As a C# developer, your code must have used string formatting. It is a technique to represent strings with placeholders that you can replace with values at runtime.
In C#, there are two ways to format strings: the String.Format() method and the C# string interpolation.
This article will discuss C# string interpolation, its syntax, examples, and performance. We will also compare it with the String.Format()
method and explore its new features in C# 10.
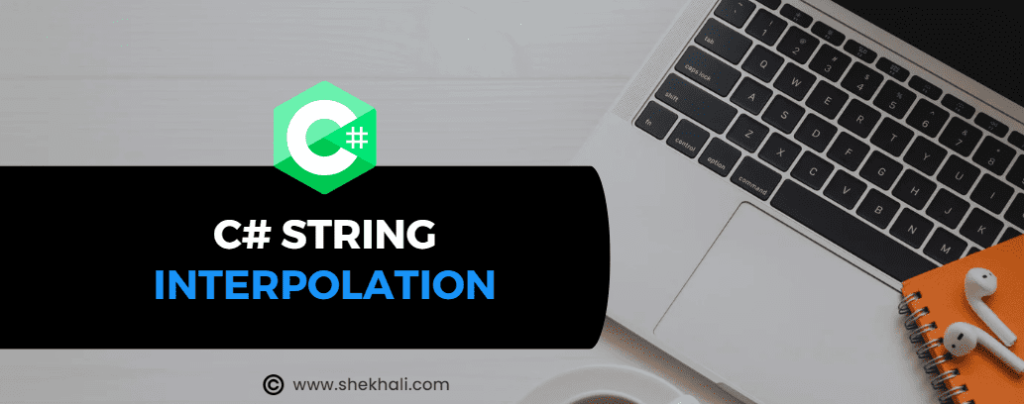
Table of Contents
- 1 C# String Interpolation
- 2 Syntax:
- 3 Example:
- 4 C# String Interpolation Example
- 5 Why use String Interpolation instead of String.Format() method?
- 6 C# String Interpolation with Escaped Characters
- 7 C# String Interpolation with Conditional Access Operator
- 8 C# String Interpolation with Interpolated Verbatim Strings
- 9 C# String Interpolation with String Concatenation
- 10 C# String Interpolation with Ternary Operator
- 11 C# String Interpolation Performance compared to String.Format
- 12 FAQs:
- 12.1 Q: What is C# string interpolation?
- 12.2 Q: How do I use C# string interpolation?
- 12.3 Q: How does C# string interpolation compare to String.Format() method?
- 12.4 Q: Can you use escaped characters in C# string interpolation?
- 12.5 Q: Can you use C# string interpolation with verbatim strings?
- 12.6 Q: Is C# string interpolation faster than String.Format() method?
- 12.7 Q: Can I concatenate strings using C# string interpolation?
- 13 Conclusion:
C# String Interpolation
C# string interpolation is a feature that allows you to embed expressions into a string literal. It is a concise way of formatting strings that replaces the placeholders with their corresponding values at runtime.
The syntax of C# string interpolation is simple and easy to read. You can use curly braces {} to embed expressions inside a string literal.
Syntax:
string interpolatedString = $"Text {expression}";
Here, the $ sign before the opening quotation mark indicates that it is an interpolated string.
The expression inside the curly braces {} can be any valid C# expression that returns a value. The expression can also contain method calls, variables, and operators.
Example:
using System;
class StringInterpolation
{
static void Main()
{
string firstName = "Shekh";
string lastName = "Ali";
int age = 29;
string message = $"My name is {firstName} {lastName} and I am {age} years old.";
Console.WriteLine(message);
Console.ReadKey();
// Output: My name is Shekh Ali and I am 29 years old.
}
}
C# String Interpolation Example
Let’s see another example of C# string interpolation that uses an object’s properties.
using System;
class StringInterpolation
{
class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
static void Main()
{
Person person = new Person { Name = "Amit", Age = 25 };
string message = $"My name is {person.Name} and I am {person.Age} years old.";
Console.WriteLine(message);
Console.ReadKey();
}
}
Output:
My name is Amit and I am 25 years old.
In this example, we have created a Person object and used its properties inside the string interpolation expression. The values of the properties are replaced with their corresponding placeholders at runtime.
Why use String Interpolation instead of String.Format() method?
The String.Format()
method is an older way of formatting strings in C#. It uses positional placeholders to replace values at runtime. However, C# string interpolation is a more modern and concise way of formatting strings. Here are some reasons to use C# string interpolation over String.Format()
method.
- Concise syntax: C# string interpolation uses a simple and easy-to-read syntax that eliminates the need for complex positional placeholders.
- Type safety: C# string interpolation expressions are type-checked at compile time, which makes it less error-prone than the
String.Format()
method. - Performance: C# string interpolation is faster than
String.Format()
method because it generates less overhead and fewer allocations.
C# String Interpolation with Escaped Characters
To add special characters to the output string, you can use escaped characters like \n (newline) and \t (tab) inside C# string interpolation expressions.
Here is an example:
string name = "John";
string message = $"Hello,\n{name}!";
Output:
Hello,
John!
In this example, we have used the \n
character to add a newline character after the word “Hello”.
C# String Interpolation with Conditional Access Operator
You can use the conditional access operator ?.
inside C# string interpolation expressions. The conditional access operator allows you to check if an object is null before accessing its properties or methods.
Here is an example:
using System;
class StringInterpolation
{
class Person
{
public string Name { get; set; }
}
static void Main()
{
Person person = null;
string message = $"My name is {(person?.Name ?? "Unknown")}";
Console.WriteLine(message);
Console.ReadKey();
}
}
Output:
My name is Unknown
In this example, we have used the ?.
operator to check if the person object is null before accessing its Name property. If the person object is null, the string “Unknown” is used instead.
C# String Interpolation with Interpolated Verbatim Strings
C# 9 introduces interpolated verbatim strings, which combine the benefits of interpolated and verbatim strings. Interpolated verbatim strings start with the $ character followed by the @ character, like @$"..."$
. You can use expressions inside the string using curly braces {}.
Here is an example:
string name = "Shekh";
string message = @$"Hello there! My name is {name}.
It's nice to meet you.";
Output:
Hello there! My name is Shekh.
It's nice to meet you.
In this example, we have used the @$”…”$ syntax to create an interpolated verbatim string and embedded an expression inside it using curly braces {}. The verbatim string allows us to include line breaks and white spaces without escaping them.
C# String Interpolation with String Concatenation
You can also concatenate multiple strings using C# string interpolation. Simply add the + operator between the strings.
Here is an example:
string name = "Johnny";
int age = 30;
string message = $"My name is " + name + $" and I am {age} years old.";
Output:
My name is Johnny and I am 29 years old.
In this example, we have used the + operator to concatenate the string “My name is ” with the interpolated string $”, and I am {age} years old.”.
C# String Interpolation with Ternary Operator
You can also use the ternary operator ? :
inside C# string interpolation expressions. The ternary operator allows you to create conditional expressions that evaluate different values based on a condition.
Here is an example:
using System;
class StringInterpolationWithTernaryOperator
{
class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
static void Main()
{
Person person = new Person { Name="Deepak", Age= 17};
string message = $"My name is {person.Name} and I am {(person.Age < 18 ? "not " : "")}an adult.";
Console.WriteLine(message);
Console.ReadKey();
}
}
Output:
My name is Deepak and I am not an adult.
C# String Interpolation Performance compared to String.Format
C# string interpolation is faster than the String.Format() method because it generates less overhead and fewer allocations. Let’s compare the performance of C# string interpolation with the String.Format() method.
Here is an example:
using System;
using System.Diagnostics;
class StringInterpolation
{
static void Main()
{
string name = "Raj";
int age = 22;
Stopwatch stopwatch = new Stopwatch();
// String.Format() method
stopwatch.Start();
string message2 = String.Format("My name is {0} and I am {1} years old.", name, age);
stopwatch.Stop();
Console.WriteLine($"String.Format() method time: {stopwatch.ElapsedTicks}");
stopwatch.Reset();
// C# string interpolation
stopwatch.Start();
string message1 = $"My name is {name} and I am {age} years old.";
stopwatch.Stop();
Console.WriteLine($"C# string interpolation time: {stopwatch.ElapsedTicks}");
Console.ReadKey();
}
}
Output:
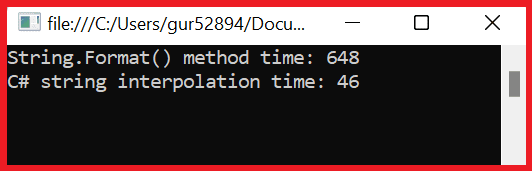
As you can see in the above example, the C# string interpolation is significantly faster than the String.Format()
method.
Reference: MSDN – C# String Interpolation
FAQs:
Q: What is C# string interpolation?
C# string interpolation is a feature that allows you to embed expressions into string literals, making it easier to create dynamic strings.
Q: How do I use C# string interpolation?
To use C# string interpolation, you need to start a string literal with the $ character and then enclose expressions inside curly braces {}.
Q: How does C# string interpolation compare to String.Format() method?
C# string interpolation is a more concise and readable way of formatting strings than the String.Format() method. It also supports more features, such as using expressions and embedded statements.
Q: Can you use escaped characters in C# string interpolation?
Yes, you can use escaped characters such as \n (newline) and \t (tab) inside C# string interpolation expressions to add special characters to the output string.
Q: Can you use C# string interpolation with verbatim strings?
Yes, C# 9 introduces interpolated verbatim strings, which allow you to combine the benefits of interpolated strings and verbatim strings.
Q: Is C# string interpolation faster than String.Format() method?
Yes, C# string interpolation is generally faster than the String.Format()
method, as it involves less overhead and fewer method calls.
Q: Can I concatenate strings using C# string interpolation?
Yes, you can concatenate multiple strings using C# string interpolation. Simply add the + operator between the strings.
Conclusion:
In conclusion, C# string interpolation is a powerful feature that allows you to create dynamic and readable strings by embedding expressions directly into string literals.
It’s a more concise and convenient way of formatting strings than traditional methods like String.Format()
.
In this article, we have covered the syntax of C# string interpolation and provided several examples to demonstrate its various capabilities, including using expressions, embedded statements, and formatting options. We’ve also covered advanced features like interpolated verbatim strings, newlines in interpolation expressions etc.
This article has given you a solid understanding of C# string interpolation and how to use it effectively in your C# programming projects.
You might also like:
- C# String Vs StringBuilder
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- 10 Difference between interface and abstract class In C#
- C# Queue with examples
- Web API vs web service: Top 10+ Differences between web API and web service in C#
- C# Polymorphism: Different types of polymorphism in C# with examples
- C# Stack
- Understanding the Difference between == and Equals() Method in C#
- Difference between SortedList and SortedDictionary in C#
- C# Hashtable vs Dictionary vs HashSet
- C# stack vs heap
- Format String in C#: A Comprehensive Guide to Understand String Formatting in C#
- Fibonacci sequence: Fibonacci series in C# (with examples)
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- Advantages and Disadvantages of Non-Generic Collection in C#
- Difference between readonly and const keywords in C#
Let others know about this post by sharing it and leaving your thoughts in the comments section.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025