In this article, we’ll learn how to write a C# program to find the longest word in a string. We’ll break down the problem, provide multiple code examples, and explain each step.
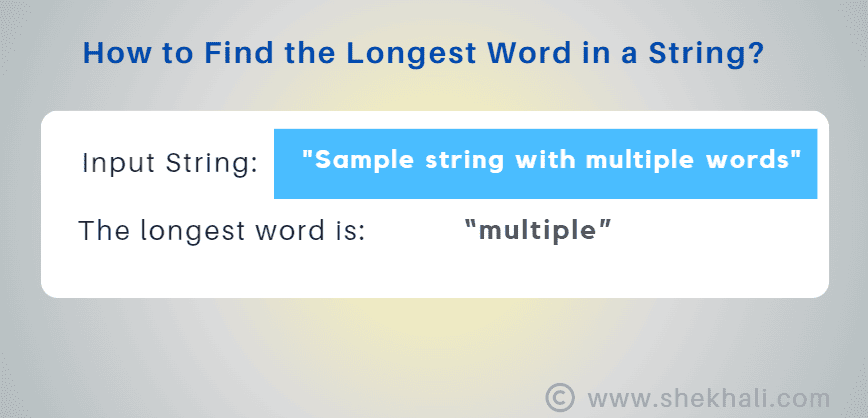
Table of Contents
Problem Statement
We want to find the longest word within an input string.
Word: A word is defined as a sequence of characters separated by spaces.
Approach
We’ll follow these steps to solve the problem:
- Split the String: We’ll split the input string into individual words based on spaces.
- Track the Longest Word: We’ll keep track of the longest word encountered so far.
- Iterate Through Words: We’ll loop through each word in the array of words.
- Compare Lengths: For each word, we’ll compare its length with the longest word found so far. If the current word is longer, we’ll update our longest word.
- Display the Result: Finally, we’ll display the longest word.
Code Example 1: Find the Longest Word in a String Using Split and Loop
using System;
public class FindLongestWord
{
public static void Main()
{
string input = "Sample string with multiple words";
// Split the string into words based on spaces
string[] words = input.Split(' ');
string longestWord = string.Empty; // Initialize an empty string to store the longest word
foreach (string word in words)
{
if (word.Length > longestWord.Length)
{
longestWord = word; // Update the longest word
}
}
Console.WriteLine($"The longest word is: {longestWord}");
}
}
Output:
The longest word is: multiple
Code Example 2: Find the Longest Word in a String Using LINQ
using System;
using System.Linq;
public class FindLongestWord
{
public static void Main()
{
string input = "Sample string with multiple words";
// Split the string into words based on spaces
string[] words = input.Split();
// Find the longest word using LINQ
string longestWord = words.OrderByDescending(w => w.Length).FirstOrDefault();
Console.WriteLine($"The longest word is: {longestWord}");
}
}
Output:
The longest word is: multiple
Code Explanation
- In both examples, we split the input string into an array of words using Split.
- We then iterate through the words, comparing their lengths to find the longest word.
- Example 1 uses a loop, while Example 2 leverages LINQ for a more straightforward solution.
Remember, understanding the problem and breaking it down step by step is crucial in software development. Happy coding!
Recommended Articles:
- Find the maximum and minimum number in an array
- How to Reverse an Array in C# with Examples
- Program to copy all elements of an array into another array
- C# Program to Convert Decimal to Binary with Examples
- C# Program to Convert Binary to Decimal with Examples
- C# Program to Check Armstrong Number
- How to remove duplicate characters from a String in C#
- C# program to count the occurrences of each character in a String
- C# Program to Print Multiplication Table of a Given Number
- Program to print prime numbers from 1 to N
- C# Program to Check if a Given Number is Even or Odd
- Program to copy all elements of an array into another array
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- C# Programs asked in Interviews
Latest posts by Shekh Ali (see all)
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024