In today’s digital world, Web APIs (Application Programming Interfaces) play a crucial role in enabling communication between different software systems. Web APIs allow applications to talk to each other and exchange data seamlessly over the internet.
This article will introduce you to the fundamentals of ASP.NET Web API with multiple examples.
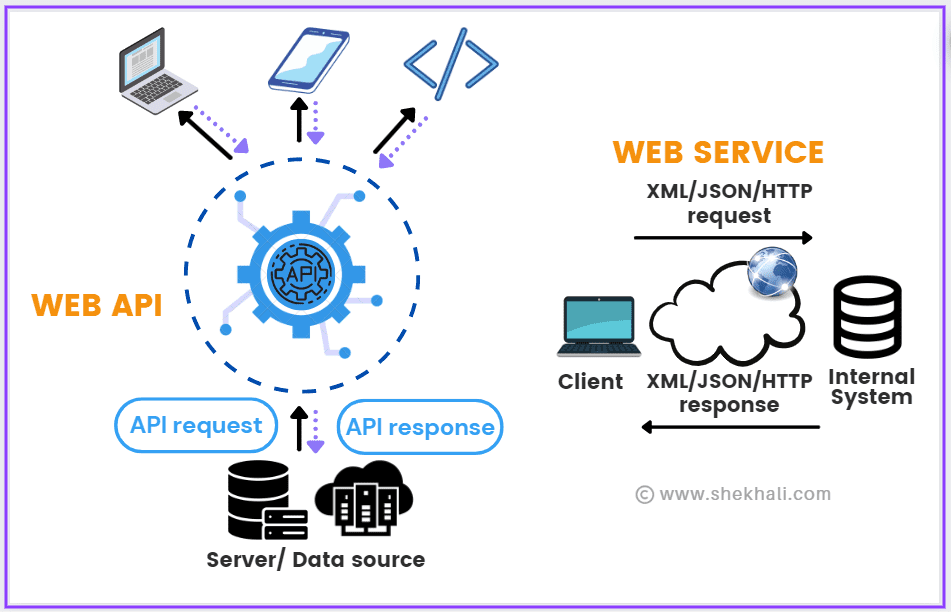
Table of Contents
What is Web API?
API stands for Application Programming Interface. Web API is a set of rules that allows different apps and websites to talk to each other over the internet. It’s like a language they use to exchange data and work together.
Imagine it as a bridge that connects apps and helps them exchange data smoothly. It defines how requests and responses should be structured for smooth data exchange.
What is ASP .NET Web API?
ASP.NET Web API is a framework designed to create services that use the HTTP protocol. These services can be accessed by various applications running on different platforms, such as web browsers, Windows applications, mobile devices , IOTs etc.
It provides a seamless way to build APIs that facilitate communication and data exchange between different software systems.
Example: Imagine you have a weather application that wants to show real-time weather data on your website. Instead of building a complete weather system from scratch, you can use a weather API provided by a third-party service to fetch the weather data and display it on your website.
Example: HTTP GET Endpoint
using Microsoft.AspNetCore.Mvc;
[ApiController]
[Route("api/weather")]
public class WeatherController : ControllerBase
{
[HttpGet("{city}")]
public ActionResult<string> GetWeather(string city)
{
// In a real application, you would fetch weather data from a service or database
// For simplicity, we'll return a static response
return $"The weather in {city} is sunny!";
}
}
Code Explanation:
The above code example shows a simple HTTP GET endpoint that takes a city parameter and returns the weather information for that city. The [ApiController]
attribute enables automatic model validation, and the [Route]
attribute specifies the URL route for the endpoint.
Characteristics of ASP.NET Web API
ASP.NET Web API is a framework provided by Microsoft for building web APIs using the ASP.NET platform. It has several characteristics that make it a popular choice among developers:
- HTTP-based: ASP.NET Web API is built on top of the HTTP protocol, which is the foundation of data communication on the Internet. It leverages standard HTTP methods, such as GET, POST, PUT, DELETE, etc., to perform CRUD (Create, Read, Update, Delete) operations. HTTP is the backbone of the World Wide Web, which makes it easy to consume APIs from different clients.
- RESTful Design:Â ASP.NET Web API follow REST (Representational State Transfer) principles. It means that APIs are organized around resources, and each resource is identified by a unique URL. RESTful APIs use standard HTTP methods and status codes for communication. The web APIs designed using REST principles are called RESTful APIs.
- Routing: Web API supports routing, allowing developers to define custom URLs for their API endpoints. It makes the API URLs more meaningful and user-friendly.
- JSON and XML Support:Â ASP.NET Web API supports JSON (JavaScript Object Notation) and XML (eXtensible Markup Language) data formats. JSON is widely used for its simplicity and lightweight nature, making it a popular choice for data exchange.
- Serialization: Web API automatically serializes and deserializes data between the client and the server. It can work with various data formats like JSON, XML, and others, making data interchange seamless.
- Model Binding:Â In Web API, Model binding is a process where incoming data from a client request is automatically mapped to the parameters of an API method. It simplifies data handling by converting JSON or form data into strongly-typed objects.
- Content Negotiation: Content negotiation is a built-in feature in ASP.NET Web API that allows clients to request data in different formats (like JSON or XML). The API automatically selects the appropriate format based on the client’s request.
Now, let’s take a look at different versions of ASP.NET Web API.
ASP.NET Web API Versions
ASP.NET Web API has evolved over time, and multiple versions have been released. Below is the detailed information about the different versions of ASP.NET Web API:
Version | Release Date | Description |
---|---|---|
ASP.NET Web API 1: | August 2012 | The initial release of ASP.NET Web API was included with ASP.NET MVC 4. It provided the foundation for building HTTP-based APIs using the ASP.NET framework. |
ASP.NET Web API 2: | October 2013 | Released with ASP.NET MVC 5, this version brought significant improvements and new features to the framework. It introduced attribute routing, CORS support, IHttpActionResult, and more. |
ASP.NET Web API 2.1: | April 2014 | A minor update that introduced new features like support for customizing property names in JSON serialization, support for OWIN self-hosting, and various bug fixes. |
ASP.NET Web API 2.2: | August 2014 | Another minor update that added features like IHttpActionResult for creating custom HTTP responses, async/await support for filters, and improvements in OWIN self-hosting. |
ASP.NET Web API 5: | July 2015 | Starting with this version, Web API was decoupled from the ASP.NET MVC framework and released independently as part of ASP.NET Core 5. |
ASP.NET Core 2.0 Web API: | August 2017 | With the introduction of ASP.NET Core, Web API underwent major changes. It provided cross-platform support, improved performance, and a new modular architecture. |
ASP.NET Core 2.1 Web API: | May 2018 | A minor update that introduced various features like HttpClientFactory, HTTPS improvements, and SignalR integration. |
ASP.NET Core 2.2 Web API: | December 2018 | This version brought features like endpoint routing, Open API (Swagger) integration, and improved performance with response caching. |
ASP.NET Core 3.0 Web API: | September 2019 | Major changes included support for C# 8.0, nullable reference types, new serialization options, and the ability to run Web API as a gRPC service. |
ASP.NET Core 3.1 Web API: | December 2019 | A long-term support release with bug fixes and improvements, maintaining compatibility with .NET Core 3.0. |
Please note that ASP.NET Core versions (starting from 5.0) are part of .NET 5 and later, which are separate from the traditional .NET Framework used in earlier versions.
The transition to ASP.NET Core brings multiple benefits, including cross-platform support, performance enhancements, and a more modular and lightweight framework.
As a result, ASP.NET Core is the recommended choice for new web API projects.
When to Choose ASP.NET Web API?
Now, let’s explore some scenarios when choosing ASP.NET Web API would be a great choice:
- Building APIs: If your project requires building a backend API to provide data and services to multiple clients, such as web applications, mobile apps, or IoT devices, ASP.NET Web API is an excellent choice. Its RESTful architecture and HTTP-based nature makes it ideal for handling API requests.
- Integration with ASP.NET Ecosystem:Â If you’re already working with the ASP.NET ecosystem, using ASP.NET Web API seamlessly integrates with other ASP.NET components, such as ASP.NET MVC, allowing you to reuse code and libraries effectively.
- Support for Various Data Formats:Â If you need to support multiple data formats like JSON and XML, ASP.NET Web API’s content negotiation feature simplifies exchanging data with different clients.
- Lightweight and Fast Development: ASP.NET Web API’s lightweight nature and flexible design allow for rapid API development. With minimal boilerplate code, developers can focus on building the actual API functionalities.
- Single Page Applications (SPAs): SPAs rely heavily on APIs to fetch and update data dynamically. Web API can seamlessly provide data to SPAs, enabling smooth user experiences.
- IoT (Internet of Things):Â When building IoT solutions, you might need to expose data from IoT devices over the Internet. Web API can handle device data and serve it to various clients.
- Microservices Architecture: Web API fits well into a microservices architecture, where different services must communicate and interact efficiently.
- Scalability and Performance:Â ASP.NET Web API is built on top of the mature ASP.NET framework, which offers excellent performance and scalability. It can handle a large number of concurrent API requests efficiently.
Now, let’s provide some code examples for better understanding:
Code Example 1: Creating a Simple ASP.NET Web API
// Step 1: Create a new ASP.NET Web API project in Visual Studio.
// Choose the "ASP.NET Web Application" template and select "API" as the project type.
// Step 2: Define a simple controller that handles API requests.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
namespace WebApiExample.Controllers
{
public class ValuesController : ApiController
{
// GET api/values
public IEnumerable<string> Get()
{
return new string[] { "Hello", "World" };
}
// GET api/values/5
public string Get(int id)
{
return "Value: " + id;
}
// POST api/values
public void Post([FromBody]string value)
{
// Code to save the 'value' to the database or perform other operations.
}
// PUT api/values/5
public void Put(int id, [FromBody]string value)
{
// Code to update the resource with 'id' with the provided 'value'.
}
// DELETE api/values/5
public void Delete(int id)
{
// Code to delete the resource with 'id'.
}
}
}
In this example, we have created a simple API controller named ValuesController
with methods to handle HTTP GET, POST, PUT, and DELETE requests. The Get methods return a list of strings or a specific value based on the provided ID.
Code Example 2: Configuring ASP.NET Web API for JSON and XML Support
// Step 1: Open the 'WebApiConfig.cs' file in the 'App_Start' folder of your project.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web.Http;
namespace WebApiExample
{
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
// Step 2: Enable attribute routing (for ASP.NET Web API 2 or higher).
config.MapHttpAttributeRoutes();
// Step 3: Configure content negotiation to support JSON and XML.
config.Formatters.JsonFormatter.SupportedMediaTypes.Add(new System.Net.Http.Headers.MediaTypeHeaderValue("text/html"));
config.Formatters.XmlFormatter.SupportedMediaTypes.Add(new System.Net.Http.Headers.MediaTypeHeaderValue("application/xml"));
}
}
}
In this example, we have configured the ASP.NET Web API to support JSON and XML data formats. JSON is widely used for data exchange, and XML can be useful in certain scenarios where specific clients require it.
These code examples give you a practical understanding of ASP.NET Web API and how it can be used to build powerful APIs for your web applications.
Frequently Asked Questions (FAQs)
Q: What is a Web API?Â
A Web API is a set of rules and protocols that allows different software applications to communicate and exchange data over the
Internet. It enables apps to request and share information with each other.
Q: What does API stand for?
API stands for “Application Programming Interface.” It’s like a bridge that allows different programs to talk to each other and use each other’s features.
Q: How does a Web API work?
A Web API works on the principles of the internet’s foundation, HTTP. Apps make requests using URLs (web addresses) to access data or perform actions on a server. The server responds with the requested information in a format like JSON or XML.
Q: What is RESTful in Web API?
RESTful means a Web API follows specific rules called “Representational State Transfer.” It organizes resources with unique URLs and uses standard HTTP methods (like GET and POST) to communicate, making it simple and easy to use.
Q: Is Web API only for websites?Â
No, Web APIs are not limited to websites. They are used in various applications, including mobile apps, desktop software, IoT devices, etc. Any program that needs to interact with other programs can use a Web API.
Articles to Check Out:
- Web services vs API: Top 10+ difference between API and Web Service
- WCF vs Web API: Top 10 Differences Between WCF and Web API
- C# Collections-(Stack, Queue, Array, dictionary, Hashtable, ArrayList, SortedList)
- C# Hashtable vs Dictionary vs HashSet
- C# Array vs List
- C# Struct vs Class
- C# Abstract class Vs Interface
- Generic Delegates in C# With Examples
- SOLID Design Principles in C#: A Complete Example
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- Constructors in C# with Examples
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024