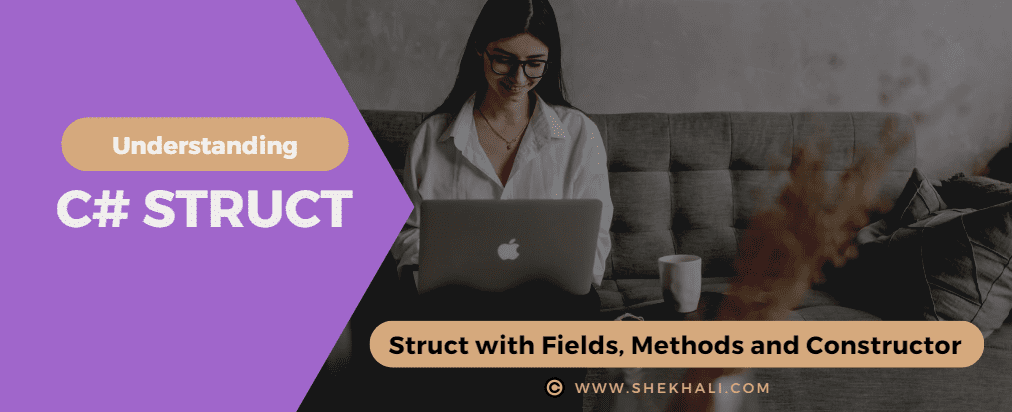
A C# Struct is a value type that represents a lightweight object. Unlike classes, which are stored on the heap, a struct is stored on the stack, making it more memory-efficient and faster to create and use.
Struct is a perfect choice for creating small, lightweight objects that hold value-type data and do not require inheritance.
It can also contain a parameterized constructor, static constructor, fields, properties, constants, indexers, operators, nested types, and events.
However, there are some limitations to consider.
Unlike classes, structs cannot be inherited from other structs or classes. Additionally, certain advanced features, such as virtual methods and polymorphism, are not supported by Struct in C#.
Table of Contents
- 1 Define struct in C#
- 2 Key points about Struct in C#
- 3 Example : Create Structure
- 4 C# Struct with Default Constructor
- 5 Can a struct have events in C#?
- 6 Difference between struct and class in C#
- 7 FAQ
- 7.1 Q: What is a struct in C#?
- 7.2 Q: How can you define a struct in C#?
- 7.3 Q: Can a struct inherit from another struct or class in C#?
- 7.4 Q: Can a struct implement interfaces in C#?
- 7.5 Q: What is the performance difference between a struct and a class in C#?
- 7.6 Q: Can a struct be null in C#?
- 7.7 Q: Can a struct have a destructor or finalizer in C#?
- 7.8 Q: Can a struct have properties in C#?
- 7.9 Q: Can a struct have methods in C#?
- 7.10 Related
Define struct in C#
In C#, you can declare a structure using the struct
keyword followed by the name of the struct.
Below is a code example of a simple struct declaration in C#:
Example 1: Creating a 2D Point Struct
public struct Point
{
public int X;
public int Y;
}
Example 2: Creating a Date Struct
public struct Date
{
public int Day;
public int Month;
public int Year;
}
Key points about Struct in C#
Following are some essential points about structures in C#:
Here are some key points about structures in C#:
- Structs in C# are value types stored on the stack.
- A struct does not support inheritance and cannot inherit from another struct or class, nor can it be used as a base class.
- A struct can contain constructors, fields, methods, properties, and other members similar to classes. However, it cannot have destructors or finalizers.
- You can create a struct object with or without the new operator, similar to primitive type variables.
- Structs are typically used to represent small, simple objects and store value-type data, such as points, rectangles, and complex numbers.
- Structures are mutable, allowing you to modify their fields and properties after creation.
- Structures are value-type or value-based, meaning they are compared by their contents rather than their reference.
- In C#, you can create structures using the
struct
keyword. - Even though structures do not support inheritance, interfaces can still be implemented by structs.
- Struct members cannot be marked as abstract, virtual, sealed, or protected.
Example : Create Structure
We can create a struct object with or without the new
keyword, similar to primitive type variables.
In the following code example, we create a structure named Person without a new operator.
using System;
// Define a C# struct called 'Person' to represent an individual.
public struct Person
{
// Struct fields to store information about the person.
public string Name;
public int Age;
public string Address;
// Parameterized constructor for the Person struct.
public Person(string name, int age, string address)
{
Name = name;
Age = age;
Address = address;
}
// Method to display information about the person.
public void DisplayInfo()
{
Console.WriteLine($"Name: {Name}");
Console.WriteLine($"Age: {Age}");
Console.WriteLine($"Address: {Address}");
}
}
class Program
{
static void Main()
{
// Create an instance of the 'Person' struct and initialize its values.
Person person1 = new Person("Shekh Ali", 30, "123 Noida, India");
// Access and modify struct fields directly.
person1.Age = 31;
// Call the method to display information about the person.
Console.WriteLine("Information of person1:");
person1.DisplayInfo();
// Create another instance of the 'Person' struct using the parameterized constructor.
Person person2 = new Person("Roman", 25, "456 Elm Avenue");
// Call the method to display information about the second person.
Console.WriteLine("\nInformation of person2:");
person2.DisplayInfo();
}
}
Output:
Information of person1:
Name: Shekh Ali
Age: 31
Address: 123 Noida, India
Information of person2:
Name: Roman
Age: 25
Address: 456 Elm Avenue
If you declare a structure type variable without using the new
keyword, the default constructor will not be invoked, and the structure members will remain uninitialized.
Consequently, this will lead to a compile-time error. To avoid this error, ensure that you assign values to each member of the structure before accessing them.
Example : C# Struct With Constructor and Method
You can also add constructors, methods, properties, and other members to a struct just like you would with a class. In the following code example, we create a new struct instance using the new operator and a constructor.
using System;
namespace StructExample
{
struct Point
{
// Fields
public int X;
public int Y;
// Constructor
public Point(int x, int y)
{
X = x;
Y = y;
}
// Method
public double CalculateDistanceToOrigin()
{
return Math.Sqrt(X * X + Y * Y);
}
}
class Program
{
static void Main(string[] args)
{
Point p1 = new Point(1, 2);
Point p2 = new Point(3, 4);
double point1 = p1.CalculateDistanceToOrigin();
double point2 = p2.CalculateDistanceToOrigin();
Console.WriteLine($" Point1 : {point1} , Point2: {point2}");
Console.ReadKey();
}
}
}
In this above code example, we added a constructor to the Point
struct that allows us to create a new Point
object and initialize its X
and Y
fields. We also added a method called CalculateDistanceToOrigin
that calculates the distance of the point from the origin (0, 0).
We have created two new Point
objects p1
& p2
with the coordinates (1, 2) and (3, 4), respectively. We then access the fields and methods of the struct using the dot operator (.
).
Once we run the code, we will get the following result:
Point1 : 2.23606797749979 , Point2: 5
C# Struct with Default Constructor
In C#, when using a struct, you cannot declare a default or parameterless constructor. Additionally, the struct does not allow field initialization with values, except for fields marked as const or static.
struct Person
{
// Compile time error
public string name = "Shekh Ali";
public int age;
// Compile time error
public Person()
{
age = 32;
}
}
Struct with static and constant fields.
using System;
// Define a C# struct called 'MyStruct'.
public struct MyStruct
{
// Constants can be initialized with values at declaration.
public const int MyConstField = 10;
// Static fields are shared across all instances and can be initialized at declaration.
public static int MyStaticField = 20;
// This is a non-const, non-static field of the struct.
// Regular fields cannot be initialized with values at declaration in a struct.
public int MyField;
}
class Program
{
static void Main()
{
// Create an instance of the 'MyStruct' struct.
MyStruct myStructInstance;
// Attempt to access the 'MyField' field without assigning a value.
// This will result in a compile-time error since 'MyField' is not marked as const or static.
// Uncomment the line below to see the compile-time error.
// Console.WriteLine(myStructInstance.MyField); // Error: Use of unassigned local variable 'myStructInstance'.
// Access the 'MyConstField' and 'MyStaticField' without creating an instance of 'MyStruct'.
// Constants and static fields are accessible without an instance.
Console.WriteLine("MyConstField: " + MyStruct.MyConstField);
Console.WriteLine("MyStaticField: " + MyStruct.MyStaticField);
// Assign a value to the 'MyField' field before accessing it.
// Since it's a non-const, non-static field, it needs explicit assignment before use.
myStructInstance.MyField = 30;
Console.WriteLine("MyField: " + myStructInstance.MyField);
}
}
Output:
MyConstField: 10
MyStaticField: 20
MyField: 30
Can a struct have events in C#?
A struct can have events in C# just like a class. Events provide a way for the struct to notify other objects when something happens or changes within the struct.
Example: Event in Struct
Here is an example of a struct with an event in C#:
using System;
namespace StructWithEventExample
{
struct Point
{
public event Action<int,string> Moved;
private int x;
private int y;
public int X
{
get { return x; }
set
{
x = value;
Moved?.Invoke(x,"X");
}
}
public int Y
{
get { return y; }
set
{
y = value;
Moved?.Invoke(y,"Y");
}
}
}
class Program
{
private static void OnPointMoved(int value, string Cord)
{
Console.WriteLine($"Coordinate {Cord}: changed to {value} ");
}
static void Main(string[] args)
{
Point p = new Point();
p.Moved += OnPointMoved;
p.X = 5;
p.Y = 10;
Console.ReadKey();
}
}
}
In the above code example, the Point struct has two fields, x, and y, representing the point coordinates. It also has two properties, X and Y, that provide access to these fields.
The setters of these properties invoke the Moved event to notify other objects whenever the point coordinates are changed.
To use the Point struct, you can create an instance of it and subscribe to the Moved event:
Then, you can define the OnPointMoved
event handler to handle the Moved event:
The output of the above program is as follows:
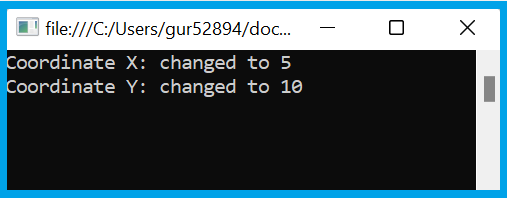
Difference between struct and class in C#
Here is a list of differences between struct and class in C#:
- Definition: A struct is a value type, while a class is a reference type.
- Memory allocation: In C#, the Stack memory stores a struct object, while a class object is stored on the heap memory. However, a reference to the heap memory is stored on the Stack.
- Default member accessibility: The members of a struct are public by default, whereas the members of a class are private by default.
- Inheritance: A struct cannot inherit from another struct or class, while a class can inherit from another class in C#.
- Destructors: A struct does not support destructors or finalizers. At the same time, a class can have a destructor or finalizer to clean up resources before the object is garbage collected.
- Nullability: A reference type (such as a class) can be assigned a null value, while a value type (such as a struct) cannot.
- Performance: Structs perform better than classes due to their value type nature and faster memory allocation on the Stack.
FAQ
The following are some frequently asked questions and answers about struct in C#:
Q: What is a struct in C#?
A: A struct in C# is a value type that represents a lightweight object. It is similar to a class but has some key differences such as memory allocation, default member accessibility, and inheritance. Structs are useful for representing small, simple objects that don’t require the overhead of a full-fledged class.
Q: How can you define a struct in C#?
A: To define a struct in C#, use the struct
keyword followed by the name of the struct and a set of curly braces { }
that contain the members of the struct. Following is an example of a struct:
struct Coordinate
{
public int X;
public int Y;
}
Q: Can a struct inherit from another struct or class in C#?
No, a struct cannot inherit from another struct or class in C#. A struct is a value type that cannot inherit from a reference type.
Q: Can a struct implement interfaces in C#?
Yes, a struct can implement interfaces in C#, just like a class. It allows a struct to define and implement the behaviors specified by the interface.
Q: What is the performance difference between a struct and a class in C#?
In general, structs can perform better than classes due to their value type nature and faster memory allocation on the stack.
Q: Can a struct be null in C#?
A: No, a struct cannot be assigned a null value in C# because it is a value type.
Q: Can a struct have a destructor or finalizer in C#?
No, a struct cannot have a destructor or finalizer in C#. Destructors and finalizers are used to clean up resources before an object is a garbage collected. Still, structs do not support garbage collection because they are value types. Instead, structs rely on their value-type nature to automatically reclaim their memory when they go out of scope or are no longer needed.
Q: Can a struct have properties in C#?
A struct can have properties in C# just like a class. Properties are a convenient way to encapsulate the data and behavior of a struct. We can use them to provide access to the underlying fields of the struct in a controlled and consistent way. Here is an example of a struct with a property:
// Defining structure
struct Coordinate
{
// private fields
private int x;
private int y;
//public Properties
public int X {
get { return x; }
set { x = value; }
}
public int Y
{ get { return y; }
set { y = value; }
}
}
Q: Can a struct have methods in C#?
A: Yes, a struct can have methods in C# just like a class.
Here are some recommended articles on collections:
- C# Tutorial
- C# Queue with examples
- Difference between SortedList and SortedDictionary in C#
- C# Stack
- Is vs As operator in C#
- C# Dictionary
- C# Hashtable
- Collections in C#
- Array vs List
- C# List with examples
- C# Arrays
- C# Hashtable vs Dictionary vs HashSet
- C# Static Class, Methods, Constructors, and Fields with examples
- C# Polymorphism: Different types of polymorphism in C# with examples
- C# Params
- Serialization and Deserialization in C# with Examples
References: MSDN-Structure type
Please share this post and let us know what you think in the comments.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025