Problem Statement:
Consider a scenario where you have a string and need to capitalize the first letter of each word in that string. For example, if the input string is “hello world,” the output should be “Hello World.”
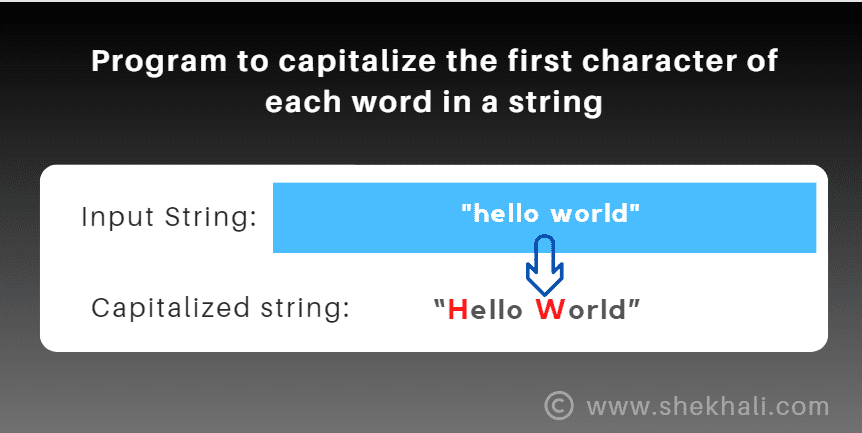
Enhance your coding skills in C#, C, C++, Java, Python, and PHP with this comprehensive list of programming examples and programs. Start coding and practicing your skills today!
Consider a scenario where you have a string and need to capitalize the first letter of each word in that string. For example, if the input string is “hello world,” the output should be “Hello World.”
In this article, we’ll learn how to write a C# program to find the longest word in a string. We’ll break down the problem, provide multiple code examples, and explain each step.
In this article, we’ll learn how to reverse an array in C# with multiple examples and code explanations.
In this article, we’ll explore how to reverse a number and string in C# with clear code examples and detailed explanations. Understanding these fundamental concepts is crucial whether you’re an experienced developer or just starting. Let’s dive in.
In this article, we will learn how to write C# program to find all duplicate elements in an integer array and display how many times they occurred. We will write multiple C# code examples with proper code explanation.
In this article, we will discuss how to write a C# program to rotate an array by k position.
Understanding Array Rotation:
Array rotation involves shifting the elements of an array to the right or left by a specified number of positions. Here, we want to rotate an array to the right, focusing on a pivot element.
An array is a collection of similar data types stored in contiguous memory locations.
In this article, we will discuss how to find the missing number in an array of 1 to 10 using C#, Python, Java, and C programming languages.
Array definition: An array is a data structure that can store multiple values of the same type in a contiguous block of memory.
Arrays can have one or more dimensions, depending on how many indices are needed to access an element. A one-dimensional (1D) array is often referred to as a vector, while a two-dimensional (2D) array is commonly known as a matrix.
In this article, we will discuss some of the common methods to convert a 2D array into a 1D array using C#, Python, Java, and C programs with code examples.
In this article, we will walk through writing a program to find the maximum and minimum number in an array in C#, C++, Python, and Java.
In this article, we’ll write a program to remove duplicate elements from an array using C#, C++, Python, and Java examples.
In this article, we’ll write a program to find largest number in an array using programming languages such as C#, C++, Python, and Java.
An array is a data structure that allows the storage of a collection of elements of the same data type under a single identifier. Array elements are stored in contiguous memory and are accessed using numeric indexes starting at 0.
Arrays provide a structured way to organize and manage data and allow developers to work with multiple values of the same data type within a single variable.
In this article, we will learn about Armstrong numbers and create a C# program to check whether a given number is an Armstrong number or not.
In this article, we’ll delve into the details of converting a hexadecimal number to its decimal equivalent.
Introduction:
In this article, we will learn how to write a C# program to convert decimal to binary with examples.
Whether you’re a beginner eager to grasp the basics or an experienced developer looking to refresh your knowledge, this guide is tailored for you.
Introduction:
In this article, we’ll explore how to write a C# Program to Convert Binary to Decimal.
In this article, we’ll explore how to create a simple C# Program to Print Multiplication Table of a Given Number. This program is helpful for both beginners and professionals.
Palindrome – What’s That?
A palindrome is a sequence of characters that reads the same forward and backwards. In the context of numbers, A palindrome number is the same number when reversed.
In this article, we’ll learn how to write a Palindrome program in C#.
Swapping two numbers is a fundamental task in programming, and in this article, we will write a C# Program to Swap Two Numbers in various ways.
In this article, we’ll explore how to write a C# program to check if a Given Number is Even or Odd.
In this article, we’ll discuss how to count vowels and consonants in a string in the C# program.
Let’s start with a simple C# program that count vowels and consonants in a string. Below is the complete code, and we’ll break it down step by step.