The main difference between local and global variables is that local variables can be accessed only within the function or block in which they are defined, whereas global variables can be accessed globally throughout the entire program.
In this article, we will try to understand the fundamental concepts of local and global variables, their use cases, Pros and Cons, and how to use them effectively in our code.
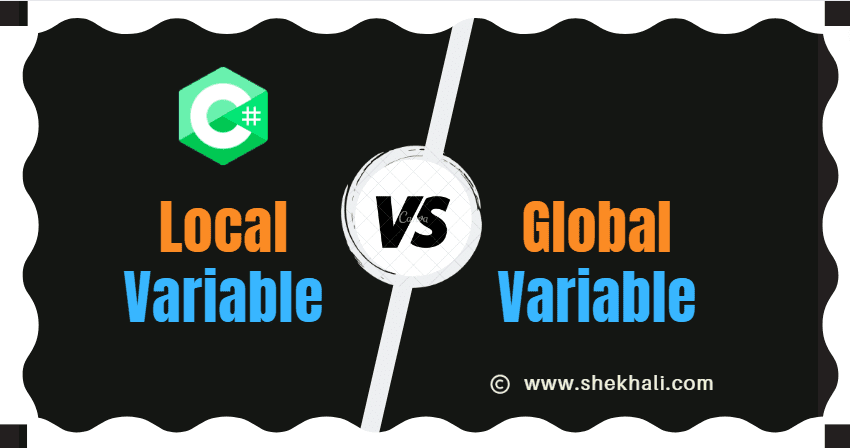
Table of Contents
- 1 What is Local Variable?
- 2 What is Global Variable?
- 3 Difference between Local and Global Variables:
- 4 Comparison between local and global variables in C#
- 5 Advantages and Disadvantages of Local and Global variables
- 6 FAQs
- 6.1 Q: What are the disadvantages of using local variables in C#?
- 6.2 Q: What are the advantages of using local variables in C#?
- 6.3 Q: What are the advantages of using global variables in C#?
- 6.4 Q: What are the disadvantages of using global variables in C#?
- 6.5 Q: What is the difference between local and global variables in terms of scope?
- 6.6 Related
What is Local Variable?
A local variable is a variable that is declared within a method or a block of code. These local variables are only accessible within the scope of the function/method or block where they are defined. Local variables are created on the stack and are automatically destroyed when the function or block is executed.
Example of a local variable in C#:
public int SampleMethod()
{
int num = 10;
return num;
}
In the above example, the variable num
is a local variable that is only accessible within the SampleMethod()
function.
What is Global Variable?
A global variable, on the other hand, is a variable that is accessible from anywhere in the code. Global variables are declared outside of any functions or blocks and can be accessed and modified by any part of the code. Global variables are stored in the heap and remain in memory until the application terminates.
Example of a global variable in C#:
int num;
public int SampleMethod()
{
num = 20;
return num;
}
In the above example, the variable num
is a global variable that we can access and modify by any part of the code.
Difference between Local and Global Variables:
- Accessibility: The Local variables are only accessible within the scope of the function or code block where they are defined, whereas global variables are accessible from anywhere in the code.
- Memory allocation: The Local variables are stored on the stack and are automatically destroyed when the function or block returns, whereas global variables are stored in the heap memory and remain in memory until the application terminates.
- Performance: The Local variables can access faster compared to global variables as they are stored on the stack memory, whereas global variables get stored on the heap which is slower compared to the stack.
- Security: Sometimes using global variables can make it easier for malicious code to access and modify sensitive information, whereas local variables are only accessible within a limited scope which makes it more difficult for malicious code to access and modify sensitive information.
Comparison between local and global variables in C#
The following is the comparison table about the difference between Local variables and Global variables in C#.
Local Variables | Global Variables |
---|---|
Local variables are declared within a function or code block. | Global variables, on the other hand, are declared outside of any functions or blocks. |
Only accessible within the scope of the function/method or block. | Accessible from anywhere in the code. |
Local variables are stored on the stack memory. | whereas, global variables are stored on the heap memory. |
Automatically destroyed when the function or block gets executed. | Remain in memory until the application terminates. |
Faster access time. | Slower access time compared to the stack. |
Local variables are more secure as they are only accessible within a limited scope. | Global variables can make it easier for malicious code to access and modify sensitive information as they can access globally throughout the entire program. |
The alterations made to a local variable will have no impact on the rest of the functions in the program. | However, any modifications made to a global variable within a function will be reflected throughout the entire program. |
Advantages and Disadvantages of Local and Global variables
We have discussed the Difference Between Local and Global Variable with examples, now let’s discuss their advantages and disadvantages:
Advantages of Local Variables:
- Faster access time: Local variables are stored on the stack memory, which has a faster access time compared to the heap.
- Better security: Local variables are only accessible within a limited scope, making it more difficult for malicious code to access and modify sensitive information.
- Improved code readability: Using local variables makes it easier to understand the scope of variables in your code, which can make your code more readable and maintainable.
Disadvantages of Local Variables:
- Limited scope: The Local variables are only accessible within the scope of the function/method or code block where they are defined, So, it will be more difficult for us to share data between different functions.
- Need for re-declaration: If you need to use the same variable in multiple functions, you will need to re-declare the variable in each function, which can make your code more verbose.
Advantages of Global Variables:
- Accessibility from anywhere in the code: We can access and modify the Global variables from any part of the code. It allows us to share data between multiple functions.
- Persistent memory: The Global variables remain in the heap memory until the application terminates, making it possible to store data that needs to persist across multiple function calls.
Disadvantages of Global Variables:
- Slower access time: Global variables are stored in the heap, which has a slower access time compared to the stack.
- Security risk: Using global variables can make it easier for malicious code to access and modify sensitive information, as they are accessible from anywhere in the code.
- Increased complexity: The use of global variables can add an extra layer of complexity to your code, which can make your code more complex and harder to maintain.
FAQs
Q: What are the disadvantages of using local variables in C#?
The Local variables have limited scope and need to be re-declared if used in multiple functions.
Q: What are the advantages of using local variables in C#?
The Local variables have faster access time, better security, and improved code readability.
Q: What are the advantages of using global variables in C#?
The Global variables can be accessed from anywhere in the code and have persistent memory.
Q: What are the disadvantages of using global variables in C#?
The Global variables have slower access time compared to local variables. It also increased security risk, and code complexity.
Q: What is the difference between local and global variables in terms of scope?
The Local variables are only accessible within the scope of the function or block where they are declared, while global variables are accessible from anywhere in the code.
References: StackOverFlow-Local vs Global Variables
You might also like:
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- 10 Difference between interface and abstract class In C#
- C# Queue with examples
- Web API vs web service: Top 10+ Differences between web API and web service in C#
- C# Polymorphism: Different types of polymorphism in C# with examples
- C# Stack
- Understanding the Difference between == and Equals() Method in C#
- Difference between SortedList and SortedDictionary in C#
- C# Hashtable vs Dictionary vs HashSet
- C# stack vs heap
- Difference between readonly and const keywords in C#
Please share this post and let us know what you think in the comments.
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024