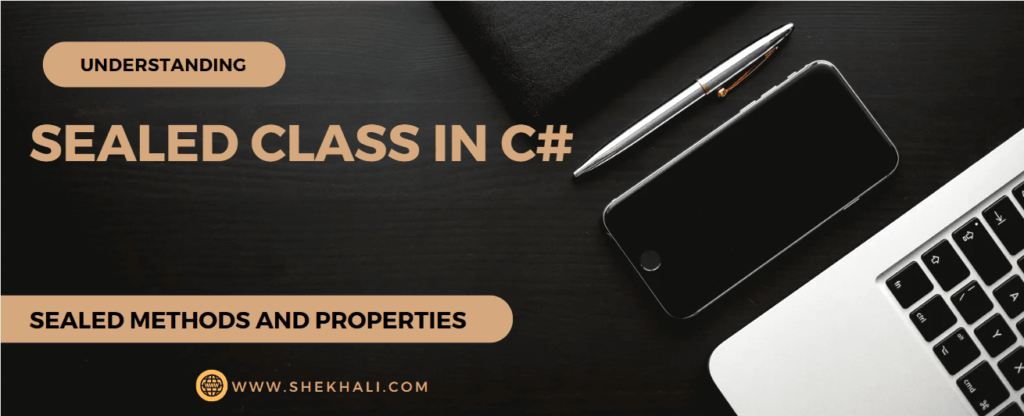
A sealed class in C# is a class that cannot be inherited or modified by any other class but can still be instantiated. It is not allowed to have any derived classes. Sealed classes are commonly used to prevent accidental inheritance, ensuring that the class remains as it is without any modifications.
The main purpose of a sealed class is to indicate that it is highly specialized and does not require any additional functionality through inheritance. It means that it cannot be extended to add new behaviors or modify its existing behavior.
Table of Contents
- 1 Points to remember about sealed class in C#
- 2 C# Sealed method
- 3 FAQs:
- 3.1 Q: What is a sealed class in C#?Â
- 3.2 Q: Why would I use a sealed class?
- 3.3 Q: Can we create an object of a sealed class in C#?
- 3.4 Q: Can you override a sealed class in C#?
- 3.5 Q: What is the advantage of the sealed keyword in C#?
- 3.6 Q: Are sealed classes beneficial for performance?
- 3.7 Q: Can a sealed class inherit from another class?
- 3.8 Q: How do I create a sealed class in C#?
- 4 Summary:
Points to remember about sealed class in C#
- Cannot be inherited: A sealed class is declared using the sealed keyword, which prevents other classes from inheriting it. It ensures that the sealed class remains as it is and cannot be extended or modified through inheritance.
- Allows instantiation: Despite not being inheritable, a sealed class can still be instantiated, meaning objects of the sealed class can be created and used in the program.
- Specialized and self-contained: The design intent of a sealed class is to indicate that it serves a specific purpose or encapsulates a certain logic. It is intended to be self-contained and doesn’t require additional functionality through inheritance.
- Prevents overriding: By sealing a class, you prevent derived classes from overriding its behavior by overriding its methods or properties. It ensures that the sealed class’s implementation remains unchanged.
- Used for encapsulation: Sealed classes are commonly used to encapsulate a specific set of functionality or logic that needs to be used across the program. They provide a way to package and reuse code without allowing modifications or extensions.
- Performance benefits: Sealed classes can provide performance benefits since the compiler can optimize calls to sealed methods more efficiently than virtual or overridden methods.
- Design choice: Choosing to make a class sealed should be based on carefully considering the class’s purpose and the need for inheritance. If a class is not intended to be extended or modified, making it sealed can help improve code maintainability and prevent unintended changes.
Sealed Class Example:
The following is an example of Sealed class in C#.
using System;
sealed class MySealedClass
{
public int num1;
public int num2;
}
class Program
{
// Main Method
static void Main()
{
// Creating an object of Sealed Class
MySealedClass sc = new()
{
num1 = 100,
num2 = 200
};
// Printing value of num1 and num2
Console.WriteLine($"num1 = {sc.num1}, num2 = {sc.num2}");
}
}
// Output: num1 = 100, num2 = 200
Now, if we try to inherit a child class from a sealed class, an error will be produced stating that ‘ChildClass’: cannot derive from the sealed type ‘MySealedClass’.
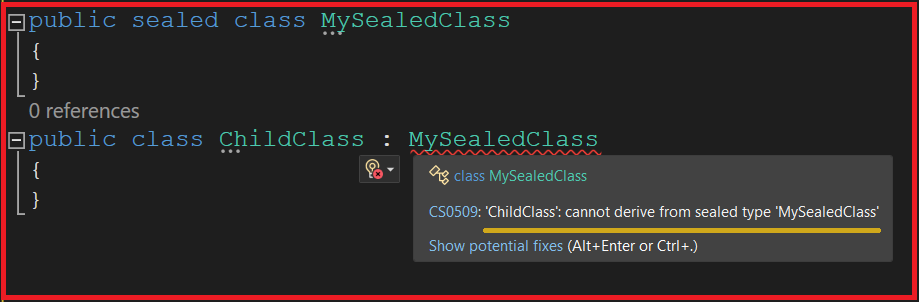
C# Sealed method
In C#, a sealed method is a method that is declared within a class and cannot be overridden by derived classes. It must be declared using sealed and override keywords. When a method is marked as sealed using the sealed keyword, it prevents any further modification or extension of that method in subclasses.
It is useful when you want to restrict the ability to override specific methods in a class hierarchy to maintain consistency or security.
Sealed method example:
In the code below, if you want to restrict the Child2
class from overriding the Display
method, you must use the sealed modifier with the override modifier in the Child1
class. This will prevent the method from being overridden in derived classes.
class Parent
{
public virtual void Display() { }
}
class Child1 : Parent
{
public sealed override void Display() { }
}
class Child2 : Child1
{
// 'Child2.Display()': cannot override inherited member 'Child1.Display()' because it is sealed
public override void Display() { }
}
FAQs:
Q: What is a sealed class in C#?Â
A sealed class in C# is a special type of class that cannot be inherited by other classes. It is marked with the sealed keyword and is designed to serve a specific purpose without allowing modifications or extensions through inheritance.
Q: Why would I use a sealed class?
Sealed classes
are used when you want to create a class that should not be inherited or modified. They are typically employed to encapsulate a specific logic or functionality that can be used throughout a program without alteration.
Q: Can we create an object of a sealed class in C#?
Yes, even though a sealed class cannot be inherited, you can still create instances (objects) of the sealed class and use them in your program.
Q: Can you override a sealed class in C#?
You cannot inherit from a sealed class, so no inheritance, no override.
Q: What is the advantage of the sealed keyword in C#?
The sealed keyword in C# allows you to prevent the inheritance of a class or certain class members that were previously marked as virtual.
Q: Are sealed classes beneficial for performance?
Sealed classes can provide performance benefits. Since sealed methods cannot be overridden, the compiler can optimize calls to these methods more efficiently than virtual or overridden methods.
Q: Can a sealed class inherit from another class?
Yes, a sealed class can inherit from another class. However, other classes cannot further inherit the sealed class itself.
Q: How do I create a sealed class in C#?
Use the sealed keyword before the class declaration to create a sealed class. It marks the class as sealed and prevents inheritance by other classes.
Summary:
In summary, we can conclude that sealed
classes or sealed methods are extremely helpful when we don`t want to expose the functionality to the derived class. In addition, they are useful when you want to restrict your code so developers can’t extend it. A sealed class cannot be derived by any other class and a sealed method cannot be overridden.
References: MSDN- sealed (C# Reference), stackoverflow-Sealed method in C#
Articles to Check Out:
- C# Abstract class Vs Interface
- DateTime Format In C# (with examples)
- Generic Delegates in C# With Examples
- Serialization and Deserialization in C# with Example
- C# Array vs List: When should you use an array or a List?
- C# Monitor class in multithreading
- C# Struct vs Class
- C# Dictionary with Examples
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- C# Dependency Injection
- Design Patterns
I hope you liked reading this article “sealed class in C#”. Please leave a comment below if you discover an error or would like to provide more details about the subject covered here.
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024