Boxing is the process of converting a value type into a reference type, and unboxing is the process of converting a reference type back into a value type.
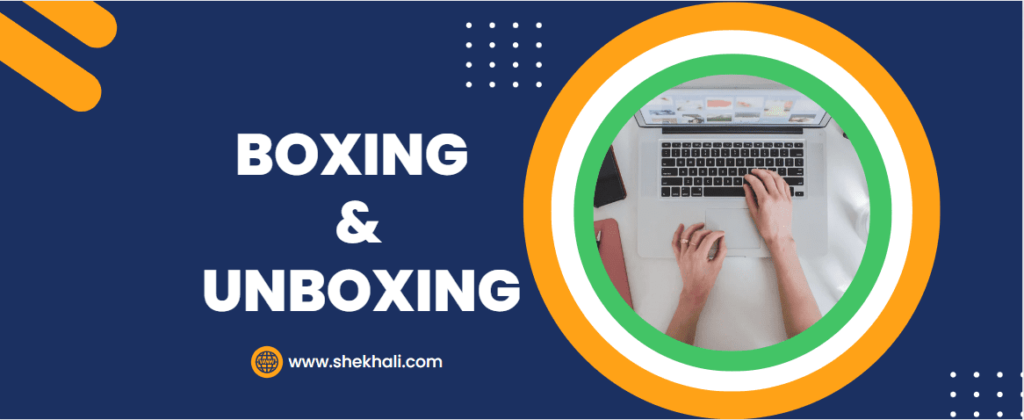
Table of Contents
- 1 What is Boxing in C#?
- 2 What is UnBoxing in C#?
- 3 Understanding boxing and unboxing in C#
- 4 FAQs:
- 4.1 Q: What is boxing in C#?
- 4.2 Q: What is unboxing in C#?
- 4.3 Q: How do generics prevent boxing?
- 4.4 Q: Why do we need boxing In C#?
- 4.5 Q: What is the difference between boxing and unboxing in C# .NET?
- 4.6 Q: What are the disadvantages of boxing in C#?
- 4.7 Q: Are there alternatives to boxing and unboxing in C#?
- 4.8 Conclusion:
- 4.9 Related
What is Boxing in C#?
Boxing in C# involves converting a value type, such as an integer or Boolean, into a reference type, like an object. When you perform boxing, you place the value inside a unique container known as an object. It allows you to treat the value type as an object.
Example of Boxing:
int myNumber = 42; // Declaring and initializing an integer value
object boxedNumber = myNumber; // Boxing the value into an object
// Print the boxed value
Console.WriteLine("Boxed Number: " + boxedNumber); // Output: 42
Code Explanation:
In the code snippet above, we first declare and initialize an integer variable myNumber
, with the value 42.Â
Next, we box this value by assigning it to an object variable boxedNumber
. This causes the value of myNumber
to be wrapped in an object on the heap memory.
What is UnBoxing in C#?
Unboxing in C# is the process of converting a reference type (like an object) back to its original value type (such as an integer or boolean).
When you unbox, you extract the value from the reference type and assign it to a value type variable.
It’s like taking the value out of a special container and using it as its original type again.
Code Example of Unboxing:
object boxedNumber = 42; // Boxing an integer value
int unboxedNumber = (int)boxedNumber; // Unboxing the value
// Outputting the unboxed value
Console.WriteLine("Unboxed Number: " + unboxedNumber);
Understanding boxing and unboxing in C#
Let’s learn more about boxing and unboxing in C# programming with some sample code examples.
Sr.No. | Boxing In C# | UnBoxing In C# |
---|---|---|
1. | Boxing converts a Value Type variable (char, int, float, double, etc.) into a Reference Type variable (such as an object or any interface type). | Unboxing is the opposite of boxing. It converts a reference type back to a value type. It extracts a value from a reference type (object) and assigns it to a value type. |
2. | Boxing is an implicit conversion process that automatically converts a value type to the Object type | Unboxing is an explicit conversion process that requires a casting operation to convert the reference type back to its original value type. |
3. | During boxing, the value on the stack is copied into the object created on the heap memory. | During unboxing, the boxed value type is explicitly casted from the object or interface type back to the value type allocated on the stack. |
4. | Value Type variables are stored in Stack memory, while Reference Type variables are stored in Heap memory. | During unboxing, the boxed value type is unboxed from the heap and assigned to the value type allocated on the stack. |
5. | Example: In the example below, the integer variable i is boxed and assigned to the object o. int i = 10; // The following line boxes variable i. object o = i; | Example: Here, you can Unbox the object o and assign it to the integer variable j. int i = 10; // a value type object o = i; // boxing int j = (int)o; // unboxing |
The concept of boxing and unboxing is the core of the type system in C# programming. It creates a bridge between value types and reference types by allowing any value of a value type to be converted to and from an object type.
The following figure demonstrates the boxing & Unboxing:
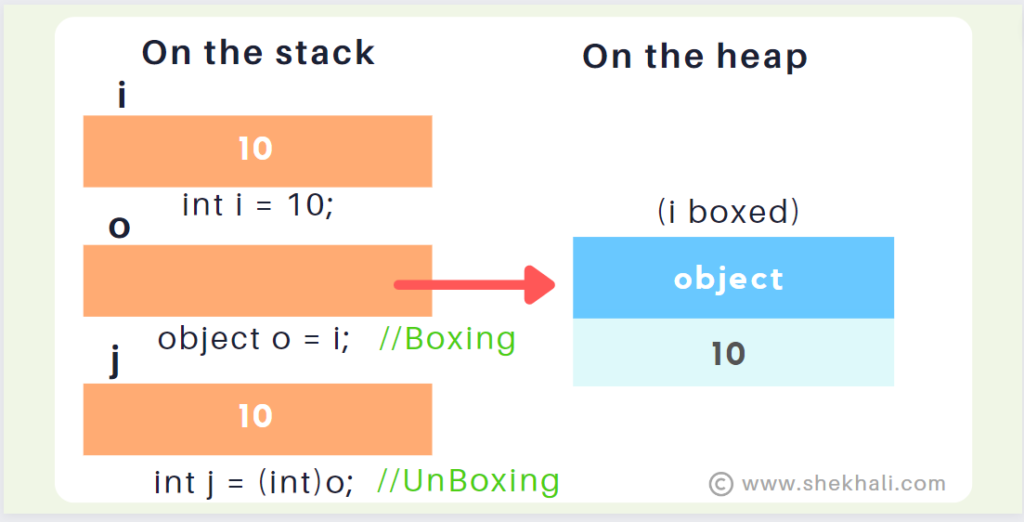
Example 2: Boxing in C#
A boxing conversion creates a copy of a value. Therefore, changing the value of one variable does not affect the other variables.
A C# program to demonstrate boxing is as follows:
using System;
public class BoxingExample
{
public static void Main(string[] args)
{
int num = 100;
object obj = num; // Boxing
Console.WriteLine($"obj = {obj}"); // Output: obj = 100
// Change the value of obj
obj = 101;
Console.WriteLine($"num ={num}, obj= {obj}");//Output: num =100, obj= 101
Console.ReadLine();
}
}
Example: UnBoxing
A C# program to demonstrate Unboxing is as follows:
using System;
public class UnBoxingExample
{
public static void Main(string[] args)
{
int num = 100;
object obj = num; // Boxing
int x = (int)obj; // Unboxing
Console.WriteLine($"obj = {obj} , x = {x}"); //Output: obj = 100 , x = 100
Console.ReadLine();
}
}
Code Explanation:
In the above code snippet, we start by declaring a variable called “num” of type integer (which is a value type).
We assign the value 100 to this variable. In the next step, we box the “num” variable by assigning it to a variable named “obj” of type object (which is a reference type). This process converts the value type into a reference type.
Later on, the value stored in “obj” is unboxed and assigned to a new integer variable named “x”.
Unboxing retrieves the original value from the boxed object and assigns it to a variable of the corresponding value type.
Note: An InvaidCastException will be thrown if the value type does not match the boxed object, as shown in the following example.
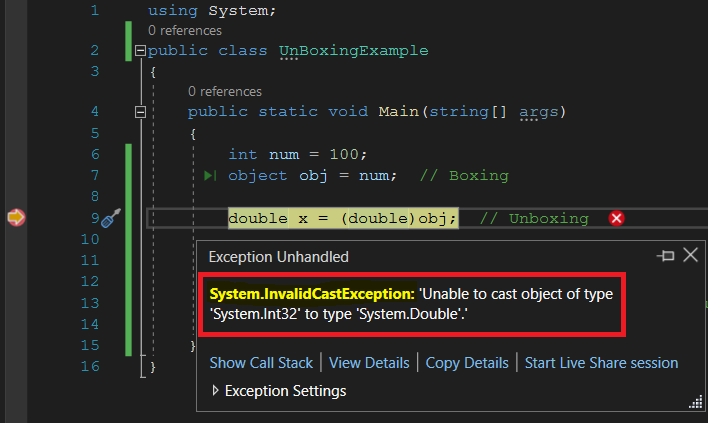
In the above example, the obj points to a boxed object of type int, but we are trying to unbox it to type double rather than int. That’s why the compiler throws InvalidCastException .
C# Boxing Conversions | C# Unboxing Conversions |
---|---|
Boxing conversions allow implicit conversions from value type to reference type. The following box conversions exist in C#: | An unboxing conversion lets you explicitly convert a reference type to a value type. The unboxing conversions are: |
1. Conversion from any value type to the object type. | Conversion from the object type to any value type. |
2. Conversion from any value type to the type System.ValueType | From the type System.ValueType to any value type. |
3. From any non-nullable value type to any interface type implemented by the value type. | From any interface type to any non-nullable value type that implements the interface type. |
4. From any nullable type to any interface type implemented by the underlying type of the nullable type. | From any interface type to any nullable type whose underlying type implements the interface type. |
5. From any enum type to the type System.Enum | From the type System.Enum to any enum type. |
FAQs:
Q: What is boxing in C#?
Boxing is the process of converting a value type to a reference type, such as converting an int to an object.
Q: What is unboxing in C#?
Unboxing is the process of converting a reference type back to its original value type, like converting an object back to an int.
Q: How do generics prevent boxing?
Ans: When you use a Generic List<T>, It allows only elements of a specified type. The compiler generates a special code for that value type and keeps the actual values in the list rather than a reference to an object that contains the values. So no boxing is required.
Q: Why do we need boxing In C#?
Ans: Boxing is required when we have a function that takes an object as a parameter, but we have different value types that need to be passed, and you can’t know the type of a value at compile time.
Q: What is the difference between boxing and unboxing in C# .NET?
The key difference lies in their nature. Boxing involves converting a value type to a reference type (object type), while unboxing refers to the conversion from an object type back to a value type.
Q: What are the disadvantages of boxing in C#?
Boxing has drawbacks, including performance impact, memory overhead, code complexity, and potential type safety issues.
Q: Are there alternatives to boxing and unboxing in C#?
Yes, there are alternatives like using generics, which provide type safety and avoid the need for boxing and unboxing. Value types can also be used directly without converting them to reference types.
Conclusion:
This article has covered the “boxing and unboxing” of variables. We discussed examples of each and examined the results of each process.
It`s also important to note that boxing and unboxing variables require a lot of memory space. When a variable is boxed, a new memory block and object are created. It is advised against using these techniques frequently because continuously boxing and unboxing variables can significantly degrade performance.
References: MSDN-Boxing and Unboxing, “The C# Programming Language” by Anders Hejlsberg
I sincerely hope you enjoyed reading “Boxing and Unboxing“. If you would like to provide more details on the topic covered here. Please leave a comment below.
Articles to Check Out:
- C# Abstract class Vs Interface
- C# Array vs List: When should you use an array or a List?
- C# List Class With Examples
- SOLID Design Principles in C#: A Complete Example
- Understanding Class vs Struct vs Record in C# with examples
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- C# Monitor class in multithreading
- C# Struct vs Class
- C# Dictionary with Examples
- Stream in C#: StreamReader and StreamWriter in C# with examples
- C# Hashtable vs Dictionary
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025