Here is an article that explains the difference between ref and out keywords in C# with multiple code examples.
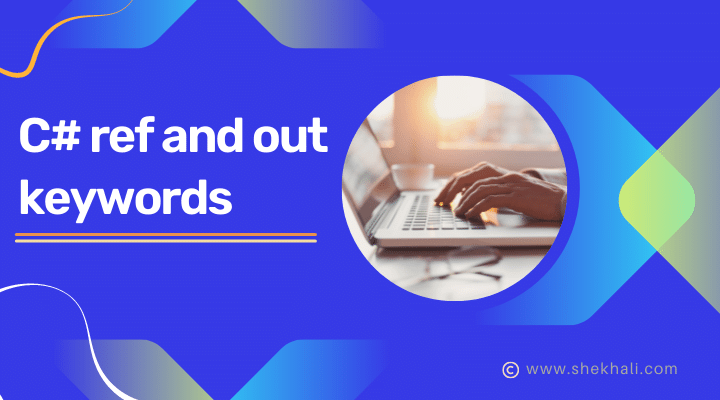
In C#, ref and out are two keywords used to pass arguments to methods by reference. Although they are similar in many ways, there are some key differences between them.
Let’s explore the differences between ref and out keywords with multiple code examples.
Table of Contents
- 1 Differences between ref and out keywords
- 2 What is the ref keyword?
- 3 What is the out keyword?
- 4 C# ref vs out
- 5 Ref and out keywords in method overloading
- 6 Summary:
- 7 FAQs
- 7.1 Q: Is it possible to use a property of a class as ref or out parameters?
- 7.2 Q: How do you use ref and out parameters?
- 7.3 Q: Are functions ref type in C#?
- 7.4 Q: Can you use the ref keyword with a value type?
- 7.5 Q: Can you use the out keyword with a reference type?
- 7.6 Q: Can we return multiple values from a function using the ref keyword?
- 7.7 Recommended Articles
- 7.8 Related
Differences between ref and out keywords
Here are some key differences between ref and out keywords:
- The ref keyword is used to pass arguments to methods by reference. The out keyword is used to pass arguments to methods by reference when the method returns multiple values.
- The main difference between ref and out keyword is that ref requires the variable to be initialized before being passed to the method, whereas out does not.
- When you pass an argument by ref, any changes made to the argument inside the method are reflected in the original variable when the method returns. When you pass an argument by out, the method can modify the argument and return a value through it.
- It is necessary to initialize the value of a parameter before returning to the calling method when using the out keyword. It is not necessary to initialize the value of a parameter before returning to the calling method when using the ref keyword.
What is the ref keyword?
The ref keyword is used to pass arguments to methods by reference. When you pass an argument by reference, any changes made to the argument inside the method will be reflected in the original variable when the method returns.
The variable must be initialized before being passed with ref
to the method.
Example: Ref keyword
Here is an example code snippet that demonstrates the use of the ref keyword:
using System;
namespace Ref
{
class program
{
public static void Main()
{
// Initializing a variable 'number' with the value 10
int number = 10;
Console.WriteLine($"Previous value of the number = {number}");
//Passing int number as ref parameter in the method
PassByReference(ref number);
Console.WriteLine($"Current value of the number = {number}");
Console.ReadLine();
}
public static void PassByReference(ref int number)
{
// Incrementing the value of the referenced parameter by 1
number ++;
}
}
}
Output:
Previous value of the number = 10
Current value of the number = 11
Code Explanation:
In the above example, we have defined a method calledÂ
 that takes an integer argument by reference using the PassByReference
ref
 keyword. Inside the method, we increment the value of the argument by one. When we call the method with the ref
 keyword, the original variable is passed to the method by reference.
Therefore, any changes made to the argument inside the method are reflected in the original variable when the method returns.
Note: In C#, the ref keyword returns the address of the variable rather than the value.
What is the out keyword?
The out keyword is also used to pass arguments to methods by reference. However, it is generally used when a method returns multiple values. When you pass an argument by out, the method can modify the argument and return a value through it.
Important Points about out keyword:
- When using out, the variable being passed does not need to be initialized before being passed to the method.
- It also allows two-way communication between the method and the calling code, similar to ref.
- However, the method must assign a new value to the parameter inside the method before it returns. The variable passed in as out must be assigned a value inside the method, or it will result in a compilation error.
Example: Out keyword in C#
using System;
class OutKeywordExample
{
// A method that initializes two out parameters
public static void GetValues(out int first, out int second)
{
// Assigning values to the out parameters
first = 10;
second = 20;
}
// Main entry point of the program
public static void Main()
{
int value1, value2;
// Calling the method GetValues and passing variables as out parameters
GetValues(out value1, out value2);
// Displaying the values of the out parameters
Console.WriteLine($"Value1: {value1}"); // Output: 10
Console.WriteLine($"Value2: {value2}"); // Output: 20
}
}
Output:
Value1: 10
Value2: 20
Example 2: Passing multiple out parameters to a method.
The following is a simple example of using out parameters to return multiple values ​​of different data types.
using System;
namespace OutKeyword
{
class program
{
public static void Main()
{
// Calling method
int id; // Initialization is optional in case of out parameter
string name;
OutMethod(out id, out name);
Console.WriteLine($"Id : {id} , Name = {name}");
Console.ReadKey();
}
public static void OutMethod(out int id, out string name)
{
//Called method
//Return multiple values using out parameters
id = 52894;
name = "Shekh Ali";
}
}
}
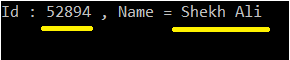
It is not possible to pass a property as a
ref
orout
parameter, because properties are implemented as methods, not variables.
In C#, a method typically returns only one data type at a time. However, by using the out
parameters, a method can return multiple values of different data types simultaneously.
C# ref vs out
Here is a comparison of the ref
and out
keywords in C#:
Comparison | ref keyword | out keyword |
---|---|---|
Definition: | The ref keyword allows passing a reference to a variable, enabling the called method to modify its value, and the changes are reflected in the calling code. | The out keyword enables passing variables to a method without requiring initialization, and the method assigns values to these variables later on. It allows multiple values to be returned from the method. |
Initialization: | The caller must initialize the value of the ref parameter before calling the function. Example: int number = 10; SomeMethod(ref number); | The caller does not need to initialize the value of the out parameter before calling the function. Example: int number; SomeMethod(out number); |
Value types: | ref can be used with value types. | out can only be used with value types. |
Reference types: | ref can be used with reference types. | out cannot be used with reference types. |
Modification: | The function can both read and modify the value of the parameter, and the changes will be reflected in the calling code. | The function can modify the value of the parameter, and the changes will be reflected in the calling code |
Use in a function definition: | ref must be used in the function definition. | It also must be used in the function definition. |
Use in a function call: | ref must be used when calling a function. | out must not be used when calling the function. |
Direction: | When the ref keyword is used, data can flow in bi-directions. | The out keyword is used to get data in a one-way mode. |
Return value: | Usually, the ref keyword is not used to return multiple values from a method. | The out keyword is useful when a function needs to return multiple values. |
Constraint: | No constraint. The ref parameter may or may not be re-initialized by the called method. | he called method must initialize the parameter passed as the out keyword. |
Declaration: | Use ref when you know the value at the time of declaration. | Use out when you don’t know the value at the time of declaration. |
When you pass a value type as a reference using ref
or out
, only a reference to the value is passed, so changes made to the value in the called function affect the original value in the calling code.
However, when you pass a value type by value, the actual value is passed, so changes made in the called function won’t affect the original value in the calling code.
Ref and out keywords in method overloading
In C#, you cannot use the ref
and out
keywords at the same time when overloading methods. This means you cannot have one version of a method that takes a ref
parameter and another version that takes an out
parameter.

Method overloading is possible in C# when one version of the method takes a ref
argument and another version takes a normal argument. In this case, the method can be called with either a ref
argument or a normal argument, depending on the needs of the calling code.
The following example is perfectly valid for the method overloading.
using System;
namespace MethodOverLoading
{
class program
{
public static void MyMethod(int a)
{
a = 10;
}
public static void MyMethod(out int b)
{
//method signature must be different.
b = 10;
}
}
}
Summary:
In C#, the ref
and out
keywords are used to pass arguments as a reference to a method. If you use ref
or out
, any changes made to the argument inside the method will affect the original value outside the method.
When you know the value before calling the method, use ref
. But when you don’t know the value before calling the method, use out
.
FAQs
Here are some common questions about the ref
and out
keywords in C#:
Q: Is it possible to use a property of a class as ref or out parameters?
No, it is not possible to use ref
or out
with properties directly. These keywords are designed for variables, and properties are accessed through getter and setter methods. However, you can use methods within the class to achieve similar functionality by accepting ref
or out
parameters.
Q: How do you use ref and out parameters?
Both the out and ref keywords are used to pass arguments to a method as a reference type, but the out keyword is generally used when a method has to return multiple values, whereas the ref keyword is typically used when an existing variable is to be modified in a method.
Q: Are functions ref type in C#?
By default, the value type variable is passed by value, while the reference type variable is passed by reference from one method to another method in C#.
The keywords ref and out in C# allow us to pass value type variables to another method by the reference.
Q: Can you use the ref
keyword with a value type?
Yes, you can use the ref
keyword with value types such as integers, float, long, structures, etc.
Q: Can you use the out
keyword with a reference type?
No, the out
keyword can only be used with value types, not reference types.
Q: Can we return multiple values from a function using the ref keyword?
Yes, we can use the ref
keyword to return multiple values from a function. However, it is more common to use the out
keyword for this purpose, as the out
keyword is specifically designed for returning multiple values through its parameters.
Related post: Value type and Reference type in C#
I hope this post was helpful. If you have any questions, please feel free to leave them in the comments section below.
Recommended Articles
- 10 Difference between interface and abstract class In C#
- C# Array vs List: When should you use an array or a List?
- C# Stack Class With Push And Pop Examples
- C# Static class
- C# Hashtable
- Top 50 C# Interview Questions And Answers
- ASP.NET Core Middleware With Examples
- WCF vs Web API
- IEnumerable Interface in C# with examples
- Constructors in C# with Examples
- C# Enum | How To Play With Enum in C#?
- Exception Handling in C#| Use of try, catch, and finally block
- Generic Delegates in C# With Examples
- C# Dictionary with Examples
- Multithreading in C#
- C# Tutorial
- C# Programs asked in interviews
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024