A static class is a class that cannot be instantiated and contains only static data members. It is a collection of utility or helper methods that can be called directly by the class name itself without creating an instance of the class.
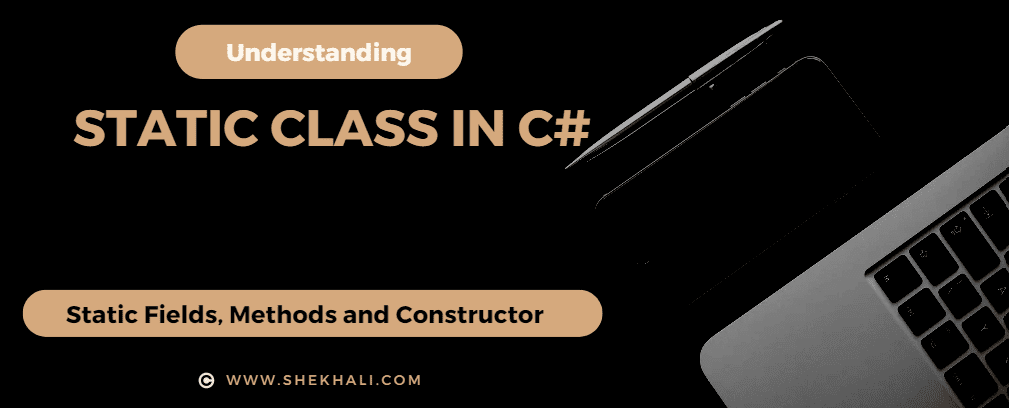
Table of Contents
What are the advantages and disadvantages of static class in C#?
Here are the advantages and disadvantages of static classes in C#:
Advantages:
- Static classes are sealed and cannot be inherited, which can help prevent bugs and unintended behavior caused by inheritance.
- They can provide a convenient way to group related methods and variables without needing to instantiate an object.
- Since they don’t require instantiation, they can be accessed quickly and easily, resulting in better performance.
- We can use static methods in various contexts, such as helper classes or utility functions.
Disadvantages:
- We can’t use static classes to implement interfaces, which can limit their flexibility in specific scenarios.
- Since we can access static classes from anywhere in the code, it can be harder to maintain encapsulation and ensure that the class is used correctly.
- They can make it more challenging to write testable code since static methods can’t be easily mocked or replaced with test doubles.
- Because they are designed to be global, they can be prone to conflicts with other global resources, such as other static classes or shared memory.
Rules for Static Class in C#
Here are some rules to keep in mind when working with static classes in C#:
- A static class in C# can only contain static members. That means all fields, methods, and nested classes within the static class must also be static.
- We cannot instantiate a static class by using the new keyword.
- A static class cannot have instance constructors. That means you cannot define a constructor for a static class that accepts arguments.
- A static class cannot inherit from any other class or implement interfaces. That is because a static class cannot have any instances and, therefore, cannot have any implementation of inherited methods.
- A static class stays in memory for the lifetime of the application domain in which the program is running.
- C# static class is by default sealed and, therefore, can’t be inherited by the child classes.
We can use a static class to contain utility methods or data shared across all instances of a particular type. However, It’s usually better to use static classes in moderation. If you need a more flexible solution, consider using other design patterns like singletons or utility classes with dependency injection.
Syntax: C# Static Class
In C#, we can create a static class by using the static keyword with the class, as shown below.
static class MyStaticClass
{
//static data members
//static methods
}
Example: C# Static Class
The following is an example of defining a static class to access the static data members and member functions without creating an instance of the static class.
using System;
namespace StaticClassExample
{
// Static class
public static class Employee
{
// Static Variables
public static string firstName;
public static string lastName;
public static string Address;
public static int Id;
// Static Method
public static void ShowFullName()
{
Console.WriteLine($" FullName: {Employee.firstName} {Employee.lastName}");
}
}
class Program
{
static void Main(string[] args)
{
// Accessing the static fields
Employee.firstName = "Shekh";
Employee.lastName = "Ali";
Employee.Address = "Delhi";
Employee.Id = 52894;
Console.WriteLine($" First Name: {Employee.firstName} , Last Name: {Employee.lastName}");
// Calling the static method
Employee.ShowFullName();
Console.WriteLine($" Address: {Employee.Address}");
Console.WriteLine($" Employee Id: {Employee.Id}");
Console.ReadLine();
}
}
}
In the above example, the Employee class is a static class that has static fields and methods. To access the Employee class members, you don’t need to create an instance of the class. Instead, you can access the members directly through the class name.
Output:
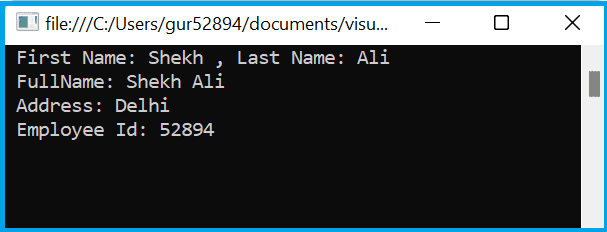
Features of a static class
- It only has static members (methods and variables).
- You can’t create an instance of the class.
- It’s sealed, which means you can’t inherit from it.
- It doesn’t have any instance constructors.
Static data member
A static data member is a class member shared across all class instances. In other words, only one copy of the static data member is shared among all instances of the class.
Here is an example of a static data member in C#:
public class Counter
{
// Static data member
public static int count = 0;
// Non-static data member
public int id;
public Counter()
{
// Increment the static data member
count++;
// Assign a unique ID to the non-static data member
id = count;
}
}
In the above code example, the Counter class has a static data member called count and a non-static data member called id. The static data member count is used to keep track of the number of Counter objects that have been created.
To access the static data member count, you don’t need to create an instance of the Counter class. You can simply access it through the class name:
// Accessing the static data member
int count = Counter.count;
On the other hand, to access the non-static data member id, you need to create an instance of the Counter class.
// Creating an instance of the Counter class
Counter counter = new Counter();
// Accessing the non-static data member
int id = counter.id;
What is a static method?
A static method is associated with the class rather than a specific class instance. That means you don’t need to create an instance of the class to call a static method. Instead, you can call the static method directly through the class name.
Here’s an example of a static method in C#:
public static class MathUtils
{
// Static method
public static int Add(int num1, int num2)
{
return num1 + num2;
}
}
In the above code example, the MathUtils
class has a static method called Add that takes two integers as arguments and returns their sum.
To call the ‘Add’ method, you don’t need to create an instance of the MathUtils
class. Instead, you can call the method directly through the class name:
// Calling the static method directly through class name.
int sum = MathUtils.Add(10, 30);
Static methods are often used to provide utility functions or to perform operations that are independent of any specific instance of a class.
C# Static Constructors
A static constructor is a special constructor used to initialize a static class or a static class member. It is called automatically by the .NET runtime when the class is first accessed. It is used to initialize static fields or run the code that needs to be executed only once.
The following is an example of a static class with a static constructor. Static Constructor in C# with examples
Static VS Non-static classes
Here is the list of some differences between static and non-static classes in C#:
- Instance creation:Â A non-static class can be instantiated using the new keyword. On the other hand, we cannot instantiate a static class.
- Member access: Members of a non-static class can be accessed through an instance of the class, while members of a static class are accessed directly through the class name.
- Inheritance: A non-static class can be inherited by another class, while we cannot inherit a static class.
- Virtual methods: Non-static classes can have virtual methods, which we can override in derived classes. Static classes cannot have virtual methods.
- Constructors: Non-static classes can have constructors, which are called when an instance of the class is created. Static classes cannot have constructors.
- Data members: Non-static classes can have static and non-static data members, while static classes can only have static data members.
When to use a static class in C#?
A static class in C# is a class that cannot be instantiated and only contains static members. It is intended to contain methods and fields independent of any particular object. A static class is created using the static keyword in the class declaration.
Here are some guidelines for when to use a static class:
- When you need a utility class that provides methods you want to call without creating an instance of the class.
- When you want to prevent the creation of instances of the class.
- When you want to represent a singleton design pattern, where you only want one instance of the class to be created.
You may want to use a static class when you need to:
- Perform math operations like finding a number’s square root or absolute value.
- Format strings or convert data types with helper methods
- Access resources used throughout the application, like a database or configuration file.
Example of Static method class:
Here are some examples of static classes in C#:
System.IO.File
:Â Provides static methods for creating, copying, deleting, moving, and opening files.System.Math
:Â Provides static methods for performing mathematical operations.System.Console
:Â Provides static methods for interacting with the console, such as reading and writing text.System.Environment
:Â Provides static methods for retrieving information about the current environment, such as the operating system and computer name.System.Diagnostics.Debug
:Â Provides static methods for debugging and tracing code, such as writing messages to the debug output window.System.Convert
:Â Provides static methods for converting between different data types, such as from a string to an integer.System.Text.RegularExpressions.Regex
:Â Provides static methods for working with regular expressions, such as matching patterns in strings.System.Threading.Tasks.Task
:Â Provides static methods for creating and managing asynchronous tasks.System.Linq.Enumerable
:Â Provides static methods for working with collections of objects, such as filtering and sorting lists.
These are just a few examples of the many static classes in the .NET Framework.
using System;
using System.IO;
using System.Text.RegularExpressions;
class Program
{
static void Main()
{
string filePath = @"C:\example.txt"; // path to the file to read
// read the file and split it into lines
string[] lines = File.ReadAllLines(filePath);
// use regular expressions to extract the numbers from each line
Regex regex = new Regex(@"\d+");
// loop through the lines and calculate the square root of each number
foreach (string line in lines)
{
MatchCollection matches = regex.Matches(line);
foreach (Match match in matches)
{
int number = Convert.ToInt32(match.Value);
double squareRoot = Math.Sqrt(number);
Console.WriteLine("The square root of {0} is {1:F2}", number, squareRoot);
}
}
Console.ReadLine();
}
}
Output:
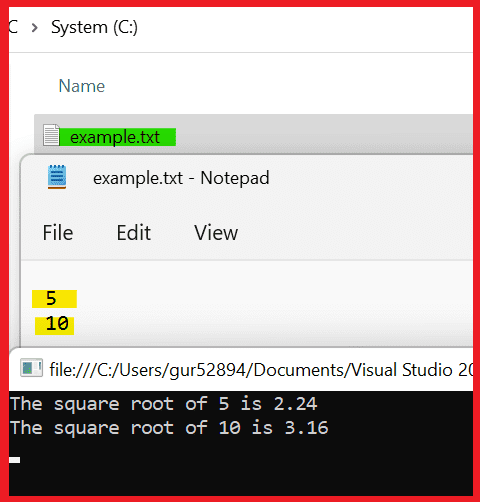
FAQ
The following are some frequently asked questions and answers about the C# static class and static data members in C#:
Q: Can we have non-static members in a static class?
No, a static class can only have static members.
Q: Can a non-static class have static data members?
Yes, a non-static class can have both static and non-static data members.
Q: How do I access a static data member in C#?
You can access a static data member by using the class name followed by the member name, like this: ClassName.MemberName
.
Q: Can you override a static method in a derived class?
No, It is impossible to override static methods in a derived class because they are only bound to the static class and not to the instance class.
Q: Why should we avoid static classes?
01. Lack of flexibility:Â Static classes cannot be inherited, so they cannot be extended or modified. That can make it more difficult to reuse code or customize the behavior of a class.
02. Difficulty in testing:Â It can be challenging to test static classes because they cannot be instantiated. That means you cannot use dependency injection to mock or stub static methods for testing.
03. Tight coupling:Â Static classes can create tight coupling between different parts of your codebase. That can make it more challenging to understand the relationships between different types and to maintain your code over time.
References: MSDN-Static class and static data members
Related articles:
- C# Static Constructor With Examples
- Difference between static and readonly
- C# Abstract class Vs Interface
- C# Array Vs List
- ref and out keyword
- C# Dictionary with Examples
- Difference between Boxing and Unboxing in C#
- C# List Class With Examples
- C# Stack Class With Push And Pop Examples
- C# Struct vs Class
- C# Goto Statement – Understanding Goto in C# with Examples
- C# System.IO Classes: An Overview of the System.IO Namespace
- Converting Celsius to Fahrenheit in C#: A Comprehensive Guide
- Difference Between if-else and switch
- Local vs Global Variables
- C# stack vs heap
- Readonly vs const in C#
Please share this post and let us know what you think in the comments.
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024