In C#, operators are special symbols or characters used to perform specific operations on one or more operands. These operators help in manipulating data and performing various computations on them.
C# has a wide range of operators, such as assignment, arithmetic, relational, logical, unary, ternary, bitwise, and compound assignment operators. Understanding and using these operators correctly is crucial for effective programming in C#.
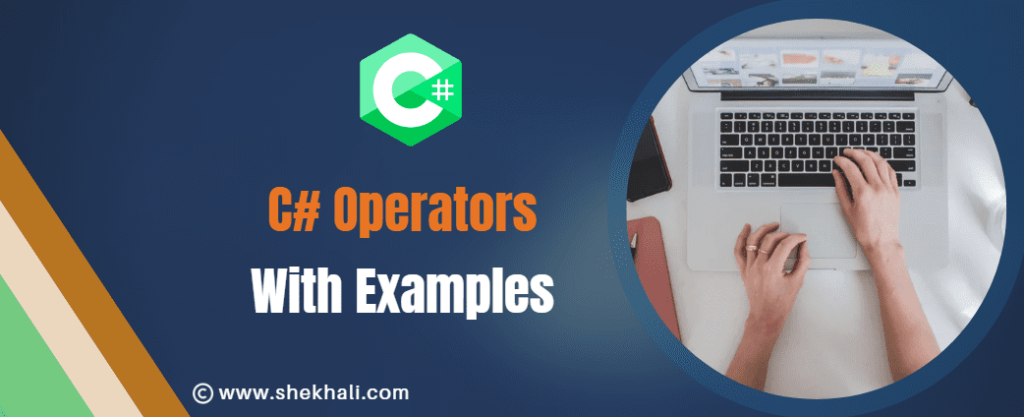
Table of Contents
- 1 C# Operators:
- 2 01. Basic Assignment Operator
- 3 02. Arithmetic Operators
- 4 03. Relational Operators
- 5 04. Logical Operators
- 6 05. Unary Operators
- 7 06. Ternary Operator
- 8 07. Bitwise and Bit Shift Operators
- 9 08. Compound Assignment Operators
- 10 Conclusion
- 11 FAQs
- 11.1 Q: What are C# operators?
- 11.2 Q: How many types of operators are there in C#?
- 11.3 Q: What is the basic assignment operator in C#?
- 11.4 Q: What are arithmetic operators in C#?
- 11.5 Q: What are relational operators in C#?
- 11.6 Q: Q: What are logical operators in C#?
- 11.7 Q: What is the ternary operator in C#?
- 11.8 Related
C# Operators:
The following are the list of C# Operators:
- Basic Assignment Operator
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Unary Operators
- Ternary Operator
- Bitwise and Bit Shift Operators
- Compound Assignment Operators
01. Basic Assignment Operator
The basic assignment operator (=) is used to assign a value to a variable. It takes the value on the right and stores it in the variable on the left.
Example 1: Basic Assignment Operator
int x = 10;
int y = 20;
x = y;
Console.WriteLine(x); // Output: 20
Table 1: Basic Assignment Operator
Name | Description | Example |
---|---|---|
= (Assignment) | Assigns the value on the right to the variable on the left. | int x = 10; |
02. Arithmetic Operators
Arithmetic operators perform mathematical operations such as addition, subtraction, multiplication, division, and modulus (remainder). The available arithmetic operators in C# are +, -, *, /, and % respectively.
Example 2: Arithmetic Operators
using System;
class Program
{ //Example: Arithmetic operators in C#
static void Main()
{
int a = 10;
int b = 5;
// Addition operator
int sum = a + b;
Console.WriteLine($"Addition: {a} + {b} = {sum}");
// Subtraction operator
int difference = a - b;
Console.WriteLine($"Subtraction: {a} - {b} = {difference}");
// Multiplication operator
int product = a * b;
Console.WriteLine($"Multiplication: {a} * {b} = {product}");
// Division operator
int quotient = a / b;
Console.WriteLine($"Division: {a} / {b} = {quotient}");
// Modulus operator
int remainder = a % b;
Console.WriteLine($"Modulus: {a} % {b} = {remainder}");
Console.ReadLine();
}
}
Output:
Addition: 10 + 5 = 15
Subtraction: 10 - 5 = 5
Multiplication: 10 * 5 = 50
Division: 10 / 5 = 2
Modulus: 10 % 5 = 0
Table 2: Arithmetic Operators
Name | Description | Example |
---|---|---|
+ (Addition) | Adds two operands. | int result = x + y; |
– (Subtraction) | Subtracts the second operand from the first operand. | int result = x – y; |
* (Multiplication) | Multiplies two operands. | int result = x * y; |
/ (Division) | Divides the first operand by the second operand. | int result = x / y; |
% (Modulus) | Divides the first operand by the second operand and returns the remainder. | int result = x % y; |
03. Relational Operators
Relational operators are used to compare two values and return a Boolean result (true or false). The available relational operators in C# are ==, !=, >, <, >=, and <= respectively.
Example 3: Relational Operators
using System;
class Program
{
static void Main()
{
int a = 10;
int b = 5;
// Greater than operator
bool greaterThan = a > b;
Console.WriteLine($"{a} > {b} = {greaterThan}");
// Less than operator
bool lessThan = a < b;
Console.WriteLine($"{a} < {b} = {lessThan}");
// Greater than or equal to operator
bool greaterThanOrEqualTo = a >= b;
Console.WriteLine($"{a} >= {b} = {greaterThanOrEqualTo}");
// Less than or equal to operator
bool lessThanOrEqualTo = a <= b;
Console.WriteLine($"{a} <= {b} = {lessThanOrEqualTo}");
// Equal to operator
bool equalTo = a == b;
Console.WriteLine($"{a} == {b} = {equalTo}");
// Not equal to operator
bool notEqualTo = a != b;
Console.WriteLine($"{a} != {b} = {notEqualTo}");
Console.ReadLine();
}
}
Output:
10 > 5 = True
10 < 5 = False
10 >= 5 = True
10 <= 5 = False
10 == 5 = False
10 != 5 = True
Table 3: Relational Operators
Name | Description | Example |
---|---|---|
== (Equal to) | Returns true if the operands are equal. | bool result = x == y; |
!= (Not equal to) | Returns true if the operands are not equal. | bool result = x != y; |
> (Greater than) | Returns true if the first operand is greater than the second operand. | bool result = x > y; |
< (Less than) | Returns true if the first operand is less than the second operand. | bool result = x < y; |
>= (Greater than or equal to) | Returns true if the first operand is greater than or equal to the second operand. | bool result = x >= y; |
<= (Less than or equal to) | Returns true if the first operand is less than or equal to the second operand. | bool result = x <= y; |
04. Logical Operators
Logical operators are used to perform logical operations on Boolean values. The available logical operators in C# are && (logical AND), || (logical OR), and ! (logical NOT), respectively.
Example 4: Logical Operators
using System;
class Program
{
static void Main()
{
bool a = true;
bool b = false;
// Logical AND operator
bool andResult = a && b;
Console.WriteLine($"{a} && {b} = {andResult}");
// Logical OR operator
bool orResult = a || b;
Console.WriteLine($"{a} || {b} = {orResult}");
// Logical NOT operator
bool notResult = !a;
Console.WriteLine($"!{a} = {notResult}");
Console.ReadLine();
}
}
Output:
True && False = False
True || False = True
!True = False
Table 4: Logical Operators
Name | Description | Example |
---|---|---|
&& (Logical AND) | Returns true if both operands are true. | bool result = x && y; |
|| (Logical OR) | Returns true if either operand is true. | bool result = x || y; |
! (Logical NOT) | Returns true if the operand is false and vice versa. | bool result = !(x == y); |
05. Unary Operators
Unary operators are used to perform operations on a single operand. The available unary operators in C# are ++ (increment), — (decrement),! (logical NOT), and ~ (bitwise NOT), respectively.
Example 5: Unary Operators
using System;
class Program
{
static void Main()
{
int a = 5;
// Unary plus operator
int unaryPlus = +a;
Console.WriteLine($"+{a} = {unaryPlus}");
// Unary minus operator
int unaryMinus = -a;
Console.WriteLine($"-{a} = {unaryMinus}");
// Increment operator
int preIncrement = ++a;
Console.WriteLine($"++{a} = {preIncrement}");
int postIncrement = a++;
Console.WriteLine($"{a}++ = {postIncrement}");
// Decrement operator
int preDecrement = --a;
Console.WriteLine($"--{a} = {preDecrement}");
int postDecrement = a--;
Console.WriteLine($"{a}-- = {postDecrement}");
Console.ReadLine();
}
}
Output:
+5 = 5
-5 = -5
++6 = 6
7++ = 6
--6 = 6
5-- = 6
Table 5: Unary Operators
Name | Description | Example |
---|---|---|
++ (Increment) | Adds 1 to the operand. | x++; or ++x; |
— (Decrement) | Subtracts 1 from the operand. | x–; or –x; |
+ (Unary plus) | Indicates a positive value. | int result = +x; |
– (Unary minus) | Indicates a negative value. | bool result = -x; |
! (Logical NOT) | Inverts the value of a Boolean expression. | bool result = !x; |
~ (Bitwise NOT) | Inverts the bits of an integer. | int result = ~x; |
06. Ternary Operator
The ternary operator ?:
is a shorthand way of writing an if-else statement. It takes three operands: the condition to evaluate, the expression to return if the condition is true, and the expression to return if the condition is false.
Example 6: Ternary Operator
using System;
class Program
{
static void Main()
{
int a = 5;
int b = 10;
// Ternary operator
int max = (a > b) ? a : b;
Console.WriteLine($"Max value: {max}");
// Nested ternary operator
int c = 15;
int min = (a < b) ? ((a < c) ? a : c) : ((b < c) ? b : c);
Console.WriteLine($"Min value: {min}");
}
}
Output:
Max value: 10
Min value: 5
Table 6: Ternary Operator
Name | Description | Example |
---|---|---|
Ternary | A conditional operator that takes three operands and returns one of two values based on the condition. | Condition? trueValue: falseValue |
True Condition | The Condition is true and returns a true value. | int a = 10; int b = 5; int max = (a > b) ? a : b; |
False Condition | The Condition is false and returns a false value. | int a = 10; int b = 5; int min = (a < b) ? a : b; |
07. Bitwise and Bit Shift Operators
Bitwise and bit shift operators are used to perform operations on the individual bits of an operand. The available bitwise and bit shift operators in C# are & (bitwise AND), | (bitwise OR), ^ (bitwise XOR), << (left shift), and >> (right shift), respectively.
Example 7: Bitwise and Bit Shift Operator
using System;
class Program
{
static void Main()
{
int a = 5; // binary 0000 0101
int b = 10; // binary 0000 1010
// Bitwise AND operator
int bitwiseAnd = a & b; // binary 0000 0000 = 0
Console.WriteLine($"Bitwise AND: {bitwiseAnd}");
// Bitwise OR operator
int bitwiseOr = a | b; // binary 0000 1111 = 15
Console.WriteLine($"Bitwise OR: {bitwiseOr}");
// Bitwise XOR operator
int bitwiseXor = a ^ b; // binary 0000 1111 = 15
Console.WriteLine($"Bitwise XOR: {bitwiseXor}");
// Bitwise complement operator
int bitwiseComplement = ~a; // binary 1111 1010 = -6
Console.WriteLine($"Bitwise Complement: {bitwiseComplement}");
// Left shift operator
int leftShift = a << 2; // binary 0001 0100 = 20
Console.WriteLine($"Left Shift: {leftShift}");
// Right shift operator
int rightShift = b >> 2; // binary 0000 0010 = 2
Console.WriteLine($"Right Shift: {rightShift}");
}
}
Output:
Bitwise AND: 0
Bitwise OR: 15
Bitwise XOR: 15
Bitwise Complement: -6
Left Shift: 20
Right Shift: 2
Table 7: Bitwise and Bit Shift Operators
Name | Description | Example |
---|---|---|
& (Bitwise AND) | Compares each bit of the first operand to the corresponding bit of the second operand and returns 1 if both bits are 1; otherwise, 0. | int result = x & y; |
| (Bitwise OR) | Compares each bit of the first operand to the corresponding bit of the second operand and returns 1 if either bit is 1; otherwise, 0. | int result = x | y; |
^ (Bitwise XOR) | Compares each bit of the first operand to the corresponding bit of the second operand and returns 1 if the bits are different; otherwise, 0. | int result = x ^ y; |
<< (Left shift) | Shifts the bits of the first operand to the left by the number of positions specified by the second operand. | int result = x << y; |
>> (Right shift) | Shifts the bits of the first operand to the right by the number of positions specified by the second operand. | int result = x >> y; |
08. Compound Assignment Operators
Compound assignment operators combine a binary operation with an assignment operation. The available compound assignment operators in C# are +=, -=, *=, /=, %=, &=, |=, ^=, <<=, and >>= respectively.
Example 8: Compound Assignment Operator
using System;
class Program
{
static void Main()
{
int a = 5;
// Addition assignment operator
a += 2; // equivalent to a = a + 2
Console.WriteLine($"Addition: {a}");
// Subtraction assignment operator
a -= 3; // equivalent to a = a - 3
Console.WriteLine($"Subtraction: {a}");
// Multiplication assignment operator
a *= 4; // equivalent to a = a * 4
Console.WriteLine($"Multiplication: {a}");
// Division assignment operator
a /= 2; // equivalent to a = a / 2
Console.WriteLine($"Division: {a}");
// Modulus assignment operator
a %= 3; // equivalent to a = a % 3
Console.WriteLine($"Modulus: {a}");
// Bitwise AND assignment operator
a &= 1; // equivalent to a = a & 1
Console.WriteLine($"Bitwise AND: {a}");
// Bitwise OR assignment operator
a |= 2; // equivalent to a = a | 2
Console.WriteLine($"Bitwise OR: {a}");
// Bitwise XOR assignment operator
a ^= 3; // equivalent to a = a ^ 3
Console.WriteLine($"Bitwise XOR: {a}");
// Left shift assignment operator
a <<= 2; // equivalent to a = a << 2
Console.WriteLine($"Left Shift: {a}");
// Right shift assignment operator
a >>= 1; // equivalent to a = a >> 1
Console.WriteLine($"Right Shift: {a}");
}
}
Output:
Addition: 7
Subtraction: 4
Multiplication: 16
Division: 8
Modulus: 2
Bitwise AND: 0
Bitwise OR: 2
Bitwise XOR: 1
Left Shift: 4
Right Shift: 2
Table 8: Compound Assignment Operators
Name | Description | Example |
---|---|---|
+= (Addition assignment) | Adds the value on the right to the variable on the left and assigns the result to the variable on the left. | x += y; is equivalent to x = x + y; |
-= (Subtraction assignment) | Subtracts the value on the right from the variable on the left and assigns the result to the variable on the left. | x -= y; is equivalent to x = x – y; |
*= (Multiplication assignment) | Multiplies the variable on the left by the value on the right and assigns the result to the variable on the left. | x *= y; is equivalent to x = x * y; |
/= (Division assignment) | Divides the variable on the left by the value on the right and assigns the result to the variable on the left. | x /= y; is equivalent to x = x / y; |
%= (Modulus assignment) | Divides the variable on the left by the value on the right, returns the remainder, and assigns the result to the variable on the left. | x %= y; is equivalent to x = x % y; |
&= (Bitwise AND assignment) | Applies a bitwise AND operation to the variable on the left and the value on the right and assigns the result to the variable on the left. | x &= y; is equivalent to x = x & y; |
|= (Bitwise OR assignment) | Applies a bitwise OR operation to the variable on the left and the value on the right and assigns the result to the variable on the left. | x |= y; is equivalent to x = x | y; |
^= (Bitwise XOR assignment) | Applies a bitwise XOR operation to the variable on the left and the value on the right and assigns the result to the variable on the left. | x ^= y; is equivalent to x = x ^ y; |
<<= (Left shift) | Shifts the bits of the left operand to the left by the number of positions specified by the right operand. | a <<= b is equivalent to a = a << b |
= (Right shift) | Shifts the bits of the left operand to the right by the number of positions specified by the right operand. | a >>= b is equivalent to a = a >> b |
Conclusion
In conclusion, operators are a fundamental part of programming in C#, and understanding how to use them correctly is essential. This article has covered the various categories of operators in C# programming language. From basic assignment to complex compound assignment operators, we have discussed each category in detail, including arithmetic, relational, logical, unary, ternary, and bitwise operators.
Additionally, we have provided code examples for each category to illustrate their working principles. By mastering these operators, you can significantly improve the efficiency and effectiveness of your C# code.
References: MSDN-C# Operators
FAQs
Q: What are C# operators?
C# operators are special symbols and keywords used to perform operations on variables and values. They can be used to perform mathematical calculations, compare values, and assign values to variables, among other things.
Q: How many types of operators are there in C#?
There are several types of operators in C#, including assignment, arithmetic, relational, logical, unary, ternary, bitwise, and compound assignment operators.
Q: What is the basic assignment operator in C#?
The basic assignment operator in C# is the equals sign (=). It is used to assign a value to a variable.
Q: What are arithmetic operators in C#?
Arithmetic operators in C# are used to perform mathematical calculations, such as addition (+), subtraction (-), multiplication (*), division (/), and modulus (%).
Q: What are relational operators in C#?
Relational operators in C# are used to compare values, such as less than (<), greater than (>), less than or equal to (<=), greater than or equal to (>=), equal to (==), and not equal to (!=).
Q: Q: What are logical operators in C#?
Logical operators in C# are used to combine and manipulate Boolean values, such as AND (&&), OR (||), and NOT (!).
Q: What is the ternary operator in C#?
The ternary operator in C# is a shorthand way of writing an if-else statement. It takes three operands and returns one value based on a condition.
Articles you might also like:
- Abstract Factory Design Pattern in C#: Real-World Example and Code Explanations
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- WCF vs Web API: Top 10 Differences Between WCF and Web API
- C# dispose vs finalize
- C# Abstract class Vs Interface
- C# Partial Class And Partial Methods With Examples
- C# Monitor class in multithreading
- C# Struct vs Class
- C# Dictionary with Examples
- C# Polymorphism
- C# Goto Statement – Understanding Goto in C# with Examples
- C# System.IO Classes: An Overview of the System.IO Namespace
- C# stack vs heap
- Readonly vs const in C#
- Fields vs Properties In C#
- URL vs URI: The Ultimate Guide to Understanding the difference between URL and URI
- Understanding the Difference between == and Equals() Method in C#
Don’t keep this post to yourself, share it with your friends and let us know what you think in the comments section.
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024