Stack in C# represents a last-in, first-out (LIFO) collection of objects. It is useful when you need last-in, first-out access to elements. Adding an element to the stack is called a push operation, and removing an element from the stack is called a pop operation.
A Stack is a collection that can be both generic and non-generic. The generic stack is defined in the System.Collections.Generic
namespace. A non-generic stack, on the other hand, is defined under System.Collections
namespace. In this post, we will discuss a non-generic type stack.
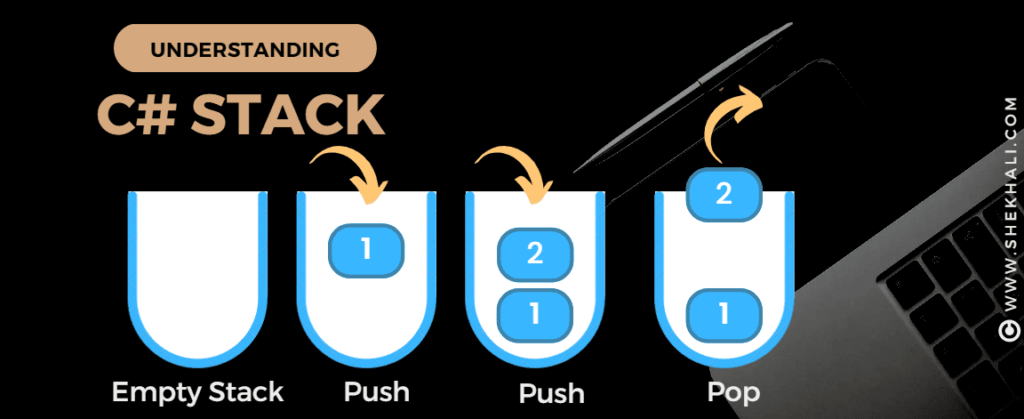
Table of Contents
C# Stack Declaration
To define a stack, you first need to declare an instance of the stack class before performing any operations like add, delete, etc.
The new
keyword is used to create an object of a Stack class as shown below.
Stack stack = new Stack();
Characteristics of Stack Class:
The following are the characteristic of the stack class.
- In C#, Stack is Last In First Out collection, the item added last will come out first.
- An item can be added to the stack using the
Push()
method. - Items can be retrieved from the stack using the
Pop()
and thePeek()
methods. - The stack
Peek()
method will always return the last top inserted element from the stack and never delete any element from the stack. - A new element is always added to the top of the stack and removed from the top of the stack.
- A stack is a collection of similar data items in which both insertion and deletion operations are performed based on the LIFO (Last in, First out) principle.
- Duplicate elements are allowed to be stored in Stack.
Null
is a valid value for reference types in a Stack.- When new elements are added to a Stack, the capacity is automatically increased through reallocation
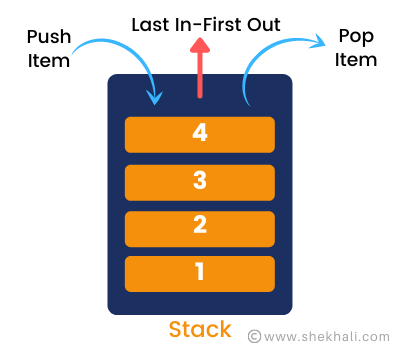
C# Stack Example
Following is an example of adding and accessing elements from the non-generic stack in c#.
using System;
using System.Collections;
namespace StackExample
{
class Program
{
public static void Main(string[] args)
{
// Creating and initializing a new Stack.
Stack stack = new Stack();
stack.Push(10);
stack.Push(20);
stack.Push(30);
// Reading elements from the stack
foreach(var item in stack)
{
Console.Write($"{item} ,");
}
Console.ReadLine();
}
// Output: 30 ,20 ,10 ,
}
}
Stack Properties & Methods
Property | Usage |
---|---|
Count | The Count property returns the total count of elements in the Stack. |
C# Stack Methods | Usage |
---|---|
Push: | The Push method is used to insert an element at the top of a stack. |
Pop: | The Pop method is used to remove and return an element from the top of the stack. |
Clear: | The Clear method is used to remove all the elements from the stack. |
Clone: | The Clone method will create a shallow copy of the stack. |
Contains: | The Contains method is used to determine whether or not an element exists in a stack. |
Peek: | The Peek method is used to return a top element from the stack without deleting it. |
Stack Pop() Method
The Pop() method returns the top element and removes it from the stack.
Following is an example to access stack elements using the Pop() method.
// Creating and initializing a new Stack.
Stack stack = new Stack();
stack.Push(10);
stack.Push(20);
stack.Push(30);
stack.Push(40);
Console.WriteLine($" Befor Pop(): Total Number of Elements in Stack: {stack.Count}");
Console.WriteLine("*** Stack Elements ***");
// Accessing Stack Elements using while loop
while (stack.Count > 0)
{
Console.WriteLine($" {stack.Pop()}");
}
Console.WriteLine($"After Pop(): Total Number of Elements in Stack: {stack.Count}");
Console.ReadLine();
Result:
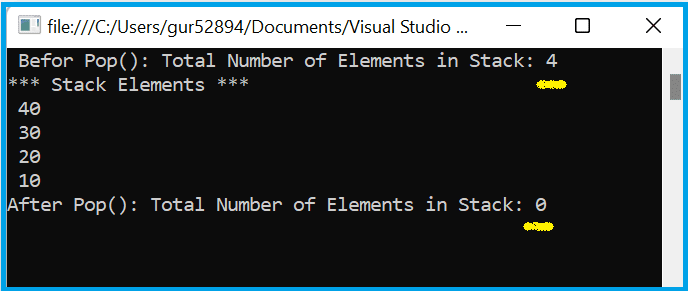
you should not use the Pop() or Peek() method on an empty stack. Otherwise, an InvalidOperation exception will be thrown.
Stack Peek() Method
The Peek() method of stack returns the lastly added element from the stack but does not remove it. It is recommended to check for elements in the stack before retrieving elements using the Peek() method. Otherwise, you will get an InvalidOperation exception.
Stack stack = new Stack();
stack.Push(10);
stack.Push(20);
stack.Push(30);
stack.Push(40);
Console.WriteLine($" Befor Peek(): Total Number of Elements in Stack: {stack.Count}");
Console.WriteLine("*** Stack Elements ***");
// Accessing Stack Elements using Peek method
if (stack.Count > 0)
{
foreach (var element in stack)
{
Console.WriteLine($" {stack.Peek()}");
}
}
Console.WriteLine($" After Peek(): Total Number of Elements in Stack: {stack.Count}");
Console.ReadLine();
When you run the above C# program, you will get the following result.
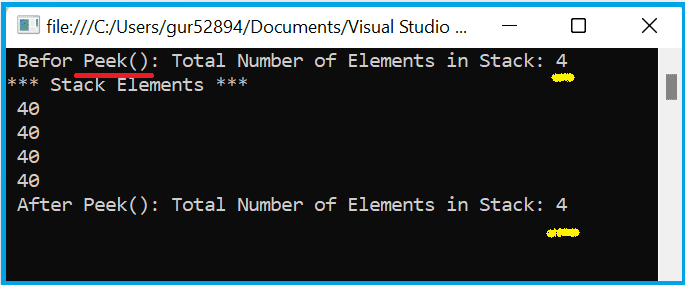
Stack Contains() Method
The Contains()
method determines whether the specified element exists in a Stack collection or not. If the element exists in the stack, It returns true Otherwise, returns false.
Stack stack = new Stack();
stack.Push(10);
stack.Push(20);
stack.Push(30);
stack.Push(40);
Console.WriteLine($"Contains Element 40: {stack.Contains(40)}");
Console.WriteLine($"Contains Element 100: {stack.Contains(50)}" );
// Output:
// Contains Element 40: True
// Contains Element 50: False
Stack Clear() Method
The Clear() method is used to remove all the elements from the Stack.
Stack stack = new Stack();
stack.Push(10);
stack.Push(20);
stack.Push(30);
stack.Push(40);
Console.WriteLine($"Total Elements before Clear() method: {stack.Count}");
stack.Clear();
Console.WriteLine($"Total Elements after Clear() method: {stack.Count}");
// Output:
// Total Elements before Clear() method: 4
// Total Elements after Clear() method: 0
Stack Count property
The Count property of the Stack class is used to return the total number of elements in a stack. The following is the code example to demonstrate this:
Stack stack = new Stack();
stack.Push("Hello");
stack.Push("World");
Console.WriteLine($"Total elements count in Stack: {stack.Count}");
Console.ReadLine();
// Output:
// Total elements count in Stack: 2
FAQ
Q: Why is stack needed in C#?
You can use stack when you need last-in, first-out access to items. Push is the process of adding an element, and Pop is the process of removing it from the stack.
Q: What is the difference between a stack and a heap?
In short, a Stack is a linear data structure and a Heap is a hierarchical data structure. Stack memory always stored blocks in LIFO (Last in, First out) order, whereas heap memory used dynamic memory allocation and deallocation.
Q: Can you explain what the term “Push” and “Pop” means in C#?
A push/pop operation is typically performed on a stack. The PUSH operation is used to add an element to a stack, and the POP operation removes the topmost element from the stack.
References: MSDN-Stack Class, geeks for geeks- C# Stack class
Related articles:
- C# Queue Class
- C# Collection
- C# Array Vs List
- C# Dictionary with Examples
- C# List Class With Examples
- C# Abstract class Vs Interface
- C# Struct vs Class
- C# Hashtable vs Dictionary
- C# Nullable types: How do you work with nullable types in C#?
- C# Tuple
- C# Dynamic Type: Var vs dynamic keywords in C#
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024