C# params are useful when you declare a method and don’t know how many arguments will be passed as parameters.
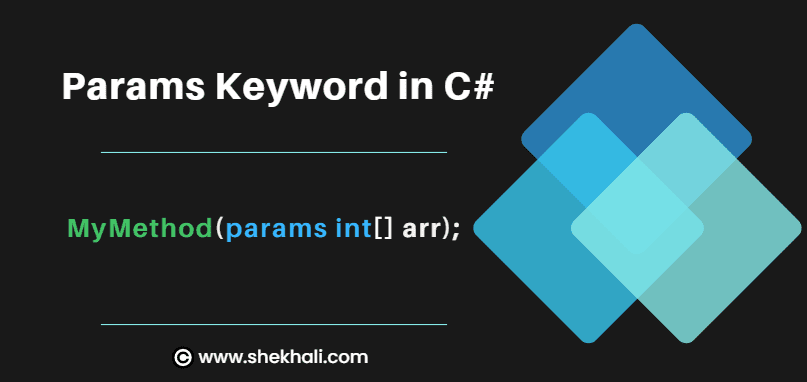
Table of Contents
What are params in C#?
The params in C# allows a method to accept a variable number of arguments. Think of it as a flexible way to pass multiple values to a function without specifying the count. Here’s how it works:
- When you define a method parameter with params, it acts as an array of objects.
- You can pass any number of arguments of any type to the method.
Syntax of params keyword:
The params
keyword in C# is used in the method parameters to allow for a variable number of arguments. Here’s the syntax:
public void ExampleMethod(params dataType[] parameterName)
{
// Method body
}
dataType
: Specifies the data type of the parameters.parameterName
: Represents the name of the parameter array.
Params example with int
data type:
public void PrintNumbers(params int[] numbers)
{
foreach (int num in numbers)
{
Console.WriteLine(num);
}
}
In this example, the PrintNumbers
method can be called with any number of integer arguments, and the params keyword allows flexibility in passing a variable number of parameters.
Example 1: C# Params with Integer
The following code example demonstrates the params keyword in C# to specify method parameters that accept multiple integer arguments.
Here, we have created a method called AddNumbers
with the params parameter and called it with an array of numbers. This method sums all the numbers passed as parameters and returns the total sum as the result.
// C# program to demonstrate the params as a method parameter in C#
using System;
namespace Params_Keyword_Example
{
class Program
{
// Params Parameter
public static int AddNumbers(params int[] arr)
{
int sum = 0;
foreach (int num in arr)
{
sum += num;
}
return sum;
}
public static void Main(string[] args)
{
int total = AddNumbers(10, 20, 30, 40);
Console.WriteLine($" Sum: {total}");
Console.ReadLine();
// Output:
// Sum: 100
}
}
}
Important Points About Params Keyword in C# :
- The Params keyword is useful when the developer doesn’t know the number of parameters to use in advance.
- The params parameter type must be declared as an array. Example:
MyMethod(params int[] arr);
- Only one params keyword is allowed to be declared as a method parameter.
- The length of params will become zero if no arguments are passed.
- A params parameter must be the last argument in the method’s parameter list. Example:
MyMethod( int a, int b, params int[] arr)
- Params cannot have a default value assigned. Example:
params int[] arr= 10;
- The params parameter accepts a comma-separated list of arguments of the type of the array elements.
- Params keyword can’t be used with the combination of ref and out.
Example 2: C# Params Keyword With Object Type
The following C# code example will show you how to pass different values to a method using params of object type.
class Program
{
// Params Parameter
public static void Print(params object[] arr)
{
foreach (var item in arr)
{
Console.WriteLine($" {item} ,");
}
}
public static void Main(string[] args)
{
Console.WriteLine($"*** Print params elements ***");
Print("INDIA","USA","FRANCE", 10, 20.5);
Console.ReadLine();
}
}
Output:
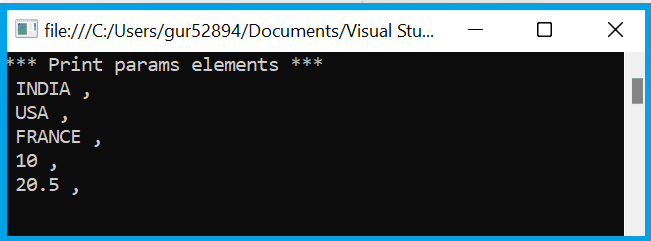
Can you give a good example of the params keyword?
A good example of using params is the String.Format()
method. This method allows users to pass in a string formatted to their requirements and the number of parameters for the values to insert into the String.
You can imagine that if the params keyword did not exist, then the String.Format()
would require an infinite number of overload methods.

String.Format
method.Difference between params and arrays in C#
Here is a tabular comparison between params
and arrays in C#:
Feature | params | Arrays |
---|---|---|
Purpose: | Params allows passing a variable number of arguments. | Array represents a fixed-size collection of elements. |
Declaration: | The params keyword is used in method parameters.public void PrintNames(params string[] names) { ... } | Arrays are declared using square brackets [] . |
Number of Elements: | Params accepts a variable number of parameters. | Array requires a predefined number of elements when declared. |
Usage Restrictions: | The params must be the last parameter in the parameter list. | It can be used anywhere in the parameter list. |
Example: | void ExampleMethod(params int[] numbers) | int[] numbers = { 1, 2, 3, 4, 5 }; |
Method Invocation: | Allows calling the method with a variable number of arguments. | Requires passing an array of specified size. |
Initialization: | Array can be used without explicitly creating an array. | Requires explicit creation and initialization of an array. |
Type: | The params keyword can be used with any data type. | Supports arrays of any data type. |
Common Use Cases: | Params can be useful when the number of arguments may vary. | Array is suitable for situations with a fixed and known number of elements. |
Compile-time Restrictions: | Params in C# allows zero or more arguments to be passed. | Array requires a fixed number of elements to be defined at compile time. |
FAQs:
Q: What is the purpose of the params keyword?
The params keyword in C# allows a method to accept a variable number of arguments.Â
When you define a parameter with params, it acts as an array of objects, enabling you to pass any number of arguments of any type to the method.
Q: Can we define a params type as multi-dimensional array?
No, The params type must always be a single-dimensional array.
Q: Is it possible to have null params in C#?
Yes, the array associated with params can be null.
Q: What is params in C#?
In C#, params is a keyword that is used to specify a parameter that takes variable number of arguments. It can be used when you don’t know the number of arguments in advance to be passed in a function.
Q: Can I use params with any data type?
Yes! The params keyword isn’t limited to specific data types. You can use it with integers, strings, custom objects, or any other type. It provides flexibility in handling various argument lists.
References: MSDN-params (C# Reference)
Conclusion
In this article, we discussed the params keyword with multiple examples.
The params keyword simplifies method signatures, making them more readable and flexible.
Whether dealing with integers, strings, or custom objects, params empower you to handle variable argument lists easily in a method.
I hope you enjoyed and found this post about the params keyword useful. If you have any questions or comments, please leave them in the comments section below.
Related articles:
- C# Array Vs List
- ref and out keyword
- C# Dictionary with Examples
- C# List Class With Examples
- C# Abstract class Vs Interface
- C# Struct vs Class
- C# Hashtable vs Dictionary
- C# Nullable types: How do you work with nullable types in C#?
- C# Tutorial
- C# Programs asked in Interviews
- C# Collections-(Stack, Queue, Array, dictionary, Hashtable, ArrayList, SortedList)
- Constructors in C# with Examples
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025