In this article, we’ll learn the Dependency Inversion Principle in C# with code examples. We will explore its significance, benefits, and implementation using real-world examples.
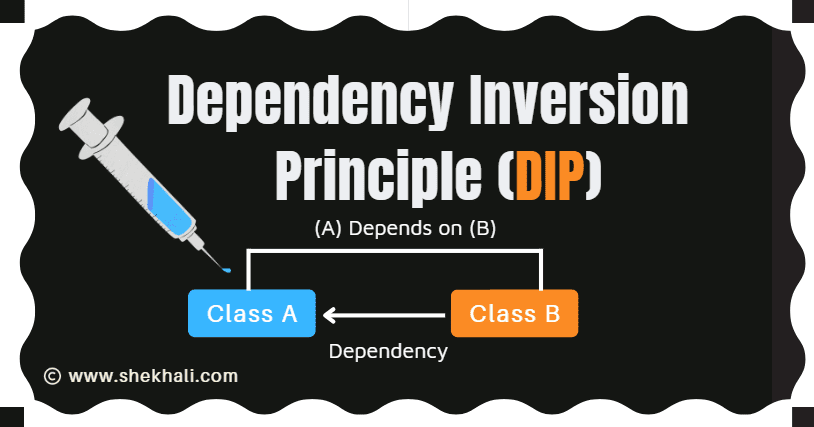
Learn about Design Patterns and their uses in software development with examples in C#. This comprehensive guide covers the three categories of Design Patterns – Creational, Structural, and Behavioral – and their specific patterns, including Abstract Factory, Adapter, Template Method, and more. This in-depth guide will improve your software development skills and create more efficient and maintainable software systems.
In this article, we’ll learn the Dependency Inversion Principle in C# with code examples. We will explore its significance, benefits, and implementation using real-world examples.
In this article, we will learn about the C# Interface Segregation Principle (ISP), which states that a class should not forced to implement Interface methods they don’t use.
It promotes the creation of focused, smaller, and modular interfaces to prevent clients from implementing methods they don’t need.
We will learn its significance and how it can be applied using C# code examples.
In this article, we will learn the C# Liskov Substitution Principle and understand implementation to build scalable and easier to maintain software applications.
When we write code, it’s important to follow certain rules and principles to make our software easier to work with any update. One of these important SOLID principles is called the Open Closed Principle
, or OCP in short.
When software developers want to write organized, clean, maintainable, and scalable code for their projects, they use design principles. One of these principles is called SOLID, which contains five rules that help to improve software design and structure.
In this article, we will focus on the first rule of SOLID
principle, called the Single Responsibility Principle (SRP) with examples.
Introduction: In software development, design patterns play an important role in creating reusable and maintainable code. One such pattern is the Chain of Responsibility design pattern, which promotes loose coupling and flexibility in handling requests.
In this article, we will explore the Chain of Responsibility design pattern in the context of C#, providing clear explanations and practical examples to help beginners grasp its concepts effectively.
Dependency Injection (DI) is a software design pattern that helps developers to build high-quality software.
It reduces tight coupling between software components and allows us to develop loosely-coupled code, which is easier to maintain and offers greater flexibility and testability.
The fundamental principle behind Dependency Injection is to minimize hard-coded dependencies among classes by dynamically injecting them during runtime instead of design time.
This approach ensures that the dependencies of a class are provided from external sources, typically through constructor parameters or property setters, rather than being directly instantiated within the class itself.
Introduction: Design patterns are reusable solutions to common problems in software design. One such pattern is the Prototype Design Pattern, which allows you to create new objects by cloning existing ones.
This article will explore the Prototype Design Pattern when to use it, real-world examples, advantages and disadvantages, UML diagram, and how to implement it with a complete code example in C#.
The Builder Design Pattern is a creational design pattern that separates the construction of complex objects from their representation, enabling developers to create different representations of the same object using the same construction process.
Design Patterns are a set of reusable solutions to commonly occurring problems in software design. They are not a finished design that we can transform directly into code but rather a guide for solving problems.
The goal of using Design Patterns is to increase the efficiency and effectiveness of software development and provide a common vocabulary for developers to discuss design solutions.
The Abstract Factory Design Pattern is a creational design pattern that provides a way to create families of related or dependent objects without specifying their concrete classes. This blog post will explore the Abstract Factory Design Pattern in C#, using a real-world example and providing code explanations.
SOLID is a set of five design principles introduced by Robert C. Martin in 2000 to make code more maintainable, flexible, and scalable.
In this article, we will learn about five important SOLID principles and how they can be used with C# programming. We’ll also see practical examples to understand how to apply these principles in real-world situations.
SOLID is a widely recognized acronym that represents a set of five fundamental design principles in software development: the Single Responsibility Principle, Open-Closed Principle, Liskov Substitution Principle, Interface Segregation Principle, and Dependency Inversion Principle.