In this article, we’ll learn how to reverse an array in C# with multiple examples and code explanations.
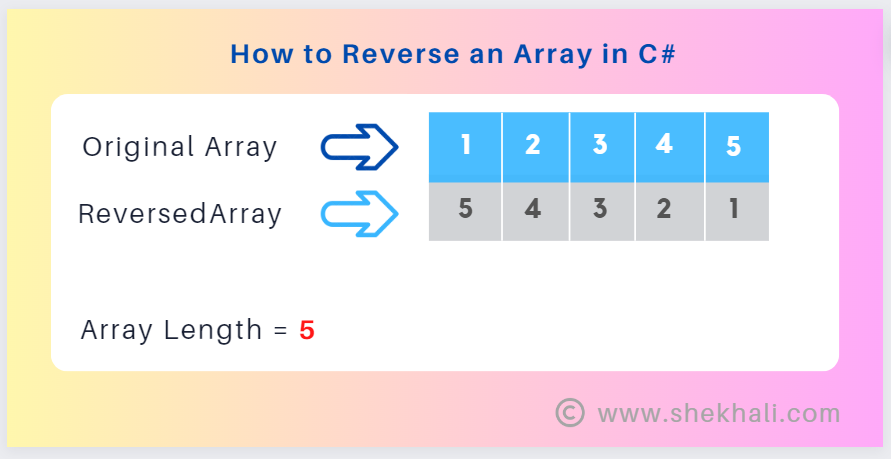
Table of Contents
Introduction
Reversing an array might seem like a simple task, but it’s a fundamental operation in programming. Mastering array reversal is essential if you’re dealing with data structures and algorithms or want to impress your fellow developers at the next code review.
The Problem
Given an array, how do we reverse its elements? Imagine you have an array like this:
int[] originalArray = { 1, 2, 3, 4, 5 };
Your goal is to transform it into:
int[] reversedArray = { 5, 4, 3, 2, 1 };
Method 1: Using the Array.Reverse()
Method
In this example, we use the built-in Array.Reverse()
method to reverse the elements of the array.
// Code Example 1: Using Array.Reverse() Method
using System;
class Program
{
static void Main()
{
// Original array
int[] originalArray = { 1, 2, 3, 4, 5 };
// Display original array
Console.WriteLine("Original Array: " + string.Join(", ", originalArray));
// Reverse the array using Array.Reverse() method
Array.Reverse(originalArray);
// Display reversed array
Console.WriteLine("Reversed Array: " + string.Join(", ", originalArray));
}
}
Output:
Original Array: 1, 2, 3, 4, 5
Reversed Array: 5, 4, 3, 2, 1
Method 2: Reverse an Array in C# using Temporary Array
In this example, we manually reverse the array by creating a temporary array and iterating through the original array in reverse order.
// Code Example 2: Manual Reversal with a Temporary Array
using System;
class Program
{
static void Main()
{
// Original array
int[] originalArray = { 1, 2, 3, 4, 5 };
// Display original array
Console.WriteLine("Original Array: " + string.Join(", ", originalArray));
// Create a temporary array to store reversed elements
int[] reversedArray = new int[originalArray.Length];
// Perform manual reversal
for (int i = 0, j = originalArray.Length - 1; i < originalArray.Length; i++, j--)
{
reversedArray[i] = originalArray[j];
}
// Display reversed array
Console.WriteLine("Reversed Array: " + string.Join(", ", reversedArray));
}
}
Output:
Original Array: 1, 2, 3, 4, 5
Reversed Array: 5, 4, 3, 2, 1
Method 3: Using LINQ to Reverse an Array in C#
This example showcases how to use LINQ (Language Integrated Query) to reverse an array effortlessly. In the LINQ, we can use the Reverse()
method, combined with ToArray()
, to reverse an array.
// Code Example 3: Using LINQ to Reverse an Array
using System;
using System.Linq;
class Program
{
static void Main()
{
// Original array
int[] originalArray = { 1, 2, 3, 4, 5 };
// Display original array
Console.WriteLine("Original Array: " + string.Join(", ", originalArray));
// Use LINQ to reverse the array
int[] reversedArray = originalArray.Reverse().ToArray();
// Display reversed array
Console.WriteLine("Reversed Array: " + string.Join(", ", reversedArray));
}
}
Output:
Original Array: 1, 2, 3, 4, 5
Reversed Array: 5, 4, 3, 2, 1
Method 4: The Swapping Technique To Reverse an Array in C#
Our approach involves swapping elements within the array. Let’s break it down:
The Swapping Technique
- Swapping Elements: We’ll swap pairs of elements to reverse an array. For example, if we have elements 3 and 4, we’ll replace 3 with 4 and vice versa. Here’s how it works:
- Create a temporary variable to store the value at the first index (let’s call it temp).
- Replace the value at the second index with the value at the first index.
- Finally, replace the value at the first index with temp.
- Iterative Swapping: We’ll apply this swapping technique iteratively. Starting from both ends of the array, we’ll move toward the center until the start index is less than the end index.
The Algorithm
Here’s the step-by-step algorithm to reverse an array:
- Initialize two variables: startIndex (set to 0) and endIndex (set to
n-1
, wheren
is the array length). - Swap the values at
startIndex
andendIndex
. - Increment
startIndex
by 1 and decrementendIndex
by 1. - Repeat steps 2 and 3 until the
startIndex
is less than theendIndex
. - Great! You’ve reversed the array.
Let’s Code!
Enough theory; let’s dive into some C# code. Below is a sample program that reverses an array using the swapping technique:
using System;
namespace ReverseAnArrayExample
{
public class Program
{
static void Main(string[] args)
{
int[] arr = { 1, 2, 3, 4, 5 };
Console.Write("Original Array: ");
for (int i = 0; i < arr.Length; i++)
{
Console.Write(arr[i] + " ");
}
ReverseArrayElements(arr, 0, arr.Length - 1);
Console.Write("\nReversed Array: ");
for (int i = 0; i < arr.Length; i++)
{
Console.Write(arr[i] + " ");
}
Console.ReadKey();
}
public static void ReverseArrayElements(int[] arr, int startIndex, int endIndex)
{
while (startIndex < endIndex)
{
int temp = arr[startIndex];
arr[startIndex] = arr[endIndex];
arr[endIndex] = temp;
startIndex++;
endIndex--;
}
}
}
}
Output:
Original Array: 1 2 3 4 5
Reversed Array: 5 4 3 2 1
Conclusion:
Reversing an array in C# is a common operation, and understanding different methods for achieving this task is crucial for every developer, whether you prefer simply the “Array.Reverse()
” method, the manual approach with a temporary array, or the use of LINQ to reverse an array.
Recommended Articles:
- Fibonacci sequence: Fibonacci series in C# (with examples)
- Reverse a number and string in C#
- How to remove duplicate characters from a String in C#
- Palindrome program in C# with examples
- C# Program to Check if a Given Number is Even or Odd
- C# Program to Swap Two Numbers (With Multiple Examples)
- C# program to count the occurrences of each character in a String
- C# program to count vowels and consonants in a string
- How to remove special characters from a string in C#
- Program to copy all elements of an array into another array
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- C# Programs asked in Interviews
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025