In this article, we will learn about the C# list with the help of multiple examples.
List<T>
is a dynamic collection that contains multiple objects of the same data type that can be accessed using an index.
It is similar to an array but can grow or shrink in size. Lists are commonly used to store and organize data in a sequential manner. It can dynamically perform operations like adding, searching, shorting, and removing elements from a collection.
The following diagram illustrates the methods and properties of the List<T>
class.
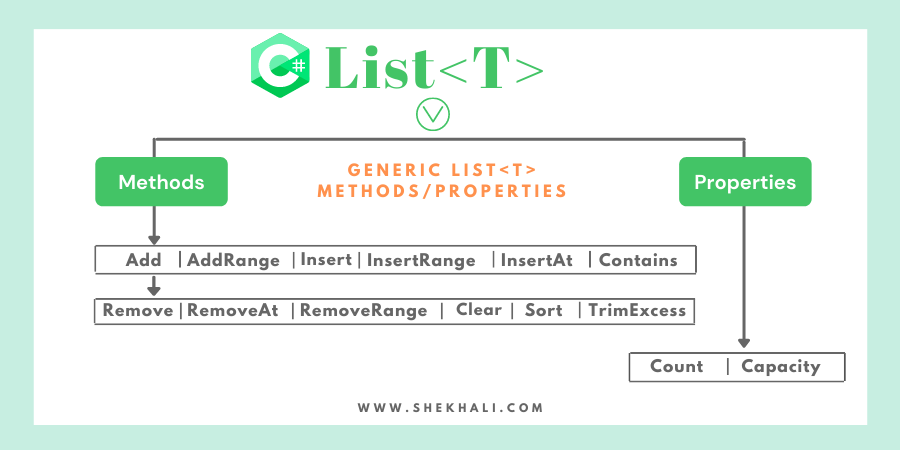
Table of Contents
- 1 Syntax
- 2 Creating a List in C#:
- 3 Adding Elements to a C# List:
- 4 Accessing Elements in a List:
- 5 Removing Elements from a List:
- 6 Iterating through a List:
- 7 List Count and Capacity:
- 8 Sorting and Searching in a List:
- 9 Insert and InsertRange method in C# List:
- 10 Characteristics of the List in C#:
- 11 FAQs
- 12 Conclusion:
Syntax
The following is the syntax to declare a generic List in C#. The parameter T
in the list represents the type of item, which can be int
, long
, string
, or any user-defined object.
List<T> myList = new List<T>();
Creating a List in C#:
To use a List in C#, you need to import the System.Collections.Generic
namespace. Here is how you can create a List:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// List of integers is created.
List<int> numbers = new List<int>();
}
}
Adding Elements to a C# List:
Lists provide a straightforward way to add elements using the Add
method.
Here is an example:
numbers.Add(10); // Adding element 10 to the list
numbers.Add(20); // Adding element 20 to the list
numbers.Add(30); // Adding element 30 to the list
Accessing Elements in a List:
You can use the indexing notation to retrieve an element from a List. The index starts from 0
for the first element. Here is an example:
int firstNumber = numbers[0]; // Accessing the first element (10)
int secondNumber = numbers[1]; // Accessing the second element (20)
int thirdNumber = numbers[2]; // Accessing the third element (30)
Removing Elements from a List:
Lists offer various methods to remove elements based on different conditions. One common method is Remove, which removes the first occurrence of a specified element. Here is an example:
numbers.Remove(30); // Removing the element 30 from the list
Iterating through a List:
You can easily iterate through the elements of a List using a foreach loop. Here is an example:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<int> numbers = new List<int>();
numbers.Add(10); // Adding element 10 to the list
numbers.Add(20); // Adding element 20 to the list
// Iterating through the numbers List.
foreach (int number in numbers)
{
Console.WriteLine(number);
}
}
}
Output:
10
20
List Count and Capacity:
Lists have a Count
property that returns the number of elements in the list. Additionally, they have a Capacity
property that indicates the total number of elements the list can hold before resizing occurs.
Console.WriteLine($"Count: {numbers.Count}"); // Output: 2 (after removing third element)
Console.WriteLine($"Capacity: {numbers.Capacity}"); // Output: 4 (default initial capacity)
Sorting and Searching in a List:
Lists provide methods like Sort
and BinarySearch to sort and search for elements. Sorting arranges the elements in ascending or descending order while searching helps find specific elements efficiently. Here’s an example:
numbers.Sort(); // Sorting the list in ascending order
int index = numbers.BinarySearch(20); // Searching for the element 20, It will return index 1
Insert and InsertRange method in C# List:
The following is an example of inserting items into the list using the Insert
and InsertRange methods.
using System;
using System.Collections.Generic;
namespace ListInCSharp
{
class Program
{
static void Main(string[] args)
{
// Creating and initializing a list in C#
List<String> fruitsNameList = new List<String>() { "Apple", "Avocado" };
// inserting elements into list using List method
fruitsNameList.Insert(0, "Banana");
fruitsNameList.Insert(3, "Grapes");
List<String> fruitsName = new List<String>() { "Mango", "Orange" };
// Inserting fruitsName into fruitsNameList at position 1
// InsertRange method
fruitsNameList.InsertRange(1, fruitsName);
Console.WriteLine("****** Print list elements ******");
foreach (string item in fruitsNameList)
{
Console.WriteLine($" {item} ");
}
Console.ReadLine();
}
}
}
Output:
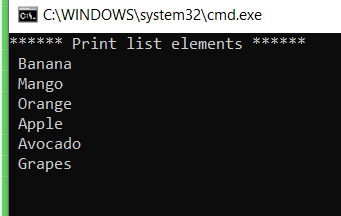
Characteristics of the List in C#:
- Dynamic Size: The List class in C# allows the size of the collection to grow or shrink as needed.
- Sequential Order: The List maintains the order of elements as they are added, allowing easy access and retrieval based on their positions.
- Element Manipulation:Â Lists provide methods to add, remove, and modify elements, making it easy to update the collection as required.
- Index-based Access: Elements in a List can be accessed using their index position, starting from 0 for the first element. You can retrieve or modify elements at specific positions using the index notation.
- Generic Type:Â The List is generic class available in
System.Collections.Generic
namespace. It can hold elements of any specific type, such as integers, strings, or custom objects. - Iteration Support:Â Lists support iteration using
foreach
loops, enabling easy traversal and processing of all elements. - Sorting and Searching: The List class offers methods to sort elements in ascending or descending order and search for specific elements efficiently.
- Performance Considerations: While Lists offer flexibility, performance can be impacted when frequently adding or removing elements from the middle. Alternative data structures like LinkedList or Queue might be more efficient for such scenarios.
- Count Property: Lists have a Count property that returns the number of elements currently in the collection.
- Built-in Methods:Â The List class provides useful methods to perform common operations like adding elements, removing elements, sorting, searching, and more. These methods simplify data manipulation tasks.
- Maximum capacity: For very large List objects on a 64-bit system, you can increase the maximum capacity to 2 billion elements by enabling a configuration setting in the run-time environment.
Reference MSDN: List<T> Class
FAQs
Q: What is a List in C#?
A List in C# is a dynamic collection of elements that can grow or shrink in size. It allows you to store and organize data in a sequential manner.
Q: How is a List different from an array?
While arrays have a fixed size, Lists can change their size dynamically. Lists also provide additional methods for easy element manipulation, such as adding, removing, and searching.
Q: How do I create a List in C#?
To create a List, you need to import the System.Collections.Generic
namespace and declare a variable of type List, specifying the data type it will hold.
For example:Â List<int> numbers = new List<int>();
Q: How can I access elements in a C# List?
Elements in a List can be accessed using index notation. The index starts from 0. For example, int firstNumber = numbers[0];
retrieves the first element.
Conclusion:
In conclusion, the List class in C# is a powerful and flexible data structure that allows you to store and manage collections of elements efficiently.
With Lists, you can dynamically add or remove elements, access them using indices, and perform common operations like sorting and searching. Lists are versatile and widely used in programming to handle sequential data effectively.
By learning and practicing the concepts covered in this article, developers can enhance their programming skills and leverage the capabilities of Lists in their projects.
In this article, We learned how to add, insert, delete, find, search, sort, and reverse items in the generic List.
Recommended Articles:
- Readonly vs const in C#
- C# Operators with [Examples]
- Fields vs Properties In C#
- URL vs URI: The Ultimate Guide to Understanding the difference between URL and URI
- Understanding the Difference between == and Equals() Method in C#
- 10 Difference between interface and abstract class In C#
- C# Struct vs Class
- Exception Handling in C#| Use of try, catch, and finally block
- C# Enum
- Converting Celsius to Fahrenheit in C#: A Comprehensive Guide
- Difference Between if-else and switch: A Side-by-Side Comparison of If-Else and Switch Case
- Local vs Global Variables
- C# stack vs heap
- C# extension methods with examples
- Properties In C# with examples
- IEnumerable Interface in C# with examples
- Constructors in C# with Examples
- C# Dictionary
- C# Goto Statement – Understanding Goto in C# with Examples
- C# System.IO Classes: An Overview of the System.IO Namespace
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024