In this article, we will walk through writing a program to find the maximum and minimum number in an array in C#, C++, Python, and Java.
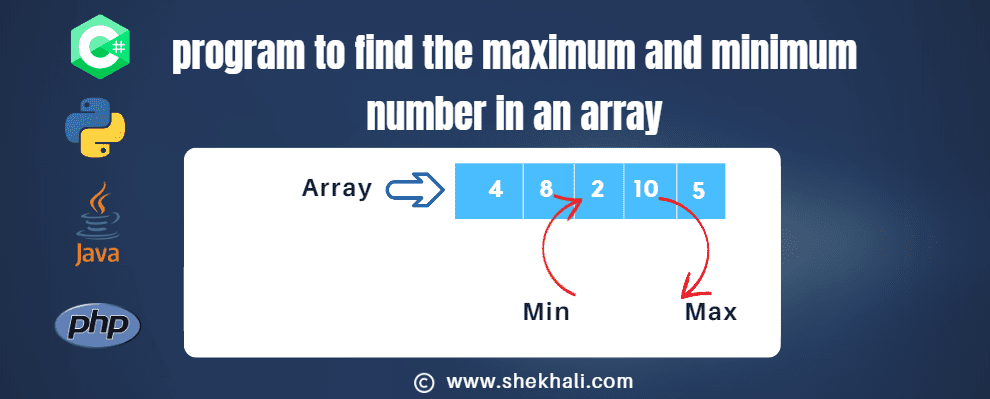
Table of Contents
C#
Approach 1: Simple Linear Search
Below is the C# program to find the maximum and minimum number in an array.
using System;
class Program
{
static void FindMinMax(int[] arr)
{
int min = arr[0];
int max = arr[0];
foreach (int num in arr)
{
if (num < min)
min = num;
if (num > max)
max = num;
}
Console.WriteLine($"Minimum number in the array is: {min}");
Console.WriteLine($"Maximum number in the array is: {max}");
}
static void Main()
{
int[] numbers = { 4, 8, 2, 10, 5 };
FindMinMax(numbers);
}
}
Output:
Minimum number in the array is: 2
Maximum number in the array is: 10
Code Explanation:
- We initialize the
min
andmax
variables with the first element of the array. - We then Iterate through the array and update
min
andmax
when a smaller or larger element in the array is found. - In the last, we are printing the results.
Approach 2: Using LINQ (Language-Integrated Query)
In the code below, we are using LINQ’s Min()
and Max()
functions to find the minimum and maximum values from an array.
using System;
using System.Linq;
class Program
{
static void Main()
{
int[] numbers = { 4, 8, 2, 10, 5 };
int min = numbers.Min();
int max = numbers.Max();
Console.WriteLine($"Minimum number in the array is: {min}");
Console.WriteLine($"Maximum number in the array is: {max}");
}
}
Output:
Minimum number in the array is: 2
Maximum number in the array is: 10
C++
Approach: Iterative Comparison
C++ program to find the maximum and minimum number in an array.
#include <iostream>
using namespace std;
void findMinMax(int arr[], int size) {
int min = arr[0];
int max = arr[0];
for (int i = 1; i < size; ++i) {
if (arr[i] < min)
min = arr[i];
if (arr[i] > max)
max = arr[i];
}
cout << "Minimum: " << min << endl;
cout << "Maximum: " << max << endl;
}
int main() {
int numbers[] = {4, 8, 2, 10, 5};
int size = sizeof(numbers) / sizeof(numbers[0]);
findMinMax(numbers, size);
return 0;
}
Output:
Minimum: 2
Maximum: 10
Code Explanation:
- Similar to the C# approach, we use a simple iterative for loop to find the minimum and maximum values from an array in C++.
Python
Approach: Built-in Functions
Python program to find the maximum and minimum number in an array.
numbers = [4, 8, 2, 10, 5]
minimum = min(numbers)
maximum = max(numbers)
print(f"Minimum: {minimum}")
print(f"Maximum: {maximum}")
Output:
Minimum: 2
Maximum: 10
In the code above, We are using Python’s built-in functions min()
and max()
to get the Minimum & Maximum value from an Array.
Java
Approach: Traditional Iteration
Below is the Java program to find the maximum and minimum number in an array.
public class MinMaxFinder {
public static void findMinMax(int[] arr) {
int min = arr[0];
int max = arr[0];
for (int num : arr) {
if (num < min)
min = num;
if (num > max)
max = num;
}
System.out.println("Minimum: " + min);
System.out.println("Maximum: " + max);
}
public static void main(String[] args) {
int[] numbers = {4, 8, 2, 10, 5};
findMinMax(numbers);
}
}
Output:
Minimum: 2
Maximum: 10
In the above code example, we are using the Traditional iteration approach to find the minimum and maximum values from an Array in Java.
Conclusion
In this article, we’ve explored various approaches to finding the maximum and minimum numbers in an array using C#, C++, Python, and Java. Whether you prefer a simple linear search or leveraging built-in functions, understanding these basics is crucial for any programmer.
Happy coding.
Recommended Articles:
- Program to copy all elements of an array into another array
- C# Program to Convert Decimal to Binary with Examples
- C# Program to Convert Binary to Decimal with Examples
- C# Program to Check Armstrong Number
- C# Program to Convert Hexadecimal to Decimal
- How to remove duplicate characters from a String in C#
- C# program to count the occurrences of each character in a String
- C# Program to Print Multiplication Table of a Given Number
- Program to print prime numbers from 1 to N
- C# Program to Check if a Given Number is Even or Odd
- Program to copy all elements of an array into another array
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- C# Programs asked in Interviews
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025