Design Patterns are a set of reusable solutions to commonly occurring problems in software design. They are not a finished design that we can transform directly into code but rather a guide for solving problems.
The goal of using Design Patterns is to increase the efficiency and effectiveness of software development and provide a common vocabulary for developers to discuss design solutions.
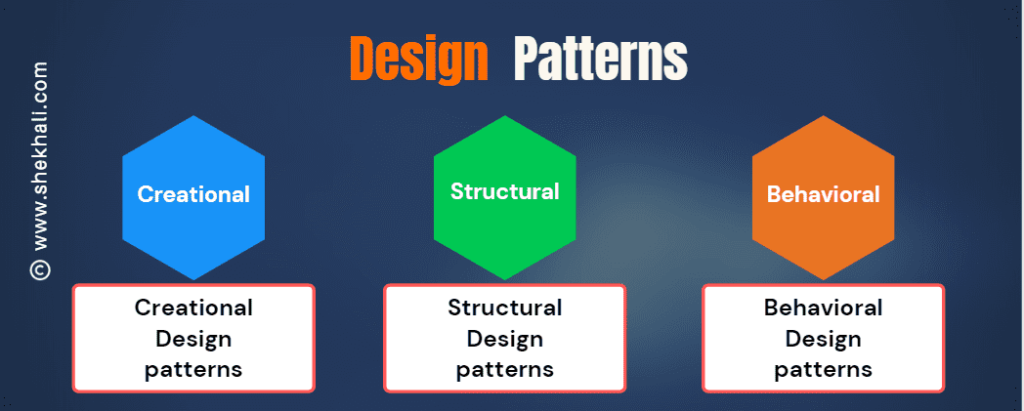
Table of Contents
Uses of Design Patterns
Design Patterns provide a standard way of solving common problems in software design. They help create more flexible, reusable, and maintainable software. Using Design Patterns can also lead to reduced development time and cost and improved communication between developers.
The following is the list of different types of Design Patterns:
Creational Design Patterns:
- Factory Method
- Abstract Factory
- Builder
- Prototype
- Singleton
Structural Design Patterns:
- Adapter
- Bridge
- Composite
- Decorator
- Façade
- Flyweight
- Proxy
Behavioral Design Patterns:
- Chain of Responsibility
- Command
- Interpreter
- Iterator
- Mediator
- Memento
- Observer
- State
- Strategy
- Visitor
- Template Method
01. Creational Design Patterns
Creational Design Patterns deal with the process of object creation. They aim to provide a way to create objects while hiding the creation logic, making the code more flexible and maintainable.
a. Abstract Factory
The Abstract Factory Design Pattern provides an interface for creating families of related or dependent objects without specifying their concrete classes. It separates the client code from the object creation logic, making it easier for us to add new families of objects without changing the client code.
For example, in a game development project, we could use an Abstract Factory to create different types of weapons, such as swords or guns, without specifying the concrete classes of each weapon.
b. Builder
The Builder Design Pattern separates the construction of a complex object from its representation, allowing the same construction process to create different representations. It allows for creating objects step-by-step, making the code more readable and maintainable.
For example, in a website development project, we could use a Builder to create a complex web page by building its components, such as headers, footers, and navigation bars, step-by-step.
c. Factory Method
The Factory Method Design Pattern provides an interface for creating objects, allowing subclasses to decide which class to instantiate. It separates the object creation logic from the client code, making it easier for users to add new types of objects without changing the client code.
For example, we could use a Factory Method in a mobile game development project to create different types of enemies, such as zombies or robots, without specifying their concrete classes.
d. Object Pool
The Object Pool Design Pattern is used to manage a pool of reusable objects, allowing for the reuse of expensive resources. It reduces the overhead of creating new objects by recycling the existing ones.
For example, in a database connection management project, we could use an Object Pool to manage a pool of database connections, allowing multiple clients to share the same connection.
e. Prototype
The Prototype Design Pattern creates new objects by cloning existing ones. It reduces the overhead of creating new objects by copying the existing ones, making the code more efficient and faster.
For example, in a graphic design project, a Prototype could be used to create different versions of the same graphic by cloning and modifying an existing one.
f. Singleton
The Singleton Design Pattern ensures that a class has only one instance, providing a global access point to that instance. It helps manage the global state of an object in an application.
For example, in a logging project, we could use a Singleton to provide a global access point to the logging object instance, ensuring that all log messages are recorded in the same file.
02. Structural Design Patterns
Structural Design Patterns deal with the composition of classes and objects, focusing on their relationships. They aim to simplify the design by identifying simple ways to realize relationships between entities.
a. Adapter
The Adapter Design Pattern converts the interface of a class into another interface that clients expect. It allows incompatible classes to work together by wrapping one class’s interface with another’s.
For example, in a legacy codebase, we could use an Adapter to convert an old interface to a new one without changing the code that uses the old interface.
b. Bridge
The Bridge Design Pattern decouples an abstraction from its implementation, allowing them to vary independently. It separates the interface from the implementation, making the code more flexible and maintainable.
For example, in a user interface design project, we could use the Bridge pattern to separate the user interface components from the underlying platform-specific implementation, allowing for easy portability to different platforms.
c. Composite
The Composite Design Pattern allows for creating a tree-like structure of objects, where individual objects and groups are treated similarly. It makes working with hierarchical data structures easier and simplifies the code.
For example, in a file system project, we could use the Composite pattern to create a tree-like structure of files and folders, where individual files and folders are treated similarly.
d. Decorator
The Decorator Design Pattern dynamically adds behavior to an object without affecting other objects’ behavior. It allows for the dynamic addition of new functionality to an object, making the code more flexible and maintainable.
For example, in a text editor project, we could use the Decorator pattern to add new formatting options to a text document, such as bold or italic, without affecting the existing formatting options.
e. Facade
The Facade Design Pattern provides a simplified interface to a complex subsystem, making it easier to use. It hides the complexity of the subsystem from the client code, making it easier to understand and use.
For example, in a graphics processing project, we could use a Facade to provide a simplified interface to a complex graphics processing subsystem, allowing the client code to use without understanding its complexity.
f. Flyweight
The Flyweight Design Pattern allows for the sharing of a large number of fine-grained objects, reducing memory consumption and improving performance. It separates objects’ intrinsic and extrinsic properties, allowing the inherent properties to be shared between objects.
For example, in a game development project, we could use the Flyweight pattern to share resources such as textures or meshes between different game objects, reducing memory usage and improving performance.
g. Private Class Data
The Private Class, Data Design Pattern, encapsulates data within a class, making it private and only accessible through the class’s methods. It allows for better control over the access and modification of class data.
For example, in a banking system project, we could use the Private Class Data pattern to encapsulate sensitive data such as account balances, ensuring that they can only be accessed and modified through the appropriate methods.
h. Proxy
The Proxy Design Pattern provides a placeholder for an object, allowing for the control of its access and behavior. It will enable additional functionality like access control, caching, or logging.
For example, in a network communication project, we could use a Proxy to provide additional functionality, such as caching or security checks, allowing for better control over the communication.
03. Behavioral Design Patterns
Behavioral Design Patterns deal with the communication between objects and delegating responsibilities among them. They aim to simplify the design by identifying common communication patterns between objects.
a. Chain of Responsibility
The Chain of Responsibility Design Pattern allows for passing requests between a chain of objects until one handles the request. It allows for separating the sender and receiver of a request, making the code more flexible and maintainable.
For example, in a logistics management project, the Chain of Responsibility pattern could handle different types of requests, such as shipping, tracking, or returns, allowing them to be processed by different handlers.
b. Command
The Command Design Pattern encapsulates a request as an object, allowing for the separation of the request’s sender and receiver. It creates a queue of requests, making it possible to undo and redo them.
For example, in a text editor project, we could use the Command pattern to encapsulate a user’s formatting commands, allowing for undo and redo functionality.
c. Interpreter
The Interpreter Design Pattern defines a language and its grammar and provides an interpreter to interpret it. It allows for creating a simple programming language, making it easier to solve complex problems.
For example, in a math processing project, we could use the Interpreter pattern to create a simple programming language for math expressions to evaluate complex formulas.
d. Iterator
The Iterator Design Pattern provides a way to access the elements of an aggregate object sequentially without exposing its internal representation. It allows for creating a flexible and efficient iteration mechanism, making it easy to traverse complex data structures.
For example, in a database management project, we could use the Iterator pattern to traverse the results of a database query, allowing for the efficient processing of large amounts of data.
e. Mediator
The Mediator Design Pattern defines an object that encapsulates how a set of objects interact. It allows for the reduction of direct coupling between objects, making the code more flexible and maintainable.
For example, in a user interface design project, we could use the Mediator pattern to define an object that manages the interactions between different user interface components, reducing direct coupling between them.
f. Memento
The Memento Design Pattern provides a way to capture and restore an object’s state without violating its encapsulation. It allows for creating a snapshot of an object’s state, making it possible to restore it later.
For example, in a game development project, we could use the Memento pattern to create a snapshot of a game’s state, allowing the player to save and load the game at any point.
g. Null Object
The Null Object Design Pattern allows one to handle null values without throwing exceptions. It allows for creating a null object that implements the same interface as a real object, making it possible to avoid null checks.
For example, in a billing system project, we could use the Null Object pattern to provide a default behavior for a null account, making it possible to avoid null checks and simplify the code.
h. Observer
The Observer Design Pattern defines a one-to-many dependency between objects so that all its dependents are notified and updated automatically when one Object changes state. It allows for creating of a loosely-coupled design, making the code more flexible and maintainable.
For example, in a stock trading project, we could use the Observer pattern to notify traders of changes in stock prices, allowing them to make informed decisions.
i. State
The State Design Pattern allows an object to alter its behavior when its internal state changes. It allows for creating a flexible design, making it possible to change an object’s behavior dynamically.
For example, in a game development project, we could use the State pattern to change the behavior of a game character when it changes its state, such as when it enters a combat mode.
j. Strategy
The Strategy Design Pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. It allows for selecting an algorithm at runtime, making the code more flexible and maintainable.
For example, in a data processing project, we could use the Strategy pattern to encapsulate different sorting algorithms, allowing the selection of a specific algorithm at runtime.
k. Template Method
The Template Method Design Pattern defines the skeleton of an algorithm in a method, allowing its subclasses to redefine certain algorithm steps without changing its structure. It allows for the creation of a flexible design.
For example, in a game development project, we could use the Template Method pattern to define the basic gameplay loop, allowing subclasses to define specific gameplay mechanics without changing the overall structure.
l. Visitor
The Visitor Design Pattern allows for the separation of an algorithm from the object structure on which it operates. It allows for creating a flexible and extensible design, adding new algorithms without changing the object structure.
For example, in a text processing project, we could use the Visitor pattern to define an algorithm that operates on a text document’s structure, allowing for adding new operations without changing the document’s structure.
Summary
Design Patterns are reusable solutions to common software development problems. They provide a way to create flexible, extensible, and maintainable software by encapsulating and abstracting complex code into simple and reusable modules. They can help developers solve complex problems and reduce the coupling between different system parts.
There are three categories of Design Patterns: Creational, Structural, and Behavioral. Each category includes several patterns that solve specific problems, such as object creation, object composition, or object communication.
Design Patterns are a fundamental concept in software development and are widely used in various programming languages, including C#. By understanding and applying Design Patterns, developers can write better code, improve their software development skills, and create more efficient and maintainable software systems.
FAQs
The following are few FAQs about the Design Patterns.
Q: What are Design Patterns?
Design Patterns are reusable solutions to common software development problems. They are a way to encapsulate and abstract complex code into simple and reusable modules, making writing more efficient, maintainable, and extensible software easier.
Q: Why are Design Patterns important?
Design Patterns are important because they can help developers to write better code and improve their software development skills. They provide a way to solve complex problems, reduce coupling between different parts of a system, and create more efficient and maintainable software systems.
Q: What is the difference between Creational, Structural, and Behavioral Design Patterns?
Creational patterns focus on object creation, and structural patterns deal with object composition, and Behavioral patterns address object communication and control flow. Each category has its specific patterns and use cases.
Q: Can Design Patterns being used in all programming languages?
Yes, Design Patterns are a fundamental concept in software development, and We can use them in almost any programming language. However, implementing specific patterns may vary depending on the language and the context.
References: Source Making-Design Patterns
Articles you might also like:
- SOLID Design Principles in C#: A Complete Example
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- Abstract Factory Design Pattern in C#
- Prototype Design Pattern: Everything You Need to Know
- Builder Design Pattern
- C# Dependency Injection
We would love to hear your thoughts on this post. Please leave a comment below and share it with others.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025