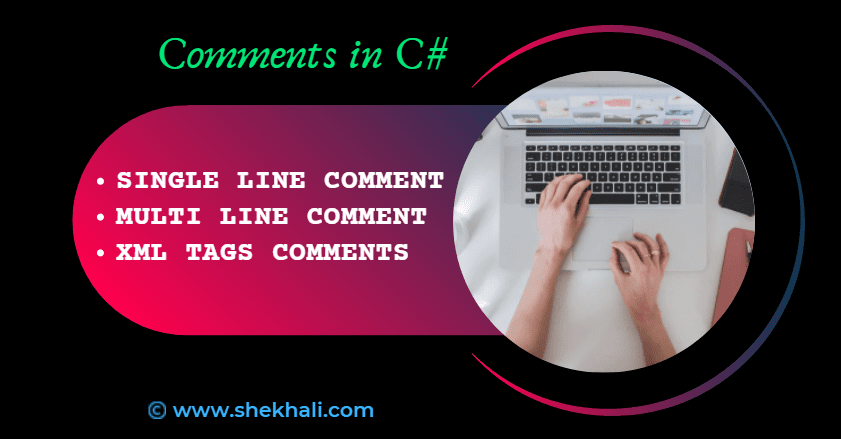
Table of Contents
Introduction: Comments in C#
Comments are used in a program to make a section of code easier to understand. They’re used to make the code more readable and provide a formal description of it and how it works.
The compiler ignores comments, so you may put them wherever in a program and not worry about them breaking it.
Types of Comments in C#
We have three different types of comments in C#. Let’s dive into each of these comments.
- Single Line Comments ( // )
- Multi Line Comments (/* */)
- XML Comments ( /// )
Single Line Comments
A single-line comment starts with a double forward slash (//). The compiler will ignore the code after the double forward slash and will not be executed.
These comments can be written in a different line or the same line as the codes and are perfect for quick explanations or highlighting important details about the code.
Here is an example:
// This is an example of single line comment
int x = 10; // Initializing a variable
When to use Single-Line Comments?
Purpose: Single-line comments are concise and serve as quick explanations or reminders. Use them for the following scenarios:
- Inline Explanations: When you need to clarify a specific line of code.
- Temporary Disabling: To temporarily disable a line of code without removing it.
- Highlighting Important Details: To draw attention to critical information.
- Syntax: Start with
//
followed by your comment text.
Multi-Line Comments in C#
Multiline Comments are used to comment on multiple lines at once. It is commonly used to write more extensive explanations of an entire section of code.
The Multi-line comments begin with /*
and end with */
.
These are great for documenting entire methods, classes, Interfaces or chunks of code.
Note: The C# compiler will ignore any text between /* and */.
Here is an example of multiline comments in C#:
/* This function computes the factorial of a given
number using recursion */
int CalculateFactorial(int num)
{
if (num == 0)
return 1;
else
return num * CalculateFactorial(num- 1);
}
When to use Multi-Line Comments (Block Comments) ?
Purpose: Multi-line comments allow for more extensive explanations. Use them for the following scenarios:
- Documenting Methods or Classes: Describe entire methods, classes, or sections of code.
- Providing Context: Explain complex logic or algorithms.
- Adding Legal Notices or Copyright Information.
- Syntax: Enclose your comment within
/*
and*/
.
XML documentation comment
The XML documentation comments start with three forward slashes (///) and use XML-like tags. These comments are crucial for creating comprehensive code documentation.
The XML file can then be styled with XML style sheets to provide elegant code documentation that can be seen in a browser.
(Please refer this MSDN link for more information: XML documentation comment)
Let’s see how they work:
using System;
/// <summary>
/// A simple class representing a geometric shape - Rectangle.
/// </summary>
public class Rectangle
{
/// <summary>
/// Gets or sets the length of the rectangle.
/// </summary>
public double Length { get; set; }
/// <summary>
/// Gets or sets the width of the rectangle.
/// </summary>
public double Width { get; set; }
/// <summary>
/// Calculates the area of the rectangle.
/// </summary>
/// <returns>The area of the rectangle.</returns>
public double CalculateArea()
{
// The area formula for a rectangle: length * width
return Length * Width;
}
/// <summary>
/// Prints information about the rectangle.
/// </summary>
public void DisplayInfo()
{
Console.WriteLine($"Rectangle - Length: {Length}, Width: {Width}, Area: {CalculateArea()}");
}
}
In this code example:
summary
tags provide a brief description of the class and its members.param
tags describe parameters for methods or properties.returns
tag describes the return value of a method.- The XML comments are placed directly above the code they document.
When to use XML Documentation Comments?
Purpose: The XML Documentation Comments are special and serve as documentation for your code. Use them when:
- Creating Libraries or APIs: Other developers will rely on your documentation.
- Generating IntelliSense and Tooltips: These comments provide context.
- Syntax: Start with
///
followed by XML-like tags.
Note: we can use
Ctrl+K+C
andCtrl+K+U
to Comment or Uncomment selected lines in C#.
Why Use Comments?
Comments enhance code readability and maintainability, especially in larger projects or when collaborating with other developers. You must Keep the following guidelines in mind:
- Single-line comments: Use them for quick explanations.
- Multi-line comments: Reserve them for more detailed context.
- XML documentation comments: Essential for thorough code documentation. These comments provide information about classes, methods, parameters, and return values.
You must strike a balance between comments and self-explanatory code. Well-named variables, clear structures, and effective design go hand in hand with comments.
Summary:
In this post, You’ve learned about the different types of comments in C#, how to use them, and, most importantly, why to use them.
We looked at single-line, multi-line and XML comments in C#.
Code comments can help you better understand your code and other programmers who will use it.
FAQs:
Q: When should you use a single-line or multi-line comment?
Ans: It is entirely up to you which approach you wish to employ. We usually use single-line // comments for short comments and multi-line /* */ comments for longer ones.
Q: What are comments in C#?
Ans: Comments are used in a program to help the reader understand a portion of the code. They’re used to make the code more readable and provide a formal description of how it works.
Q: What are the various types of comments in C#?
Ans: There are three different types of comments in C#: single line, multi-line, and XML documentation comments.
Q: How do you comment multiple lines in C#?
Ans: Multi-line comments begin with /* and ends with */ . The compiler in C# will ignore any text between / *and */.
Q: What are some of the benefits of XML comments?
Ans: XML comments help speed up development by providing intellisense to custom methods and other code structures. XML comments facilitate code reuse by providing a path for generating API-style reference documents from source code.
XML documentation comments in C# begin with a triple slash, ///. Comments are enclosed in XML tags.
Articles to Check Out:
- C# Tutorial
- C# Programs asked in Interviews
- C# Collections-(Stack, Queue, Array, dictionary, Hashtable, ArrayList, SortedList)
- Constructors in C# with Examples
- C# Abstract class Vs Interface
- Abstraction vs Encapsulation in C#
- WCF vs WEB API
- Top 50 C# Interview Questions And Answers For Freshers And Experienced
- Collections in C# .NET
- ASP.NET Core Middleware With Examples
- C# Struct vs Class
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024