As you know, a value type variable cannot be assigned a null value. For example, if you try to assign a null value to the value type int i = null it will give you a compile-time error.
In this post, you will learn about the C# Nullable Types with the help of multiple examples.
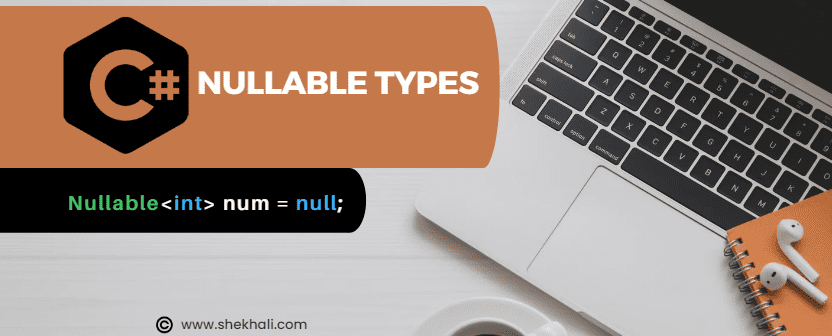
Table of Contents
What are Nullable types?
In C#, Nullable types allow variables to hold either a value or null.
We can declare a null value using Nullable<T>
where T
is a type like an int, double, float, bool, and so on.
C# 2.0 introduced us to the Nullable types to assign a value data type to null but later in C# 8.0, the nullable types were also introduced to hold the null value for Reference Type.
Declaration
The operator “?” can be used to declare any data type as a nullable type.
Syntax:
Nullable<T> variable_name
// or
< data_type> ? <variable_name> = null;
// Example:
int? i = null;
Properties of Nullable types
Following are the two properties of nullable types.
Properties | Description |
---|---|
HasValue: | This property returns a boolean value based on whether the nullable variable has a valid value. It returns true if the variable has a value. Otherwise, returns false if the value is missing or null. |
Value: | This property returns the value of the current Nullable type variable if it has been assigned a valid underlying value. Otherwise, it will throw a runtime InvalidOperationException exception when the variable value is null. |
Example 1:
The example below shows how to declare variables as nullable types in C#.
// C# program to demonstrate the Nullable types in C#
using System;
namespace Nullable_Types_Example
{
class Program
{
static void Main(string[] args)
{
try
{
Nullable<int> num1 = null;
Console.WriteLine($" num1: {num1.HasValue}"); // Print False
// You can declare nullable type like below as well.
int? num2 = 10;
Console.WriteLine($" num2: {num2.HasValue}"); // Print True
int? num3 = 20;
Console.WriteLine($" num3: {num3.Value}"); // Print 20
int? num4 = null;
Console.WriteLine($" num3: {num4.Value}"); // Thow run time 'InvalidOperationException'
}
catch(Exception ex)
{
Console.WriteLine(ex);
}
Console.ReadLine();
}
}
}
As shown in the image below, the Value property of the nullable variable “num4” throws an InvalidOperationException exception because it is null and does not contain a value.
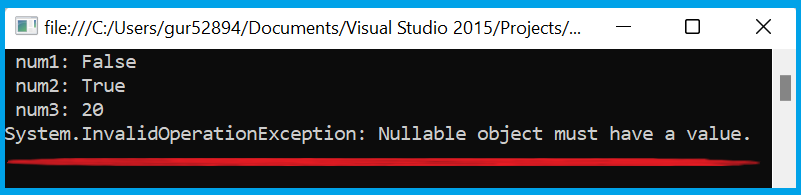
Example 2:
int? x = null;
int? y = 10;
Console.WriteLine($" x = {x} , y = {y}");
Console.ReadLine();
// Output: x = , y = 10
In the above example, the value of x is null, so nothing was printed; only the value of y is printed because it has a value.
GetValueOrDefault() method of Nullable Types
The GetValueOrDefault() method is used to get the value of a nullable type. It will return the value of the nullable type if it contains a value, or the default value if null.
Nullable<int> num1 = null;
Nullable<int> num2 = 5;
double? a = null;
double? b = 99.9;
bool? c = true;
bool? d = false;
bool? e = null;
Console.WriteLine(num1.GetValueOrDefault());// Returns 0
Console.WriteLine(num2.GetValueOrDefault()); // Returns 5
Console.WriteLine(a.GetValueOrDefault()); // Returns 0
Console.WriteLine(b.GetValueOrDefault()); // Returns 99.9
Console.WriteLine(c.GetValueOrDefault()); // Returns True
Console.WriteLine(d.GetValueOrDefault()); // Returns False
Console.WriteLine(e.GetValueOrDefault()); // Returns False
Console.ReadLine();
The null coalescing operator (??)
The null coalescing operator (??) can be used with the nullable value types and reference types. It is used to define the default value that will be returned when you assign a nullable type to a non-nullable type.
If the value of the first operand is null, then the operator returns the value of the second operand, otherwise, the operator returns the value of the first operand. Here is an example of code that illustrates this.
// C# program to demonstrate the null-coalescing operator in C#
using System;
namespace Nullable_Types_Example
{
class Program
{
static void Main(string[] args)
{
int? x = null;
int y = x ?? 100;
Console.WriteLine($" x = {x} , y = {y}");
Console.ReadLine();
// Output: x = , y = 100
}
}
}
If you look at the above code example, you will notice that we used a null-coalescing operator ?? to assign the value of the nullable variable x to non-nullable variable y and specified the value (100) to be assigned to variable y if the variable x value is null.
Assignment rule for Nullable Type
We must assign a value to the nullable type while declaring the local variable. Otherwise, the program will give a compile-time error. Here is an example of code to demonstrate this.
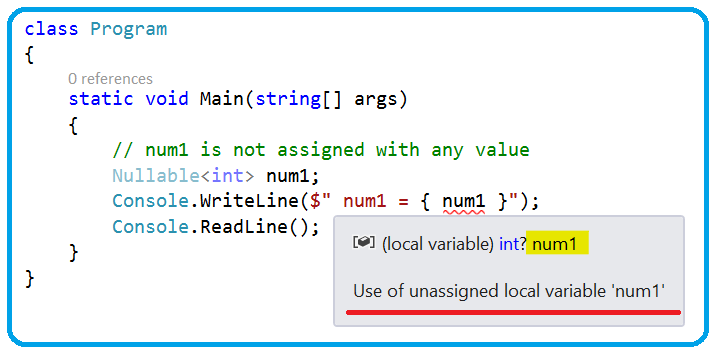
C# Nullable Types Characteristics
The following are the key features of the nullable type in C#.
Nullable<T>
types are useful to assign a null value to the value type variables such as int, long, float, etc.- We can declare nullable types like
Nullable<T>
or?
both are the same. The syntax?
is shorthand forNullable<T>
- The
HasValue
property of the nullable type will return true if the variable has a value and false if it is null. - The
Value
property of the nullable type variable will throw anInvalidOperationException
if the value is null; otherwise, it will return the value. - The nullable type concept is not valid with the “var” keyword.
- It is illegal to assign a nullable type variable value to a non-nullable type variable.
- Example:
int? x = 10;
int y = x;
// Throw compile time error. - We can use
==
and!=
operators with a nullable type. - If a nullable type variable is declared at the class level then it will have a null value by default.
- A nullable type variable declared at the function level must have value otherwise a compile-time error will occur. Example:
int? num;
Refer to this link to read more about the Value Type and Reference Type in C#
FAQ
Q: Why should you use nullable types?
If a variable does not have a value, you can set it to null with nullable types. This makes it clear that the variable has no value and can help prevent errors.
For example, the default value for int is 0. However, 0 could represent a valid value. This can result in a number of bugs that are difficult to track down.
Q: What is the difference between “Nullable” and “?” annotation?
Nullable<T> and “?” are the same. The “?” annotation is a shortcut for Nullable<T> in C#.
Q: How do nullable types get declared in C#?
Nullable types can be declared using Nullable<T> where T is a type. For Example: Nullable<int> i = null;
References: MSDN- Nullable value types
Conclusion
In this Post, We learned about the nullable types with multiple examples. I hope this has been informative and useful to you and that you have found what you were looking for. If you like this post, please do comment and share it with others.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025