C# Partial classes and partial methods will allow you to split the implementation of a class or a method across multiple files. This can be useful when working with automatically generated code, as it allows you to make changes to the generated code without losing them when the code is regenerated.
The partial
keyword allows you to split the definition of a class, struct, interface, or method into multiple source(.cs) files. Each file contains a part of the definition, and all the parts are combined when the application is compiled.
This article will provide an overview of partial classes and partial methods in C#, including how and why they are implemented.
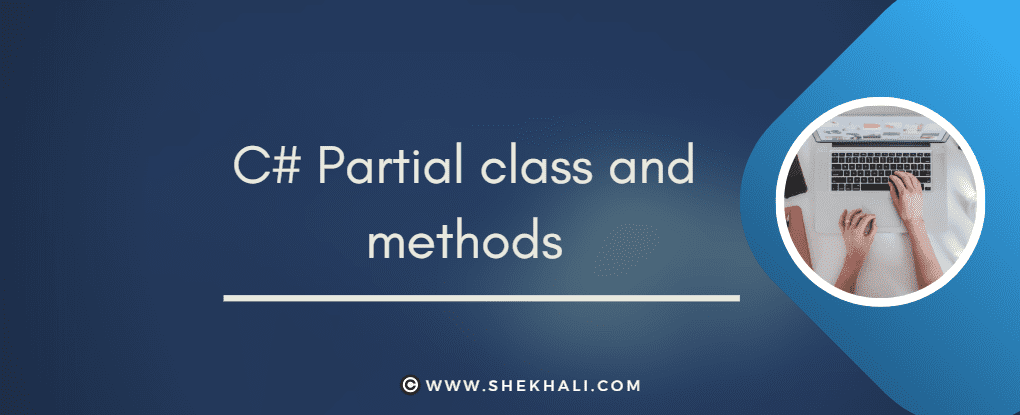
Table of Contents
Introduction: C# Partial Classes
A partial class is a class that is defined across multiple files. To create a partial class, we can use the partial
keyword before the class
keyword in the class definition, like this:
// File: MyClass.cs
public partial class MyClass
{
// Partial class implementation goes here..
}
// File: MyClass.OtherFile.cs
public partial class MyClass
{
// More class implementation goes here..
}
During runtime, the two partial class definitions are merged into a single class. This allows you to use the class like any other class, and you will have access to all the methods, properties, and fields defined in the partial class definitions.
Here is an example of how you might use a partial class in C#:
MyClass obj = new MyClass();
obj.MyMethod(); // Calling a method defined in one of the partial class definitions.
Why do we use partial class in C#?
C# partial classes are useful in a few different scenarios:
Use case | Description |
---|---|
Splitting large classes into smaller chunks: | If we have a large class with a lot of code, it can be helpful to split the class into smaller partial classes, each contained in a separate file. This can make the code easier to navigate and understand. |
Enabling concurrent development: | partial classes can be useful for enabling multiple developers to work on the same class concurrently. Each developer can work on a different part of the class, and the separate parts will be combined into a single class at compile time. |
Working with generated code: | C# Partial classes are often used in conjunction with code generation tools, such as LINQ to SQL or Entity Framework. These tools generate code that defines the structure of a class, and partial classes enable us to write our own code that interacts with the generated code without modifying it. |
Partial Methods in C#
A partial method is a method that is defined in one part of a partial class and implemented in another. Partial methods are declared using the partial
keyword before the void
return type and have no implementation.
Here is an example of how we can declare a partial method in a partial class MyClass.cs
:
namespace PartialClassExample
{
// File: MyClass.cs
public partial class MyClass
{
// Declaration of the partial method.
partial void PartialMethod();
}
}
To implement a partial method, we need to define the method in another part of the partial class, like this:
using System;
namespace PartialClassExample
{
// File: MyClass.Other.cs
public partial class MyClass
{
public void CallPartialMethod()
{
PartialMethod();
}
// Implementation of the partial method.
// Note: a partial method can't have access modifiers
partial void PartialMethod()
{
Console.WriteLine(" Calling Partial method.");
}
}
}
A partial method is a method that can be implemented in any part of a partial class. If it is not implemented, the compiler will remove the method and any calls to it from the generated code. This feature allows you to create optional methods that can be implemented by client code if necessary.
Here is an example of how we might call a partial method in C#:
using System;
namespace PartialClassExample
{
class Program
{
static void Main(string[] args)
{
MyClass obj = new MyClass();
obj.CallPartialMethod();
Console.ReadKey();
}
}
}
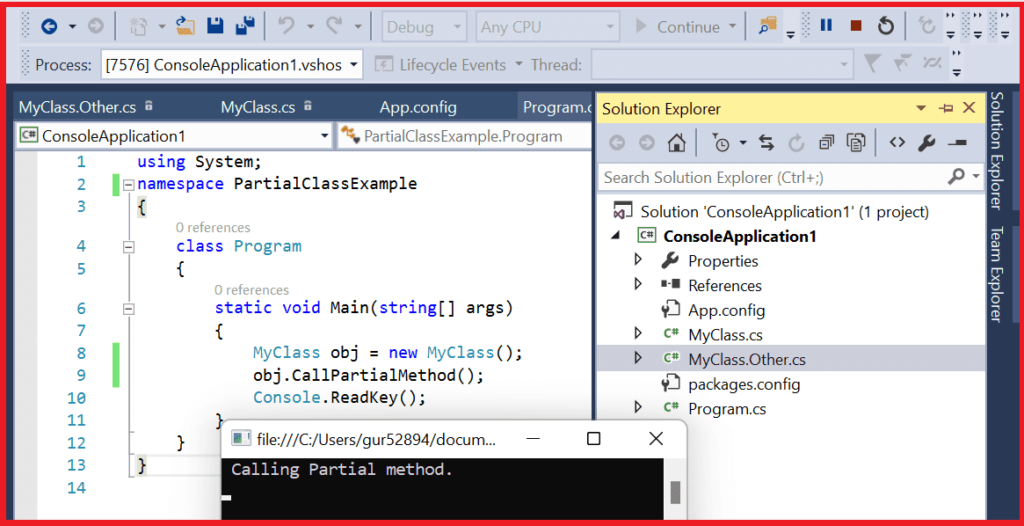
C# Partial class rules
The following are the rules for using partial classes in C#:
- A C# partial class must be declared with the
partial
keyword before theclass
keyword in the class definition. - All of the partial class definitions must be defined in the same namespace.
- The class name must be the same in all of the partial class definitions.
- Partial classes can contain any type of member (fields, properties, methods, etc.) just like any other class.
- Partial classes are often used with code that is generated automatically, as it allows you to make changes to the generated code without losing those changes when the code is regenerated.
- The partial class definitions are combined into a single class during runtime.
- You can create partial class definitions in separate files or in the same file.
- Partial methods can only be declared within partial classes.
- Partial methods must have the
partial
keyword before the return type and must have no implementation. They can have parameters, but they cannot have out parameters. - If a partial method is not implemented in any part of the partial class, the compiler will remove the method and any calls to it from the generated code. This allows you to declare optional methods that can be implemented by client code if needed.
C# Partial method rules
There are a few rules that we need to follow when working with partial methods in C#:
- A partial method in C# must be declared in a partial class or struct.
- The declaration of a partial method must include the partial modifier.
- The return type of a partial method must be
void
. - A partial method can be a static method.
- A partial method must have no access modifiers (such as private or public).
- A partial method can have
ref
but notout parameters.
- A partial method in C# doesn’t have any of the following modifiers virtual, override, sealed, new, or extern.
- A partial method can have parameters, but it cannot have default values for its parameters.
- A partial method must be implemented in the same partial class or struct in which it is declared, or it must be removed entirely.
- If a partial method is not implemented, all references to the method will be removed during compile time.
FAQ:
The following are some common questions about C# partial classes and methods:
Q: Can partial methods have a return type other than the void?
A: No, partial methods in C# must have a return type of void. This is because partial methods are designed to be used as a way of providing an optional implementation for a method that is declared in one part of a partial class and implemented in another part of the same class. If a partial method had a return type other than void, it would not be possible to remove the implementation of the method at compile time if it is not implemented, which is one of the key features of partial methods.
Q: Can partial methods have out parameters?
A: No, A partial method in C# can have a ref
but not out
parameters.
Q: Can partial methods be private or protected access modifiers?
A: Partial methods can’t have any access modifiers (public, private, protected, etc.)
References: MSDN–Partial Classes and Methods
If you enjoyed this post, please share it with your friends or leave a comment below.
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024