This post includes the top 50 C# interview questions and answers for freshers and experienced professionals. This article will assist you in improving your C# knowledge and evaluating your present C# understanding.
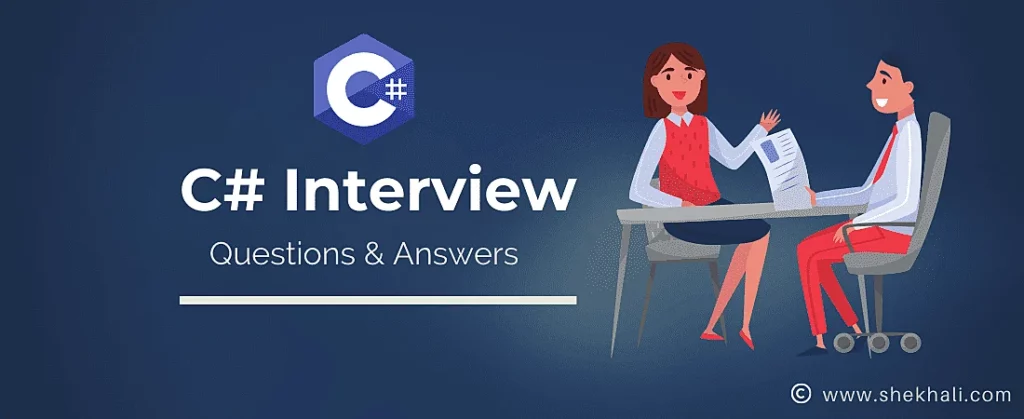
Table of Contents
- 1 Basic C# Interview Questions and Answers
- 2 Q1. What is C#?
- 3 Q2. Can you run multiple catch blocks?
- 4 Q3. What is the difference between a struct and a class in C#?
- 5 Q4. What is a constructor in C#?
- 6 Q5. What is the destructor in C#?
- 7 Q6. Can we use access modifiers with a destructor?
- 8 Q7. What Is Common Language Runtime (CLR) In C#?
- 9 Q8. What is Boxing and Unboxing in C#?
- 10 Q9. What is the purpose of the ‘using’ statement in C#?
- 11 Q10. What are partial classes in C# .NET?
- 12 Advanced C# Interview Questions And Answers
- 13 Q11. What are the differences between string and StringBuilder in C#?
- 14 Q12. What is the difference between Finalize() and Dispose() methods in C#?
- 15 Q13. What is the difference between ref and out keywords?
- 16 Q14. What is the difference between “is” and “as” operator in C# .NET?
- 17 Q15. What is managed and unmanaged code in c#?
- 18 Q16. What are indexers in C# .NET?
- 19 Q17. Can we define a try block with multiple catch blocks in C#?
- 20 Q18. What is the use of ‘using’ statement in C#?
- 21 Q19. What is the difference between the “throw” and “throw ex” Clause?
- 22 Q20. What is the difference between finally and finalize block?
- 23 Q21. What is Serialization in C#?
- 24 Q22. What is the difference between constants and readonly in C#?
- 25 Q23. What is multithreading in C#?
- 26 Q24. What is Reflection in C#?
- 27 Q25. What are Collections in C#?
- 28 Q26. What is Constructor Chaining in C#?
- 29 Q27. What is monitor class in C#?
- 30 Q28. What are generics in C#.NET?
- 31 Q29. Explain Access Modifiers in C#?
- 32 Q30. Can you declare struct members as protected or protected internal in C#?
- 33 Q31. What is the difference between static class and singleton pattern?
- 34 Q32. What exactly is the anonymous type in C#?
- 35 Q33. Define sealed classes in C#?
- 36 Q34. What is a multicast delegate in C#?
- 37 Q35. What are generic delegates in C#?
- 38 Q36. What is the difference between Array and Arraylist in C#?
- 39 Q37. What is an extension method in C#?
- 40 Q38. Why this keyword is used in c#?
- 41 Q39. Can we use this keyword in a static method in C#?
- 42 Q40. What is the difference between an interface and an abstract class?
- 43 Q41. What is the difference between late binding and early binding in C#?
- 44 Q42. What is a Jagged Array in C#?
- 45 Q43. What is the difference between Continue and Break Statement?
- 46 Q44. What is Thread Pooling in C#?
- 47 Q45. What is the difference between an EXE and a DLL?
- 48 Q46. What is the difference between ‘string’ keyword and ‘System.String’ class?
- 49 Q47. What is the difference between int.Parse and int.TryParse method in C#?
- 50 Q48. Give some examples of value types and reference types
- 51 Q49. What is the volatile keyword in C#?
- 52 Q50. What is an enum (enumeration) in C#?
Basic C# Interview Questions and Answers
Here is a list of C# interview questions and answers for freshers as well as candidates with 5 or 10 years of experience
Q1. What is C#?
C# is a modern, object-oriented, and type-safe programming language developed by Microsoft in the year 2000. C# enables developers to build many types of secure and robust applications including Windows apps, Web apps, Mobile (native iOS and Android apps), Web services, blockchain applications, Artificial intelligence (AI), and machine learning software.
The most recent version of C# is Version 10, which was introduced with Visual Studio 2022. You can learn more about C# 10
here: C# 10 new features
Q2. Can you run multiple catch blocks?
No, you can’t run multiple catch blocks of the same type. Once the correct catch code is executed, control is transferred to the finally block and then the code that follows the finally block will get executed.
Q3. What is the difference between a struct and a class in C#?
The following are the main differences between the struct and class in C#.
- Struct is a value type inherited from
System.ValueType
whereas Class is a reference type inherited fromSystem.Object
class. - Classes are stored in heap memory, whereas structs are stored on the stack.
- A class can inherit from another class, but a struct cannot inherit from another struct or class except interfaces.
- The structure is implicitly sealed and cannot be a base class, whereas the class can be a base class.
- The class members are private by default, whereas struct members are public.
Check out this article for more information: C# Struct vs Class
Q4. What is a constructor in C#?
A Constructor is a special method that gets executed when an instance of the class or struct is created. It is used to initialize and set default values for the data members of the new object. The constructor’s name is the same as the class or structure.
The compiler will automatically create a default constructor for the newly created class. Each class required at least one constructor.
The following are five types of constructors available in C#.
- Default Constructor
- Parameterized Constructor
- Copy Constructor
- Static Constructor
- Private Constructor
Check out this article for more information: Types of constructors in C#
Q5. What is the destructor in C#?
A destructor is a class member function that is called automatically to destroy objects of class when the scope of an object ends. A class can have only one destructor, which must be preceded by a tilde ~
and has the same name as the class.
The parameters and access modifiers aren’t allowed in the destructor.
// Class
public class MyClass
{
MyClass()
{
// Constructor
}
~MyClass()
{
// Destructor
}
}
Q6. Can we use access modifiers with a destructor?
Putting an access modifier on the destructor has no meaning, as the Garbage collector (GC) calls the destructor automatically, not by the user-written code.
Q7. What Is Common Language Runtime (CLR) In C#?
The Common Language Runtime (CLR) is an execution engine (Virtual Machine) that provides an environment to manage the execution of programs on a target machine written in any of the.NET Framework’s supported programming languages (for example, C#, VB.NET, F#, and so on).
The JIT compiler, a component of CLR, converts every source code into a common language known as MSIL or Intermediate Language (IL), which is then executed on the computer’s CPU.
The CLR provides essential services, such as memory management, exception handling, type safety, garbage collection, security, and thread management.
To read more, check out this MSDN article: (CLR) overview
The architecture of common language runtime (CLR) in C#
The following diagram represents the architecture of the Common Language Runtime (CLR):
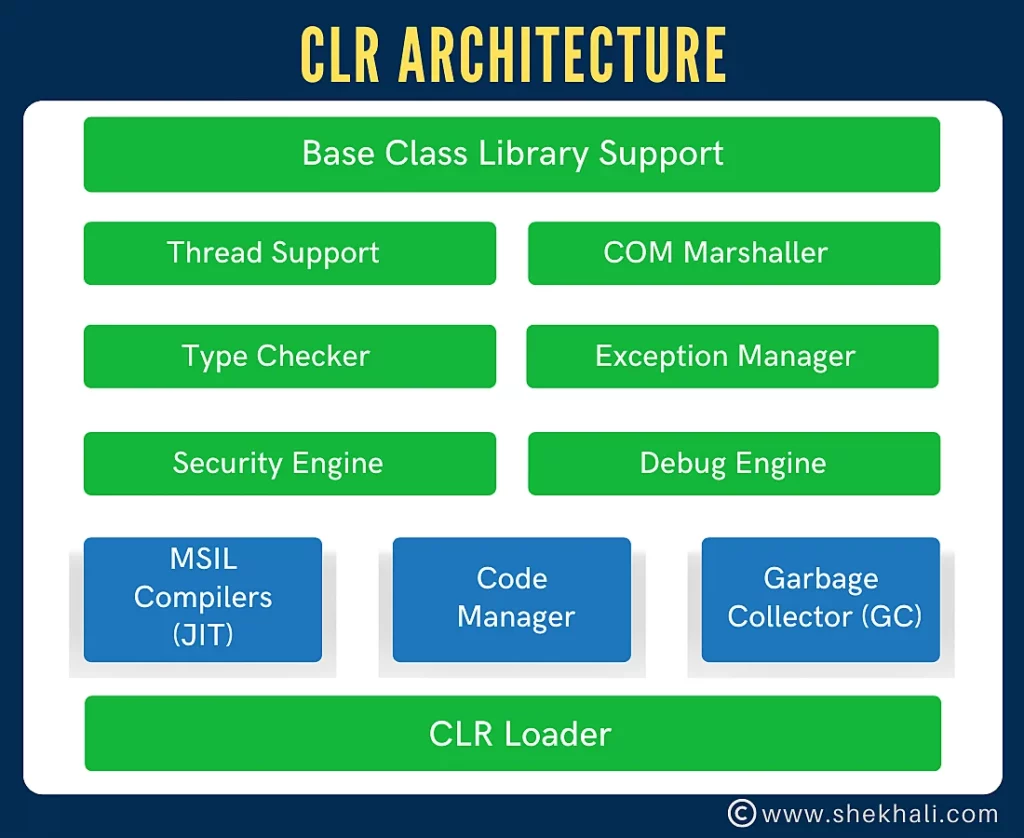
Q8. What is Boxing and Unboxing in C#?
Boxing is the process of converting a value type (int, float, bool, char) to the reference type (object).
It encapsulates the value inside an Object instance and saves it on the managed heap.
Unboxing is the process of converting the reference type (object) into the value type.
Boxing is implicit, whereas unboxing is an explicit conversion process.
//Boxing:
// int 'number' (value type) is created on the Stack
int number = 100;
// The following line boxes int 'number' and stored it on the Heap (reference type).
object obj = number;
The object obj is now unboxed and assigned to the integer variable myNumber:
// Unboxing:
// The existing boxed int value is unboxed from the managed heap and assigned to int
// myNumber, which is then stored on the stack.
int myNumber = (int)obj;
Read more about boxing and unboxing
Q9. What is the purpose of the ‘using’ statement in C#?
Using statements in C# is a simple technique to automatically dispose of the objects that implement the IDisposable interface in order to free up resources that garbage collection cannot handle automatically.
Q10. What are partial classes in C# .NET?
C# has a unique feature called a partial class. It has the unique capability of breaking out the functionality of a single class into many files, which are then reassembled into a single class file during compilation.
The partial class allows you to segregate UI design and business logic codes, making it easier to read and understand. (For example, both Default.aspx.cs
and Default.designer.cs
 files in asp.net contain the same class name with the partial keyword, and after compilation, they merge into a single file.)
Advanced C# Interview Questions And Answers
Q11. What are the differences between string and StringBuilder in C#?
The string is an object of the System.String
type. It is immutable (unchangeable), meaning the value once assigned to a String object cannot be changed after its creation.
If we try to update the value of the String object, it will create a new instance with the changed value in a different memory address.
The StringBuilder is a dynamic object that belongs to the System.Text
namespace. It is mutable and doesn’t create a new object in the heap memory whenever a new string is concatenated.
To read more, check out this article: C# String VS StringBuilder
Q12. What is the difference between Finalize() and Dispose() methods in C#?
The main difference between dispose()
and finalize()
is that the dispose() method belongs to the IDisposable
Interface and must be called explicitly by the user, whereas the finalize()
method is a member of the Object
class and is called by the garbage collector right before the object is destroyed.
In C#, you can use the destructor to implement Finalize()
.
Read more about dispose() and finalize().
Q13. What is the difference between ref and out keywords?
C# Ref keyword | Out keyword |
---|---|
1. The ref parameters must be initialized with certain values before passing as method arguments.Example: int number = 100; SomeMethod(ref number); | It is not necessary to initialize out parameters before passing them to a method.Example: int number; SomeMethod(out number); |
2. The ref keyword allows data to flow in bi-directions. | The out keyword is used to simply get data in one-way mode only. |
3. A method cannot return multiple values using the ref. | The out keyword comes in handy when a function wants to return many values. |
Read more about ref and out keywords.
Q14. What is the difference between “is” and “as” operator in C# .NET?
IS Operator: The is
operator determines whether or not an object is compatible with a specific type. If the object is of the same type, it returns true; otherwise, it returns false.
AS Operator: The as
Operator is used to explicitly convert an Object to a given Type or a Class. The as operator returns null if the conversion isn’t possible.
if(obj is Person)
{
// Code here ...
}
// Example: As operator
Person p = obj as Person;
if(p != null)
{
// Code here ...
}
Q15. What is managed and unmanaged code in c#?
In the.NET Framework, managed code is code that is managed by the CLR (Common Language Runtime). Unmanaged code, on the other hand, is unsafe code that is run directly by the operating system.
The other services, such as Garbage collection, type checking, memory allocation, exception handling, and bounds checking, are provided to the managed code by the CLR.
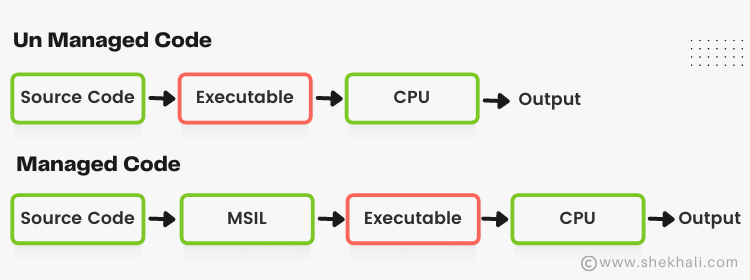
Q16. What are indexers in C# .NET?
Indexer is a smart property in C# that allows an instance of a class or struct to be indexed as a virtual array.
Indexers are defined in the same way as properties but the only difference is that the indexer can be defined using this
keyword and square brackets []
.
Here is an example of C# Indexer:
using System;
class Program
{
static void Main()
{
// Create a simple integer indexer
SimpleIndexer indexer = new SimpleIndexer();
// Set values using the indexer
indexer[0] = 10;
indexer[1] = 20;
indexer[2] = 30;
// Retrieve and print values using the indexer
Console.WriteLine($"Value at index 0: {indexer[0]}");
Console.WriteLine($"Value at index 1: {indexer[1]}");
Console.WriteLine($"Value at index 2: {indexer[2]}");
}
}
// Define a class with a simple integer indexer
class SimpleIndexer
{
private int[] data = new int[3]; // Private data array to store values
// Define the indexer
public int this[int index]
{
get
{
return data[index];
}
set
{
data[index] = value;
}
}
}
Output:
Value at index 0: 10
Value at index 1: 20
Value at index 2: 30
To read more about Indexers, check out this article: Indexers in C#
Q17. Can we define a try block with multiple catch blocks in C#?
Yes, multiple catch blocks can be defined with a single try block in the C# program. The only constraint is that we must specify all derived class exceptions (for example, NullReferenceException
, ArgumentNullException
, IndexOutOfRangeException
, and so on) in the catch block first, and then the base class exception (i.e. Exception) should be defined in the last. It means the catch blocks must be placed in the correct order.
try
{
// try block
}
catch (NullReferenceException ex1)
{
// catch Block 1
}
catch (ArgumentNullException ex2)
{
// catch Block 2
}
catch (IndexOutOfRangeException ex3)
{
// catch Block 3
}
catch (IOException ex4)
{
// catch Block 4
}
catch (Exception exception)
{
// base exception catch block
}
Q18. What is the use of ‘using’ statement in C#?
In C#, the using
keyword has two main purposes:
- Namespace Usage: The using keyword is used to import namespaces. A namespace is a way to organize and group related classes, structs, interfaces, and other types.
using System; // Example: Importing the System namespace
class Program
{
static void Main()
{
Console.WriteLine("Hello, World!"); // Here, Console is in the System namespace
}
}
- Resource Management:Â The
using statement
ensures the proper disposal of resources, such as files, database connections, or other objects that implement theIDisposable
interface.
using (var fileStream = new FileStream("example.txt", FileMode.Open))
{
// Use the fileStream here
} // The fileStream is automatically disposed when the using block exits
Q19. What is the difference between the “throw” and “throw ex” Clause?
- “throw” Clause:Â When you use “throw” without specifying an exception object (e.g., throw;), it rethrows the current exception, preserving the original exception’s type message and, most importantly, its stack trace.
try
{
// Some code that throws an exception
}
catch (Exception ex)
{
// Log or handle the exception
throw; // Preserves the original exception details and stack trace
}
“throw ex” Clause:
- When you use “throw ex” (e.g., throw ex;), it rethrows the exception but replaces the original exception with a new one. This results in the loss of the original exception’s stack trace information.
- It’s generally not recommended to use “throw ex” because it can make debugging more challenging since the original context of the exception is lost.
try
{
// Some code that throws an exception
}
catch (Exception ex)
{
// Log or handle the exception
throw ex; // Replaces the original exception, losing its stack trace
}
The main difference is that the “Throw ex” overwrites the stack trace, making it difficult to locate the original code line number where the exception was thrown.
Throw, on the other hand, essentially keeps the stack information and also provides more precise error information.
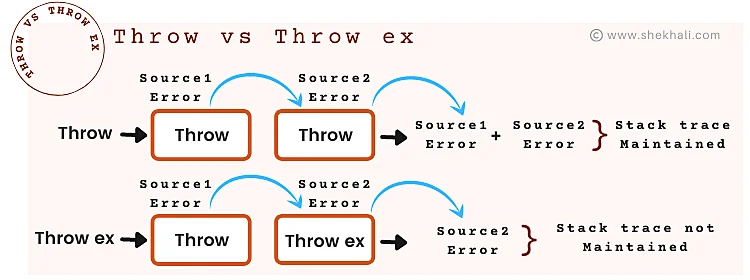
Q20. What is the difference between finally and finalize block?
- Finally is a code block executes immediately after the try-catch block, whereas Finalize is a method of Object class.
- The finally block in exception handling is used to execute the crucial cleanup-type code to free the resources regardless of whether the try block raised an exception.
- The finalize method in C# is used to perform clean-up processing just before the object is garbage collected.
Q21. What is Serialization in C#?
Serialization in C# refers to the process of converting an object into a stream of bytes that can be saved to memory, a file, or a database. Deserialization is the opposite of Serialization, in which an object is created from a stream of bytes.
The SerializableAttribute must be applied to the type in order to serialize the object.
The major goal of serialization is to save an object’s state so that we can restore it whenever we need it, and we can transfer an object to other applications via web or rest services in the form of a stream of bytes.
To read more about Serialization, check out this MSDN article:Â Serialization (C#)
Q22. What is the difference between constants and readonly in C#?
- In C#, Constant variables are declared and initialized at compile-time only. The value once assigned cannot be changed from anywhere in the program including constructors and member functions, whereas the value of a readonly field can be initialized at the time of declaration or in default or parameterized constructor.
- Readonly fields cannot be declared in methods, but const variables can.
- A const value is the same for all objects in a program, whereas readonly values can differ for each object.
- The readonly field can be used for run-time constants, while the const field is used for compile-time constants.
Q23. What is multithreading in C#?
Multithreading allows you to develop applications that perform multiple operations at the same time. Each operation can execute on a separate thread. Multithreading makes an application more responsive and allows you to perform parallel processing so that you can do more than one operation at a time.
You can create a new thread by using the System.Threading.Thread class.
To read more about multithreading, refer to this article:Â Multithreading (C#)
Q24. What is Reflection in C#?
In C# .NET, Reflection is the process of retrieving the type from an existing object and accessing its fields, properties, Methods, Events, and other members at the runtime.
The System.Reflection
 namespace contains types that retrieve information about an assembly. It allows you to create an instance of a type dynamically.
Some of the most commonly used classes in the reflection namespace are Assembly, MethodInfo, PropertyInfo, FieldInfo, EventInfo, ConstructorInfo, etc.
To read more about reflection, refer to this article:Â Reflection In C#
Q25. What are Collections in C#?
Collections in C# are a predefined set of specialized classes that are available in the System.Collections
 namespace for data storage and retrieval. Collections can be used for multiple operations on objects, including storing, updating, retrieving, searching, sorting, and deleting.
Collections are used to work with a group of related objects that can dynamically grow or shrink when objects are added or deleted depending on our needs.
The System.Collections
 namespace has various commonly used classes such as ArrayList, Hashtable, SortedList, Stack, and Queue.
To read more about Collections, refer to this article:Â Collections in C#
Q26. What is Constructor Chaining in C#?
Ans- Constructor Chaining is a process of calling one constructor from another constructor in the same class or base class by using this or base keyword.
Following is the example of constructor chaining using this keyword.
using System;
namespace ConstructorChainingExample
{
class MyClass
{
// Constructor
public MyClass(int setId)
{
Id = setId;
}
// Calling one constructor from another using 'this' keyword.
public MyClass(int id, string setName) : this(id)
{
//Id = id;
Name = setName;
}
public int Id { get; set; }
public string Name { get; set; }
}
class Program
{
static void Main(string[] args)
{
// Here MyClass(10) construct will call by the below mentioned
// parameterized constructor
MyClass obj = new MyClass(10, "Shekh Ali");
Console.WriteLine($"Id: {obj.Id} Name:{ obj.Name}");
Console.ReadLine();
}
}
// Output:
// Id: 10 Name:Shekh Ali
}
Q27. What is monitor class in C#?
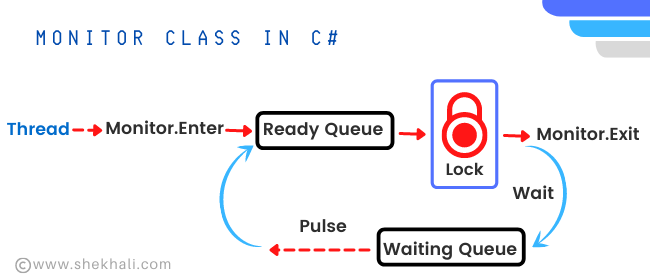
In C#, the Monitor class is a static class that allows you to synchronize access to the critical section of code by locking and releasing a specific object using the Monitor.Enter , Monitor.TryEnter , and Monitor.Exit methods. It ensures that only one thread at a time can access a critical section of code. While one thread holds the lock on the object, the other thread cannot acquire the lock.
To read more about monitor class, refer to this article: Monitor class in C#
Q28. What are generics in C#.NET?
Ans- Generics is the most powerful feature introduced in C# 2.0 to define type-safe classes, interfaces, methods, events, delegates, and strongly typed generic collections.
A generic type is defined by enclosing a type parameter in angle brackets after the type name, such as TypeName<T>
, where T
is the type parameter.
Generic types can be used to maximize code reusability, type safety, and performance. All generic collection classes are available in System.Collections.Generic
namespace.
To read more about generics, refer to this article: Generics in C# with examples
Q29. Explain Access Modifiers in C#?
Access modifiers are keywords in C# that specify how a class, its properties, fields, and methods are accessible in a program. The access modifiers are mainly used to define the scope of the type and its members, also it is used to safeguard data from being accidentally modified by external classes or programs.
The six access modifiers available in C# are listed below.
- public
- protected
- internal
- protected internal
- private
- private protected
To read more about access modifiers, refer to this article: Access Modifiers in C#
Q30. Can you declare struct members as protected or protected internal in C#?
Ans- Struct members in C# cannot be declared as protected, protected internal, or private protected because structs do not support inheritance.
Q31. What is the difference between static class and singleton pattern?
Singleton and static classes are both used to provide only one instance of a class that can be accessed globally across the application.
The Following are the main differences:
- A singleton class can implement interfaces as it supports inheritance, whereas a static class cannot.
- If it’s a large object, a singleton class can be lazy-loaded, however, a static class is always eagerly loaded.
- writing unit tests for singleton classes is easier than static classes as you can pass mock objects whenever singleton is expected.
- A static class can have only static data members whereas a singleton class can have both static and non-static members.
- Singleton objects can be passed to methods, whereas a static class cannot.
The following is an example of a singleton class.
using System;
public class Singleton
{
private static Singleton instance;
// Private Constructor
private Singleton(){}
public static Singleton Instance
{
get
{
if (instance == null)
{
instance = new Singleton();
}
return instance;
}
}
}
To read more about the singleton pattern, refer to this MSDN link: Singleton in C#
Q32. What exactly is the anonymous type in C#?
Ans- Anonymous type was introduced in C# 3.0. Anonymous type is a kind of temporary data type that doesn’t have a name and only has public read-only properties.
The compiler automatically generates the type of each property based on the value provided to it. It cannot have other members like class, such as fields, methods, events, etc.
The anonymous type can be created by using the new
operator with an object initializer. The var variable is commonly used to store the reference of an anonymous type.
// Example: Access Anonymous Type in C#
var person = new { Id = 10, Name = "Shekh Ali"};
Console.WriteLine($"Id: {person.Id} Name:{ person.Name}");
// output: Id: 10 Name:Shekh Ali
Console.ReadLine();
Q33. Define sealed classes in C#?
In C#, A Sealed class can be created by using the sealed keyword which restricts the class from being inherited.
The sealed keyword indicates to the compiler that the class is sealed and cannot be extended further.
The sealed modifier can be also applied to a method or property in a base class that overrides a virtual method or property. This allows other derived classes to inherit from your class but prevents them from overriding specific virtual methods or properties that have been declared as sealed.
Q34. What is a multicast delegate in C#?
Ans- A multicast delegate is a delegate that holds references to several methods with the same signature in its invocation list. When we call the multicast delegate, all of the methods that the delegate references are called in sequence.
In multicast delegate, We can use Delegate.Combine
method or +(plus) operator to chain delegates together and – (minus) operator to remove them from the invocation list.
To read more about multicast delegates, refer to this article: Multicast delegate in C# with examples, MSDN Combine Delegates (Multicast Delegates)
Q35. What are generic delegates in C#?
The three generic delegates, Func , Action , and Predicate , were introduced in.NET 3.5 and are available in the System namespace.
When we want to invoke the methods without explicitly defining the delegates, we can use any of these three predefined generic delegates.
Generic delegates | Description |
---|---|
1. Func: | Func delegate can accept up to 16 input parameters of the same or different data types, as well as a single output parameter. |
2. Action: | The Action delegate and the Func delegate are quite similar. It can also take up to 16 input parameters, but it doesn’t return any value.Action delegate can be used to point to those methods that return void and accept zero or more input parameters. |
3. Predicate: | A predicate delegate is generally used to check whether a parameter meets a specific condition or not.If the requirements are met, it will return true; otherwise, it will return false. |
To read more about Generic delegates, refer to this article: Generic delegates in C#
Q36. What is the difference between Array and Arraylist in C#?
- The size of an Array is fixed and cannot be changed dynamically, whereas the size of an ArrayList can be changed dynamically as items are added or removed.
- Array class is available in System.Array namespace, whereas ArrayList belongs to the System.Collections namespace.
- An array is a collection that can only contain items of the same data type, but an ArrayList can contain items of different data types.
- An Array cannot accept null, however null is allowed in the ArrayList.
Q37. What is an extension method in C#?
Ans- Extension methods were introduced in C# 3.0, which allows developers to add new methods to existing classes or structures without having to create new derived types, recompile code, or change the original types.
An extension method is a static method and is always defined in the static class. The first parameter of the extension method must be of the type for which the extension method is suitable, followed by this keyword.
namespace ExtensionMethodsExample
{
public static class MyExtensionClass
{
// Extension method applicable for string
public static int WordCount(this String str)
{
return str.Split(new char[] { ' ', '.', '?' },
StringSplitOptions.RemoveEmptyEntries).Length;
}
}
}
To read more about Extension methods, refer to this article: Extension methods in C#
Q38. Why this keyword is used in c#?
In C#, this
keyword refers to the current instance of the class. It’s also used to distinguish between method arguments and class fields when their names are the same.
This keyword can also be used in constructor chaining to invoke one constructor from another in the same class.
Q39. Can we use this keyword in a static method in C#?
No, we can not use the this
keyword inside a static method. because this
keyword refers to the current instance of the class, and Static Member functions do not belong to a particular instance. They exist without creating an instance of the class and can be called directly by the class name. However, in an exceptional case, this keyword can be used as the first parameter of the extension method which is a static method.
Q40. What is the difference between an interface and an abstract class?
- All the methods of the Interface are abstract by default, with only declaration and no definition. However, an abstract class can contain both abstract and concrete methods.
- All methods in an interface are public by default, whereas an abstract class can have also private methods.
- A class can be inherited from multiple interfaces, but a class cannot be inherited from more than one abstract class.
- An Interface doesn’t contain a constructor, whereas an abstract class can have constructors.
To read more about Interface and abstract class, refer to this article: Top 10 differences between Abstract class and Interface with examples in C#
Q41. What is the difference between late binding and early binding in C#?
Polymorphism has two concepts: early binding and late binding.
Early binding (also known as static binding) occurs at compile time when the compiler already knows what type of object it is and what methods or properties it contains.
Late Binding (or dynamic binding) occurs at runtime. In this process, the binding of overridden methods or properties takes place at the run time.
The late binding can be achieved by using virtual methods.
Read more about early binding vs late binding in C#
Q42. What is a Jagged Array in C#?
In C#, A jagged array is an array of arrays. Its elements are basically arrays of different sizes.
A jagged array can be initialized using two square brackets [][]. The first square bracket specifies the array’s size, while the second specifies the array’s dimension, which is stored as a value.
Following is an example of a jagged array in C#.
namespace JaggedArrayExample
{
class Program
{
static void Main(string[] args)
{
// Declare the array
int[][] arr1 = new int[2][];
// Jagged Array with Two Dimensional Array
// int[][,] arr2 = new int[4][,];
arr1[0] = new int[] { 10, 20, 30, 40 };// Initialize the array
arr1[1] = new int[] { 55, 65, 75, 85, 95, 100 };
// Traverse ( or loop) array elements
for (int i = 0; i < arr1.Length; i++)
{
for (int j = 0; j < arr1[i].Length; j++)
{
System.Console.Write(arr1[i][j] + " ");
}
System.Console.WriteLine();
}
Console.ReadLine();
}
}
// Output:
// 10 20 30 40
// 55 65 75 85 95 100
}
Q43. What is the difference between Continue and Break Statement?
-
Break: The break statement breaks the loop when a given condition is met.
- Continue: The continue statement skips one iteration of the loop when a specified condition is met and resumes with the next iteration.
// Example of break statement:
for (int i = 0; i < 10; i++)
{
if (i == 5)
{
break;
}
Console.WriteLine(i);
}
// Example of continue statement:
for (int i = 0; i < 10; i++)
{
if (i == 5)
{
continue;
}
Console.WriteLine(i);
}
Q44. What is Thread Pooling in C#?
A thread pool is a collection of threads used in a multithreaded application. These threads can be used to perform the new task on-demand instead of creating new threads.
When the task is completed, the thread will return to the thread pool and will be available again.
The System.Threading.ThreadPool
namespace contains classes that handle the threads in the pool.
Q45. What is the difference between an EXE and a DLL?
- An EXE is a self-executable file that can run by itself, whereas DLL can’t execute independently.
- A DLL does not have an entry point (main method), whereas an EXE file does.
- A DLL can be versioned and reused by the other DLL or Executable Files. An EXE, on the other hand, Cannot.
- A DLL is an in-process component but EXE is an out-process component. When the system launches an EXE, a new process is created, whereas for DLL a new process is not created.
- A DLL can be reused in the application whereas an EXE Cannot.
- An EXE stands for executable, whereas DLL stands for Dynamic Link Library
Q46. What is the difference between ‘string’ keyword and ‘System.String’ class?
Basically, there is not much difference between “string” and “String” in C#. “string” keyword is just an alias of the System.String
class.
The keyword “string” is widely used to declare a variable, whereas System.String
is a class and used for accessing string static methods such as String.Format()
, String.Compare()
, String.Concat()
etc.
using System;
namespace ConsoleApplicationDemo {
public class Program {
public static void Main(string[] args) {
string s1 = "Hello ";
string s2 = "World";
string s3 = String.Concat(s1, s2);
Console.WriteLine(s3);
Console.ReadLine();
}
}
}
Q47. What is the difference between int.Parse and int.TryParse method in C#?
The int.Parse() method throws an exception if the string you are trying to parse is not a valid number, whereas int.TryParse() method returns false and doesn’t throw an exception if parsing fails. So int.TryParse() method is more efficient than Parse, It is useful for determining whether or not a string value has been converted to an integer.
Q48. Give some examples of value types and reference types
- Value types: Enum, Struct, bool, byte, char, decimal, double, float, int, long
- Reference types: Class, Interface, Delegate, String, Array
Read more about Value type and Reference type in details.
Q49. What is the volatile keyword in C#?
The volatile keyword in C# is to avoid data conflicts when many threads are trying to access the same data at the same time.
The volatile keyword tells the JIT compiler that the value of the field should never be cached since it could be altered by the operating system, hardware, or another thread running at the same time.
As a result, the compiler avoids any optimizations on the variable that might lead to data conflicts because of different threads accessing different values of the variable.
To read more about Interface and Volatile keywords, refer to this article: Volatile (c#)
Q50. What is an enum (enumeration) in C#?
Ans- In C#, Enum is a value data type with a set of related named constants, commonly known as an enumerator list.
The enum keyword is used to assign names or string values ​​to integer constants, making the program easier to read and maintain. Items in the enum can be declared in curly brackets {} separated by commas. The enum is a user-defined primitive data type that can be an integer, float, double, bytes, and so on, but you’ll need to cast it if you use it with int. By default, the underlying type of enumeration element is an integer.
using System;
namespace EnumExample
{
class Program
{
// Declared an enum inside a class
enum Season
{
Spring = 1,
Summer = 2,
Autumn = 3,
Winter = 4
}
static void Main(string[] args)
{
//Array of string
string[] seasonNames = Enum.GetNames(typeof(Season));
Console.WriteLine("*** Seasons Names ***");
//iterate enum variables.
foreach (var season in seasonNames)
{
Console.WriteLine(season);
}
Console.ReadKey();
}
}
}
To read more about enum, refer to this article: C# Enum: How to Use enumeration type in C#?
Thank you for taking the time to read the blog.
A blog without comments isn’t really a blog at all, so If you have any queries or would like to provide feedback, please leave a comment below.
Articles you might also like:
- C# Tutorials
- SOLID Design Principles in C#: A Complete Example
- Singleton Design Pattern with examples
- C# dispose vs finalize – Understanding the difference between dispose and finalize in C#
- C# Abstract class Vs Interface
- C# Partial Class And Partial Methods With Examples
- C# Monitor class in multithreading
- C# Struct vs Class
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025