The Fibonacci series is a sequence of numbers starting with 0 and 1, and each subsequent number is the sum of the previous two numbers. It is a popular mathematical concept that has many real-world applications.
This blog post will explore the Fibonacci sequence and its implementation in C# programming language.
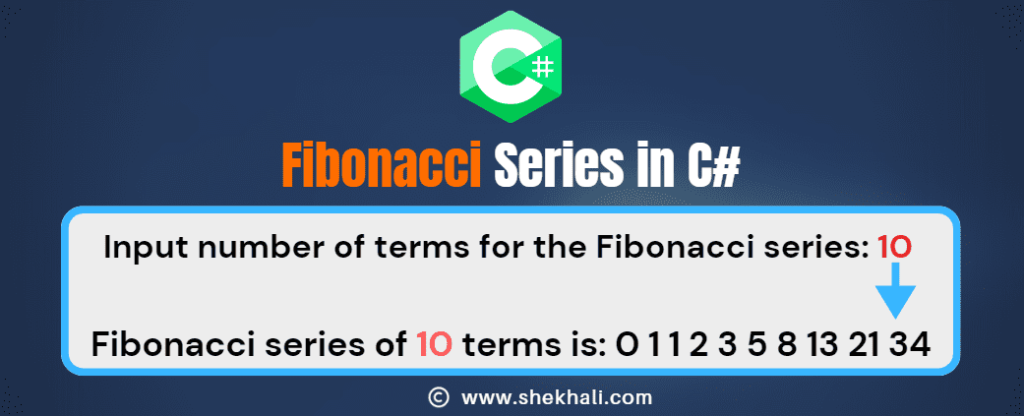
Table of Contents
What is Fibonacci Series in C#?
The Fibonacci series is a sequence in which the next number is the sum of the previous two numbers.
This pattern continues, resulting in a sequence that starts with 0 and 1, followed by 1, 2, 3, 5, 8, 13, 21, and so on. Therefore, the first two numbers in the Fibonacci series are always 0 and 1, and the subsequent numbers are obtained by adding the previous two numbers in the sequence.
What are the different ways to implement Fibonacci in C#?
Two popular ways to implement the Fibonacci series in C# are loops or recursion.
Loop-based implementation is the most common approach. It involves using a for or a while loop to iterate through the sequence and calculates each number in the series.
01. Print Fibonacci series using For Loop
Here is an example of the loop-based implementation of Fibonacci in C#:
using System;
class FibonacciSeries
{
static void Main()
{
int numberOfElements = 10;
int currentElement = 0;
int nextElement = 1;
Console.Write(currentElement + " " + nextElement + " ");
for (int i = 2; i < numberOfElements; i++)
{
int sum = currentElement + nextElement;
Console.Write(sum + " ");
currentElement = nextElement;
nextElement = sum;
}
Console.ReadLine();
}
}
Output:
0 1 1 2 3 5 8 13 21 34
Code Explanation:
In this program, we use numberOfElements
to represent the total number of elements we want to print in the fibonacci series. We use currentElement
and nextElement
to represent the current and next elements in the series, respectively.
We then use a for loop to iterate over the remaining elements in the series and calculate each element by adding the previous two elements. We print each element as we calculate it using the Console.Write()
method. Finally, we update the values of the currentElement
and nextElement
for the next iteration of the loop.
02. Fibonacci Series with Recursive Method in C#
using System;
class FibonacciSeries
{
static void Main()
{
Console.Write(" Input number of terms for the Fibonacci series : ");
int numberOfElements = Convert.ToInt32(Console.ReadLine());
Console.Write($"\n The Fibonacci series of {numberOfElements} terms is : ");
for (int i = 0; i < numberOfElements; i++)
{
Console.Write(Fibonacci(i) + " ");
}
Console.ReadKey();
}
static int Fibonacci(int n)
{
if (n <= 1)
{
return n;
}
return Fibonacci(n - 1) + Fibonacci(n - 2);
}
}
Output:
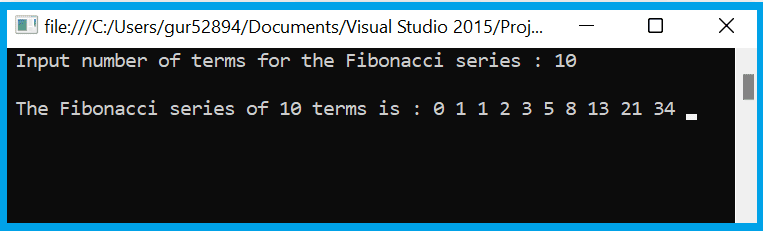
In this program, we first define the number of elements we want to print in the Fibonacci series, which is 10 in this case.
We then use a for loop to iterate over the elements in the series and call the Fibonacci method to calculate each element. The Fibonacci method is implemented using recursion, which means it calls itself to calculate the previous two numbers in the series until it reaches the base case of n <= 1
.
How to Print the Fibonacci Series up to a given number in C#?
To print the Fibonacci series up to a given number in C# using the loop-based approach, we can iterate through the sequence and print each number until we reach the desired value.
Here is an example:
using System;
class FibonacciSeries
{
static void Main()
{
Console.Write(" Prints the Fibonacci sequence up to : ");
int numberOfElements = Convert.ToInt32(Console.ReadLine());
// Prints the Fibonacci sequence up to 100
PrintFibonacci(numberOfElements);
Console.ReadKey();
}
public static void PrintFibonacci(int n)
{
int previous = 0;
int current = 1;
while (current <= n)
{
Console.Write(current + " ");
int next = previous + current;
previous = current;
current = next;
}
}
}
Output:
// Prints the Fibonacci sequence up to : 100
1 1 2 3 5 8 13 21 34 55 89
History of the Fibonacci sequence
The Fibonacci sequence is named after Leonardo Fibonacci, an Italian mathematician who lived in the 12th century. He introduced the sequence to the Western world in his book “Liber Abaci” which he wrote in 1202. The sequence was initially used to model the growth of rabbit populations, but it has since found many other applications in various fields, including computer science, finance, and biology.
Conclusion:
The Fibonacci sequence is a fascinating mathematical concept that has many real-world applications.
This article explored the Fibonacci sequence and its implementation in C# programming language. We discussed the different ways to implement the sequence using loops or recursion, and we showed how to find the nth Fibonacci number and print the sequence up to a given number.Â
Finally, we discussed the history of the Fibonacci sequence and its relevance in today’s world. With this knowledge, you can now apply the Fibonacci sequence in your projects.
FAQs
Q: What is the Fibonacci sequence?
The Fibonacci sequence is a series of numbers where each number is the sum of the previous two numbers. The sequence starts with 0 and 1, and the next number is the sum of the previous two numbers (0+1=1, 1+1=2, 1+2=3, 2+3=5, and so on).
Q: What are the different ways to implement the Fibonacci sequence in C#?
Two common ways to implement the Fibonacci sequence in C# are iteration and recursion. The iterative approach uses a loop to generate the sequence, while the recursive approach involves calling a function that recursively generates the sequence.
Articles you might also like:
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- Design Patterns
- Builder Design Pattern: A Comprehensive Guide with C# Code Examples
- SOLID Design Principles in C#: A Complete Example
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- Abstract Factory Design Pattern in C#
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024