Introduction: Design patterns are reusable solutions to common problems in software design. One such pattern is the Prototype Design Pattern, which allows you to create new objects by cloning existing ones.
This article will explore the Prototype Design Pattern when to use it, real-world examples, advantages and disadvantages, UML diagram, and how to implement it with a complete code example in C#.
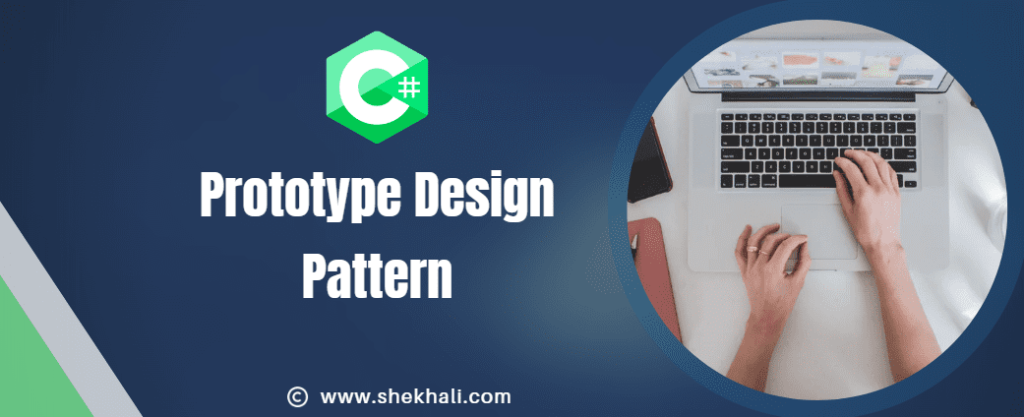
Table of Contents
- 1 Prototype Design Pattern
- 2 When Will We Need the Prototype Design Pattern?
- 3 Real-World Example of Prototype Design Pattern
- 4 Advantage of Prototype Pattern:
- 5 Disadvantages of prototype design pattern:
- 6 Usage of Prototype Pattern:
- 7 UML for Prototype Pattern:
- 8 Complete code Example of Prototype Design Pattern in C#
- 9 Conclusion:
- 10 FAQs
- 10.1 Q: What is the Prototype Design Pattern?
- 10.2 Q: When should you use the Prototype Design Pattern?
- 10.3 Q: What are the disadvantages of the Prototype Design Pattern?
- 10.4 Q: What are the advantages of the Prototype Design Pattern?
- 10.5 Q: What is the difference between a shallow and deep copy?
- 10.6 Related
Prototype Design Pattern
The Prototype Design Pattern is a creational pattern that creates new objects by cloning existing ones. It allows you to avoid creating objects from scratch and instead copy an existing object to create a new one.
This pattern specifies the kinds of objects to create using a prototypical instance, and it creates new objects by copying this Prototype.
When Will We Need the Prototype Design Pattern?
You might consider using the Prototype Design Pattern in the following scenarios:
- When creating new objects is expensive and time-consuming.
- When a class cannot anticipate the type of objects it needs to create
- When a class wants to delegate the responsibility of object creation to another class
Real-World Example of Prototype Design Pattern
Suppose you have a game where you can create different types of units, such as archers, knights, and mages. Each unit has specific characteristics such as attack, defense, and speed.
Instead of creating each unit from scratch, you can use the Prototype Design Pattern to clone an existing unit and modify its attributes as needed.
Advantage of Prototype Pattern:
- It reduces the need for creating new objects from scratch, saving time and resources.
- It allows you to configure objects at runtime dynamically.
- It provides a way to isolate the client code from the complexity of object creation.
Disadvantages of prototype design pattern:
- It can lead to the creation of deep object hierarchies and complex object graphs.
- It can increase memory usage since objects are cloned instead of instantiated.
- Implementing natively can be challenging in languages that do not support object cloning.
Usage of Prototype Pattern:
The Prototype Design Pattern is commonly used in scenarios where object creation is expensive and time-consuming, and the system wants to delegate the responsibility of object creation to another class. We can also use the Prototype Design Pattern with other creational patterns, such as the Abstract Factory Pattern.
UML for Prototype Pattern:
The UML diagram for the Prototype Design Pattern includes the following elements:
- Prototype: An abstract class that defines the clone method.
- ConcretePrototype: A concrete implementation of the Prototype class that implements the clone method.
- Client: The class that uses the Prototype to create new objects.
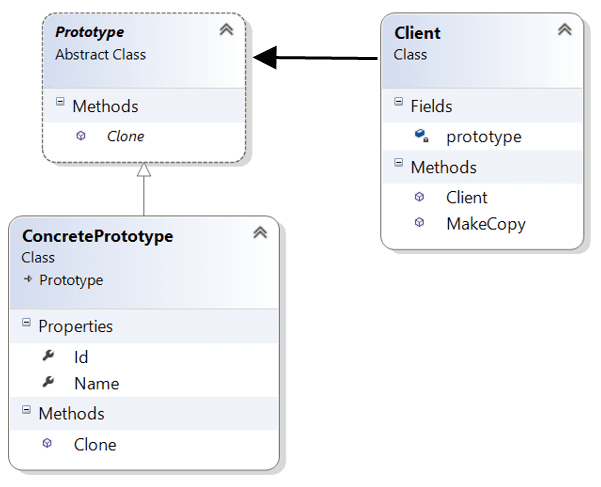
Complete code Example of Prototype Design Pattern in C#
Here is an example of how to implement the Prototype Design Pattern in C#:
using System;
namespace PrototypePattern
{
// Step 1: Create an abstract Prototype class that defines the clone method.
abstract class Prototype
{
public abstract Prototype Clone();
}
// Step 2: Create concrete implementations of the Prototype class that implement the clone method.
class ConcretePrototype : Prototype
{
public int Id { get; set; }
public string Name { get; set; }
public override Prototype Clone()
{
// Use the MemberwiseClone() method to create a shallow copy of the object.
return (Prototype)this.MemberwiseClone();
}
}
// Step 3: Create a client class that uses the Prototype to create new objects.
class Client
{
private Prototype prototype;
public Client(Prototype prototype)
{
this.prototype = prototype;
}
public Prototype MakeCopy()
{
return prototype.Clone();
}
}
class Program
{
static void Main(string[] args)
{
// Step 4: In the client code, create an instance of the concrete Prototype class and clone it to create a new instance.
ConcretePrototype prototype = new ConcretePrototype() { Id = 1, Name = "Prototype 1" };
Client client = new Client(prototype);
ConcretePrototype copy = (ConcretePrototype)client.MakeCopy();
Console.WriteLine("Original Object: Id = {0}, Name = {1}", prototype.Id, prototype.Name);
Console.WriteLine("Cloned Object: Id = {0}, Name = {1}", copy.Id, copy.Name);
Console.ReadLine();
}
}
}
The ConcretePrototype
class defines two properties in the above example: Id and Name.
The Clone() method creates a shallow copy of the object using the MemberwiseClone() method.
The client code creates an instance of the ConcretePrototype
class and passes it to the Client constructor.
The MakeCopy()
method then clones the Prototype to create a new instance.
The output of this program will be:
Original Object: Id = 1, Name = Prototype 1
Cloned Object: Id = 1, Name = Prototype 1
Conclusion:
The Prototype Design Pattern is a useful design pattern that allows you to create new objects by cloning existing ones.
It can help you save time and resources by reducing the need to create new objects from scratch. However, it also has its disadvantages, such as increasing memory usage and creating complex object hierarchies. By understanding when to use this pattern and how to implement it, you can take benefit of its in your software development projects.
References: Wikipedia-Prototype design Pattern
FAQs
Q: What is the Prototype Design Pattern?
Instead of creating a new object from scratch, you can create a copy of an existing object and then modify it as needed. This pattern can be helpful when creating objects is expensive or time-consuming.
Q: When should you use the Prototype Design Pattern?
For example, if you need to create a large number of objects that share some common properties, you can create a prototype object and then clone it to create the new objects. It can save time and reduce memory usage.
Q: What are the disadvantages of the Prototype Design Pattern?
01. It can be difficult to implement correctly, especially if the cloned objects have complex or nested properties.
02.Maintaining consistency between the original object and its clones can be hard, especially if the original object is modified after the clones are created.
03. It can be less efficient than creating new objects from scratch, especially if the cloned objects are simple or small.
Q: What are the advantages of the Prototype Design Pattern?
01. It can save time and memory by reusing existing objects instead of creating new ones.
02. It can simplify object creation by hiding the complexity of creating new objects from the client code.
03. It can provide a way to create new objects similar to existing ones without duplicating code or properties.
Q: What is the difference between a shallow and deep copy?
A shallow copy share references to objects, while a deep copy creates new instances of objects. That means changes to shared objects will affect all shallow copies, not deep ones.
However, deep copies can be more expensive to create, leading to more memory usage.
Articles you might also like:
- Design Patterns
- Builder Design Pattern: A Comprehensive Guide with C# Code Examples
- SOLID Design Principles in C#: A Complete Example
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- Abstract Factory Design Pattern in C#
We would love to hear your thoughts on this post. Please leave a comment below and share it with others.
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024