This article will delve into the TRY…CATCH statement in SQL Server and its usage for error handling.
Additionally, we’ll examine how the try-catch statement in SQL Server can be used to manage errors and present more informative error messages to the end-users.
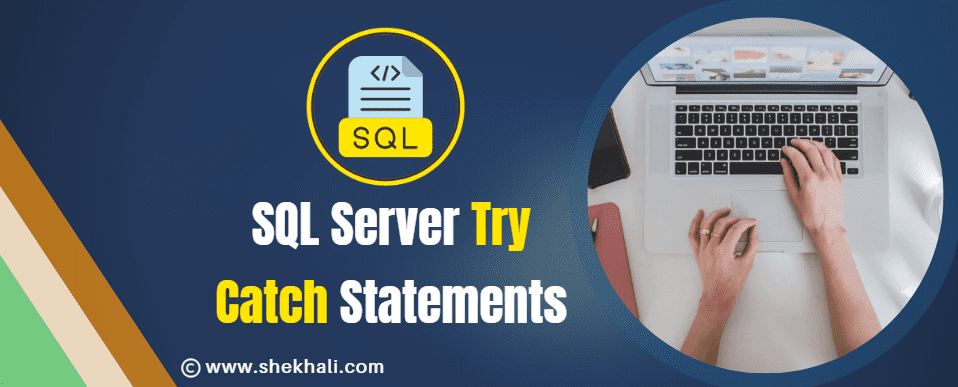
Table of Contents
- 1 Introduction to SQL Server Try Catch statements
- 2 Error Handling in SQL Server with Try-Catch
- 3 Using SQL Server Try Catch With Transactions
- 4 Using a try-catch statement within a transaction in SQL Server
- 5 Advantages of using the try catch in SQL Server
- 6 FAQs
- 6.1 Q: What is a try-catch statement in SQL Server?
- 6.2 Q: How can you use a try-catch statement within a transaction in SQL Server?
- 6.3 Q: What is the difference between a SQL Server try catch statement and an error check using IF @@ERROR <> 0?
- 6.4 Q: Can a try-catch statement be nested within another try-catch statement?
- 6.5 Related
Introduction to SQL Server Try Catch statements
The try-catch statement in SQL Server is used to handle exceptions that occur during the execution of a T-SQL statement. It provides a way to capture and process errors in a controlled manner, rather than having the query or stored procedure fails abruptly.
Syntax:
The basic structure of a try-catch statement in SQL Server is as follows:
BEGIN TRY
-- T-SQL statements that may cause an error
END TRY
BEGIN CATCH
-- Code to handle the error
END CATCH
The try block contains the T-SQL statements that may cause an error. If an error occurs, control is transferred to the catch block, where the error can be handled and processed.
Error Handling in SQL Server with Try-Catch
When an error occurs in a T-SQL statement, SQL Server raises an error and terminates the execution of the current batch. The try-catch statement can be used to handle these errors and continue processing.
Example 1: try catch in SQL
Here is an example of using the try-catch statement to handle a divide-by-zero error:
BEGIN TRY
DECLARE @x INT = 7, @y INT = 0;
DECLARE @result INT = @x / @y;
END TRY
BEGIN CATCH
PRINT 'A divide-by-zero error occurred.';
END CATCH
In this example, if a divide-by-zero error occurs, control is transferred to the catch block, where a message is printed indicating that the error occurred. The query does not fail, and processing continues.
Using SQL Server Try Catch With Transactions
The try-catch statement can also be used in conjunction with transactions to handle errors and ensure that the database remains in a consistent state.
Here is an example of using the try-catch statement within a transaction:
Syntax:
BEGIN TRANSACTION;
BEGIN TRY
-- T-SQL statements that may cause an error
COMMIT TRANSACTION;
END TRY
BEGIN CATCH
PRINT 'An error occurred.';
ROLLBACK TRANSACTION;
END CATCH
In this example, if an error occurs within the try block, control is transferred to the catch block, where the transaction is rolled back to ensure that the database remains in a consistent state.
Function Name | Description |
---|---|
ERROR_NUMBER: | It returns the internal number of the error. |
ERROR_STATE: | It returns information about the source of the error. |
ERROR_SEVERITY: | It returns information about the severity of the error, ranging from informational errors to fixable errors. |
ERROR_LINE: | It returns the line number at which the error occurred. |
ERROR_PROCEDURE: | It returns the name of the stored procedure or function where the error occurred. |
ERROR_MESSAGE: | It returns the most important information, which is the message text of the error. |
This is an example of using the try-catch statement in SQL Server to handle an error. The script has two main parts: the try block and the catch block.
The try block contains a SELECT statement that intentionally generates an error by dividing by zero. The statement 5 / 0 will result in a divide-by-zero error.
The catch block is used to handle the error that occurred in the try block. The catch block contains a SELECT statement that returns information about the error using several system functions.
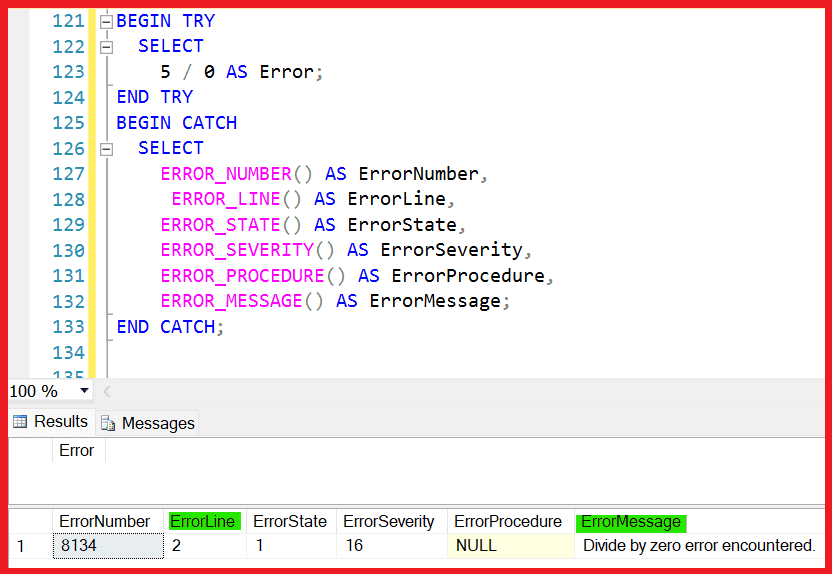
Example 2: Handling Null Value Errors
To understand this example, Let’s first create a Customers table in SQL Server and insert some records into it.
-- Creating customer table.
CREATE TABLE Customers
(
CustomerID INT PRIMARY KEY IDENTITY(1,1),
FirstName NVARCHAR(50) NOT NULL,
LastName NVARCHAR(50) NOT NULL,
Email NVARCHAR(100) NOT NULL,
Phone NVARCHAR(20) NOT NULL
);
-- Inserting few records into the table.
INSERT INTO Customers (FirstName, LastName, Email, Phone)
VALUES ('Shekh', 'Ali', 'shekh.ali@example.com', '555-555-5555'),
('Rohit', 'Kumar', 'rohit.kumar@example.com', '555-555-5556'),
('Will', 'Smith', 'will.smith@example.com', '555-555-5557');
We have created a table named Customers with five columns: CustomerID, FirstName, LastName, Email, and Phone. The CustomerID column is defined as the primary key and is set to auto-increment (IDENTITY). The other columns are defined with their respective data types and a NOT NULL constraint, which means that a value must be provided for these columns when inserting a new record into the table.
CustomerID | FirstName | LastName | Phone | |
---|---|---|---|---|
1 | Shekh | Ali | shekh.ali@example.com | 555-555-5555 |
2 | Rohit | Kumar | rohit.kumar@example.com | 555-555-5556 |
3 | Will | Smith | will.smith@example.com | 555-555-5557 |
Now, let’s try to use the SQL try-catch statement to handle null value errors, which can occur when we try to insert a null value into a column that does not allow null values. The following code demonstrates how to use the try-catch statement to handle this type of error:
BEGIN TRY
INSERT INTO Customers (FirstName, LastName, Email, Phone)
VALUES ('Shekh', 'Ali', NULL, '555-555-5555')
END TRY
BEGIN CATCH
PRINT 'Error: Cannot insert a null value into the Email column.';
END CATCH
Output:
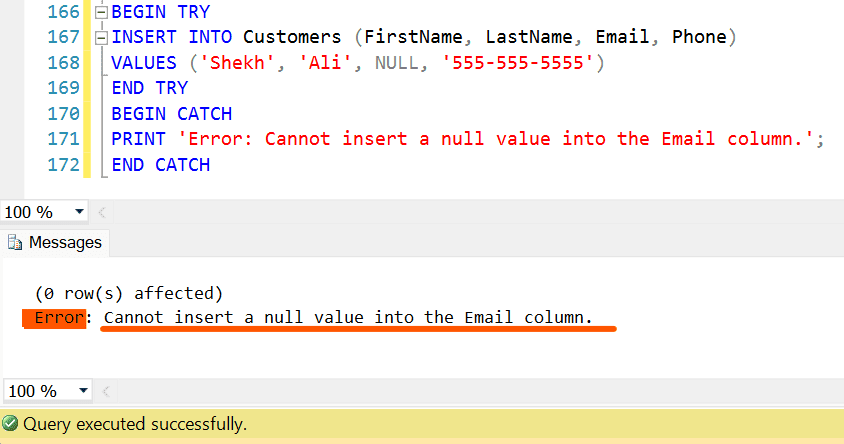
Using a try-catch statement within a transaction in SQL Server
The following is a complete example of using a try-catch statement within a transaction in the SQL Server:
BEGIN TRANSACTION
BEGIN TRY
UPDATE Customers
SET Phone = '555-555-1212'
WHERE CustomerID = 1;
-- This statement will generate an error
UPDATE Customers
SET Email = NULL
WHERE CustomerID = 2;
END TRY
BEGIN CATCH
SELECT
ERROR_NUMBER() AS ErrorNumber,
ERROR_STATE() AS ErrorState,
ERROR_SEVERITY() AS ErrorSeverity,
ERROR_LINE() AS ErrorLine,
ERROR_PROCEDURE() AS ErrorProcedure,
ERROR_MESSAGE() AS ErrorMessage;
-- Rollback the transaction
ROLLBACK TRANSACTION;
END CATCH;
-- If there were no errors, commit the transaction
IF @@TRANCOUNT > 0
COMMIT TRANSACTION;
In this example, a transaction is started with the BEGIN TRANSACTION
statement. Within the transaction, there’s a try-catch statement. The try block contains two UPDATE statements. The first statement updates the phone number of the customer with CustomerID 1, while the second statement generates an error by trying to update an invalid email address.
The catch block is used to handle any errors that occur in the try block. If an error occurs, the catch block will be executed, and information about the error will be displayed using the system functions ERROR_NUMBER()
, ERROR_STATE()
, ERROR_SEVERITY()
, ERROR_LINE()
, ERROR_PROCEDURE()
, and ERROR_MESSAGE()
.
After catching the error, the transaction is rolled back using the ROLLBACK TRANSACTION statement, so any changes made within the transaction are discarded. If there were no errors in the try block, the transaction will be committed using the COMMIT TRANSACTION statement. The @@TRANCOUNT variable is used to check if a transaction is still active, and the transaction will be committed only if the count is greater than zero.

Advantages of using the try catch in SQL Server
- Improved error handling: The try-catch statement in SQL Server provides a structured way of handling errors which helps you to easily manage and resolve the issues as they arise.
- Enhanced code quality: By using the try-catch statement, you can avoid having to sprinkle error-checking code throughout your scripts, making the code easier to read and maintain.
- Better error reporting: The try-catch statement provides a range of system functions like ERROR_NUMBER(), ERROR_STATE(), and ERROR_MESSAGE() that allow you to capture and report detailed information about the errors that occur in your code.
- Better control over transactions: When using the try-catch statement within a transaction, you can better control how errors are handled and ensure that the transaction is either fully committed or fully rolled back, depending on the outcome.
- Efficient error handling: The try-catch statement provides a way of handling errors that is much more efficient than using traditional error handling methods like checking the @@ERROR variable after each statement.
FAQs
Here are some frequently asked questions and answers related to the SQL Server Try Catch statements.
Q: What is a try-catch statement in SQL Server?
A try-catch statement is a way to handle errors in SQL Server, which allows you to execute a block of code and catch any errors that occur during its execution. The try block contains the code that might raise an error, and the catch block contains the code that handles the error.
Q: How can you use a try-catch statement within a transaction in SQL Server?
To use a try-catch statement within a transaction in SQL Server, you can start the transaction with the BEGIN TRANSACTION
statement and then wrap the code that might raise an error in a try-catch statement. If an error occurs, you can roll back the transaction using the ROLLBACK TRANSACTION
statement in the catch block. If there were no errors, you can commit the transaction using the COMMIT TRANSACTION
statement.
Q: What is the difference between a SQL Server try catch statement and an error check using IF @@ERROR <> 0?
A try-catch statement is a more structured and readable way to handle errors in SQL Server, while the IF @@ERROR <> 0
check is a simple and straightforward way to check for errors. The try-catch statement provides more information about the error and allows you to write a more complex error-handling code, while the IF @@ERROR <> 0
check only checks for the presence of an error and returns the error code.
Q: Can a try-catch statement be nested within another try-catch statement?
Yes, a try-catch statement can be nested within another try-catch statement, which can be useful for handling errors within a catch block and writing more complex error-handling logic.
Reference: MSDN-try catch in sql
You might want to read this too:
- Having VS Where Clause in SQL
- Delete vs Truncate vs Drop in SQL Server
- UNION vs UNION ALL in SQL SERVER
- View In SQL | Types Of Views In SQL Server
- Triggers in SQL Server
- Different ways to delete duplicate rows in SQL Server
- Types of Joins in SQL Server
- Function vs Stored procedure in SQL Server
- SQL pivot tables: Understanding pivot tables in SQL Server
- Group by Month in SQL: A Comprehensive Guide with Examples in SQL Server
Let others know about this post by sharing it and leaving your thoughts in the comments section.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025