Property in C# is a member of a class that allows classes to read, write, and expose private fields while keeping the implementation details hidden.
In this post, we will try to understand how to use properties in C# with multiple examples.
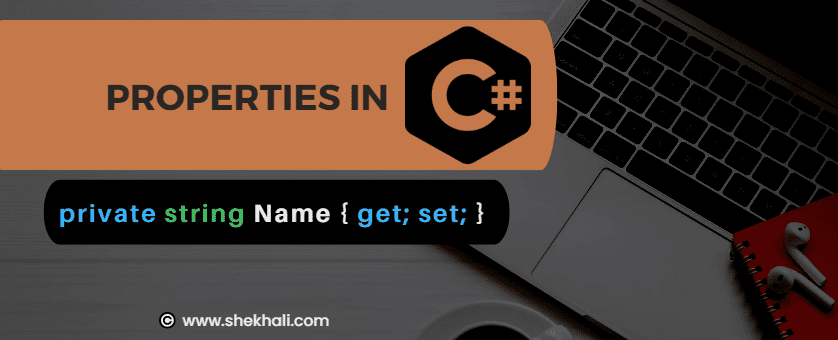
Table of Contents
Understanding properties in C#
C# Properties are special methods used to assign and read the value from a private field by using set and get accessors. A get accessor returns a property value, whereas a set accessor assigns a new value.
- C# Properites don’t have a storage location, unlike fields. Instead, they have accessors to read, write, and compute the values.
- Properties offer another level of abstraction to expose the private fields as we can’t directly access the private fields outside the class scope.
( get : Retrieve the value set : Assign the value)
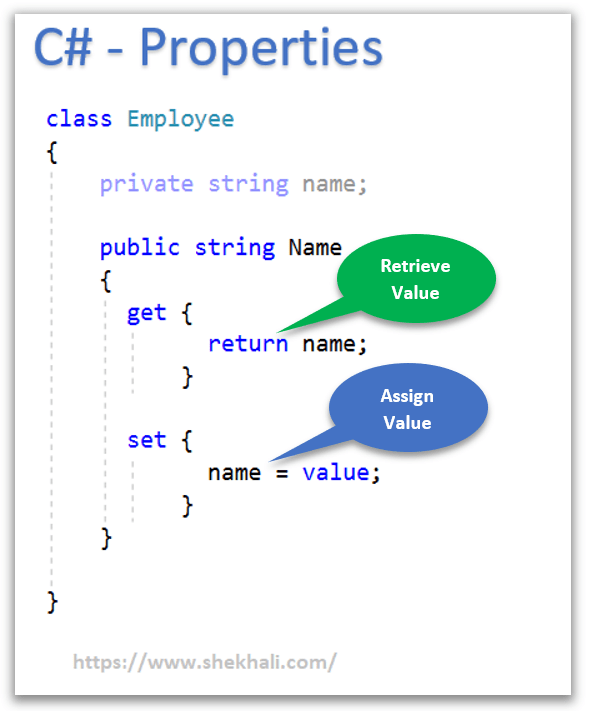
C# Property Syntax
The following is the syntax to define a property in C#.
<Access Modifier> <Return Type> <Property Name>
{
get {
// get accessor
// return value
}
set {
// set accessor
// assign a new value
}
}
Example: Property in C#
The following example shows how to create a property in C#.
public class Employee
{
private string name; // Private field to store the name
// Property with get and set accessors
public string Name
{
get
{
return name; // Get the value of the name
}
set
{
name = value; // Set the value of the name
}
}
}
Code Explanation:
We define a C# class called Employee
with a private field called name
to store the employee’s name. It also includes a public property named Name
, which has both a “get” and a “set” accessor.
- The
get
accessor allows you to retrieve or get the value of the private name field. - The
set
accessor will allow you to assign or set a new value to the name field.
The get and set accessors are not only limited to reading or writing the value to the property but also allow us to perform complex calculations or business logic within the set or get blocks.
Apply specific rules using property in C#
Let’s create a C# code example that demonstrates how properties are used to enforce encapsulation and apply specific rules or constraints to the underlying data of a class. In this example, we’ll create a BankAccount
class with a Balance property with a get and set accessor that enforces restrictions on the account balance.
using System;
public class BankAccount
{
private decimal balance; // Private field to store the balance
// Property with get and set accessors for the balance
public decimal Balance
{
get
{
return balance; // Get the current balance
}
set
{
if (value < 0)
{
// Don't allow negative balances
Console.WriteLine("Error: Balance cannot be negative.");
}
else
{
balance = value; // Set the balance if it's non-negative
}
}
}
}
public class Program
{
public static void Main()
{
// Create a new bank account
BankAccount account = new BankAccount();
// Try to set a negative balance
account.Balance = -1000; // This will trigger the constraint check
// Check the balance (it should not have changed due to the constraint)
Console.WriteLine("Account Balance: $" + account.Balance);
// Deposit money into the account
account.Balance += 2000;
// Check the updated balance
Console.WriteLine("Deposit money: Account Balance: $" + account.Balance);
// Withdraw money from the account (if sufficient balance)
account.Balance -= 500;
// Check the updated balance
Console.WriteLine($"Withdraw money: {500}");
// Check the updated balance
Console.WriteLine("Updated Balance: $" + account.Balance);
Console.ReadKey();
}
}
Output:
Error: Balance cannot be negative.
Account Balance: $0
Deposit money: Account Balance: $2000
Withdraw money: 500
Updated Balance: $1500
What are the types of properties in C#?
Following is the list of different types of properties in C#.
S.NO | C# Property Types | Description | Use Cases |
---|---|---|---|
01. | Read-Write Property: | Contains both get and set accessor. | Read-Write property allows you to read and modify the value of the property. |
02. | Read-Only Property: | Contains a get accessor only. | Read-Only property typically used when you want to provide read-only access to a property. |
03. | Write-Only Property: | Contains a set accessor only. | Write-Only property is useful when you need to set a property value but don’t want to allow reading. |
04. | Auto-implemented Property: | Contains both get and set accessors without logic. | Auto implemented property is a concise way to define properties without writing explicit getter and setter methods. |
C# readonly property
The property that has only the get
accessor and no set
accessor is called readonly
property.
it allows you to retrieve the value of a property but doesn’t allow you to change or set a new value for that property once it’s been initialized.
It is often used when you want to provide read-only access to a value while preventing any modifications.
Key characteristics of a read-only property:
- No Set Accessor: A read-only property does not have a “set” accessor. This means you can’t assign a new value to the property after it’s been initially set.
- Initialization: Typically, the value of a read-only property is set during object initialization or within the constructor of the class. Once set, the read-only property’s value cannot be changed.
A compilation error occurs when you try to set the value of the read-only field/property.
class Employee
{
private string name;
// ReadOnly Property
public string Name
{
get {
return name;
}
}
// Constructor
public Employee(string name)
{
this.name = name;
}
}
public class Programs
{
public static void Main(string[] args)
{
Employee employee = new Employee("Shekh Ali"); // Constructor
string name = employee.Name; // Calling get block of the ReadOnly property
Console.WriteLine($"Name : {name}");
employee.Name = "Mark Adams"; // Calling set block to assign the new value.
Console.WriteLine($"Name : {employee.Name}");
Console.ReadLine();
}
}
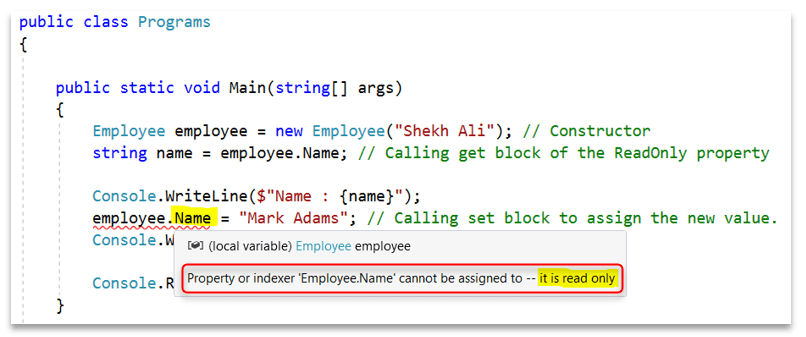
As we can see in the above example, It throws an error when we try to set the value to the property outside the constructor.
Write Only Properties in C#
The Write-Only property has only the set
block and not the get
block. It allows us to only set the values and not to read the value.
If we try to read the value, It will throw an error message.
private string name;
// Write Only Property
public string Name
{
set {
name =value;
}
}
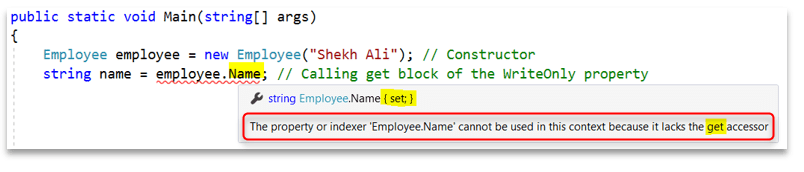
Auto Implemented Properties in C#
In C#, An auto-implemented property contains both the get accessor and set accessor without having any business logic. It makes code more clean and readable and used when no additional business logic or calculation is needed in the get or set block.
The properties which do not require any code to be written inside the get method and set method of the class are known as Auto Implemented Properties in C#.
Key characteristics of auto-implemented properties in C#:
- No Explicit Field: With auto-implemented properties, you don’t need to declare an explicit private field to store the property’s value. The compiler generates an anonymous backing/private field for you.
- Simplified Syntax: Auto-implemented properties use a simplified syntax, reducing the amount of code you need to write compared to traditional properties with explicit backing fields.
Here’s a simple example of an auto-implemented property in C#:
// Auto Implemented Property
public string Name { get; set; }
In the case of auto-implemented properties, the compiler internally creates private fields that can only be accessed through the property’s getter or setter.
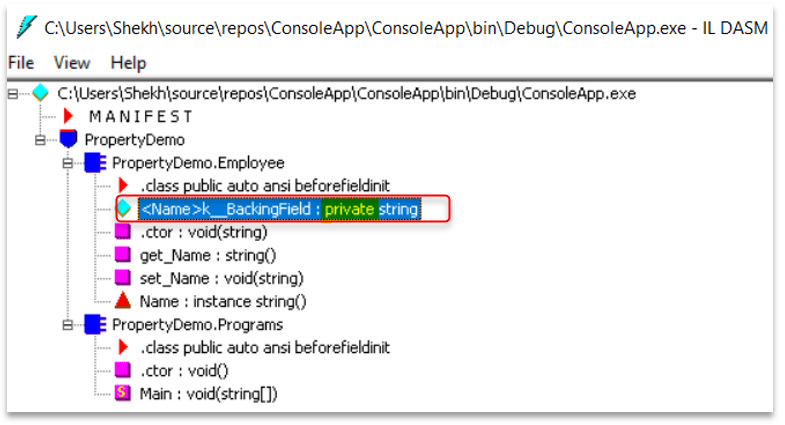
You can also apply access modifiers and customize the access to the get and set methods if needed. For example, you can make the set accessor private to allow setting the property value only from within the class:
public class Person
{
public string FirstName { get; private set; } // Auto-implemented property with private set accessor
public string LastName { get; private set; } // Auto-implemented property with private set accessor
}
In C#, Auto-implemented properties are beneficial when you need straightforward properties that don’t require additional logic or validation.
Static Properties in C#
In C#, A property that is created using the static
keyword is known as a static property.
A static property can access directly by the class name without creating an instance of that class because the static property belongs to the class rather than the object of the class.
A static property will only allow you to use the static variables inside the getter and setter block.
using System;
namespace PropertyDemo
{
public class Employee
{
// Static field
private static string name;
// Static Property
public static string Name
{
get
{
return name;
}
set
{
name = value;
}
}
}
public class Programs
{
public static void Main(string[] args)
{
// Accessing static property by the class name.
Employee.Name = "Shekh Ali"; // Set Value
Console.WriteLine($"Name : {Employee.Name}"); // Get Value
}
}
}
C# properties vs fields
In C#, a field is a variable of any type that is declared directly in the class, whereas a property is a member that provides a flexible mechanism to read, write, or compute the value of a private field.
- Fields: Fields are variables that can be directly declared within a class or struct. They are typically kept as private members of a class, allowing access through public
get
andset
properties. Fields are essential for data encapsulation, which is a fundamental principle in Object-Oriented design. - Properties: In C#, a property is a class member that offers a versatile way to read, write, or compute data from a private field. Properties introduce a level of abstraction, enabling us to modify the field’s value without impacting how it’s used within a class.
We can encapsulate the business logic in properties, and adding an extra level of validation in the get and set accessor is acceptable but only sometimes recommended.
Conclusion
This article has provided us with the following insights:
- Use of the properties and its Syntax.
- About the write-only and
readonly
property in C# - Use of static property
- Use of Auto implemented properties in C#
I hope you enjoyed and found this post useful. If you have any questions or comments, please leave them below.
References: MSDN- Properties in C#
FAQs: Properties in C#
Q1: What is a property in C#?
A property in C# is a class member that allows controlled access to the data or attributes of an object. It defines how you can read, write, or compute the value of a private field.
Q2: How does a property differ from a field?
A field is a variable that directly stores data within a class or struct, while a property provides an interface to access that data. Properties often include logic to control access or manipulate data, whereas fields are typically raw data storage.
Q3: What are the key components of a C# property?
A C# property consists of a name, a data type, and get
and set
accessors. The get accessor retrieves the value, and the set accessor assigns a new value.
Q4: What is a read-only property in C#?
A read-only property is a property that only has a “get” accessor, meaning it allows you to retrieve the value but not set a new value. It provides read-only access to data.
Q5: What is an auto-implemented property in C#?
An auto-implemented property is a simple property that automatically generates the underlying code for storing and retrieving data. It’s used when no additional logic is required for the property.
Recommanded Articles:
- 10 Difference between interface and abstract class In C#
- C# Dynamic Type: Var vs dynamic keywords in C#
- C# 10 New features with examples | What’s new in C# 10?
- C# Array vs List
- C# Monitor class in multithreading with examples
- Exception Handling in C#| Use of try, catch, and finally block
- C# Enum | How To Play With Enum in C#?
- C# extension methods with examples
- Properties In C# with examples
- IEnumerable Interface in C# with examples
- Constructors in C# with Examples
- Top 10 Differences between Stored Procedure and Function in SQL Server
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024