In simple terms, a delegate in C# is like a pointer to a method. It allows you to refer to a method by its name and invoke it later. Think of it as a “method container” that holds a reference to a particular method with a specific signature (parameters and return type).
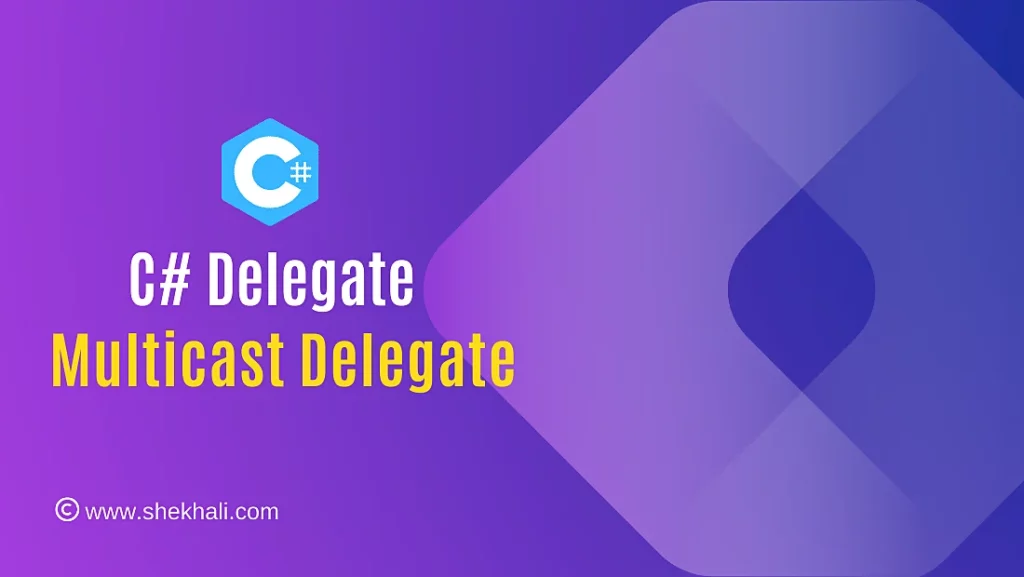
Table of Contents
- 1 What is Delegate in C#?
- 2 Declaring a Delegate:
- 3 Using a Delegate:
- 4 Invoking a Delegate:
- 5 Types of Delegates in C#
- 6 Single cast Delegate:
- 7 Multicast Delegate:
- 8 Important points about C# delegates
- 9 Conclusion
- 10 FAQs
- 10.1 Q: What is a C# delegate?
- 10.2 Q: How is a delegate different from a regular method call?
- 10.3 Q: Why should I use delegates instead of directly invoking the method in C#?
- 10.4 Q: How many types of delegates are there in C#?
- 10.5 Q: Can delegates be private in C#?
- 10.6 Q: What is a Singlecast delegate?
- 10.7 Q: What is a multicast delegate?
- 10.8 Q: How do I remove a method reference from a multicast delegate?
- 10.9 Q: Can a multicast delegate have methods with different signatures?
- 10.10 Q: Can a delegate return a value?
- 10.11 Q: When would I use delegates in my code?
- 10.12 Related
What is Delegate in C#?
In C#, A delegate is a type that represents a reference to a method with a specific signature (parameters and return type).
A delegate is like a container for a method. It’s like having a remote control that can activate a specific function in your code.
Instead of directly calling a method, you can use a delegate to call it indirectly. Delegates are helpful when you want to pass methods as parameters into the other methods as if they were objects.
Delegates are useful for events, callbacks, and handling async operations. They let you define and execute different actions based on conditions or user interactions.
So, a C# delegate is a way to indirectly store and refer to a method. It’s like a remote control or note representing a task to be done later in your program.
The C# delegate shares similarities with the function pointer in C and C++. However, unlike the function pointer in C, the C# delegate is object-oriented, type-safe, and secure.
Declaring a Delegate:
To declare a delegate, you need to define the delegate type. The delegate type specifies the method signature that the delegate can point to.
Here is an example of declaring a delegate called “MyDelegate” that can point to a method taking two integers and returning an integer:
delegate int MyDelegate(int x, int y);
Using a Delegate:
Once you have declared a delegate, you can create an instance of it and assign it a method to invoke. Here is how you can create a delegate
to point to a method:
int Add(int a, int b)
{
return a + b;
}
// Create an instance of MyDelegate and assign it the Add method
MyDelegate del = Add;
Invoking a Delegate:
To invoke a delegate and execute the method it points to, you can use the delegate instance as if it were the method itself:
int result = del(5, 3);
Console.WriteLine(result); // Output: 8
Types of Delegates in C#
There are three types of delegates in C#:
- Single cast Delegates
- Multicast Delegates
- Generic Delegates ( Func, Action, and Predicate delegate)
- A single-cast delegate holds a reference to a single method.
- A multi-cast delegate holds a reference to multiple methods.
- A generic delegate can hold a reference to a method with any signature.
As per MSDN:
The Delegate is an abstract class and it is the base class for all the delegate types.
Even though it is an abstract class only the compilers can derive explicitly from the Delegate class or from the MulticastDelegate class, not the user.
It is not allowed to derive a new type from a delegate type.
The Delegate class is not considered a delegate type. However, it is used to derive delegate types implicitly by the compiler.
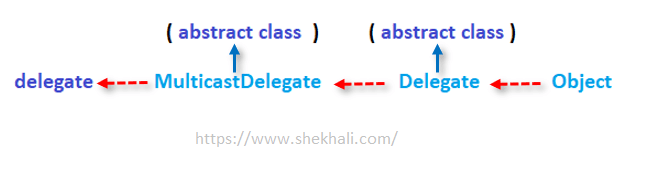
Single cast Delegate:
A single-cast delegate is a type of Delegate that can only point to one method at a time. It is derived from the System.Delegate
class. This means that a single-cast delegate holds a reference to a single method and can invoke that method when called.
Following is the example of the single cast delegate in C#.
using System;
namespace DelegateExample
{
// Defining a delegate to refer the methods having same signature.
public delegate void MyDelegate(string message);
class Program
{
// Defining a method
public static void Print(string message)
{
Console.WriteLine($"{message}");
}
static void Main(string[] args)
{
// Instantiating delegate and passing the method name
// as a parameter which have the same signature
MyDelegate myDelegate = new MyDelegate(Print);
myDelegate.Invoke("Hello Shekh");
// Or
// myDelegate("Hello Shekh");
Console.ReadLine();
}
// Output: Hello Shekh
}
}
Multicast Delegate:
A multicast delegate is a type of Delegate that can hold references to multiple methods. It is derived from the System.MulticastDelegate
class. This means that a multicast delegate can store and invoke more than one method from its invocation list, executing them in sequence when invoked.
To add multiple methods to a multicast delegate, we can use the Delegate.Combine
method or the “+” operator. To remove a method reference from the invocation list, we can use the “-” operator. It’s important to note that when invoking multiple methods using a multicast delegate, all the methods should have the same signature (parameter types and return type must match), and the return type must be void.
Here is an Example of Multicast Delegate:
delegate void MyMultiDelegate();
void Method1()
{
Console.WriteLine("Method 1");
}
void Method2()
{
Console.WriteLine("Method 2");
}
// Create a multicast delegate and add both methods to it
MyMultiDelegate multiDel = Method1;
multiDel += Method2;
// Invoke the multicast delegate, which will call both methods
multiDel(); // Output: Method 1
// Method 2
Example 2: Multicast delegates
The following program demonstrates the multicasting of a delegate.
Example of multicast delegate using Delegate.Combine method.
using System;
namespace MulticastDelegate
{
class Program
{
// Declaring a delegate
public delegate void MyDelegate();
static void Main(string[] args)
{
// Declares the single delegate that points to MethodA
MyDelegate delegateA = new MyDelegate(MethodA);
// Declares the single delegate that points to MethodB
MyDelegate delegateB = new MyDelegate(MethodB);
// multicast delegate combining both delegates A and B
MyDelegate multicastDeligate = (MyDelegate)Delegate.Combine(delegateA, delegateB);
// Invokes the multicast delegate
multicastDeligate.Invoke();
}
static void MethodA()
{
Console.WriteLine("Executing method A.");
}
static void MethodB()
{
Console.WriteLine("Executing method B.");
}
}
}
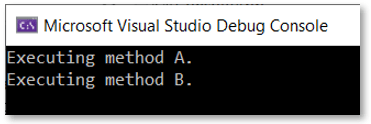
Example of multicast delegate using “+” and “-“ operator.
using System;
namespace MulticastDelegate
{
class Program
{
/// Declaring a delegate
public delegate void MyDelegate();
static void Main(string[] args)
{
// Add MethodA
MyDelegate multicastDelegate = Program.MethodA;
// To MethodB
multicastDelegate += Program.MethodB;
// To remove MethodA from the invocation list
// multicastDelegate -= Program.MethodA;
// Get the list of all delegates added to Multicast Delegate.
Delegate[] delegateList = multicastDelegate.GetInvocationList();
Console.WriteLine($"MulticastDelegate contains {delegateList.Length} delegate(s).");
// Invokes the multicast delegate
multicastDelegate.Invoke();
}
static void MethodA()
{
Console.WriteLine("Executed method A.");
}
static void MethodB()
{
Console.WriteLine("Executed method B.");
}
}
}
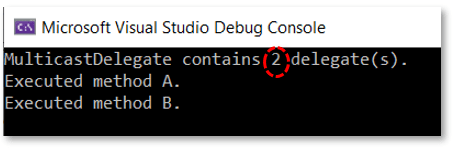
In the above example, a multicast delegate is used to hold a reference to the multiple methods.
C# delegate callback example
The following declaration shows how to use a delegate to declare a callback notification.
using System;
using System.Threading;
namespace DelegateExample
{
public delegate void MyDelegate(int num);
class DelegateCallBack
{
public static void LongRunningMethod(MyDelegate myDelegate)
{
for (int num = 1; num <= 5; num++)
{
Thread.Sleep(TimeSpan.FromSeconds(2));
myDelegate(num);
}
}
}
class program
{
public static void Main()
{
DelegateCallBack.LongRunningMethod(Print);
Console.ReadLine();
}
public static void Print(int number)
{
Console.WriteLine($" num : {number}");
}
}
}
Output:
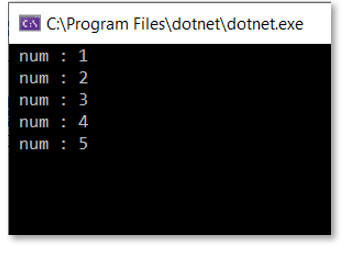
C# Anonymous method using the delegate keyword
We can use the delegate to invoke the anonymous method.
An anonymous method is a method without a name and return type.
It can be created using the delegate keyword and optional parameters and can be assigned to a variable of the delegate type.
The following is an example of the anonymous method.
using System;
namespace AnonymousMethodDemo
{
class Program
{
// Declaring a delegate
public delegate int MyDelegate(int num1, int num2);
static void Main(string[] args)
{
// Instantiating the delegate using an anonymous method
MyDelegate myDelegate = delegate (int num1, int num2)
{
return num1 + num2;
};
Console.WriteLine(myDelegate(10,20));
}
}
// Output: 30
}
Important points about C# delegates
Here are some important points about C# delegates explained simply:
- C# delegates are like containers that hold the name and signature of a method.
- Delegates allow you to indirectly call methods by invoking the delegate.
- Delegates provide a way to pass methods around as if they were objects.
- They enable flexible and reusable code by decoupling method calls from their implementations.
- Delegates are object-oriented, type-safe, and secure.
- Singlecast delegates can point to only one method at a time.
- Multicast delegates can hold references to multiple methods and invoke them in sequence.
- To add multiple methods to a multicast delegate, you can use the
Delegate.Combine
method or the “+” operator. - To remove a method reference from a multicast delegate, you can use the “-” operator.
- All methods in a multicast delegate must have the same signature (parameter types and return type must match).
- Delegates are commonly used in event handling, callback functions, and asynchronous programming.
- Delegates provide a higher level of abstraction and ensure type safety during compilation to prevent runtime errors.
Conclusion
C# delegates provide a powerful way to encapsulate and pass around methods as objects. They enable you to write more flexible and reusable code by separating method invocation from method implementation.
FAQs
Q: What is a C# delegate?
A C# delegate is like a container that holds a reference to a method. It allows you to indirectly call a method by invoking the delegate.
Q: How is a delegate different from a regular method call?
A delegate provides a way to decouple method calls from their implementations. It allows you to pass methods around as if they were objects, enabling flexible and reusable code.
Q: Why should I use delegates instead of directly invoking the method in C#?
1. You may sometimes need to pass a method as a parameter to another method, so in that case, you’ll need delegates.
Â
2. Delegates are commonly used for event handling, allowing a function to be invoked by a module that has no knowledge of the function’s implementation.
Delegates come in handy when components need to notify other components that they’ve subscribed. It can be used to implement the observer pattern, often known as the publish/subscribe pattern.
Q: How many types of delegates are there in C#?
Ans: There are three different types of delegates in C#. Single Delegate, Multicast Delegate, and Generic Delegate
Q: Can delegates be private in C#?
Ans: Yes, delegates can be declared as private, public, or internal just like classes.
Q: What is a Singlecast delegate?
A Singlecast delegate is a type of delegate that can only point to one method at a time. It holds a reference to a single method and can invoke that method when called.
Q: What is a multicast delegate?
To add multiple methods to a multicast delegate, you can use the Delegate.Combine method or the “+” operator.
Q: How do I remove a method reference from a multicast delegate?
To remove a method reference from a multicast delegate’s invocation list, you can use the “-” operator.
Q: Can a multicast delegate have methods with different signatures?
No, all methods in a multicast delegate should have the same signature. This means that their parameter types and return type must match.
Q: Can a delegate return a value?
Yes, a delegate can return a value. However, when using a multicast delegate, the return type of all methods in the invocation list should be void.
Q: When would I use delegates in my code?
Delegates
are useful in scenarios such as event handling, callback functions, and asynchronous programming, where you need to pass methods as arguments or define different actions based on conditions or user interactions.
I hope you like this article “C# Delegates“, if you have any questions, please ask your questions in the comment section below.
References MSDN: C# Delegates, Delegates, geeksforgeeks- C#|Delegates
Recommended Articles:
- Generic Delegates in C# With Examples
- C# Array vs List: When should you use an array or a List?
- Serialization and Deserialization in C# with Example
- SOLID Design Principles in C#: A Complete Example
- Understanding Class vs Struct vs Record in C# with examples
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- C# Struct vs Class
- IEnumerable Interface in C# with examples
- Constructors in C# with Examples
- Properties In C# with examples
- 10 Difference between interface and abstract class In C#
- Value Type and Reference Type in C#
- Multithreading in C#
- C# Dictionary with Examples
- Sealed Class in C# with examples
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024