Calculating factorial is a common task in programming. In C#, a factorial can be calculated in several ways, including loops and recursion. In this article, we will explore different ways to calculate factorial in C# and provide full code examples with explanations.
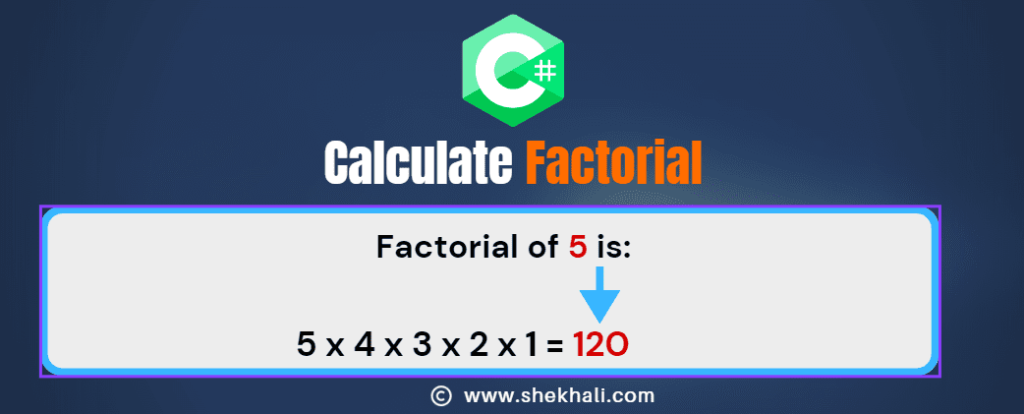
Table of Contents
What is Factorial in C#?
Factorial is a mathematical operation that multiplies a given number by all the positive integers that come before it. In C#, it is commonly used in various programming tasks, such as probability calculations and combinatorial analysis.
Different Ways to Calculate Factorial in C#:
In C#, you can calculate the factorial of a number by using a loop that continues until the number is no longer equal to 1.
Simply set the initial value of n to the number for which you want to find the factorial, and then loop through until n is no longer equal to 1.
The following are the different ways to calculate factorial in C#.
01. Using For Loop:
We can use a for loop to calculate factorial in C#. Here is an example:
using System;
namespace FactorialUsingForLoop
{
class Program
{
static void Main(string[] args)
{
int n = 5;
int factorial = 1;
for (int i = 1; i <= n; i++)
{
factorial = factorial * i;
}
Console.WriteLine($"Factorial of {n} is {factorial}");
Console.ReadLine();
}
}
}
Output:
Factorial of 5 is 120
02. Using Recursion:
Recursion is another way to calculate factorial in C#. Here is an example:
using System;
namespace FactorialUsingRecursion
{
class Program
{
// Factorial Using Recursion Method:
public static int Factorial(int n)
{
if (n == 0)
return 1;
return n * Factorial(n - 1);
}
static void Main(string[] args)
{
int n = 5;
Console.WriteLine("Factorial of {0} is {1}", n, Factorial(n));
Console.ReadLine();
}
}
}
// Output: Factorial of 5 is 120
Code Explanation:
The above C# code calculates the factorial of a number using a recursive function. The factorial of a number is the product of all the positive integers from 1 to that number.
For example, the factorial of 5 is 5 x 4 x 3 x 2 x 1, which equals 120.
The function Factorial(int n)
takes a number as its input and returns its factorial. If the input number is 0, the function returns 1.
Otherwise, it calculates the factorial recursively by calling itself with the argument n-1 and multiplying the result with the original n. This process continues until the input number becomes 0. When the function returns 1, then recursion ends.
03. Using While Loop:
We can also use a while loop to calculate factorial in C#. Here is an example:
using System;
namespace FactorialUsingWhileLoop
{
class Program
{
static void Main(string[] args)
{
int n = 5;
int factorial = 1;
int i = 1;
while (i <= n)
{
factorial = factorial * i;
i++;
}
Console.WriteLine($"Factorial of {n} is {factorial}");
Console.ReadLine();
}
}
}
// Output: Factorial of 5 is 120
04. Using do While Loop:
do while
loop can also be used to calculate factorial in C#. Here is an example:
using System;
namespace FactorialUsingDoWhileLoop
{
class Program
{
static void Main(string[] args)
{
int n = 5;
int factorial = 1;
int i = 1;
do
{
factorial = factorial * i;
i++;
}
while (i <= n);
Console.WriteLine($"Factorial of {n} is {factorial}");
Console.ReadLine();
}
}
}
// Output: Factorial of 5 is 120
05. Calculate Factorial in C# Using LINQ:
C# provides a powerful feature called Language-Integrated Query (LINQ) which allows us to perform complex queries on data. We can use LINQ to calculate factorial as follows:
using System;
using System.Linq;
namespace FactorialUsingLINQ
{
class Program
{
static void Main(string[] args)
{
int n = 5;
int factorial = Enumerable.Range(1, n).Aggregate((x, y) => x * y);
Console.WriteLine("Factorial of {0} is: {1}", n, factorial);
Console.ReadLine();
}
}
}
//Output: Factorial of 5 is: 120
In the code above, we first create a range of numbers from 1 to n using the Enumerable.Range
method. We then use the Aggregate
method to multiply all the numbers in the range together, resulting in the factorial value.
Conclusion:
In conclusion, calculating factorial in C# can be done in several ways, including loops and recursion. Each method has its advantages and disadvantages, and the choice of method depends on the specific requirements of the task. It is important to choose the right method to optimize performance and accuracy.
FAQs
Q: What is the maximum value of n that can be used to calculate factorial in C#?
The maximum value of n that can be used to calculate factorial in C# depends on the data type used to store the result. For example, if an int data type is used, the maximum value of n is 12. If a long data type is used, the maximum value of n is 20.
Q: Can recursion be used to calculate large factorials in C#?
Recursion can be used to calculate large factorials in C#, but it may not be the most efficient method for large values of n.
Q: Can we use nested loops to calculate factorial in C#?
Yes, Nested loops can be used to calculate factorial in C#. However, it may not be the most efficient method for large values of n and can result in slower execution time.
Q: What are some practical applications of calculating factorial in C#?
Factorial can be used in various programming tasks, such as probability calculations, combinatorial analysis, and cryptography. For example, a factorial can be used to calculate the number of permutations and combinations of a set of objects.
Articles you might also like:
- Design Patterns
- Builder Design Pattern: A Comprehensive Guide with C# Code Examples
- SOLID Design Principles in C#: A Complete Example
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- Abstract Factory Design Pattern in C#
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025