The main difference between ArrayList and List in C# is that ArrayList can hold any type of object, but it doesn’t enforce type-safety. On the other hand, List is a generic collection that enforces type-safety by allowing you to specify the type of elements it stores.
List is generally preferred over ArrayList for better performance, readability, and compile-time type checking.
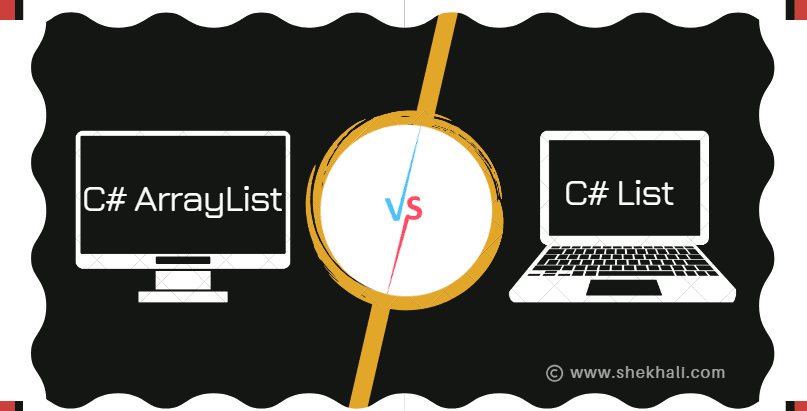
In this article, we will explore the key differences and benefits of C# ArrayList and List, along with code examples and explanations.
Table of Contents
- 1 What is ArrayList in C#?
- 2 What is List in C#?
- 3 Differences between ArrayList and List in C#
- 4 ArrayList:
- 5 List:
- 6 FAQs
- 6.1 Q: What is the difference between ArrayList and List in C#?
- 6.2 Q: Which collection type is recommended for modern C# development?
- 6.3 Q: Can ArrayList and List automatically resize when adding or removing elements?
- 6.4 Q: Should I use List or ArrayList?
- 6.5 Q: Does ArrayList allow duplicates in C#?
- 6.6 Q: Which is faster, ArrayList or List in C#?
- 6.7 Related
What is ArrayList in C#?
ArrayList in C# is a collection that can store different types of data without any restrictions. That means we can store integers, strings, or other objects based on our needs.
To use ArrayList in our applications, we must include the System.Collections
namespace. It was introduced in the .NET 2.0
framework, but now we have better alternatives, such as List<T>, which provide more benefits and type safety.
ArrayList
was used when C# didn’t have generics, but now it’s considered old-fashioned. It’s recommended not to use ArrayList in new code higher than .NET version 2.0
unless you need to work with an older API that specifically requires it.
Here is an example of ArrayList in C#:
using System;
using System.Collections;
class Program
{
static void Main()
{
// Creating an ArrayList
ArrayList arrayList = new ArrayList();
// Adding elements to the ArrayList
arrayList.Add("Shekh Ali");
arrayList.Add(25);
arrayList.Add(true);
// Reading data from the ArrayList by index
string name = (string)arrayList[0];
int age = (int)arrayList[1];
bool isAdult = (bool)arrayList[2];
// Displaying the values
Console.WriteLine("Name: " + name);
Console.WriteLine("Age: " + age);
Console.WriteLine("Is Adult: " + isAdult);
}
}
Output:
Name: Shekh Ali
Age: 25
Is Adult: True
Code Explanation:
In the above code, we create an ArrayList
and add elements of different types, such as a string, an integer, and a boolean.
ArrayList
allows this heterogeneous mixture of elements. However, when retrieving elements from an ArrayList, we need to explicitly cast them to their respective types, which can lead to runtime errors if not handled properly.
What is List in C#?
The List is a generic collection introduced in .NET 3.0, and it provides type safety by allowing you to specify the type of objects it can hold. List<T>
is a generic class, where T represents the type of elements that the List can store.
In C#, we need to include the System.Collections.Generic
namespace to use a Generic List in our application.
A List allows us to store and manipulate a group of objects of the same type. It is like a dynamic array that can grow or shrink as needed. With a List, we can add, remove, or access elements easily using indexes. It provides type safety, meaning we can specify the type of objects it can store.
Code Example:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// Creating a List of integers
List<int> numberList = new List<int>();
// Adding elements to the List
numberList.Add(10);
numberList.Add(20);
numberList.Add(30);
// Accessing elements in the List
int firstElement = numberList[0];
int lastElement = numberList[numberList.Count - 1];
// Displaying the elements
Console.WriteLine("First Element: " + firstElement); //Output: 10
Console.WriteLine("Last Element: " + lastElement); //Output: 30
}
}
Code Explanation:
- We include the
System.Collections.Generic
namespace to use the List<T> class. - We create a List of integers named
numberList
using the generic type parameter<int>
. This ensures that the List can only store elements of typeint
. - We add three elements to the List using the
Add()
method: 10, 20, and 30. - To access elements in the List, we can use indexes just like in an array.
Differences between ArrayList and List in C#
Here is a comparison table highlighting the key differences between ArrayList and List in C#:
Sr.No. | ArrayList | List |
---|---|---|
Type Safety: | ArrayList is not type-safe as it can store any type of object. | List is Type-safe as it can store elements of a specific type only. |
Performance: | ArrayList may be slower due to boxing/unboxing. | List performs better as it avoids boxing/unboxing. |
Code Readability: | ArrayList may require explicit type casting. | List provides clear type specification to improved readability. |
.NET Version: | ArrayList was introduced in earlier versions of .NET framework (2.0) | List was introduced in .NET 3.0 |
Flexibility: | ArrayList can store elements of any type. | List can only store elements of the specified type. |
Auto-sizing: | ArrayList automatically resizes when elements are added or removed. | List also automatically resizes with element additions or removals. |
Here are the pros and cons of ArrayList and List in a simplified manner:
ArrayList:
Pros:
- ArrayList can store elements of any type.
- Available in earlier versions of the .NET framework (2.0).
- ArrayList auto-resizes when elements are added or removed.
- The ArrayList collection can store null values and allows duplicate elements. However, it does not support using multidimensional arrays as elements.
Cons:
- ArrayList is not type-safe, which can lead to type-related errors.
- Performance can be slower due to the need for boxing and unboxing.
- ArrayList may require explicit type casting, which can affect code readability.
List:
Pros:
- The List is Type-safe which ensures elements are of a specific type.
- Better performance as no boxing and unboxing operations are required.
- Clear type specification enhances code readability.
- Generic
List<T>
was introduced in.NET 3.0
.
Cons:
- List<T> can only store elements of the specified type.
- List<T> requires additional memory compared to ArrayList for storing object references and type information.
- Not recommended for interacting with older or legacy APIs that use ArrayList.
FAQs
Q: What is the difference between ArrayList and List in C#?
ArrayList can store elements of any type, while List is type-safe and can only store elements of a specific type.
Q: Which collection type is recommended for modern C# development?
List is recommended for modern C# development due to its type safety, improved performance, and code readability.
Q: Can ArrayList and List automatically resize when adding or removing elements?
Yes, Both ArrayList and List can automatically resize when elements are added or removed.
Q: Should I use List or ArrayList?
You should generally use List in your code because it provides type safety, better performance, and improved code readability.
ArrayList is considered legacy and lacks type safety, which can lead to potential errors. The List is the preferred choice for modern C# development unless you need to work with older systems or APIs using ArrayList.
Q: Does ArrayList allow duplicates in C#?
Yes, ArrayList does allow duplicates in C#. That means you can add multiple elements with the same value to an ArrayList. The ArrayList does not enforce uniqueness, so it is possible to have duplicate elements within the collection.
Q: Which is faster, ArrayList or List in C#?
In general, List is faster than ArrayList in C#. This is because List is type-safe and avoids the need for boxing and unboxing operations, which can slow down performance. The List provides better performance when working with collections of a specific type.
References: MSDN-ArrayList in C#
Articles to Check Out:
- C# Collections-(Stack, Queue, Array, dictionary, Hashtable, ArrayList, SortedList)
- C# Hashtable vs Dictionary vs HashSet
- C# Array vs List
- C# Struct vs Class
- C# Abstract class Vs Interface
- Generic Delegates in C# With Examples
- SOLID Design Principles in C#: A Complete Example
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- Constructors in C# with Examples
- Properties In C# with examples
- Multithreading in C#
- C# List Class With Examples
- C# Monitor class in multithreading
- C# Dictionary with Examples
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024