C# switch case statement is a selection statement that lets you choose which code block to execute from among many options.
It evaluates an expression and executes the code corresponding to the expression’s matching value.
A switch statement is similar to if-else statements, but it provides a more concise way to compare a single value against a series of multiple possible constant expressions.
In this article, we will explore the C# switch statement and its various use cases.
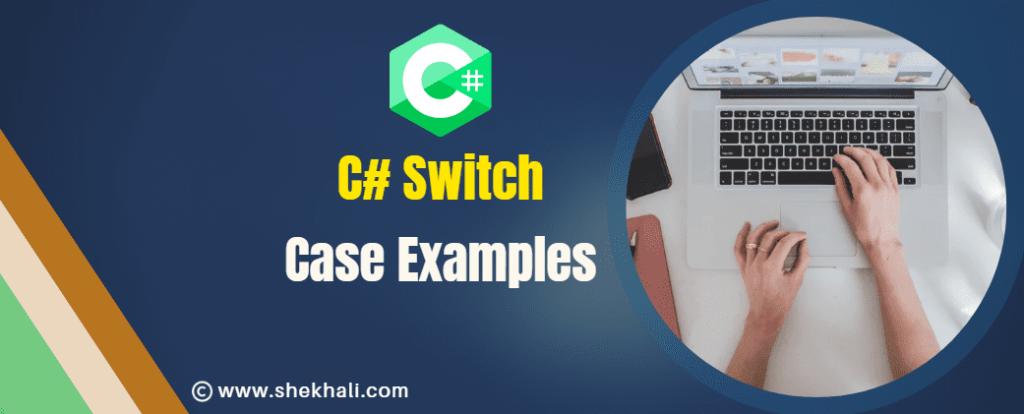
Table of Contents
- 1 Control Flow – C# switch case expression:
- 2 Key Facts About Switch Case in C#
- 3 The break keyword
- 4 The default keyword
- 5 Using goto in the Switch Case Expression
- 6 Case Patterns
- 7 Using when in Switch Expression
- 8 FAQs
- 8.1 Q: What is a switch statement in C#?
- 8.2 Q: What data types can be used with a switch statement in C#?
- 8.3 Q: How do you handle unexpected values in a switch statement?
- 8.4 Q: What is a switch expression in C#?
- 8.5 Q: How do you use the “when” keyword in a switch expression?
- 8.6 Q: Why do we use Switch Statements instead of if-else statements?
- 9 Summary
C# Switch Case Syntax:
The following is the syntax of the switch/case statement.
switch (expression)
{
case value1:
// code to execute when expression == value1
break;
case value2:
// code to execute when expression == value2
break;
default:
// code to execute when none of the above cases match
break;
}
Here, the expression
is the value to be evaluated, and each case is a possible matching value. The default case is executed when no case value matches the expression. The break statement is used to exit the switch block.
Despite its advantage of making the code appear more straightforward than an if-else if statement, the switch statement in C# has certain limitations regarding the data types it can work with.
Specifically, the switch statement is only able to handle the following types of data:
- Primitive data types, such as bool, char, and integral types
- Enumerated types (Enum)
- The String class
- Nullable types of data, as mentioned above types.
Control Flow – C# switch case expression:
The following is the flowchart of the C# Switch statement.
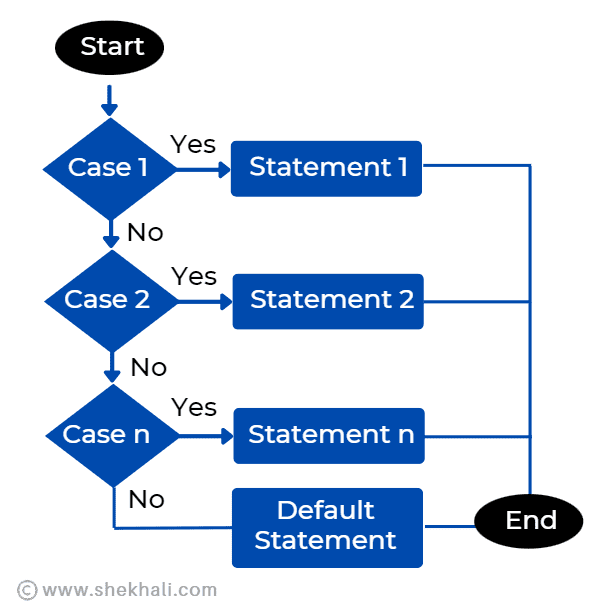
Key Facts About Switch Case in C#
Here are some important points to remember when using switch statements in C#:
- Alternative to Multiple if-else: A switch case statement is an alternative way to handle multiple conditions compared to a series of if-else statements.
- Value-Based Comparison: It involves comparing a single variable or expression against multiple possible constant values.
- Efficient for Multiple Checks: It’s more efficient than using a series of if-else statements when there are several cases to check, as it directly jumps to the correct case without evaluating all conditions.
- Syntax: The syntax includes the keyword
switch
followed by the variable or expression to be checked. Then, each case is specified with the keywordcase
followed by a constant value and a colon. - Break Statement: After executing a case, it’s important to include the break statement to exit the switch block; otherwise, execution will continue to the next case and subsequent cases (fall-through behavior).
- Default Case: The default case is optional and acts as a catch-all if none of the other cases matches. It’s usually placed at the end of the switch statement.
The break keyword
The “break” statement is used to immediately exit or break out of a loop or switch case statement. It stops the further execution of the current code block and moves to the next statement or iteration outside the switch block.
If “break” is not used in a switch case statement, the program will continue executing the code in subsequent cases without any further checks. This is called “fall-through behavior,” where all the code after the matching case will be executed until the switch block’s end or another “break” statement is encountered.
The default keyword
The default
keyword in a switch statement specifies the code to be executed when none of the cases match the input value. It is optional but recommended to include it to handle unexpected values.
Example 1: C# switch Statement
Let’s take a look at an example of C# switch case expression:
using System;
class Program
{
static void Main()
{
int day = 5;
string dayName;
switch (day)
{
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
case 4:
dayName = "Thursday";
break;
case 5:
dayName = "Friday";
break;
case 6:
dayName = "Saturday";
break;
case 7:
dayName = "Sunday";
break;
default:
dayName = "Invalid day";
break;
}
Console.WriteLine("Today is " + dayName);
}
}
Output:
//Output: Today is Friday
In this example, the value of the day
variable is 5, so the case 5 block will be executed, and the output will be “Today is Friday”.
Example 2: C# switch Statement with grouped cases
In some cases, we might want to execute the same code for multiple cases. In this scenario, we can group the cases as follows:
using System;
class Program
{
static void Main()
{
char grade = 'B';
switch (grade)
{
case 'A':
case 'B':
case 'C':
Console.WriteLine("Pass");
break;
case 'D':
case 'F':
Console.WriteLine("Fail");
break;
default:
Console.WriteLine("Invalid grade");
break;
}
}
}
In the above example, we use grouped cases to execute the same code for multiple cases. If the grade variable is A, B, or C, the program will output Pass
. If the grade variable is D or F, the program will output Fail
. The program will output an Invalid grade if the grade variable is any other character.
Example 3: Creating a Basic Calculator Program in C# with Switch Statement
Now, let’s take a look at a more complex example. We can use the switch statement to build a simple calculator program:
using System;
class Program
{
static void Main()
{
int num1, num2, result;
char op;
Console.Write("Enter first number: ");
num1 = int.Parse(Console.ReadLine());
Console.Write("Enter second number: ");
num2 = int.Parse(Console.ReadLine());
Console.Write("Enter operator (+, -, *, /): ");
op = char.Parse(Console.ReadLine());
switch (op)
{
case '+':
result = num1 + num2;
Console.WriteLine("Result: " + result);
break;
case '-':
result = num1 - num2;
Console.WriteLine("Result: " + result);
break;
case '*':
result = num1 * num2;
Console.WriteLine("Result: " + result);
break;
case '/':
if (num2 == 0)
{
Console.WriteLine("Cannot divide by zero");
}
else
{
result = num1 / num2;
Console.WriteLine("Result: " + result);
}
break;
default:
Console.WriteLine("Invalid operator");
break;
}
Console.ReadLine();
}
}
Output:
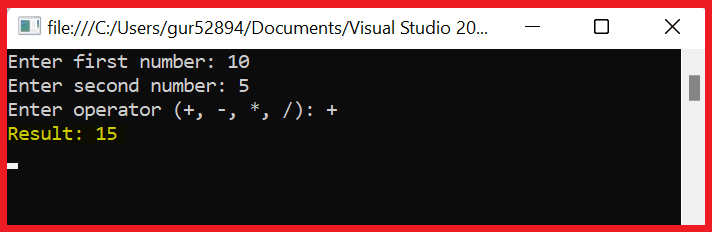
In the above example, we take two numbers, ask for an operator as input from the user, and use the switch statement to perform the corresponding arithmetic operation.
Example 4: Using Enum in a switch statement
Enums are a powerful way to define a set of related values. We can use enums in a switch statement as follows:
using System;
class Program
{
// Using Enum with Switch statement.
enum Days { Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday }
static void Main()
{
Days day = Days.Sunday;
switch (day)
{
case Days.Monday:
Console.WriteLine("Today is Monday");
break;
case Days.Tuesday:
Console.WriteLine("Today is Tuesday");
break;
case Days.Wednesday:
Console.WriteLine("Today is Wednesday");
break;
case Days.Thursday:
Console.WriteLine("Today is Thursday");
break;
case Days.Friday:
Console.WriteLine("Today is Friday");
break;
case Days.Saturday:
Console.WriteLine("Today is Saturday");
break;
case Days.Sunday:
Console.WriteLine("Today is Sunday");
break;
}
Console.ReadLine();
}
}
Output:
Today is Sunday
In this example, we are defining an enum
called Days with seven possible values and using the switch statement to output the name of the selected day.
Example 5: Using multiple case statements in one switch
We can use multiple case statements in a single switch block as follows:
using System;
class Program
{
static void Main()
{
int age = 25;
switch (age)
{
case 18:
case 19:
case 20:
case 21:
case 22:
case 23:
case 24:
case 25:
Console.WriteLine("You are between 18 and 25 years old");
break;
default:
Console.WriteLine("You are not between 18 and 25 years old");
break;
}
Console.ReadLine();
}
}
Switch statements are often more concise and easier to read than a series of if-else statements. They also allow for faster execution in some cases, as the compiler can optimize the code for switch statements.
Using goto
in the Switch Case Expression
In C#, we can use the goto
statement to jump to a specific label in a switch block. Here is an example:
using System;
class Program
{
static void Main()
{
int num = 1;
switch (num)
{
case 1:
Console.WriteLine("Case 1");
goto case 2;
case 2:
Console.WriteLine("Case 2");
break;
}
Console.ReadLine();
}
}
In this example, we are using the `goto` statement to jump from `case 1` to `case 2`.
Case Patterns
Starting with C# 7.0, we can use patterns in switch cases to match more complex values. Here are a few examples:
Type Pattern
using System;
class Program
{
static void Main()
{
object obj = "Hello";
switch (obj)
{
case string s:
Console.WriteLine("The object is a string with value " + s);
break;
case int i:
Console.WriteLine("The object is an integer with value " + i);
break;
default:
Console.WriteLine("The object is neither a string nor an integer");
break;
}
}
}
In this example, we are using the type pattern to match the type of the obj
object.
Using when
in Switch Expression
Starting with C# 7.0, you can use the “when” keyword to add additional conditions to a switch expression. These conditions can filter the values of the switch expression and only execute the code in the corresponding case if the condition is true.
Here is an example that uses the “when” keyword in a switch expression:
using System;
public class WhereInSwitchStatement
{
public static void Main(string[] args)
{
int age = 25;
string result = age switch
{
int n when n < 18 => "You are not an adult",
int n when n >= 18 && n < 65 => "You are an adult",
int n when n >= 65 => "You are a senior citizen",
_ => "Invalid age input"
};
Console.WriteLine(result);
}
}
Output:
You are not an adult
Reference: MSDN-Selection Statements
FAQs
Here are some frequently asked questions (FAQs) about the C# switch statement:
Q: What is a switch statement in C#?
A switch statement in C# is a control statement that allows you to select one of many code blocks to be executed based on the value of an input expression.
Q: What data types can be used with a switch statement in C#?
We can use primitive data types like bool, char, and integral types, as well as enumerated types and the String class with a switch statement in C#.
Q: How do you handle unexpected values in a switch statement?
The default case is a catch-all case that is executed when none of the cases match the input value. Including a default case in the switch statement is important to handle unexpected values.
Q: What is a switch expression in C#?
A switch expression is a newer feature in C# that allows you to use a switch statement as an expression, which can be assigned to a variable or used as part of a larger expression.
Q: How do you use the “when” keyword in a switch expression?
The “when” keyword is used to add additional conditions to a switch expression, allowing you to filter the input values and execute code in the corresponding case only if the condition is true.
Q: Why do we use Switch Statements instead of if-else statements?
We use switch statements instead of if-else statements when we have a single value to check against multiple possible values. Switch statements offer a more organized and efficient way to handle such scenarios, making the code clearer and potentially faster because they directly jump to the right case, avoiding unnecessary checks.
Summary
In this article, we learned about the switch statement in C#. We have seen several examples of switch statements with different input types, including strings, integers, and enums.
We have also discussed why switch statements are often preferred over if-else statements and some important points to remember when using switch statements in C#. Using switch statements effectively can write more concise, readable, and maintainable code in C#.
You might also like:
- C# Tutorial
- Difference Between if-else and switch: A Side-by-Side Comparison of If-Else and Switch Case
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- C# Struct vs Class |Top 15+ Differences Between C# Struct and Class
- 10 Difference between interface and abstract class In C#
- Local vs Global Variables
- Generic Delegates in C# With Examples: Func, Action, and Predicate delegates
- Constructors in C#: A Comprehensive Guide with Code Examples
- Top 5 Difference between Call by Value and Call by Reference
- C# stack vs heap
- Readonly vs const in C#
- C# String VS StringBuilder
- Fields vs Properties In C#: Understanding the difference between Field and Property in C#
Let others know about this post by sharing it and leaving your thoughts in the comments section.
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024