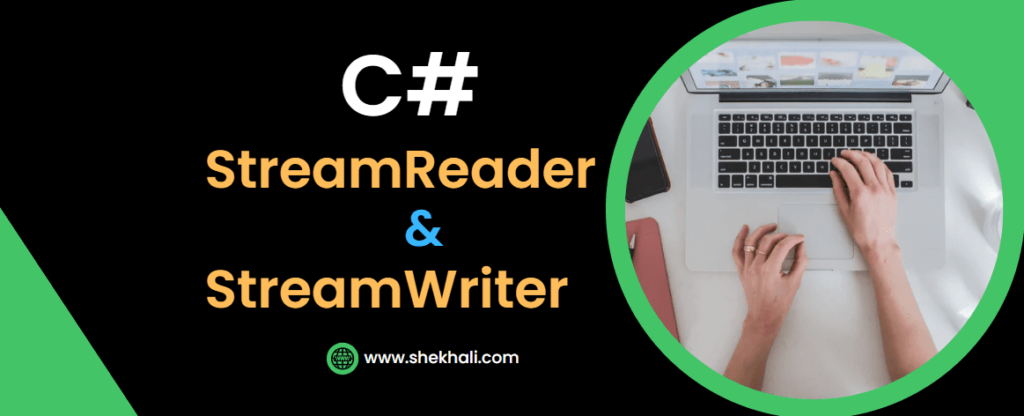
Table of Contents
- 1 C# Stream
- 2 StreamWriter in C#
- 3 C# Stream Reader
- 4 FAQs: StreamReader and StreamWriter
- 4.1 Q: What do stream reader and stream writer mean in C#?
- 4.2 Q: Does StreamReader provide thread safety?
- 4.3 Q: What is the use of FileStream class in C#?
- 4.4 Q: What exactly do System.IO classes used for?
- 4.5 Q: What is the FileStream buffer size?
- 4.6 Q: Does the StreamWriter class create files in C#?
- 4.7 Q: How do I read a text file in C#?
- 4.8 Q: What is the difference between a StreamReader and StreamWriter in C#?
- 4.9 Q: What is the buffer size for the StreamWriter object in C#?
- 4.10 Summary:
- 4.11 Related
C# Stream
Streams in C# allow you to transfer data from one point to another quickly and efficiently. The data transfer can be between files, sockets, objects, or even other streams.
Usually, Streams are used while reading data from large files. By using streams, the data from large files are split into small chunks and sent to the stream. These chunks of data can then be read by the application.
The stream is basically a sequence of bytes passing through a communication path. The two main streams are the input stream and the output stream. The input stream is used to read data from the file and the output stream is used to write data into the file.
StreamWriter in C#
The C# StreamWriter class is used to write characters to a stream. It is derived from the Abstract class “TextReader” and provides overloaded methods for writing data to files.
The StreamWriter is generally a helper class for writing a string to a Stream by converting characters into bytes. It can be used to write strings to different Streams including FileStream, MemoryStream, and so on.
// StreamWriter class implements a TextWriter for writing characters
// to a stream in a particular encoding.
public class StreamWriter : System.IO.TextWriter
The StreamWriter provides the following useful writing methods.
Method | Description |
---|---|
1. Write | Writes data (character, string, text) to the stream. |
2. WriteAsync | Asynchronously writes data (character, string, text) to the stream. |
3. WriteLine | Writes a line terminator to the text stream. |
4. WriteLineAsync | Asynchronously writes a line terminator to the stream. |
Example:
using System;
using System.IO;
public class StreamWriterExample
{
public static void Main(string[] args)
{
string fileName = @"C:\Temp\textFile.txt";
//Create object of FileInfo for specified path
FileInfo fileInfo = new FileInfo(fileName);
// Create a FileStream
FileStream fileStream = fileInfo.Open(FileMode.OpenOrCreate, FileAccess.Write, FileShare.Read);
// Create a StreamWriter using FileStream
using (StreamWriter streamWriter = new StreamWriter(fileStream))
{
streamWriter.WriteLine("Hello World");
streamWriter.Close();
}
Console.ReadLine();
}
// Output: Hello World
}
Once you run the application, you will get the following result in the text file.
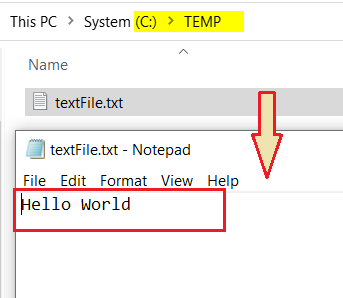
C# Stream Reader
You can use the StreamReader class In order to read a physical file in C#. The following example will demonstrate to you how to read a file using StreamReader.
StreamReader Class Implements a TextReader that reads characters from a byte stream in a particular encoding.
public class StreamReader : System.IO.TextReader
Example:
using System;
using System.IO;
public class StreamReaderExample
{
public static void Main(string[] args)
{
// File name
string fileName = @"C:\Temp\textFile.txt";
//Creating an object of FileInfo class for specified path
FileInfo fileInfo = new FileInfo(fileName);
//Open a file for Read\Write operation
using (FileStream fileStream = fileInfo.Open(FileMode.OpenOrCreate, FileAccess.Read, FileShare.Read))
{
using(StreamReader streamReader = new StreamReader(fileStream))
{
//Use the ReadToEnd method to read all the content from file
string text = streamReader.ReadToEnd();
Console.WriteLine(text);
Console.ReadLine();
}
}
}
// Output: Hello World
}
FAQs: StreamReader and StreamWriter
Q: What do stream reader and stream writer mean in C#?
Ans: The StreamReader and StreamWriter classes are used to read and write data to text files. These classes are derived from an abstract parent class Stream, which facilitates the ability to read from and write to file streams.
Q: Does StreamReader provide thread safety?
Ans: A StreamReader is not by default thread-safe.
Q: What is the use of FileStream class in C#?
Ans: The FileStream is a class used for reading and writing files in C#. It belongs to the System.IO namespace.
You must include the System.IO namespace before using the FileStream class to create a new file or open an existing one.
Q: What exactly do System.IO classes used for?
Ans: The System.IO namespace has various classes for creating, removing, reading from, or writing to files, closing files, and other file-related activities.
Q: What is the FileStream buffer size?
Ans: The default buffer size for the FileStream object is 8192 bytes.
Q: Does the StreamWriter class create files in C#?
Ans: The StreamWriter constructor initializes a new instance of the StreamWriter class for the given file using the default encoding and buffer size. If the file already exists, it can be either overwritten or appended. The constructor creates a new file if the specified file does not already exist.
// public StreamWriter(string path, bool append);
Q: How do I read a text file in C#?
Ans: The File.ReadAllText() method opens a text file, reads every text into a string, and then closes the text file.
Example:
// Read every text into a string
string strText = File.ReadAllText(textFile);
Console.WriteLine(strText);
Q: What is the difference between a StreamReader and StreamWriter in C#?
Ans: A StreamReader is used when data has to be read from a file. On the other hand, a StreamWriter is used when data needs to be written to a file.
Q: What is the buffer size for the StreamWriter object in C#?
Ans: The default buffer size is 1024 characters for the StreamWriter object in C#.
Summary:
In C#, The System.IO namespace provides various classes and methods that enable developers to perform read-write operations on different files.
Example: FileStream, StreamReader, StreamWriter, TextReader, TextWriter, etc.
- C# Stream is the abstract base class of all streams. A stream is an abstraction of a sequence of bytes like a file, an input/output device, an inter-process communication pipe, or a TCP/IP socket. It acts as an intermediate layer between your application and your files.
- C# StreamReader provides methods to read strings from a FileStream by converting a stream of bytes into strings.
- C# StreamWriter provides helper methods to write strings to FileStream by converting a string into a stream of bytes.
References: MSDN- StreamWriter Class , StreamReader Class
I hope you enjoyed reading this article “StreamReader and StreamWriter in C#“. If you find something incorrect or wish to offer more information regarding the subjects discussed here, please leave a comment below.
Articles to Check Out:
- C# Dictionary with Examples
- C# Array Vs List
- C# List Class With Examples
- C# Abstract class Vs Interface
- C# Struct vs Class
- C# HashTable Vs Dictionary
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024