Difference Between Struct and Class in C#:
One key difference between structs and classes is that Structs are value types, whereas classes are reference types.
In the case of structs, they are copied by value, meaning that a complete replica of the data is created when passed or assigned. On the other hand, classes are copied by reference, meaning that only a reference to the original data is passed around rather than a full duplication.
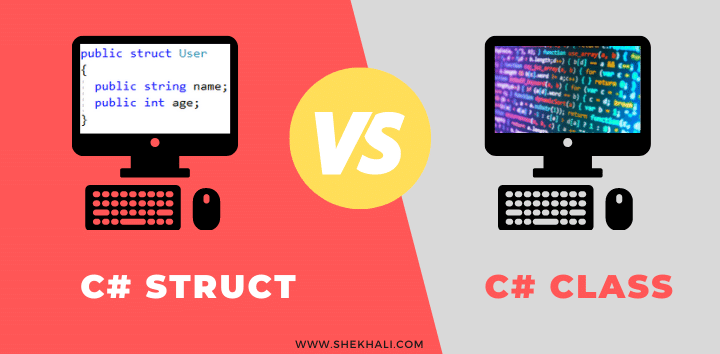
Classes are reference types allocated on the heap and used for creating more complex objects with properties, fields, and methods, and they support inheritance. On the other hand, Structs are value types and are used for small, lightweight data structures.
Table of Contents
- 1 Comparison Table: Struct vs Class in C#
- 2 What is a class?
- 3 What is a C# struct?
- 4 C# Struct vs Class
- 5 Parameter less constructors and field initializers in the struct in C# 10.0
- 6 C# Struct Example
- 7 When to Choose Struct Over Class in C#?
- 8 When to Choose Class Over Struct in C#?
- 9 FAQs
- 9.1 Q: Can structs inherit from other structs or classes?
- 9.2 Q: Is struct faster than class in C#?
- 9.3 Q: Can a struct be null in C#?
- 9.4 Q: What is the difference between a struct and a class in C#?
- 9.5 Q: When should I use a struct instead of a class?
- 9.6 Q: When should I use a class in C#?
- 9.7 Q: Are there any limitations on the size of a struct in C#?
- 9.8 Q: Can structs have methods in C#?
- 10 Conclusion:
Comparison Table: Struct vs Class in C#
Here is the differences between structs and classes in C#:
Parameters | Struct | Class |
---|---|---|
Definition: | A struct is a value type that represents a small and lightweight object. It is typically used for small data structures that do not require inheritance or complex behavior | A class is a reference type that represents a more complex object. It is used to create objects with properties and methods and can support inheritance. |
Memory Allocation: | Structs are usually allocated on the stack and are directly stored where they are declared or as part of another struct or class. | Classes are allocated on the heap and require memory allocation using the “new” keyword. They are accessed through references. |
Copying and Assignment: | A copy of the entire struct is made when a struct is assigned to another struct or passed as a parameter. | When a class is assigned to another class or passed as a parameter, only the reference to the object is copied, not the entire object. |
Default Initialization: | Structs are automatically initialized with default values (e.g., numeric types are set to zero) if not explicitly initialized. | Classes are not automatically initialized, and their fields and properties are set to default values (e.g., null for reference types) if not explicitly initialized. |
Inheritance: | Structs cannot inherit from other structs or classes and cannot be the base of a class. | Classes support single inheritance, meaning they can inherit from one base class, but C# does not support multiple inheritance. |
Performance: | Structs can offer better performance for small data types since they are stack-allocated and don’t require heap allocation and garbage collection. | Classes might have slightly lower performance due to heap allocation and the need for garbage collection, but they offer more flexibility and features. |
It’s important to note that while structs may offer better performance for small data types, classes provide more flexibility and support for inheritance.
The choice between struct and class depends on the specific requirements and characteristics of the objects you need to create for your project.
What is a class?
In C#, a class is a user-defined data type that serves as a blueprint or template for creating objects. It is a reference type stored in the heap that allows you to define fields, methods, properties, and other members.
Why should we use classes?
We use classes in programming because they offer several advantages:
- Organization and Structure: Classes help us organize and structure our code in a logical and modular way. Class groups related data and functions together, making our code more readable and maintainable.
- Code Reusability: Classes allow us to create reusable code. By defining a class once, we can create multiple objects (instances) of that class with similar characteristics and behavior. It saves our time and effort in writing repetitive code.
- Inheritance: Classes support Inheritance, a powerful feature in object-oriented programming. With Inheritance, we can create a hierarchy of classes where a derived class inherits properties and methods from a base class. It promotes code reuse, reduces duplication, and allows for more efficient code maintenance.
- Encapsulation: Classes facilitate encapsulation, which means hiding the internal details and exposing only the necessary information through well-defined interfaces (properties and methods). It enhances code security, prevents unintended modifications, and promotes abstraction.
- Polymorphism: Classes enable polymorphism, which means that objects of different classes can be treated as objects of a common base class. It promotes flexibility, extensibility, and the ability to write generic code that can work with different objects.
Class Syntax:
public class MyClass
{
// Class members go here
}
This is the syntax of a class named MyClass
in C#. You can then add fields, properties, methods, and other members inside the class as needed.
Class Example:
using System;
// Defining a class named 'Person'
public class Person
{
// Fields (attributes) of the class
private string name;
private int age;
// Constructor to initialize the object with values
public Person(string name, int age)
{
this.name = name;
this.age = age;
}
// Property to get or set the 'Name' field
public string Name
{
get { return name; }
set { name = value; }
}
// Property to get or set the 'Age' field
public int Age
{
get { return age; }
set
{
// Adding validation for age to ensure it's non-negative
if (value >= 0)
{
age = value;
}
else
{
Console.WriteLine("Age cannot be negative.");
}
}
}
// Method to display information about the person
public void DisplayInfo()
{
Console.WriteLine($"Name: {name}, Age: {age}");
}
}
class Program
{
static void Main()
{
// Creating an instance (object) of the 'Person' class
Person person1 = new Person("Shekh Ali", 25);
// Using properties to get or set values
Console.WriteLine($"Original Age: {person1.Age}");
person1.Age = 26;
Console.WriteLine($"Updated Age: {person1.Age}");
// Calling a method to display information
person1.DisplayInfo();
}
}
Output:
Original Age: 25
Updated Age: 26
Name: Shekh Ali, Age: 26
What is a C# struct?
In C#, a struct is a value type that represents a small and lightweight object. It is used for creating simple data structures that hold related data together, typically without the need for complex behavior or inheritance.
Structs are similar to classes because they can also contain fields, methods, and properties, but they have several key differences.
The main difference is that structs are value types, meaning they are stored directly in stack memory and don’t require garbage collection. It can make them faster and more efficient for storing small data.
Struct Syntax:
We can create a structure using the struct
keyword followed by the structure name.
struct MyStruct
{
// Fields, properties, and methods go here
}
C# Struct vs Class
The following is the list of differences between struct and class in C#.
- In C#, Class is a reference type, whereas Struct is a value type.
- A class object is allocated on the heap memory, whereas Struct type variables are allocated on the stack memory.
- All struct types implicitly inherit from the class
System.ValueType
, whereas every Class is implicitly a subclass of theSystem.Object
class. - Value types are immediately destroyed when the scope is lost, whereas the garbage collector only destroys reference-type objects. As a result, value type allocations and deallocations are generally less expensive than reference types.
- A class object is passed by reference to a method, whereas a structure is passed as a value rather than a reference to the method (except ref and out parameter variables).
- Since Struct is not a reference type, Boxing and unboxing operations convert between a struct type and an object in C#.
- In C#, Destructors are not allowed in structures, but they are allowed in classes.
- Parameterless constructors are not allowed in the structure. However, parameterized and static constructors are allowed. A class, on the other hand, can have any constructor.
- Structs can be instantiated with or without the new keyword, whereas classes require the new keyword to be instantiated.
- A class can inherit from another class, but a struct cannot inherit from another struct or Class. However, a structure type can implement interfaces.
- The structure cannot be a base class and is implicitly sealed, while the Class can be a base class.
- By default, members of a class are private, whereas members of a structure are public.
- The data member of a class can be declared as protected, while the data member of a struct cannot.
- A struct type variable directly contains the struct data, whereas a class type variable contains a reference to the data.
- Instance fields cannot have initializers in a struct, which means that you cannot declare a member of the structure like the below image:
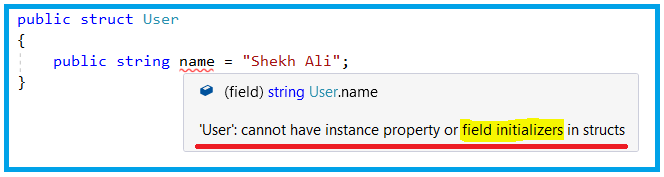
Parameter less constructors and field initializers in the struct in C# 10.0
Note: Starting with
C# 10.0
, you can declare a (default) parameter less constructor and field Initializers in a struct type. You are now allowed to initialize an instance field or property during declaration time with C# 10.
Example:
using System;
namespace StructInCSharp
{
// Example: field Iinitializers in a struct in C#10
public readonly struct Employee
{
public double Salary { get; init; }
// Initialize an instance field or property at its declaration
public string Name { get; init; } = "Shekh Ali";
public Employee(double salary)
{
Salary = salary;
}
public override string ToString() => $" Salary= $ {Salary} Name=({Name})";
}
public static void Main(string[] args)
{
Employee employee = new Employee(5000);
Console.WriteLine(employee); // output: Salary= $ 5000 Name=(Shekh Ali)
}
}
C# Struct Example
A structure can be created by using the struct keyword.
In C#, a structure-type variable can’t be null so you must initialize a declared variable before it can be used.
The following is an example of how to create a structure in C#.
using System;
// Defining a struct named 'Point'
public struct Point
{
// Fields (attributes) of the struct
public int X;
public int Y;
// Constructor to initialize the struct with values
public Point(int x, int y)
{
X = x;
Y = y;
}
// Method to display information about the point
public void DisplayInfo()
{
Console.WriteLine($"X: {X}, Y: {Y}");
}
}
class Program
{
static void Main()
{
// Creating an instance (object) of the 'Point' struct
Point point1 = new Point(10, 20);
// Accessing and modifying struct fields directly
Console.WriteLine($"Original X: {point1.X}");
point1.X = 15;
Console.WriteLine($"Updated X: {point1.X}");
// Calling a method to display information
point1.DisplayInfo();
}
}
Output:
Original X: 10
Updated X: 15
X: 15, Y: 20
When to Choose Struct Over Class in C#?
Choosing between Structs and Classes in C# can be difficult, and it ultimately depends on the specific requirements of your code. However, here are five reasons why you might choose to use a Struct over a Class:
- Avoiding Garbage Collection Overhead: Structs are value types allocated on the stack, meaning they do not require garbage collection. That can result in faster performance and reduced memory usage, especially for small data types.
- Faster Performance for Small Data Types: Because Structs are allocated on the stack and do not require memory management overhead, they can be created and copied more quickly than Classes. That makes them ideal for small data types that do not need to be modified frequently.
- Creation of Immutable Types: Structs are often used for immutable types, objects that cannot be changed once created. Because Structs are value types, any changes to the Struct result in creating a new Struct, which can help ensure immutability.
- Simpler Syntax: Structs have a simpler syntax than Classes, making them easier to use and understand for developers new to C#.
- Compatibility with Other Languages: Structs are compatible with other languages that use similar value types, such as C and C++. That can make it easier to work with code written in other languages or integrate C# code with existing systems.
When to Choose Class Over Struct in C#?
While Structs have their advantages, there are also situations where a Class might be a better choice. Here are five reasons why you might choose to use a Class over a Struct in C#:
- Inheritance and Polymorphism: Classes support inheritance and polymorphism, which means that you can create complex object hierarchies and use them in a flexible and extensible way. Structs, however, do not support inheritance and cannot be used similarly.
- Ability to Create Complex Objects: Classes are reference types that can create complex objects with properties, methods, and events. That makes them ideal for situations where you need to create objects with a lot of functionality and state.
- Better Memory Management for Large Data Types: While Structs are ideal for small data types, Classes are better suited to large data types that require more memory management. Classes are allocated on the heap and can be garbage collected when they are no longer needed, which can help reduce memory usage and prevent memory leaks.
- Dynamic Allocation: Classes can be dynamically allocated at runtime, meaning you can create objects on the fly and use them flexibly. Structs, however, are allocated on the stack and must be explicitly created and destroyed.
- When Working with Libraries or Frameworks: Many libraries and frameworks are designed to work with Classes, so if you want to take advantage of these tools, you will need to use Classes in your code. Additionally, if you are working in a team environment, using Classes can make it easier for other developers to understand and work with your code.
Reference MSDN: Class and struct differences
FAQs
Q: Can structs inherit from other structs or classes?
No, in C#, structs cannot inherit from other structs or classes. They support only single inheritance and do not allow for the creation of a struct derived from another struct or class.
Q: Is struct faster than class in C#?
Structs are faster than classes for small data types because they avoid the overhead of garbage collection.
However, classes might be faster for larger data types because they can take advantage of the CPU cache.
Q: Can a struct be null in C#?
No, a struct cannot be null in C#. Structs are value types allocated on the stack, so they cannot be assigned a null value. However, you can use nullable value types to represent the absence of a value for a struct variable.
Q: What is the difference between a struct and a class in C#?
Structs are value types allocated on the stack, while classes are reference types allocated on the heap. Structs are typically used for small data types, while classes are used for more complex object types.
Q: When should I use a struct instead of a class?
Use a struct when you have a small, lightweight object that represents a simple data structure (like a Point or Color). Structs are good for scenarios where copying the entire data is efficient, and you don’t need the features of reference types, such as inheritance or nullable types.
Q: When should I use a class in C#?
Classes are best used for more complex object types that require inheritance and polymorphism. They are also useful for creating objects with lots of functionality and managing memory for larger data types.
Q: Are there any limitations on the size of a struct in C#?
Yes, there are size limitations for structs. In C#, the size of a struct must be less than or equal to 16 bytes. If it exceeds this limit, it’s advisable to use a class instead.
Q: Can structs have methods in C#?
Yes, structs can have methods. While they can’t have explicit parameterless constructors (except the default one), they can have parameterized constructors and methods.
Conclusion:
In summary, Structs and Classes have different advantages and disadvantages, and the choice between the two depends on the specific requirements of your code.
Structs are ideal for small, simple data types and immutable types, while Classes are better for complex objects and inheritance.
When making a decision, consider the memory usage and performance requirements of your code, as well as the specific needs of your project. Choosing the right data type ensures that your code is efficient, easy to maintain, and performs well.
Recommended Articles:
- C# Struct with [Examples]
- Understanding Class vs Struct vs Record in C# with examples
- Difference Between if-else and switch: A Side-by-Side Comparison of If-Else and Switch Case
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- C# Struct vs Class |Top 15+ Differences Between C# Struct and Class
- 10 Difference between interface and abstract class In C#
- Local vs Global Variables
- Generic Delegates in C# With Examples: Func, Action, and Predicate delegates
- Constructors in C#: A Comprehensive Guide with Code Examples
- Top 5 Difference between Call by Value and Call by Reference
- C# stack vs heap
- C# switch Statement (With Examples)
- Readonly vs const in C#
- C# List Class |Top 12 Amazing Generic List Methods
- Exception Handling in C#| Use of try, catch, and finally block
- C# Enum
- C# extension methods with examples
- Properties In C# with examples
- IEnumerable Interface in C# with examples
- Constructors in C# with Examples
- C# Dictionary
Let others know about this post by sharing it and leaving your thoughts in the comments section.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025