources, tutorials, and help available online.
- Libraries/Frameworks: Many tools like React and Angular speed up development.
- Popular Language: Widely used, increasing job opportunities for developers.
52. What is Object Destructuring in JavaScript?
Object destructuring is a feature in JavaScript that allows you to extract individual properties from an object and assign them to variables. It makes working with objects more concise and easier to manage.
Here is an example:
// Object
const person = {
firstName: "Amit",
lastName: "Kumar",
age: 20,
city: "New York"
};
// Destructuring
const { firstName, lastName, age } = person;
console.log(firstName); // Output: Amit
console.log(lastName); // Output: Kumar
console.log(age); // Output: 20
Summary
This list covers a wide range of topics commonly asked in JavaScript interviews. Remember to practice and review the concepts, and feel free to adapt the answers and code examples for your understanding.
Good luck with your interview!
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025