In this post, we will be discussing a common confusion among C# developers: the difference between == and equals in C#. Whether you are a beginner or an experienced developer, understanding the difference between these two operators is crucial for writing efficient and accurate code.
In C#, the equality operator ‘==’ evaluates the equality of two operands, while the
Object.Equals()
method determines if two object instances are equal or not.
In this post, we will cover the purpose, usage, speed, and flexibility of ‘==’ and ‘.Equals()’, as well as some frequently asked questions. So, let’s get started!
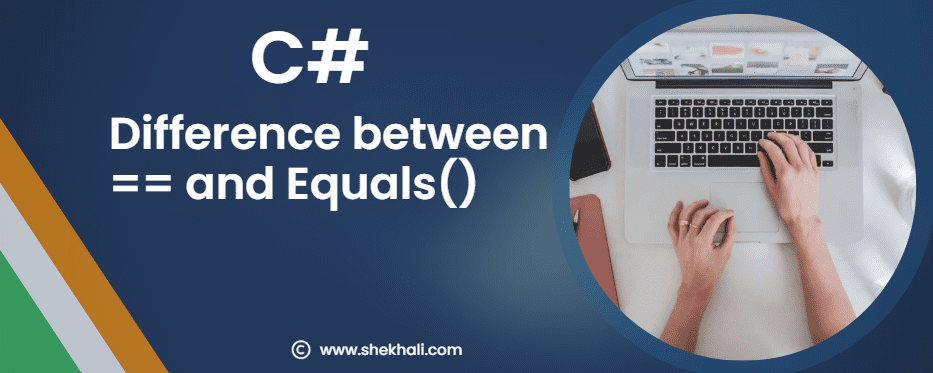
Table of Contents
- 1 Equality operator ‘==’ in C#
- 2 Equals() method in C#
- 3 Difference between == and equals in C#: A Quick Comparison
- 4 Difference between == and equals in C#
- 5 When to use ‘==’ and when to use ‘.Equals()’?
- 6 Avoiding the NullReferenceException: A Comparison of the ‘==’ Operator and the Equals() Method in C#
- 7 FAQ:
- 7.1 Q: What is the purpose of the ‘==’ operator in C#?
- 7.2 Q: What is the purpose of the ‘.Equals()’ method in C#?
- 7.3 Q: What is the difference between ‘==’ and ‘.Equals()’?
- 7.4 Q: When should you use ‘==’ and when should I use ‘.Equals()’?
- 7.5 Q: Can the equality operator ‘==’ be overloaded for custom comparison?
- 7.6 Q: Is Equals() slower than ‘==’?
- 7.7 Related
Equality operator ‘==’ in C#
- The equality operator ‘==’ is used to compare the values of two variables.
- It checks if both variables are equal in terms of value, not reference.
- Example: int x = 5, y = 5; (x == y) is true.
The == operator in C# returns true if:
- the operands are Value Types and their values are equal, or
- the operands are Reference Types (excluding string) and both refer to the same instance, or
- the operands are string type and their values are equal.
- Otherwise, it returns false.
Example: Comparing Values with ‘==’ Operator in C#
using System;
namespace EqualityOperatorExample
{
class Program
{
static void Main(string[] args)
{
int x = 5;
int y = 5;
bool result = x == y;
Console.WriteLine(result); // Output: True
float a = 1.0f;
float b = 1.0f;
result = a == b;
Console.WriteLine(result); // Output: True
char c = 'A';
char d = 'A';
result = c == d;
Console.WriteLine(result); // Output: True
Console.ReadLine();
}
}
}
Equals() method in C#
- The
Object.Equals()
method is used to compare the contents of two objects. - It checks if both objects are equal in terms of content, not reference.
- Example: object x = 5, y = 5; x.Equals(y) is true.
Example: Comparing Contents with Equals() Method in C#
using System;
using System.Text;
namespace EqualMethodExample
{
class Program
{
static void Main(string[] args)
{
string str1 = "Hello";
string str2 = "Hello";
bool result = str1.Equals(str2);
Console.WriteLine($"\n str1.Equals(str2): {result}"); // Output: True
string[] arr1 = { "A", "B", "C" };
string[] arr2 = { "A", "B", "C" };
result = arr1.Equals(arr2);
Console.WriteLine($" arr1.Equals(arr2): {result}"); // Output: False
StringBuilder sb1 = new StringBuilder("Hello");
StringBuilder sb2 = new StringBuilder("Hello");
Console.WriteLine(" sb1.Equals(sb2): {0}", sb1.Equals(sb2)); // Output: True
Console.WriteLine(" Object.Equals(sb1, sb2): {0}", Object.Equals(sb1, sb2)); // Output: False
Object sb3 = new StringBuilder("Hello");
Console.WriteLine("\n sb3.Equals(sb2): {0}", sb3.Equals(sb2)); // Output: False
Console.ReadLine();
}
}
}
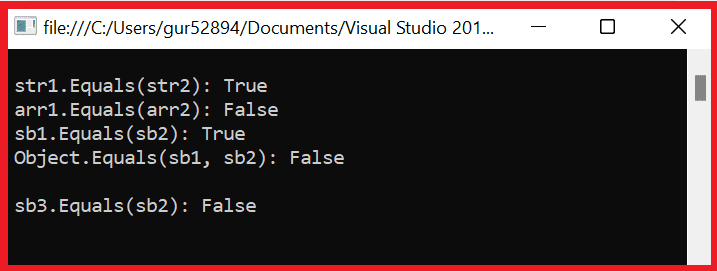
Note: In the second example arr1.Equals(arr2)
, the Equals() method returns False because the arrays are reference types and the Equal() method compares references, not contents. To compare the contents of arrays, you can use a loop to compare each element or use a custom comparison method.
In the above example, the sb1.Equals(sb2)
returns True because the Equals method of the StringBuilder class compares the contents of the two StringBuilder objects, which are the same in this case.
Object.Equals(sb1, sb2)
return False because it checks for reference equality, meaning it returns True only if the two objects being compared refer to the same instance in memory. Since sb1 and sb2 are two separate instances of the StringBuilder class, they are not equal in reference.
Difference between == and equals in C#: A Quick Comparison
Feature | ‘==’ operator | Equals() method |
---|---|---|
Purpose: | Compare the values of two variables. | Compare the contents of two objects. |
Speed: | Faster | Slower |
Overloading: | Overloaded for specific value types. | It can be overridden for custom comparison. |
Usage: | Used for value types (e.g. int, float, char, etc.) | Used for reference types (e.g. objects, strings, arrays, etc.) and for custom comparison |
The ‘==’ operator is a faster way to compare values of two variables in C#, but it is limited to specific value types and cannot be overloaded for custom comparison. On the other hand, the Object.Equals() method allows for more flexibility and can be overridden for custom comparison, but it is slower due to its detailed comparison of object contents. Choose wisely based on your needs and the data types you are working with.
Difference between == and equals in C#
- The ‘==’ operator compares values, while the Equals() method compares content.
- The ‘==’ operator is faster, while the ‘.Equals()’ method is slower but more flexible.
- The ‘==’ operator is overloaded for specific types, while the ‘.Equals()’ method can be overridden for custom object comparison.
- The “==” operator in C# is used for comparing values, while the “.Equals()” method is specifically designed to compare the contents of objects, particularly strings. The “.Equals()” method focuses solely on the content comparison.
When to use ‘==’ and when to use ‘.Equals()’?
- Use the equality operator
==
when comparing value types (e.g. int, float, char, etc.). - Use
Equals()
method when comparing reference types (e.g. objects, strings, arrays, etc.). - Use ‘.Equals()’ when a custom comparison is needed for reference types.
Avoiding the NullReferenceException: A Comparison of the ‘==’ Operator and the Equals() Method in C#
using System;
namespace EqualMethodExample
{
class Program
{
static void Main(string[] args)
{
string str = null;
// Using the == operator to compare values
bool result = str == "Hello";
Console.WriteLine(" Using == operator: " + result);
// Using the .Equals() method to compare contents
try
{
result = str.Equals("Hello");
Console.WriteLine(" Using .Equals() method: " + result);
}
catch (NullReferenceException e)
{
Console.WriteLine(" Using .Equals() method: " + e.Message);
}
Console.ReadLine();
}
}
}
Output:
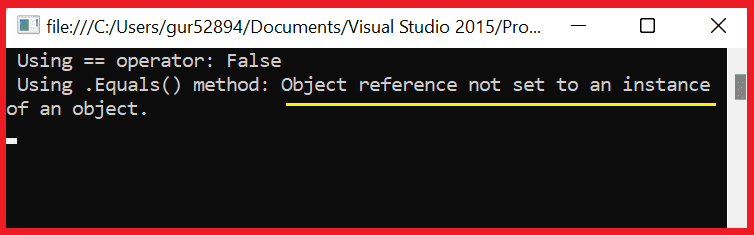
The code example defines a string variable “str” with a null value. The ‘==’ operator is used to compare “str” to “Hello”, which returns False due to “str” being null. The Equals() method is then used to compare “str” to “Hello”, but accessing the contents of “str” will result in a NullReferenceException as it is null.
FAQ:
Q: What is the purpose of the ‘==’ operator in C#?
The ‘==’ operator is used to compare the values of two variables in C#. It checks if both variables are equal in terms of value, not reference.
Q: What is the purpose of the ‘.Equals()’ method in C#?
The Equals()
method is used to compare the contents of two objects in C#. It checks if both objects are equal in terms of content, not reference.
Q: What is the difference between ‘==’ and ‘.Equals()’?
The main difference between ‘==’ and Equals() method is that the ‘==’ operator compares values, while the Equals() method compares content. The ‘==’ operator is faster, but the ‘.Equals()’ method is more flexible and can be overridden for custom comparison.
Q: When should you use ‘==’ and when should I use ‘.Equals()’?
You should use the equality operator ‘==’ when comparing value types (e.g. int, float, char, etc.), and use Equals() method when comparing reference types (e.g. objects, strings, arrays, etc.) or when a custom comparison is needed.
Q: Can the equality operator ‘==’ be overloaded for custom comparison?
No, the equality operator ‘==’ is overloaded only for specific types in C#. To perform a custom comparison, use the Equals() method.
Q: Is Equals() slower than ‘==’?
Yes, Equals() method is slower than ‘==’ because it performs a more detailed comparison of the contents of two objects.
You might also like:
- SOLID Design Principles in C#: A Complete Example
- C# Struct vs Class |Top 15+ Differences Between C# Struct and Class
- 10 Difference between interface and abstract class In C#
- C# Queue with examples
- Web API vs web service: Top 10+ Differences between web API and web service in C#
- C# Polymorphism: Different types of polymorphism in C# with examples
- C# Stack
- C# Hashtable
- C# Hashtable vs Dictionary vs HashSet
- Generic Delegates in C# With Examples
- Params Keyword in C# With Examples
- Difference between SortedList and SortedDictionary in C#
- Understanding the Difference between int, Int16, Int32, and Int64 in C#
- Jump Statements in C# (Break, Continue, Goto, Return, and Throw)
- Is vs As operator in C#: Understanding the differences between is and as operator in C#
We would love to hear your thoughts on this post. Please leave a comment below and share it with others.
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024