In this article, we will take a deep dive into HashSet in C# with real world examples and clear explanations along the way.
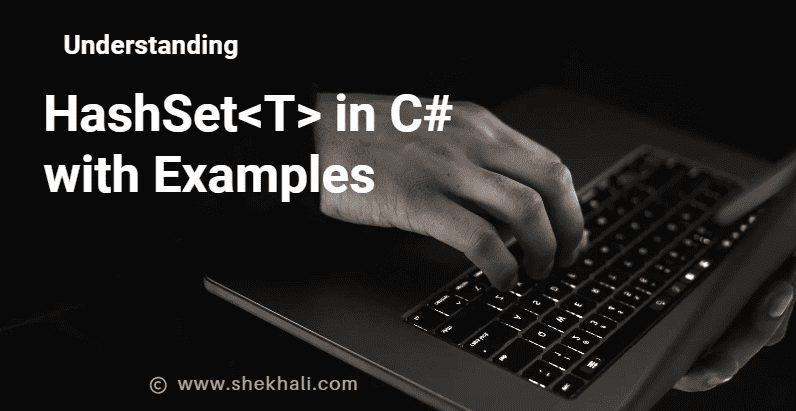
Table of Contents
What is HashSet in C#?
A HashSet is an unordered collection that stores a unique set of elements and does not allow duplicate elements. It’s a generic type of collection and is available in the System.Collections.Generic
namespace.
HashSet uses a hash table to store its elements, which means it provides fast retrieval of data and prevents duplicate elements from being stored in the collection.
key points about HashSet in C#
Here are the key points to remember about HashSet in C#:
- No Duplicates: HashSet is a generic collection that does not allow duplicate elements. It automatically ensures that each element is unique.
- Fast Access: HashSet uses a hash table internally which provides efficient storage and fast retrieval of data.
- Namespace:
HashSet<T>
is a generic class in theSystem.Collections.Generic
namespace. - Adding Elements: To add elements to a HashSet, use the Add method. It will add the element if it’s not already present.
- Removing Elements: We can use the Remove() method to delete specified elements from the HashSet.
- Iteration: You can iterate through the elements of a HashSet using a foreach loop. The
Count
property provides the number of elements in the HashSet. - Performance: HashSet is particularly useful when you need to perform quick checks for duplicates. It offers near-constant time complexity for adding, removing, and searching for elements.
- Real World Usage: HashSet is valuable in scenarios where you need to maintain a collection of unique items, such as student registration systems or managing unique user IDs.
Creating a HashSet:
To create a HashSet in C#, you’ll need to use the System.Collections.Generic
namespace. Here’s how you can create one:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{ // Creating a HashSet
HashSet<string> fruits = new HashSet<string>();
fruits.Add("Apple");
fruits.Add("Apple");
fruits.Add("Banana");
fruits.Add("Cherry");
fruits.Add("Mango");
fruits.Add("Mango");
// Total Items
Console.WriteLine( $"Total Items: {fruits.Count}");
foreach (var fruit in fruits)
{
Console.WriteLine(fruit);
}
}
}
Output:
Total Items: 4
Apple
Banana
Cherry
Mango
Code Explanation:
In this example, we create a HashSet
of strings named fruits. We added different fruit names to it and then iterate through the HashSet using a foreach
loop to print each fruit.
Since HashSet does not allow duplicates, it will automatically remove any additional occurrences of the same fruit.
Adding and Removing Elements
Now, let’s explore how to add and remove elements from a HashSet.
Adding Elements
To add elements to a HashSet, you can use the Add method. Here’s an example:
HashSet<int> numbers = new HashSet<int>();
numbers.Add(1);
numbers.Add(2);
numbers.Add(3);
In this code, we create a HashSet of integers and add three numbers. HashSet takes care of ensuring that there are no duplicates.
Removing Elements
To remove elements, you can use the Remove
method. Here is an example:
numbers.Remove(2);
We first added three numbers to the HashSet. Then, we remove the number 2 using the Remove method. After removal, the HashSet
will contain only the numbers 1 and 3.
HashSet and Performance
One of the significant advantages of using a HashSet is its performance, especially when searching for elements.
HashSet uses a hash table, which provides near-constant time complexity for adding, removing, and searching for elements.
It makes HashSet an excellent choice for scenarios where you need to check for duplicates quickly.
Real-World Example of HashSet: Student Registration
Let’s take a real-world example to understand how HashSet can be used effectively.
Imagine you are developing a student registration system, and you want to ensure that each student is registered only once. HashSet can help you achieve this efficiently.
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
HashSet<string> registeredStudents = new HashSet<string>();
Console.WriteLine("Student Registration System");
while (true)
{
Console.Write("Enter student name (or 'exit' to quit): ");
string input = Console.ReadLine();
if (input.ToLower() == "exit")
{
break;
}
if (registeredStudents.Add(input))
{
Console.WriteLine($"{input} has been successfully registered.");
}
else
{
Console.WriteLine($"{input} is already registered.");
}
}
}
}
Output:
Student Registration System
Enter student name (or 'exit' to quit): Surya
Surya has been successfully registered.
Enter student name (or 'exit' to quit): Shekh
Shekh has been successfully registered.
Enter student name (or 'exit' to quit): Shekh
Shekh is already registered.
Enter student name (or 'exit' to quit):
Methods in the HashSet Collection in C#:
These methods provide various operations for working with HashSet in C#:
Method | Description |
---|---|
Add(T) | Adds the specified element to the set. |
Clear() | Removes all the elements from a HashSet<T> object. |
Contains(T) | Determines whether a HashSet<T> object contains the specified element. |
CopyTo(T[]) | Copies the elements of a HashSet<T> object to an array. |
CreateSetComparer() | Returns an IEqualityComparer object for equality testing of a HashSet<T> object. |
Equals(Object) | Determines if the specified object is equal to the current object. |
GetEnumerator() | Returns an enumerator that iterates through a HashSet<T> object. |
GetHashCode() | Default hash function. |
GetType() | Gets the Type of the current instance. |
IntersectWith(IEnumerable<T>) | Modifies the current HashSet<T> object to contain only elements present in both the object and the specified collection. |
MemberwiseClone() | Creates a shallow copy of the current Object. |
Remove(T) | Removes the specified element from the HashSet<T> object. |
RemoveWhere(Predicate<T>) | Removes elements matching conditions defined by a specified predicate from a HashSet<T> collection. |
SetEquals(IEnumerable<T>) | Determines if a HashSet<T> object and the specified collection contain the same elements. |
SymmetricExceptWith(IEnumerable<T>) | Modifies the current HashSet<T> object to contain only elements present in either the object or the specified collection, but not in both. |
ToString() | Returns a string representation of the current object. |
TrimExcess() | Sets the capacity of a HashSet<T> object to the actual number of elements it contains, rounded up to a nearby, implementation-specific value. |
TryGetValue(T, T) | Searches the set for a given value and returns the equal value if found. |
UnionWith(IEnumerable<T>) | Modifies the current HashSet<T> object to contain all elements present in itself, the specified collection, or both. |
Properties in the HashSet<T> in C#:
The following are the properties of the HashSet Collection:
Property | Description |
---|---|
Comparer | This property is used to get the IEqualityComparer<T> object to determine equality for the values in the set. |
Count | The Count property is used to get the total number of elements contained in the set. |
Find an element in a HashSet:
Here is a simple C# program that demonstrates how to find an element in a HashSet:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// Create a HashSet of integers
HashSet<int> numbers = new HashSet<int>();
// Add some numbers to the HashSet
numbers.Add(5);
numbers.Add(10);
numbers.Add(15);
numbers.Add(20);
numbers.Add(25);
// Element to find
int elementToFind = 15;
// Check if the element exists in the HashSet
if (numbers.Contains(elementToFind))
{
Console.WriteLine($"Element {elementToFind} was found in the HashSet.");
}
else
{
Console.WriteLine($"Element {elementToFind} was not found in the HashSet.");
}
}
}
Output:
Element 15 was found in the HashSet.
HashSet vs. List:
A common question is when to use a HashSet over a List. If you need to store a collection of unique elements and quickly check for existence, a HashSet is the way to go.
Lists allow duplicates and are better when maintaining a specific order.
List<string> names = new List<string> { "John", "Bob", "John" };
HashSet<string> uniqueNames = new HashSet<string> { "John", "Bob", "John" };
// uniqueNames will have only "Alice" and "Bob"
Here’s a detailed tabular comparison of HashSet and List in C#:
This table should help you understand the key differences between HashSet and List in C# and when to choose one over the other based on your specific requirements.
Feature | HashSet | List |
---|---|---|
Duplicates: | HashSet does not allow duplicates and stores unique items. | List allows duplicates items. |
Order of Elements: | HashSet does not maintain a specific order of elements. | List maintains the order of elements as added. |
Retrieving Elements: | Retrieving elements in HashSet is generally faster due to hashing. | Retrieving elements in List may be slower for large collections. |
Performance: | HashSet is excellent for checking existence and uniqueness. | List is best when you need to maintain a specific order. |
Memory Usage: | It may use more memory because of hash table overhead. | List typically uses less memory than a HashSet. |
Adding Elements: | Adding elements is quick if they are unique. | Adding elements is generally fast. |
Removing Elements: | Removing elements is quick. | Removing elements may require searching, so it can be slower for large collections. |
Contains: | Ideal for checking if an item exists. | Requires iterating through the list for checking. |
Use Cases: | HashSet is Ideal for scenarios where uniqueness is important and order doesn’t matter. | List is suitable when order needs to be preserved, and duplicates are allowed. |
Sorting: | HashSet doesn’t provide sorting. | List supports sorting using methods like Sort . |
FAQs
Here are some frequently asked questions (FAQs) about the HashSet in C#:
Q1: What is a HashSet in C#?
A HashSet in C# is a collection that stores a unique set of elements, meaning no duplicate elements are allowed. It uses hashing for efficient storage and fast retrieval of elements.
Q2: How do I create a HashSet in C#?
To create a HashSet in C#, you need to use the System.Collections.Generic
namespace and declare a HashSet with the desired data type.
For example, you can create a HashSet of integers like this:
HashSet numbers = new HashSet();
Q3: Can I add and remove elements from a HashSet?
Yes, you can add elements to a HashSet using the Add
method and remove elements using the Remove
method.
Q4: Does a HashSet maintain the order of elements?
No, a HashSet does not maintain a specific order of elements. If order is important, you should consider using a List instead.
Q5: When to Use HashSet in C#?
Use HashSet when you need to store unique elements, remove duplicates, quickly check for existing elements, perform set operations, and quickly retrieve data.
References: MSDN-HashSet<T> Class
Articles to Check Out:
- C# Collections-(Stack, Queue, Array, dictionary, Hashtable, ArrayList, SortedList)
- C# Hashtable vs Dictionary
- C# Hashtable vs Dictionary vs HashSet
- C# Array vs List
- C# Struct vs Class
- C# ArrayList vs List
- C# Abstract class Vs Interface
- Generic Delegates in C# With Examples
- SOLID Design Principles in C#: A Complete Example
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024