C# Queue is a linear data structure that follows the First-In-First-Out (FIFO) principle. In other words, the first element added to the queue will be the first one to be removed. This makes queues useful for storing data that needs to be processed in a specific order. The queue is the opposite of the Stack<T> collection.
In C#, the
Queue
class is a generic collection that implements theIEnumerable
interface and provides a variety of methods for adding, accessing, and removing elements in the queue.
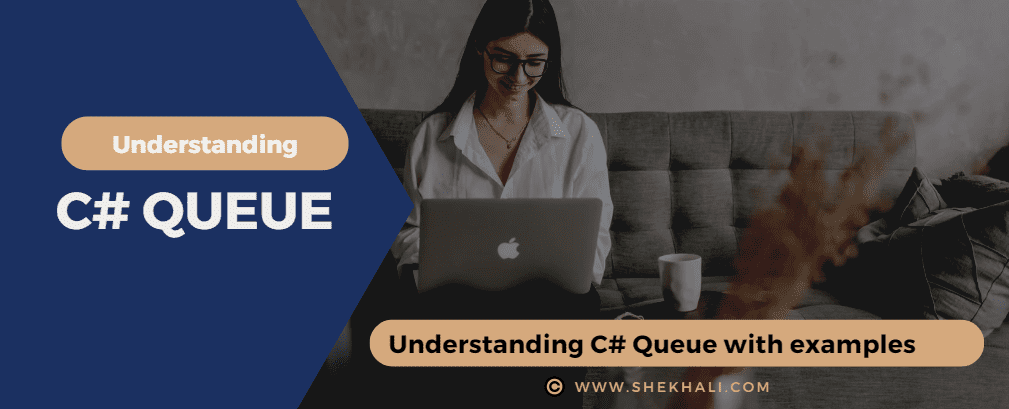
Table of Contents
Adding elements to a C# Queue
You can use the Enqueue
method to add an element to a C# queue. This method is used to add the element to the end of the queue, making it the last element in the queue.
Example:
Following is an example of adding elements to a queue.
Queue<int> myQueue = new Queue<int>();
myQueue.Enqueue(1);
myQueue.Enqueue(2);
myQueue.Enqueue(3);
myQueue.Enqueue(4);
Removing elements from a C# Queue
You can use the Dequeue
method to remove an element from a C# queue. This method removes the first element in the queue (the one added first) and returns it.
Example:
Following is an example of removing elements from a queue.
// Removing first element from a Queue.
int firstElement = queue.Dequeue();
Accessing elements in a C# Queue
You can use the Peek
method to access the elements in a C# queue. This method returns the first element in the queue without removing it.
Example:
Following is an example of accessing elements from a queue.
// Accessing first element from a queue.
int firstElement = queue.Peek();
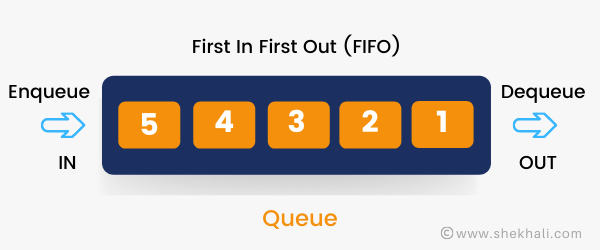
C# Queue Properties and Methods
The following are the few commonly used properties and methods of the C# Queue class.
Property/Method | Description |
---|---|
Count | The count property returns the number of elements in the queue. |
Enqueue(T item) | Adds an element to the end of the queue. |
Dequeue() | Removes and returns the first element in the queue. |
Peek() | Returns the first element in the queue without removing it. |
Clear() | Removes all elements from the queue. |
Contains(T item) | Determines whether the queue contains a specific element. |
ToArray() | Copies the elements of the queue to a new array. |
TrimExcess() | Sets the capacity to the actual number of elements in the queue. |
Characteristics of C# Queue Class:
The C# Queue
class has the following characteristics:
Queue<T>
is a generic class, meaning that it can store elements of any data type. You can specify the data type using angle brackets like this:Queue<int>
orQueue<string>
.- It is a reference type, meaning that it is stored on the heap and accessed via a reference.
- It is available in
System.Collection.Generic
namespace. - It is thread-safe, meaning that multiple threads can access and modify the queue without causing race conditions or other synchronization issues.
- C# Queue implements the
IEnumerable
interface, which means it can be used with a foreach loop and LINQ queries. - Queue provides methods for adding elements to the queue (
Enqueue
), removing elements from the queue (Dequeue
), and accessing the first element in the queue (Peek
). - It provides properties for checking the number of elements in the queue (
Count
). - It does not allow null elements to be added to the queue.
C# Queue Example
Following is the example of Queue in C#.
using System;
using System.Collections.Generic;
namespace QueueExample
{
class Program
{
static void Main(string[] args)
{
Queue<string> queue = new Queue<string>();
// Adding elements to the queue
queue.Enqueue("Apple");
queue.Enqueue("Banana");
queue.Enqueue("Guava");
queue.Enqueue("Orange");
// Check the number of elements in the queue
int count = queue.Count;
Console.WriteLine($" There are {count} elements in the queue.");
// Print all the elements from the queue
int index = 0;
foreach(var element in queue)
{
Console.WriteLine($" Element at index {index}: {element}");
index++;
}
// Remove and return the first element in the queue
string firstElement = queue.Dequeue();
Console.WriteLine($" Removing first element: {firstElement}");
// Clear the queue
queue.Clear();
// Check if the queue contains a specific element
bool containsApple = queue.Contains("Apple");
Console.WriteLine($" Does the queue contain apple? {containsApple}");
// Copy the elements of the queue to a new array
string[] array = queue.ToArray();
Console.ReadKey();
}
}
}
Following is the output of the above C# code:
There are 4 elements in the queue.
Element at index 0: Apple
Element at index 1: Banana
Element at index 2: Guava
Element at index 3: Orange
Removing first element: Apple
Does the queue contain apple? False
Conclusion
In summary, the C# Queue<T>
class is a generic class that provides a convenient way to store and process data in a FIFO manner. By using the Enqueue
, Dequeue
, and Peek
methods, you can easily add, remove, and access elements in the queue.
References: MSDN-Queue<T> Class
FAQ
Following are some frequently asked questions about C# queues:
Q: What is a queue in C#?
A: A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle. It is used to store data that needs to be processed in a specific order. The Queue
class is a generic collection that provides methods for adding, removing, and accessing elements in the queue.
Q: How do I create a queue in C#?
A: you can use the Queue
class To create a queue in C# and specify the data type using angle brackets.
you can use the following syntax:Queue<T> queue = new Queue<T>();
Where T
is the type of objects that you want to store in the queue.
Example:Queue<string> queue = new Queue<string>();
// creating a queue of strings
Q: How do I add elements to a C# Queue?
A: you can use the Enqueue
method to add an element to a queue in C#. This method adds the element to the end of the queue. For example:queue.Enqueue("Apple");
Q: How can you remove elements from a queue in C#?
you can use the Dequeue
method to remove an element from a queue in C#.
For example:string firstElement = queue.Dequeue();
Q: How do I check if a Queue is empty in C#?
you can use the Count
property or the Any
method of the queue class to check if a Queue is empty or not.
For Example:if (queue.Count == 0){// queue is empty}
// Using LINQ Any method.if (!queue.Any()){ // queue is empty}
If you enjoyed this post, please share it with your friends or leave a comment below.
Related Articles:
- C# Hashtable vs Dictionary: When should you use a Hashtable or a Dictionary?
- C# Stack Class With Push And Pop Examples
- Understanding C# Queue Class With Examples
- C# Struct: Understanding Structure in C# With Examples
- Generic Delegates in C# With Examples
- C# Hashtable vs Dictionary vs HashSet
- IEnumerable Interface in C# with examples
- Constructors in C# with Examples
- Properties In C# with examples
- 10 Difference between interface and abstract class In C#
- Value Type and Reference Type in C#
- C# List Class |Top 12 Amazing Generic List Methods
- C# Struct vs Class
- C# Static class
- Params keyword in C#
- Multithreading in C#
- C# Polymorphism: Different types of polymorphism in C# with examples
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024