Difference between const and readonly:
The primary difference between read-only
and const
keywords is that const
represents a compile-time constant, whereas read-only
is a runtime constant.
The Constant variables must be initialized at compile-time with fixed values. Their values are known during compilation and, once assigned, cannot be changed afterwards.
On the other hand, Read-Only variables are also immutable. They can be assigned a value either at the time of declaration or at runtime within the constructor but cannot be modified afterwards for the life of the program.
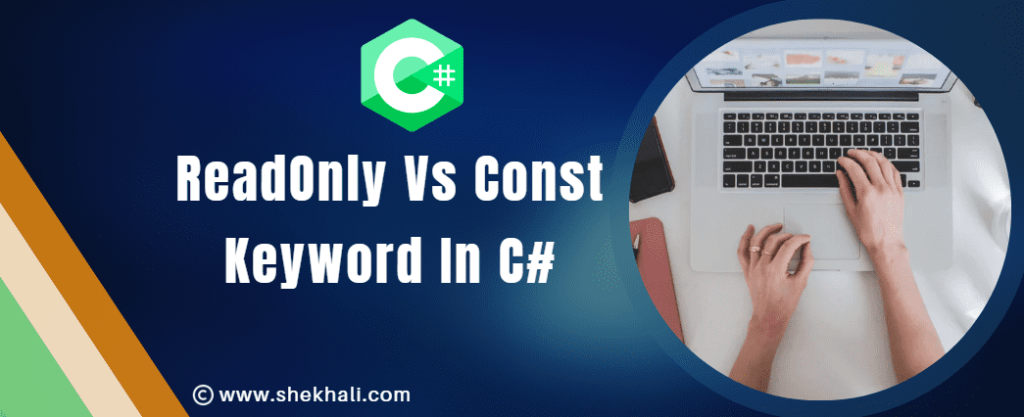
In this article, We will look at the differences between the readonly and const keywords in and when to use each.
Table of Contents
What is read-only in C#?
The readonly
keyword is used to declare a variable whose value can only be set during initialization or within the constructor of the same class. Once set, it cannot be modified.
It allows each instance of the class to have its own distinct value for the readonly
fields.
Example: ReadOnly variable in C#
Let’s take an example of the readonly variable in C#.
using System;
class Person
{
// Declare a readonly field
public readonly int age = 18;
public Person() // Constructor
{
age = 22;
}
static void Main(string[] args)
{
Person obj = new Person();
Console.WriteLine(obj.age); // Output: 22
}
}
In the above code, age is declared as a readonly variable with a value of 18. However, its value can be changed to 22 in the constructor.
What is const in C#?
The const
keyword is used for declaring a fixed value that remains unchanged during the entire execution of a program.
The value assigned to a constant variable is determined during the compilation phase and cannot be changed during runtime. In simple words, When you declare a variable as const, you are telling the compiler that the value of this variable will never change.
A constant variable is a readonly
variable, but the value is determined at the time of compilation and stored in the assembly metadata.
The
const
ant variable is implicitly static and is embedded in the Intermediate Language (IL) code of every assembly that uses them, whilereadonly
variables are stored in heap memory.
The Constants are frequently used for values which do not change at runtime, such as mathematical constants or enumeration values.
For example, if you have a mathematical constant such as π (pi)
, you should declare it as a constant:
Example: Const keyword in C#
Let’s take an example of the const
keyword in C#.
using System;
class Program
{
const double PI = Math.PI;// using const keyword
static void Main(string[] args)
{
Console.WriteLine(PI); //Output: 3.14159265358979
}
}
During the compilation process, the value of constant fields is saved within the DLL or EXE file and can be viewed using the ILDASM tool.
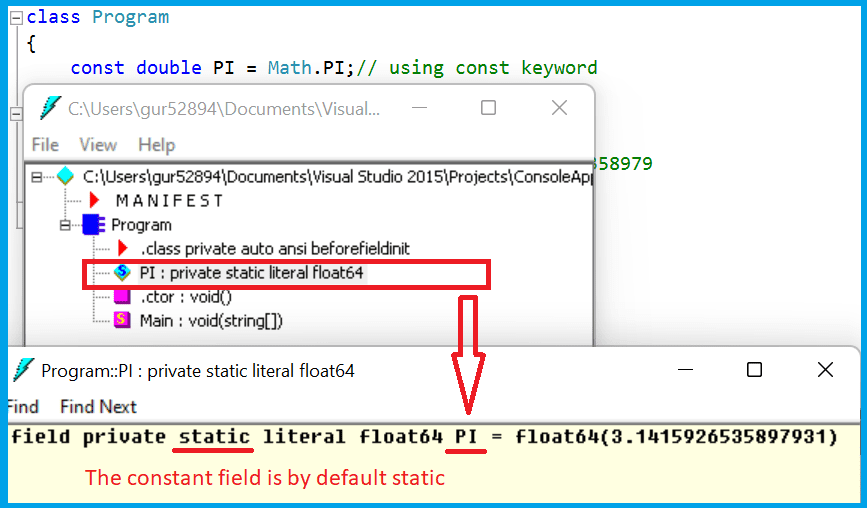
- The const keyword is used to declare a constant value that we cannot change during the execution of a program.
- The readonly keyword is used to declare variables that we can initialize only once, either during declaration time or in a constructor.
Difference between readonly and const keyword in C#
01. Modification:
- We cannot change the “const” keyword values once they are assigned.
- The “readonly” keyword values can only be assigned once at the declaration time or in a constructor and cannot be modified afterward.
02. Initialization:
- We must initialize the “const” keyword values at the declaration.
- We can initialize the “readonly” keyword values either at the declaration or in a constructor.
03. Memory allocation:
- The “const” keyword values are stored in memory as a part of the program’s metadata.
- The “readonly” keyword values are stored in memory in the same way as other variables.
04. Accessibility:
- The “const” keyword values are accessible throughout the program.
- The “readonly” keyword values are accessible only within the class where they are declared.
05. Performance:
- The “const” keyword values have better performance as they are stored in memory as part of the assembly or program metadata.
- The “readonly” keyword values have slightly lower performance as they are stored in memory like other variables.
When to use constant?
- When you need to declare a constant value that will not change during the execution of the program.
- When you need to access the constant value from multiple classes without creating an instance of the class.
- You can use the constant when performance is a concern.
When to use readonly?
- When you need to declare values to be initialized only once, either at the declaration or in a constructor.
- When you need to restrict the accessibility of the value to only within the class where it is declared.
- When you need to change the value at runtime based on some conditions.
Comparison of read-only and const variable in C#
Key | read-only keyword | const keyword |
---|---|---|
Keyword: | In C#, read-only variable can be created using the readonly keyword. | The Constant variable are created using the const keyword. |
Type: | readonly is a runtime constant. | At the same time, const is a compile-time constant. |
Access: | We can access readonly variables by using an instance of a class. | We can use a ClassName.ConstantName notation to access constant variable. |
Change Value: | The value of a readonly variable can only be altered using the constructor during runtime, but not through a member function. | On the other hand, constants are automatically static, and We cannot modify their value from any source, including the constructor, or function during runtime. |
Declaration: | The fields marked as readonly cannot be declared within a method. | However, fields that are labeled as const can be declared within a method. |
Value: | The readonly value can vary with each instance. | at the same time, the value of a constant is the same across all objects and must be initialized with a literal expression. |
When to use const and readonly in C#
The choice between const and readonly depends on the specific use case. If you have a value that will never change, then you should use const. If you have a value that may change during runtime but only during initialization or in the constructor, then you should use readonly.
For example, if you have a mathematical constant such as π (pi), you should declare it as const:
const double pi = 3.14159265358979;
On the other hand, if you have a value that needs to be initialized from a database or user input, you should declare it read-only:
class Person
{
public readonly int userAge;
public Person(int age)
{
userAge = age;
}
}
FAQs:
Below is a list of frequently asked questions about the difference between read-only
and const
keywords in C#
Q: What is the purpose of using the read-only keyword in C#?
The readonly
keyword is used to create a read-only variable in C#, meaning we cannot change its value after it is declared. However, we can only set it during declaration or runtime in the constructor.
Q: What is the purpose of using the const keyword in C#?
The const keyword creates a constant field in C#, meaning its value must be assigned at compile time and cannot change afterward.
Q: Can a read-only field be declared within a method in C#?
No, a read-only field cannot be declared within a method. It must be declared as an instance variable and assigned a value when declared or in a constructor of the same class.
Q: Can a const field be declared within a method in C#?
Yes, a const field can be declared inside a method in C#, but we must assign its value during declaration time.
References: MSDN-ReadOnly in C#, geeks for geeks- readonly and const keyword in C#
Articles you might also like:
- C# Tutorial
- C# Abstract class Vs Interface
- C# Array Vs List
- Is vs As operator in C#
- Difference Between Static, ReadOnly, and Constant in C#
- Jump Statements in C# (Break, Continue, Goto, Return, and Throw)
- Singleton Design Pattern in C#
- Value Type and Reference Type in C#
- C# Stack Class With Push And Pop Examples
- C# Struct vs Class
- Difference between Boxing and Unboxing in C#
- C# dispose vs finalize
- ref and out keyword
Let others know about this post by sharing it and leaving your thoughts in the comments section.
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024