In this blog post, we will take a deep dive into C# hashtables, explaining what they are, how they work, and how to use them effectively in our C# code.
We will also cover some common scenarios where hashtables can be particularly useful, as well as some tips and best practices for working with them. By the end of this post, you should have a solid understanding of hashtables and how to leverage their power in your C# projects.
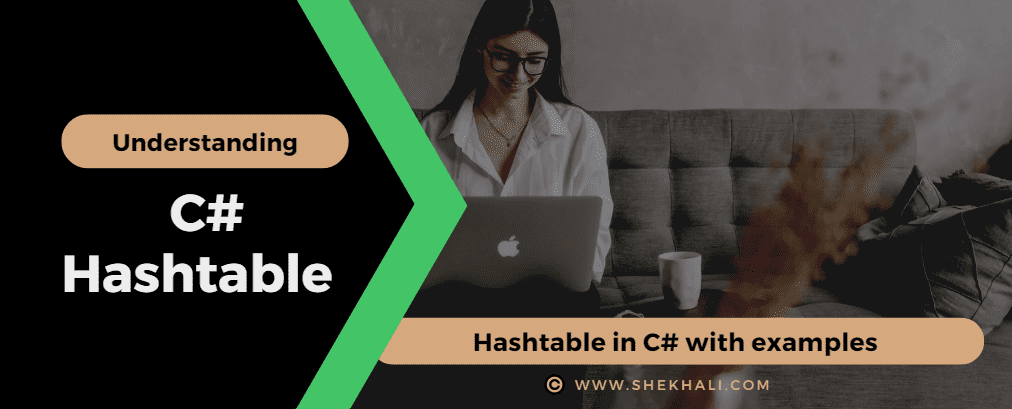
Table of Contents
Introduction to C# Hashtable
A C# hashtable is a non-generic data structure that allows you to store and retrieve data efficiently, based on key-value pairs. The key is used to access the elements in the hashtable collection.
It works by using a hash function to map keys to specific locations in a table, allowing you to quickly find and access the data you need. Hashtable is commonly used in C# projects because of its fast performance and flexibility.
Common Use Cases for C# Hashtable
Here are some of the situations where C# hashtables can be particularly helpful:
- Storing and retrieving large amounts of data quickly: Hashtables are very efficient at storing and retrieving data, making them a good choice for situations where you need to work with large amounts of data.
- Implementing a dictionary or lookup table: Hashtables are often used to implement dictionaries or lookup tables, where you need to quickly find a value based on a key.
- Caching data: C# Hashtable is a useful collection for storing and quickly retrieving data that you use often, without having to go to a slower source like a database.
Creating a Hashtable In C#
To create a C# hashtable, you can use the Hashtable
class from the System.Collections
namespace. Here is an example of how to create a hashtable and add some key-value pairs to it:
Example:
Following is an example of how to create a C# Hashtable and add elements to it.
using System;
using System.Collections;
namespace HashTableExample
{
class Program
{
static void Main(string[] args)
{
// Creating a new hashtable
Hashtable hashtable = new Hashtable();
// Adding some key-value pairs to the hashtable
hashtable.Add("key1", "Apple");
hashtable.Add("key2", "Banana");
hashtable.Add("key3", "Mango");
hashtable.Add("key4", "Orange");
// Getting the number of key-value pairs in the hashtable
int count = hashtable.Count;
Console.WriteLine($"Number of key-value pairs: {count}");
// Check if the hashtable is fixed size
bool isFixedSize = hashtable.IsFixedSize;
Console.WriteLine($"Is fixed size: {isFixedSize}");
// Check if the hashtable is read-only
bool isReadOnly = hashtable.IsReadOnly;
Console.WriteLine($"Is read-only: {isReadOnly}");
// Getting the keys from the hashtable
ICollection keys = hashtable.Keys;
Console.WriteLine("*** Keys ***");
foreach (var key in keys)
{
Console.WriteLine(key);
}
// Getting the values from the hashtable
ICollection values = hashtable.Values;
Console.WriteLine("*** Values ***");
foreach (var value in values)
{
Console.WriteLine(value);
}
// Check if the hashtable contains a specific key
bool containsKey = hashtable.ContainsKey("key4");
Console.WriteLine($"Contains key 'key4': {containsKey}");
// Check if the hashtable contains a specific value
bool containsValue = hashtable.ContainsValue("value2");
Console.WriteLine($"Contains value 'Mango': {containsValue}");
// foreach loop to Iterate over the key-value pairs in the hashtable
Console.WriteLine("Key-value pairs:");
foreach (DictionaryEntry entry in hashtable)
{
string key = (string)entry.Key;
string value = (string)entry.Value;
Console.WriteLine($"Key: {key}, Value: {value}");
}
// Remove a key-value pair from the hashtable
hashtable.Remove("key2");
// Clear all the records from the hashtable
hashtable.Clear();
Console.ReadKey();
}
}
}
In the above code, we have created a new hashtable and added four key-value pairs to it. Then we retrieve the number of key-value pairs in the hashtable, check if the hashtable is fixed size or read-only, and retrieve the keys and values in the hashtable.
We also checked if the hashtable contains a specific key or value, and iterate over the key-value
pairs in the hashtable using foreach
loop. Finally, we removed a key-value pair and then clear all the records from the hashtable.
Once we run the above code, we will get the following output:
Number of key-value pairs: 4
Is fixed size: False
Is read-only: False
*** Keys ***
key2
key3
key1
key4
*** Values ***
Banana
Mango
Apple
Orange
Contains key 'key4': True
Contains value 'Mango': False
Key-value pairs:
Key: key2, Value: Banana
Key: key3, Value: Mango
Key: key1, Value: Apple
Key: key4, Value: Orange
C# Hashtable Properties
Following are the properties of the System.Collections.Hashtable
class in C#, along with a description of each property:
Property | Description |
---|---|
Count : | Gets the number of elements contained in the Hashtable . |
IsFixedSize : | Gets a value indicating whether the Hashtable has a fixed size. |
IsReadOnly : | Gets a value indicating whether the Hashtable is read-only. |
IsSynchronized : | Gets a value indicating whether access to the Hashtable is synchronized (thread safe). |
Item : | Gets or sets the value associated with the specified key. |
Keys : | Gets an ICollection containing the keys in the Hashtable . |
Values : | Gets an ICollection containing the values in the Hashtable . |
SyncRoot : | Gets an object that can be used to synchronize access to the Hashtable . |
Example:
Following is an example of how you can use these properties of Hashtable collection:
// Creating a new Hashtable.
Hashtable ht = new Hashtable();
// Add some key/value pairs to the hashtable
ht.Add("Key1", "Value1");
ht.Add("Key2", "Value2");
ht.Add("Key3", "Value3");
// Check the number of elements in the hashtable
int count = ht.Count;
// Check if the hashtable is read-only
bool isReadOnly = ht.IsReadOnly;
// Check if the hashtable is synchronized
bool isSynchronized = ht.IsSynchronized;
// Get the value associated with the key "Key3"
object value = ht["Key3"];
// Set the value associated with the key "Key2" to "NewValue"
ht["Key2"] = "NewValue";
// Get the keys in the hashtable
ICollection keys = ht.Keys;
// Get the values in the hashtable
ICollection values = ht.Values;
C# Hashtable Methods
Following are the methods of the System.Collections.Hashtable
class in C#, along with a description of each method:
Method | Description |
---|---|
Add: | Adds an element with the specified key and value to the Hashtable . |
Clear: | Removes all elements from the Hashtable . |
Contains: | This method determines whether the Hashtable contains a specific key. |
ContainsKey: | It determines whether the Hashtable contains a specific key. |
ContainsValue: | Determines whether the Hashtable contains a specific value. |
CopyTo: | Copies the Hashtable elements to an array, starting at a particular array index. |
GetEnumerator: | It returns an IDictionaryEnumerator that iterates through the Hashtable . |
Remove: | It removes the element with the specified key from the Hashtable . |
Example:
Here is an example of how we can use these methods:
using System;
using System.Collections;
namespace HashTableExample
{
class Program
{
static void Main(string[] args)
{
// Creating new Hashtable.
Hashtable hashtable = new Hashtable();
// Add some key/value pairs to the hashtable
hashtable.Add("Key1", "Value1");
hashtable.Add("Key2", "Value2");
hashtable.Add("Key3", "Value3");
// Check if the hashtable contains the key "Key1"
bool containsKey = hashtable.ContainsKey("Key1");
// Check if the hashtable contains the key "Key3"
bool containsKey3 = hashtable.Contains("Key3");
// Check if the hashtable contains the value "Value2"
bool containsValue = hashtable.ContainsValue("Value2");
// foreach loop to Iterate through the hashtable
foreach (DictionaryEntry entry in hashtable)
{
Console.WriteLine("Key: {0}, Value: {1}", entry.Key, entry.Value);
}
// Remove the element with the key "Key1" from the hashtable
hashtable.Remove("Key1");
// Clear all elements from the hashtable
hashtable.Clear();
Console.ReadKey();
}
}
}
Remove elements from the Hashtable
- We can use the
Remove()
method to remove a specific key-value pair from a C# Hashtable, However, if the key you specify doesn’t exist in the Hashtable, this method will throw a KeyNotfoundException. To avoid this, you can use theContainsKey()
method to check if the key exists before calling Remove(). - If we want to remove all the key-value pairs from a C# Hashtable at once, we can use the
Clear()
method.
// Creating new Hashtable.
Hashtable fruits = new Hashtable();
// Add some key/value pairs to the hashtable
fruits.Add("Key1", "Mango");
fruits.Add("Key2", "Apple");
fruits.Add("Key3", "Banana");
fruits.Remove("Key2"); // removes Key2 data
//fruits.Remove("Key4"); //throws runtime exception: KeyNotFoundException
if (fruits.ContainsKey("key4"))
{ // check key before removing it from the hashtable.
fruits.Remove("key4");
}
fruits.Clear(); //Removes all elements from the collection
Following are some advantages and disadvantages of using the Hashtable
class in C#:
Advantages of Hashtable:
- Fast lookup: Hashtables provide fast lookup for key/value pairs, with average-case O(1) time complexity for most operations.
- Thread-safe: The
Hashtable
class is thread-safe, so we can use it in multithreaded applications without the need for external synchronization. - Fast data access: It allows you to quickly find and access the data even when the amount of data is huge.
Disadvantages of Hashtable:
- Unordered keys: The keys in a Hashtable are not stored in a particular order, so you cannot rely on the order in which they are enumerated.
- Non-generic: The
Hashtable
class is not a generic collection, so you need to cast the keys and values to the appropriate data types when accessing them. - Slower insertion and deletion: Inserting and deleting elements from a
Hashtable
can be slower than other data structures, with average-case O(1) time complexity.
In general, Hashtable is a good choice when you need fast lookups and thread safety, but if you need faster insertion and deletion, you may need to consider using a different data structure such as Dictionary.
References: MSDN-Hashtable class
FAQ: C# Hashtable
Here are some frequently asked questions (FAQs) about the System.Collections.Hashtable
class in C#:
Q: What is a hashtable in C#?
A: A hashtable is a non-generic collection that stores key/value pairs and provides fast lookups for those pairs.
Q: How you can add elements to a hashtable in C#?
A: you can use the Add
method to add elements to a Hashtable
in C#. For example:Hashtable ht = new Hashtable();
ht.Add("Key1", "Value001");
Q: How can you remove elements from a hashtable in C#?
A: you can use the Remove
method to remove or delete an element from a Hashtable
in C#.ht.Remove("Key1");
Q: What is the time complexity of operations on a hashtable in C#?
A: The time complexity of operations on a Hashtable
in C# is generally O(1) for most operations, including lookup, insertion, and deletion. However, in the worst case, the time complexity can be O(n), where n is the number of elements in the Hashtable
.
Here are some recommended articles on collections:
- C# Queue with examples
- C# Stack
- C# Dictionary
- Collections in C#
- Array vs List
- C# List with examples
- C# Arrays
If you enjoyed this post, please share it with your friends or leave a comment below.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025