The concepts of Covariance and Contravariance in C# might initially sound complex, but fear not! By the end of this article, you’ll have a crystal-clear understanding of how they work and how to leverage them in your C# programming.
“Covariance and Contravariance are terms used in programming languages to describe how subtypes relate to their base types. Covariance is when a derived type can be used where a base type is expected. On the other hand, Contravariance is when a base type can be used where a derived type is expected.“
Covariance and contravariance deal with how type conversions are allowed between reference types in C#. These concepts come into play when working with arrays, delegates, and interfaces.
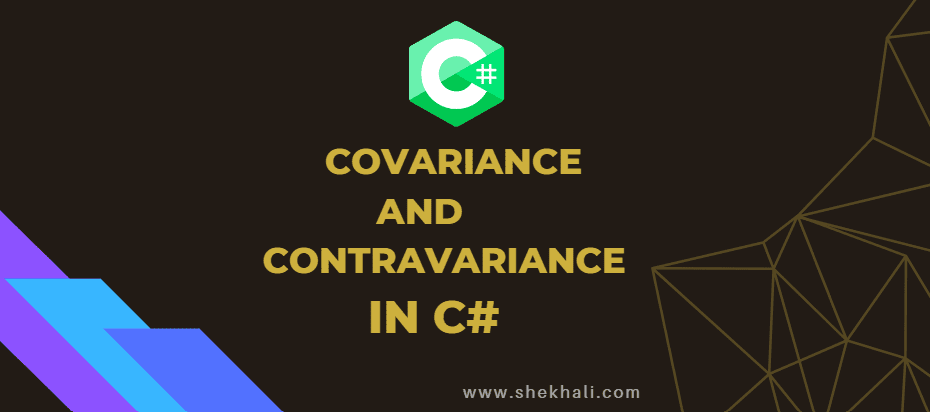
Let’s start by understanding each concept separately.
Table of Contents
Covariance in C#
Covariance allows a conversion from a more derived type to a less derived type. In simpler terms, it lets us use a derived type where a base type is expected.
For example, suppose a class B is derived from a class A. In that case, a covariant type allows us to seamlessly substitute an instance of B wherever an instance of A is expected.
It preserves the assignment compatibility, enabling you to treat a collection of derived types as a collection of base types. Covariance can be applied on array, delegate, interface, generic etc.
Example 1: Using Covariance With Array
Here, the array of derived type Dog
is treated as an array of base type Animal
.
using System;
// Defines a base class 'Animal'
class Animal { }
// Defines a derived class 'Dog'
class Dog : Animal { }
class Program
{
static void Main()
{
// Creating an array of dogs
Dog[] dogs = new Dog[] { new Dog(), new Dog() };
// Covariance: Treating the array of dogs as an array of animals
Animal[] animals = dogs; // Covariance in action
// Demonstrating the result
Console.WriteLine("Animals array contains " + animals.Length + " animals.");
Console.WriteLine("First animal is a " + animals[0].GetType().Name);
}
}
Output:
// Output:
Animals array contains 2 animals.
First animal is a Dog
Code Explained:
- We have created the
Animal
andDog
classes.Dog
class is derived fromAnimal
. - We create an array of
Dog
objects nameddogs
. - Covariance occurs when we assign the
dogs
array (of derived typeDog
) to theanimals
array (of base typeAnimal
). This is possible becauseDog
is a subtype ofAnimal
. - The
animals
array can now hold objects of typeDog
, even though it’s declared as an array of typeAnimal
. - Output shows the length of the
animals
array and the type of the first animal, which is indeed aDog
.
This example illustrates how covariance allows us to treat a more specific type (Dog
) as a more general type (Animal
) in certain contexts, such as arrays. The power of covariance lies in its ability to enable code reusability and flexibility when working with polymorphic structures.
Example 2: Using Covariance With Interface
The IEnumerable interface exhibits covariance.
Let’s use the same example:
using System;
using System.Collections.Generic;
using System.Linq;
class Animal { }
class Dog : Animal { }
class Program
{
static void Main()
{
// Creating an enumerable of dogs
IEnumerable<Dog> dogEnumerable = new List<Dog> { new Dog(), new Dog() };
// Covariance with IEnumerable<out T>: Treating dogEnumerable as a collection of animals
IEnumerable<Animal> animalEnumerable = dogEnumerable; // Covariance in action
Console.WriteLine($"Animals array contains {animalEnumerable.Count()} animals ");
// Iterating through the animalEnumerable and demonstrating the result
foreach (Animal animal in animalEnumerable)
{
Console.WriteLine($"Animal type: {animal.GetType().Name}");
}
}
//Output:
//Animals array contains 2 animals
// Animal type: Dog
// Animal type: Dog
}
This example demonstrates covariance using the IEnumerable<out T>
interface. It shows how a collection of a more specific type (Dog
) can be treated as a collection of a more general type (Animal
).
Example 3: Using Covariance With Func<out TResult>
Delegates can also be covariant with return types.
Explaining covariance with return types using the Func<out TResult>
delegate:
using System;
using System.Collections.Generic;
using System.Linq;
class Animal { }
class Dog : Animal { }
class Program
{
static void Main()
{
// Creating an enumerable of dogs
IEnumerable<Dog> dogEnumerable = new List<Dog> { new Dog(), new Dog() };
// Covariance with IEnumerable<out T>: Treating dogEnumerable as a collection of animals
IEnumerable<Animal> animalEnumerable = dogEnumerable; // Covariance in action
Console.WriteLine($"Animals array contains {animalEnumerable.Count()} animals ");
// Iterating through the animalEnumerable and demonstrating the result
foreach (Animal animal in animalEnumerable)
{
Console.WriteLine($"Animal type: {animal.GetType().Name}");
}
}
//Output:
//Animals array contains 2 animals
// Animal type: Dog
// Animal type: Dog
}
This example illustrates how covariance works with delegate return types using the Func<out TResult>
delegate.
It showcases how a delegate returning a more specific type (Dog) can be treated as a delegate returning a more general type (Animal).
Contravariance in C#
Contravariance is a powerful type compatibility concept in C# that allows a more general (base) type to be used where a more specific (derived) type is expected.
This might sound contradictory at first, but it becomes incredibly useful when working with delegates, method parameters, and interfaces.
Imagine you have a class hierarchy where one class is derived from another. Contravariance lets you use methods or components that require the base class as arguments, even if you provide instances of the derived class.
This enhances code reusability and adaptability by enabling methods and components to accept a wider range of inputs without sacrificing type safety.
For example, when defining delegates or method parameters, you can use contravariance to accept arguments of more general types, providing a level of flexibility that can simplify your code and make it more versatile.
Contravariance in C# enables developers to design more flexible and reusable code by allowing them to work with base types where derived types are expected.
Example: Contravariance using delegate
using System;
namespace ContravarianceExample
{
public class Manager
{
public string Skill = "Can Talk";
}
public class Employee : Manager
{
public string Position = "Regular Employee";
}
delegate void Factory<in T>(T employee);
class Program
{
static void PrintSkill(Manager manager)
{
Console.WriteLine($"Skill: {manager.Skill}");
}
static void Main(string[] args)
{
Factory<Manager> managerFactory = PrintSkill;
Factory<Employee> employeeFactory = managerFactory; // Contravariance
Employee emp = new Employee();
employeeFactory(emp);
Console.ReadKey();
}
}
}
Output:
Skill: Can Talk
Code Explained:
The Factory<in T>
delegate is contravariant, which means that you can assign a delegate that takes a more general type (Manager
) to a delegate that takes a more specific type (Employee
).
In the Main
method:
- We create an instance of the
Factory<Manager>
delegate namedmanagerFactory
, which points to thePrintSkill
method. - We assign the
managerFactory
delegate to an instance of theFactory<Employee>
delegate namedemployeeFactory
, showcasing contravariance. - We then invoke the
employeeFactory
delegate, passing an instance ofEmployee
as an argument. This demonstrates that the contravariant delegate can handle a more specific type (Employee
) even though the delegate is defined to accept a more general type (Manager
).
When you run the code, you’ll see that the employeeFactory
delegate, assigned through contravariance, successfully calls the PrintSkill
method with an Employee
object.
FAQs
Q: What is covariance and contravariance in C#?
Covariance and contravariance are concepts that deal with the compatibility of types in C#. Covariance enables a more specific type to be used in place of a more general type, while contravariance allows a more general type to be used in place of a more specific type.
Q: How does covariance work in C#?
Covariance allows a derived type to be used where a base type is expected. For example, if you have a class hierarchy with a base class Animal
and a derived class Dog
, you can treat a collection of Dog
instances as a collection of Animal
instances.
Q: How does contravariance work in C#?
Contravariance allows a base type to be used where a derived type is expected. It’s often used with method parameters or delegates. For instance, you can pass a method that accepts a BaseClass
parameter to a delegate that expects a DerivedClass
parameter.
Q: Where are covariance and contravariance commonly used?
Covariance is commonly used when working with collections, interfaces like IEnumerable, and scenarios where you want to treat specific types as more general types. Contravariance is widely used with method parameters, delegates, and scenarios where you want to accept broader inputs.
Q: Can covariance and contravariance be used interchangeably?
No, covariance and contravariance in C# are different concepts. They work in opposite directions: covariance uses derived types where base types are expected, while contravariance uses base types where derived types are expected. Each has its own specific use cases and benefits.
Recommended Articles:
- C# Tutorial
- Generic delegates in C# with examples
- 10 Differences between interface and abstract class In C#
- C# Dynamic Type: Var vs dynamic keywords in C#
- Generics in C#
- Top 50 C# Interview Questions And Answers For Freshers And Experienced
- Top 5 Differences between Call by Value and Call by Reference
- C# Struct vs Class
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025