A leap year is a year that has an extra day added to its calendar, making it 366 days instead of the usual 365 days. For example, this extra day is added to the month of February, which has 29 days in a leap year instead of the usual 28 days.
We have leap years to keep our calendar year synchronized with the astronomical year, which is based on the Earth’s orbit around the Sun. The Earth takes approximately 365.24 days to complete one orbit around the Sun, so by adding an extra day every four years, and we can keep our calendar year in sync with the astronomical year.
However, not every fourth year is a leap year which we will discuss further in this article.
Table of Contents
- 1 What is a Leap Year?
- 2 Logic to Check If a given Year Is a Leap Year or Not?
- 3 Flow Diagram Of a Leap Year
- 4 Leap Year Program in C
- 5 Leap Year Program in C#
- 6 Leap Year Program in C++
- 7 Leap Year Program in Java
- 8 Leap Year Program in Python
- 9 Leap Year Program in PHP
- 10 Leap Year Calculation for Other Calendars
- 11 Leap Second
- 12 Applications of Leap Years
- 13 Limitations of Leap Years
- 14 Conclusion
- 15 FAQs
What is a Leap Year?
Before we dive into implementing the leap year program in C, let’s first understand what a leap year is.
A leap year is a year divisible by 4 but not divisible by 100 unless it is also evenly divisible by 400. For example, the year 2000 was a leap year because it was divisible by 4 and 400, but the year 1900 was not a leap year because it was divisible by 4 and 100 but not divisible by 400.
Here are some examples of leap years:
- 2020
- 2016
- 2012
- 2008
- 2004
- 2000
- 1996
- 1992
- 1988
- 1984
Logic to Check If a given Year Is a Leap Year or Not?
The logic to check if a given year is a leap year or not is as follows:
- If the year is divisible by 400, it is a leap year.
- If the year is not divisible by 400, but it is divisible by 100, it is not a leap year.
- If the year is divisible by 4 and also divisible by 100 and 400, then it is a leap year.
- If the year is not divisible by 100 but it is divisible by 4, it is a leap year.
- If the year is not divisible by 4, it is not a leap year.
Flow Diagram Of a Leap Year
The following is a flow diagram of how to write a leap year program.
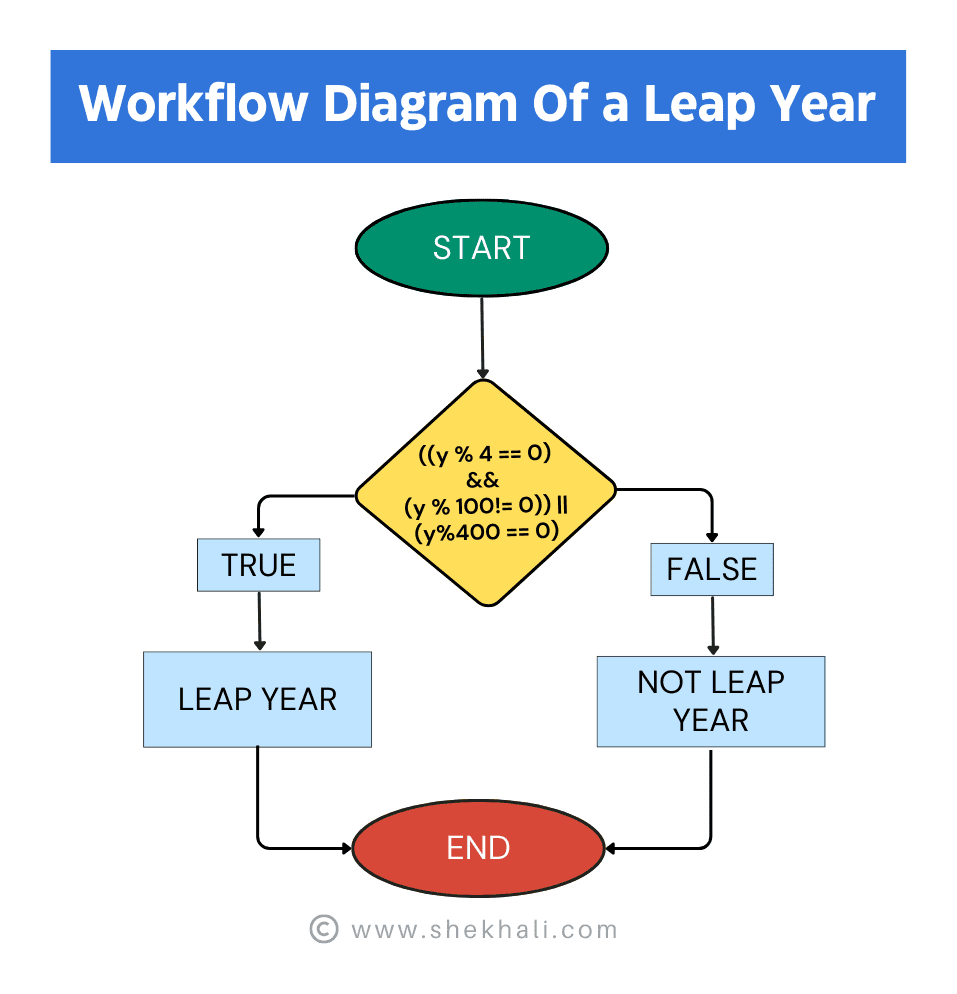
Leap Year Program in C
Let’s now see how to implement the leap year program in C.
Here, we will check if a given year is a leap year or not in C:
#include <stdio.h>
int main()
{
int year;
printf("Enter a year: ");
scanf("%d", &year);
if (((year % 4 == 0) && (year % 100 != 0)) || (year % 400 == 0))
{
printf("%d is a leap year.\n", year);
}
else
{
printf("%d is not a leap year.\n", year);
}
return 0;
}
Output:
Enter a year: 2000
2000 is a leap year.
Leap Year Program in C#
Let’s see how to implement the leap year program in C#. But, first, here is the code to check if a given year is a leap year or not:
using System;
public class LeapYearProgram
{
public static void Main()
{
int year;
Console.Write("Enter a year: ");
year = int.Parse(Console.ReadLine());
if (((year % 4 == 0) && (year % 100 != 0)) || (year % 400 == 0))
{
Console.WriteLine("{0} is a leap year.", year);
}
else
{
Console.WriteLine("{0} is not a leap year.", year);
}
Console.ReadLine();
}
}
Output:
Enter a year: 2020
2020 is a leap year.
Leap Year Program in C++
Here is the program to implement the leap year program in C++. Here we are using the code to check if a given year is a leap year or not:
#include <iostream>
using namespace std;
int main()
{
int year;
cout << "Enter a year: ";
cin >> year;
if (((year % 4 == 0) && (year % 100 != 0)) || (year % 400 == 0))
{
cout << year << " is a leap year." << endl;
}
else
{
cout << year << " is not a leap year." << endl;
}
return 0;
}
Output:
Enter a year: 2016
2016 is a leap year.
Leap Year Program in Java
Let’s now see how to implement the leap year program in Java. Here we will check if a given year is a leap year or not:
import java.util.Scanner;
public class LeapYearProgram {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter a year: ");
int year = input.nextInt();
if (((year % 4 == 0) && (year % 100 != 0)) || (year % 400 == 0)) {
System.out.println(year + " is a leap year.");
} else {
System.out.println(year + " is not a leap year.");
}
input.close();
}
}
Output:
Enter a year: 2000
2000 is a leap year.
Leap Year Program in Python
Let’s now see how to implement the leap year program in Python.
year = int(input("Enter a year: "))
if ((year % 4 == 0 and year % 100 != 0) or year % 400 == 0):
print(year, "is a leap year.")
else:
print(year, "is not a leap year.")
Output:
Enter a year: 2004
2004 is a leap year.
Leap Year Program in PHP
Let’s see how to implement the leap year program in PHP. Here is the PHP code to check if a given year is a leap year or not:
<?php
$year = readline("Enter a year: ");
if (((int)$year % 4 == 0 && (int)$year % 100 != 0) || (int)$year % 400 == 0) {
echo $year . " is a leap year.";
} else {
echo $year . " is not a leap year.";
}
?>
Output:
Enter a year: 2000
2000 is a leap year.
Let’s learn a few more things about the leap year.
Leap Year Calculation for Other Calendars
Many calendars besides the Gregorian calendar also use leap years to keep their calendars in sync with the solar year.
For example, the Islamic calendar uses a lunar calendar and has a different approach to calculating leap years.
Similarly, the Chinese calendar uses a lunisolar calendar, which means it considers both the moon phase and the solar year to determine leap years. It’s interesting to study the different approaches various calendars take to calculate leap years.
Leap Second
 In addition to leap years, there is another concept called a “leap second.” Leap seconds are added to the Coordinated Universal Time (UTC) to keep it synchronized with the Earth’s rotation. The Earth’s orbit is not uniform and varies due to tides, atmospheric pressure, and earthquakes. Therefore, leap seconds are added to the UTC to keep it aligned with the Earth’s rotation.Â
Applications of Leap Years
Leap years are used in various applications, including astronomy, finance, and computer science. For example, leap years are used to calculate the position of planets and stars in the sky. In finance, leap years are used to calculate the interest on loans and bonds. In computer science, leap years are used to calculate the number of days between two dates.
Limitations of Leap Years
While leap years are an effective way to keep the calendar in sync with the solar year, they could be better. For example, the Gregorian calendar assumes that the solar year is 365.2425 days long, while in reality, it is approximately 365.2422 days long. It means that the Gregorian calendar will drift out of sync with the solar year over time. The International Earth Rotation and Reference Systems Service (IERS) adds leap seconds to the UTC to mitigate this issue.
Conclusion
In conclusion, leap years are an essential concept in the Gregorian calendar, and they play an important role in keeping the calendar in sync with the solar year. In this article, we have discussed the algorithm to check whether a given year is a leap year in various programming languages such as C, C++, JAVA, C#, PHP, and PYTHON.
We have also covered some additional aspects related to leap years, such as their calculation for other calendars, the concept of leap seconds, and their applications and limitations.
We hope this article has provided a comprehensive understanding of leap years and their importance in various fields.
FAQs
Q: What is a leap year?
A leap year is a year that is divisible by 4, except for years that are divisible by 100 but not by 400. Leap years have 366 days instead of the usual 365 days.
Q: Why do we have leap years?
Leap years are necessary to keep the calendar in sync with the solar year, which is approximately 365.2422 days long. With leap years, the calendar would stay in sync with the seasons.
Q: What is the algorithm to check if a year is a leap year?
The algorithm to check if a year is a leap year is as follows: If the year is divisible by 4 and not divisible by 100, or if the year is divisible by 400, then it is a leap year.
Q: What other calendars use leap years?
Many calendars other than the Gregorian calendar use the concept of leap years to keep their calendars in sync with the solar year. For example, the Islamic and Chinese calendars use leap years but have different methods for calculating them.
Programs you might also like:
- Bubble sort programs in C, C++, JAVA, and PYTHON
- Program to copy all elements of an array into another array
- Program to print prime numbers from 1 to N
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- Fibonacci sequence: Fibonacci series in C# (with examples)
- Program to Convert Fahrenheit to Celsius: Algorithm, Formula, and Code Examples
- Converting Celsius to Fahrenheit in C#
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025