Stored procedures and functions are database objects which contain a set of SQL statements to execute a task. Both are different in many respects.
In this post, We will learn the differences between stored procedures and functions.
Before diving into the differences, it is important to understand the fundamental concepts such as stored procedures, functions, advantages, basic syntax, etc.
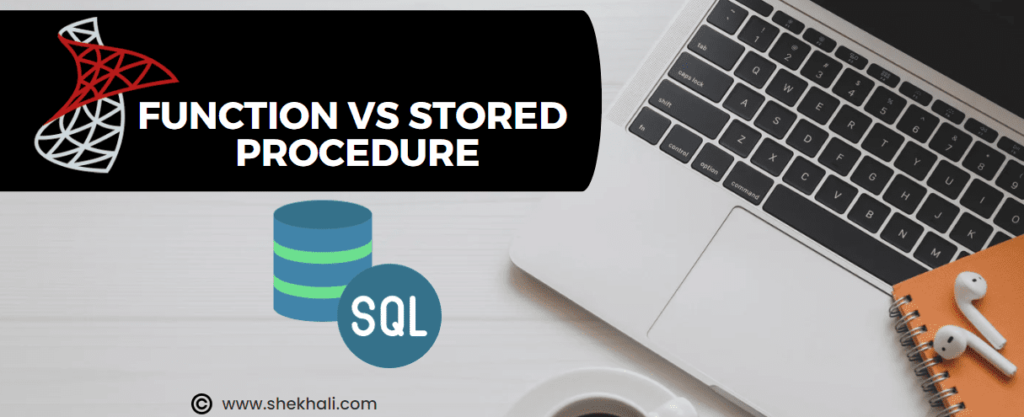
Table of Contents
- 1 What is stored procedure?
- 2 What is function in SQL?
- 3 Basic Difference Between Stored Procedure and Function in SQL Server
- 4 Function vs Stored Procedure in SQL
- 5 FAQs
- 5.1 Q: What is a stored procedure in SQL Server?
- 5.2 Q: Which is faster, a stored procedure or a function?
- 5.3 Q: Can you call a function in a stored procedure?
- 5.4 Q: Is it possible for a procedure to return a value?
- 5.5 Q: Why would you create a SQL procedure rather than a function?
- 5.6 Q: When would a function be preferable to a stored procedure in SQL?
- 5.7 Q: Can both Stored Procedures and Functions accept parameters?
- 5.8 Q: How are exceptions handled in Stored Procedures and Functions?
- 5.9 Q: What operations can be performed in Stored Procedures and Functions?
- 6 Summary
What is stored procedure?
In SQL Server, a Stored procedure is a collection of pre-compiled SQL statements saved in the database. It is like a reusable set of instructions or commands that can be called and executed over and over again.
The stored procedure accepts input and output parameters, performs DML operations or calculations, and may or may not return a value.
Now, why should we use stored procedures? Here are a few reasons:
- Reusability: Once you create a stored procedure, you can use it multiple times without having to rewrite the same code again and again. It saves you time and effort.
- Improved Performance: When you run a stored procedure for the first time, SQL Server creates a plan to execute it. This plan is saved and reused whenever you run the stored procedure again. As a result, the execution is faster because SQL Server doesn’t need to figure out how to run the commands every time.
- Security: Stored procedures can help protect your data. Instead of giving direct access to tables, you can control who can access the data by granting permissions to execute specific stored procedures. It adds a layer of security to your database.
- Efficiency: Stored procedures are pre-compiled, which means they are prepared and optimized before you even run them. It makes them faster than writing ad-hoc queries every time. It’s like having a well-organized kitchen where all the ingredients are easily accessible, saving you time and effort.
- Maintenance: If you need to change your database logic, you only need to update the stored procedure. You don’t have to modify every single part of your application that uses that logic. It makes it easier to maintain and update your code.
A stored procedure is a pre-compiled collection of SQL commands that we can reuse multiple times. It’s like having a ready-to-use script stored in the database.
To learn more about the stored procedure, please refer to the article Different types of Stored Procedures in SQL Server.
Syntax to create the stored procedure
CREATE PROCEDURE <procedure_name>
AS
BEGIN
<sql_statements>
END
Syntax to execute the stored procedure
EXECUTE <procedure_name> <parameters seprated by comma>
What is function in SQL?
In SQL Server, a function is a reusable database object that encapsulates a set of SQL statements to perform specific tasks. Functions only accept input parameters, process data, and return a single value, such as a scalar value, table variable, or table result set.
They help to simplify complex queries, promote code modularity, and enhance code reusability within the database system.
Syntax to create a user-defined function (UDF)
CREATE FUNCTION <function_name> (<@paramater_name> <datatype>)
RETURNS <return_datatype>
AS
BEGIN
<SQL statements>
RETURN expression
END
Syntax to execute a user-defined function (UDF)
SELECT * FROM <Function_name>(Parameter)
-- OR
SELECT Field1,Field2 <function_name(parameters)> FROM table_name
Basic Difference Between Stored Procedure and Function in SQL Server
- Stored procedures are used to perform actions in the database, such as inserting or updating data. They can also retrieve data, but their main purpose is executing tasks. On the other hand, functions are used to calculate and return specific values based on input parameters from the database.
- A stored procedure can return zero or multiple values, and returning values is optional. On the other hand, a function is always bound to return a value and can only return a single value.
- In SQL Server, functions are limited to input parameters, while procedures can have both input and output parameters.
- In SQL, you can invoke functions from within stored procedures, but you cannot call procedures from functions.
- In SQL Server, stored procedures do not require a return type since they are not bound to return any value. Conversely, functions have a specified return type that indicates the data type of the value they will always return.
- The procedure allows SELECT and DML(INSERT/UPDATE/DELETE) statements, whereas Function allows only SELECT statements.
Function vs Stored Procedure in SQL
The following table describes the differences between functions and stored procedures in a SQL Server database.
No. | Stored Procedure (SP) | User Defined Function (UDF) |
---|---|---|
01. | Stored Procedures can return zero, single, or multiple values. | Functions must return a single value, which can be either a scalar or a table. |
02. | Stored Procedures can have both input and output parameters. | User Defined Functions can only accept input parameters. |
03. | In procedure we can use Try-Catch blocks to handle exceptions. | Try-Catch blocks cannot be used in Functions. |
04. | Stored Procedures can call functions. | Functions cannot call Stored Procedures. |
05. | Stored Procedures cannot be used in a SELECT statement. | Functions can be used in a SELECT statement. |
06. | Stored Procedures can perform various operations on database objects, including select and DML (INSERT/UPDATE/DELETE) statements. | Functions in SQL are limited to only SELECT statements. |
07. | Transactions can be used in Stored Procedures. | Functions do not support transactions. |
08. | Stored Procedures cannot be used in the Join clause. | User Defined Functions can be used in the Join clause as a result set. |
09. | Stored Procedures can have both table variables and temporary tables. | Functions can only have table variables and are not allowed to use temporary tables. |
10. | Stored Procedures (SP) cannot be used in the WHERE , HAVING , or SELECT statement of SQL queries. | However, User Defined Functions (UDF) can be used in the SELECT , WHERE , or HAVING statement. |
Example: Stored Procedure
The following is an example of creating a Stored procedure in SQL Server to add two numbers.
-- Create a sample stored procedure
Create procedure AddNumbers(@num1 float, @num2 float)
as
begin
print 'The sum of '+cast(@num1 as varchar)+' + '+cast(@num2 as varchar)+' = '+ cast((@num1+ @num2) as varchar)
end
-- Execute stored procedure to print two numbers
Execute AddNumbers 20, 30;
Output:
If you execute the above code, you will be able to see the following result:
The sum of 20 + 30 = 50
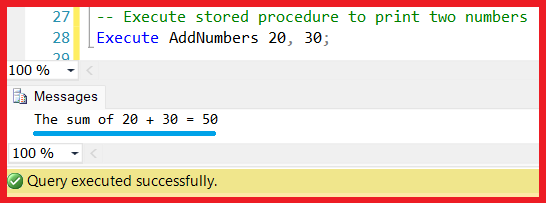
Example: Function (UDF) in SQL Server
Let’s create a sample example of a Scalar Function (UDF)
-- Create a sample user defined function in SQL Server
Create function fn_AddNumbers(@num1 int,@num2 int)
returns int
as
Begin
Return @num1 + @num2
End
-- Calling function in the SELECT statement
Select dbo.fn_AddNumbers(20,30) as result;
-- result: 50
FAQs
Q: What is a stored procedure in SQL Server?
A stored procedure is a precompiled set of SQL statements stored in the database. It can accept input and output parameters, perform SQL operations, and return a result set If needed.
Q: Which is faster, a stored procedure or a function?
In general, stored procedures are often faster than functions because they can be compiled and reused, resulting in improved performance for subsequent executions.
Q: Can you call a function in a stored procedure?
A Stored Procedure can call a Function. However, a Function cannot directly call a Stored Procedure.
Q: Is it possible for a procedure to return a value?
A stored procedure cannot return value but may accept input/output parameters. A stored procedure can use an output parameter to return results.
Q: Why would you create a SQL procedure rather than a function?
A Stored Procedure supports SELECT and DML (INSERT/UPDATE/DELETE) statements, whereas a Function only supports SELECT statements. We can’t use procedures in SELECT statements, but Functions can.
Q: When would a function be preferable to a stored procedure in SQL?
We cannot use stored procedures anywhere in a SQL statement’s SELECT, WHERE, or HAVING section, but functions can.
Q: Can both Stored Procedures and Functions accept parameters?
Yes, both Stored Procedures and Functions can accept input parameters. However, Stored Procedures can also have output parameters, whereas Functions can only accept input parameters.
Q: How are exceptions handled in Stored Procedures and Functions?
Stored Procedures can use the Try-Catch block to handle exceptions, whereas Functions cannot use the Try-Catch block for exception handling.
Q: What operations can be performed in Stored Procedures and Functions?
Stored Procedures can perform various operations on database objects, including SELECT and DML statements like INSERT, UPDATE, and DELETE. Functions, on the other hand, are limited to performing only SELECT statements.
Summary
This blog provides detailed information about SQL Server stored procedures and functions. Now you understand the difference between stored procedures and functions and how to use them for your basic requirement.
- In SQL Server, A function must return a value, which is optional in a Stored Procedure.
- A Function can only have input parameters, whereas Procedures can have input and output parameters.
- You can call a function from a stored procedure, but you cannot call a procedure from a function in SQL Server.
Reference: tutorials point: function vs stored procedure
You might want to read this too:
- Having VS Where Clause in SQL
- Function vs Stored procedure in SQL Server
- Stored Procedure in SQL Server – A Complete Guide [with Examples]
- COALESCE in SQL With Examples and Use Cases for Handling NULL Values
- SQL Server CONVERT Function: How to Convert Data Types in SQL Server
- SQL Comparison Operators (Less than, Greater than, Equal, Not Equal operators)
- Primary Key Constraint In SQL Server
- View In SQL | Types Of Views In SQL Server
- SQL Server Trigger Update, Insert, Delete Examples
- SQL pivot tables
- Different ways to delete duplicate rows in SQL Server
- UNION vs UNION ALL in SQL SERVER
- SQL Server Try Catch: Error Handling in SQL Server With [Examples]
- Group by Month in SQL
Let others know about this post by sharing it and leaving your thoughts in the comments section.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025