Introduction:Â In software development, design patterns play an important role in creating reusable and maintainable code. One such pattern is the Chain of Responsibility design pattern, which promotes loose coupling and flexibility in handling requests.
In this article, we will explore the Chain of Responsibility design pattern in the context of C#, providing clear explanations and practical examples to help beginners grasp its concepts effectively.
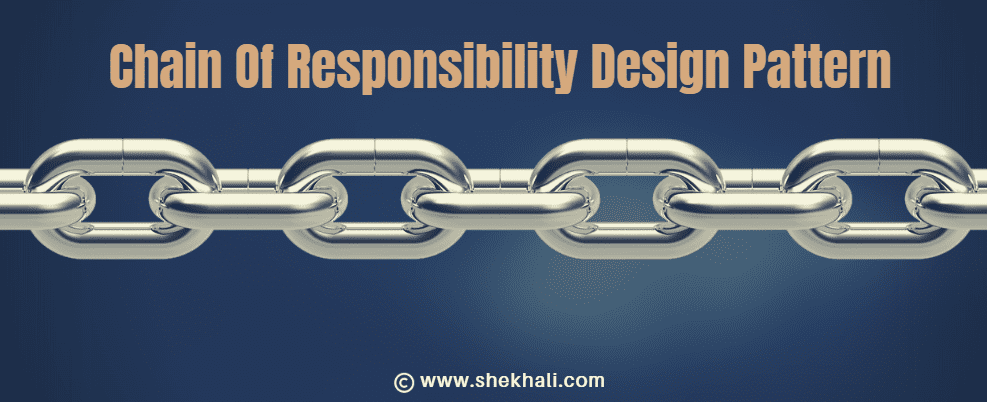
Table of Contents
- 1 What is the Chain of Responsibility Design Pattern?
- 2 Key Participants in the Chain of Responsibility Pattern:
- 3 UML Diagram-Chain Of Responsibility Pattern
- 4 Implementing the Chain of Responsibility Design Pattern in C#:
- 5 Real-time example of using the Chain of Responsibility pattern
- 6 Benefits and Drawbacks of the Chain of Responsibility Pattern:
- 7 Conclusion:
- 8 FAQs:
- 8.1 Q: What is the Chain of Responsibility design pattern?Â
- 8.2 Q: When should I use the Chain of Responsibility pattern?
- 8.3 Q: How does the Chain of Responsibility pattern work in C#?Â
- 8.4 Q: Can you modify the chain dynamically?Â
- 8.5 Q: What are the benefits of using the Chain of Responsibility pattern?Â
- 8.6 Q: Are there any drawbacks when using the Chain of Responsibility pattern?Â
- 8.7 Q: Can the Chain of Responsibility pattern be combined with other design patterns?Â
- 8.8 Related
What is the Chain of Responsibility Design Pattern?
The Chain of Responsibility design pattern is a behavioral pattern that allows an object to pass a request along a chain of potential handlers until the request is handled or reaches the end of the chain.Â
This pattern decouples the sender of a request from its receivers, giving multiple objects a chance to handle the request independently.
Key Participants in the Chain of Responsibility Pattern:
- Client : The client sends the request to the Chain of Receivers.
- Request:Â The request represents the information or action being passed along the chain. It encapsulates the request details and is passed from one handler to another until it is appropriately handled.
- Handler: The handler is an interface or an abstract class that defines the common methods for handling requests. It contains a reference to the next handler in the chain and provides a method to set or modify this reference.
- Concrete Handlers: Concrete handlers are the actual objects responsible for processing requests. Each concrete handler decides whether to handle the request or pass it to the next handler in the chain.
UML Diagram-Chain Of Responsibility Pattern
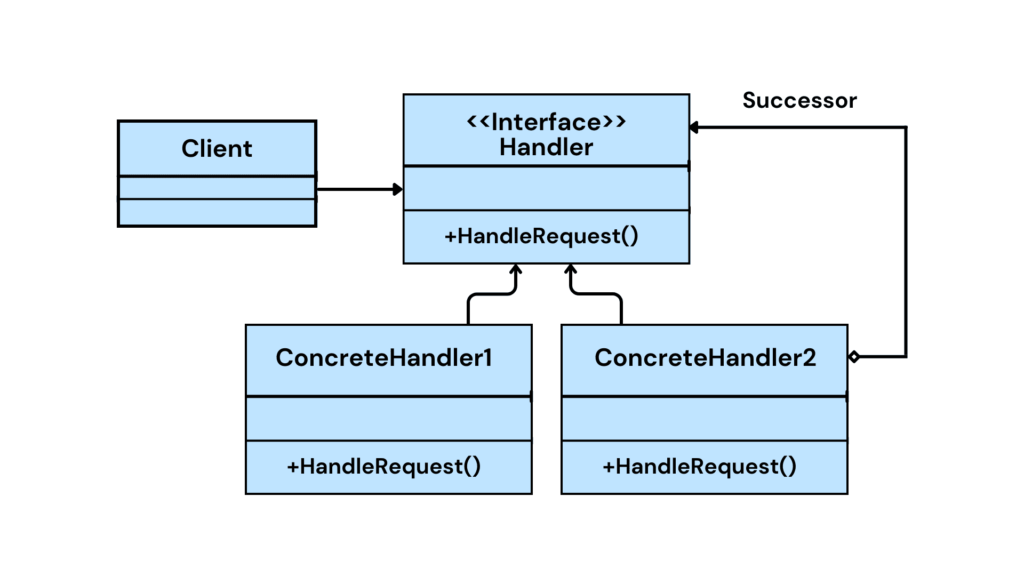
Implementing the Chain of Responsibility Design Pattern in C#:
Let’s dive into the implementation details of the Chain of Responsibility pattern in C#
Here is a code example that demonstrates the Chain of Responsibility design pattern:
using System;
// Request class
public class Request
{
public string Content { get; set; }
public Request(string content)
{
Content = content;
}
}
// Handler interface
public interface IHandler
{
void SetNext(IHandler handler);
void HandleRequest(Request request);
}
// ConcreteHandlerA class
public class ConcreteHandlerA : IHandler
{
private IHandler _nextHandler;
public void SetNext(IHandler handler)
{
_nextHandler = handler;
}
public void HandleRequest(Request request)
{
if (request.Content.Contains("A"))
{
Console.WriteLine($"ConcreteHandlerA: Handling the request:{request.Content}");
}
else if (_nextHandler != null)
{
Console.WriteLine($"ConcreteHandlerA: Passing the request '{request.Content}' to the next handler.");
_nextHandler.HandleRequest(request);
}
else
{
Console.WriteLine($"ConcreteHandlerA: End of the chain. Request '{request.Content}' cannot be handled.");
}
}
}
// ConcreteHandlerB class
public class ConcreteHandlerB : IHandler
{
private IHandler _nextHandler;
public void SetNext(IHandler handler)
{
_nextHandler = handler;
}
public void HandleRequest(Request request)
{
if (request.Content.Contains("B"))
{
Console.WriteLine($"ConcreteHandlerB: Handling the request:{request.Content}");
}
else if (_nextHandler != null)
{
Console.WriteLine($"ConcreteHandlerB: Passing the request '{request.Content}' to the next handler.");
_nextHandler.HandleRequest(request);
}
else
{
Console.WriteLine($"ConcreteHandlerB: End of the chain. Request '{request.Content}' cannot be handled.");
}
}
}
// ConcreteHandlerC class
public class ConcreteHandlerC : IHandler
{
private IHandler _nextHandler;
public void SetNext(IHandler handler)
{
_nextHandler = handler;
}
public void HandleRequest(Request request)
{
if (request.Content.Contains("C"))
{
Console.WriteLine($"ConcreteHandlerC: Handling the request :{request.Content}");
}
else if (_nextHandler != null)
{
Console.WriteLine($"ConcreteHandlerC: Passing the request '{request.Content}' to the next handler.");
_nextHandler.HandleRequest(request);
}
else
{
Console.WriteLine($"ConcreteHandlerC: End of the chain. Request '{request.Content}' cannot be handled.");
}
}
}
// Client code
public class Client
{
public static void Main()
{
// Creating instances of the concrete handlers
var handlerA = new ConcreteHandlerA();
var handlerB = new ConcreteHandlerB();
var handlerC = new ConcreteHandlerC();
// Building the chain
handlerA.SetNext(handlerB);
handlerB.SetNext(handlerC);
// Creating a request
var request1 = new Request("A");
var request2 = new Request("B");
var request3 = new Request("C");
var request4 = new Request("D");
// Handling the requests
handlerA.HandleRequest(request1);
Console.WriteLine();
handlerA.HandleRequest(request2);
Console.WriteLine();
handlerA.HandleRequest(request3);
Console.WriteLine();
handlerA.HandleRequest(request4);
}
}
Output:
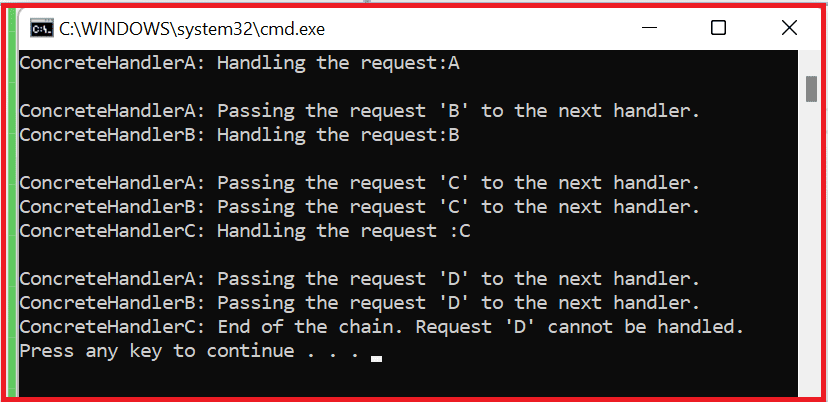
Real-time example of using the Chain of Responsibility pattern
Let’s consider a real-time example of using the Chain of Responsibility pattern in a logging system.
Imagine you have a system that logs various events, such as errors, warnings, and information messages. You want to implement a logging system that can handle different types of events and route them to the appropriate loggers based on their severity level.
The following is an example implementation using the Chain of Responsibility pattern:
using System;
// Handler interface
public interface ILogHandler
{
void SetNext(ILogHandler handler);
void HandleLog(Log log);
}
// ConcreteHandlerA class
public class FileLogHandler : ILogHandler
{
private readonly LogLevel _level;
private ILogHandler _nextHandler;
public FileLogHandler(LogLevel level)
{
_level = level;
}
public void SetNext(ILogHandler handler)
{
_nextHandler = handler;
}
public void HandleLog(Log log)
{
if (log.Level <= _level)
{
// Handle the log message
Console.WriteLine($"[FileLogHandler]: {log.Message}");
}
else if (_nextHandler != null)
{
_nextHandler.HandleLog(log);
}
}
}
// ConcreteHandlerB class
public class ConsoleLogHandler : ILogHandler
{
private readonly LogLevel _level;
private ILogHandler _nextHandler;
public ConsoleLogHandler(LogLevel level)
{
_level = level;
}
public void SetNext(ILogHandler handler)
{
_nextHandler = handler;
}
public void HandleLog(Log log)
{
if (log.Level <= _level)
{
// Handle the log message
Console.WriteLine($"[ConsoleLogHandler]: {log.Message}");
}
else if (_nextHandler != null)
{
_nextHandler.HandleLog(log);
}
}
}
// Log class
public class Log
{
public string Message { get; set; }
public LogLevel Level { get; set; }
}
// LogLevel enumeration
public enum LogLevel
{
Info,
Warning,
Error
}
// Client code
public class Client
{
public static void Main()
{
// Creating instances of the concrete log handlers
var fileHandler = new FileLogHandler(LogLevel.Error);
var consoleHandler = new ConsoleLogHandler(LogLevel.Info);
// Building the chain
fileHandler.SetNext(consoleHandler);
// Logging messages
var log1 = new Log { Message = "Information message", Level = LogLevel.Info };
var log2 = new Log { Message = "Warning message", Level = LogLevel.Warning };
var log3 = new Log { Message = "Error message", Level = LogLevel.Error };
// Handling log messages
fileHandler.HandleLog(log1);
fileHandler.HandleLog(log2);
fileHandler.HandleLog(log3);
}
}
Output:
[FileLogHandler]: Information message
[FileLogHandler]: Warning message
[FileLogHandler]: Error message
Benefits and Drawbacks of the Chain of Responsibility Pattern:
The followings are the benefits and Drawbacks of the Chain of Responsibility Pattern.
Benefits:
- The chain of Responsibility Pattern enables loose coupling between the sender and receiver objects.
- Offers flexibility in dynamically configuring the chain and adding or removing handlers.
- It provides a straightforward way to handle requests hierarchically.
Drawbacks:
- Requests may go unhandled if the chain is not configured correctly.
- The performance might be impacted if the chain is long or complex.
Conclusion:
The Chain of Responsibility design pattern provides an elegant solution for decoupling the sender and receiver of a request. We can easily extend and modify the behavior by implementing a chain of handlers without impacting the client code. Understanding and applying this pattern can significantly enhance the flexibility and maintainability of your C# applications.
FAQs:
Here are some frequently asked questions (FAQs) related to the Chain of Responsibility design pattern in C#:
Q: What is the Chain of Responsibility design pattern?Â
The Chain of Responsibility is a behavioral design pattern that allows an object to pass a request along a chain of potential handlers until the request is handled or reaches the end of the chain.
It decouples the sender of the request from its receivers, providing flexibility and extensibility in handling requests.
Q: When should I use the Chain of Responsibility pattern?
The Chain of Responsibility pattern is useful in scenarios where multiple objects can handle a request, and the handler is determined dynamically at runtime. It is beneficial when you want to avoid coupling the sender and receiver and allow different objects to handle a request independently.
Q: How does the Chain of Responsibility pattern work in C#?Â
In C#, the Chain of Responsibility pattern typically involves defining an interface for the handlers, each implementing the interface with its handling logic. Each handler has a reference to the next handler in the chain. When a request is made, it is passed through the chain until a handler can handle it or until it reaches the end of the chain.
Q: Can you modify the chain dynamically?Â
Yes, one of the advantages of the Chain of Responsibility pattern is its flexibility in dynamically configuring the chain. You can add or remove handlers at runtime, modify the order of handlers, or change the chain altogether to suit your application’s needs.
Q: What are the benefits of using the Chain of Responsibility pattern?Â
The Chain of Responsibility pattern promotes loose coupling between objects, allows for dynamic configuration of the chain, and provides a way to handle requests hierarchically. It enhances flexibility, extensibility, and maintainability in handling requests, making the code more modular and reusable.
Q: Are there any drawbacks when using the Chain of Responsibility pattern?Â
One drawback is that requests may go unhandled if the chain is not configured correctly, leading to potential issues. Additionally, if the chain becomes too long or complex, performance may be impacted as each handler needs to be traversed until a suitable one is found.
Q: Can the Chain of Responsibility pattern be combined with other design patterns?Â
Yes, The Chain of Responsibility pattern can be combined with other design patterns. For example, it is often used with the Command pattern to encapsulate requests as objects or with the Decorator pattern to add responsibilities to the handlers dynamically.
References: WikiPedia-Chain-Of-Responsibility-Pattern
Recommended Articles:
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- C# Dependency Injection
- Design Patterns
- Builder Design Pattern: A Comprehensive Guide with C# Code Examples
- Abstract Factory Design Pattern in C#
- Prototype Design Pattern
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024