When it comes to working with collections in C#, you’ll often find yourself deciding between using a List or a Dictionary, as both serve for storing and managing data and belong to System.Collection.Generics
namespace.
In this article, we’ll discuss List vs Dictionary in C# with Examples and will understand when to use each and why.
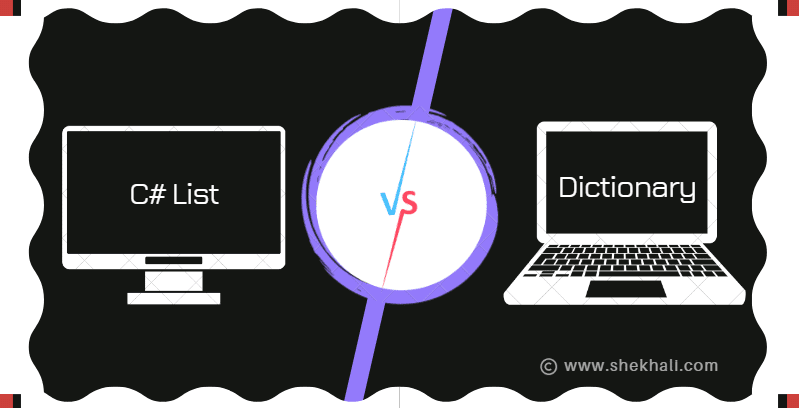
Table of Contents
- 1 What is List in C#?
- 2 What is Dictionary in C#?
- 3 List vs Dictionary in C#
- 4 Frequently Asked Questions (FAQs)
- 4.1 Q1: What is the main difference between List and Dictionary in C#?
- 4.2 Q2: When should I use a List in C#?
- 4.3 Q3: In which scenarios should I use Dictionary in C#?
- 4.4 Q4: Is it possible to iterate through a Dictionary in C#?
- 4.5 Q5 : What are the time complexities for insertion and deletion operations in Lists and Dictionaries?
- 4.6 Related
What is List in C#?
A List in C# is a dynamic array that can hold a collection of elements of the same type. It allows you to store and manipulate a list of items, such as integers, strings, or custom objects.
Lists maintain the order of elements and are accessible using indexes.
Code Example of List:
In this example, we create a List of strings, add some names to it, and then loop through the List to print each name.
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<string> names = new List<string>();
names.Add("Shekh Ali");
names.Add("Selena");
names.Add("Roman");
foreach (string name in names)
{
Console.WriteLine(name);
}
Console.ReadLine();
}
}
Output:
Shekh Ali
Selena
Roman
What is Dictionary in C#?
A Dictionary in C# is used to store key-value pairs where each element is associated with a unique key.
It’s like a real dictionary where you have a word (the key) and its definition (the value). You can use the key to retrieve the associated value quickly.
A Dictionary in C# is a generic collection class available in the System.Collections.Generic
namespace.
Code Example of Dictionary:
In this C# Dictionary example, we use names as keys and ages as values. You can access the value associated with a key efficiently.
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
Dictionary<string, int> ages = new Dictionary<string, int>();
ages["Shekh Ali"] = 25;
ages["Bob"] = 30;
ages["Marry"] = 35;
Console.WriteLine("Marry's age: " + ages["Marry"]);
Console.ReadLine();
}
}
Output:
Marry's age: 35
List vs Dictionary in C#
Now, let’s delve into the differences between List and Dictionary in C#.
Aspect | List | Dictionary |
---|---|---|
Purpose: | List in C# is used for storing a collection of elements of same type. | Dictionary in C# is used for storing key-value pairs where each key is unique. |
Data Structure: | List is an ordered collection. Elements are stored in the order they were added. | Dictionary stores Key-Value pairs where elements are accessed by a unique key. |
Duplicate Values: | List allows duplicate values. | Dictionary requires unique keys. Adding a duplicate key will overwrite the existing value. |
Lookup Efficiency: | You must iterate through the list to find a specific element. This can be slow for large lists. | Dictionary Offers constant-time lookup for values based on keys. It’s lightning-fast for retrieval. |
Memory Consumption: | List uses less memory because it only stores elements. | On the other hand, Dictionary uses more memory because it stores both keys and values. |
Error Handling: | Index Out of Range: Accessing an index beyond the List’s size results in an exception. Searching can be slow for large Lists. | Key Not Found: Trying to access a key that doesn’t exist in a Dictionary throws an exception. |
Common Use Cases: | List is suitable when you need to maintain the order of elements or when duplicate values are allowed. | Dictionary is Ideal for situations where you need fast lookups based on unique keys. |
Frequently Asked Questions (FAQs)
We understand that comparing Lists and Dictionaries in C# can raise several questions.
Here, we’ve gathered some of the most common queries to provide further clarity on the topic.
Q1: What is the main difference between List and Dictionary in C#?
The main difference is in how they store and access data.
Lists are dynamic arrays that store a collection of objects and provide fast access by index, while Dictionaries are key-value pairs and optimized for quick access of data using keys.
Q2: When should I use a List in C#?
Lists are suitable when you need to maintain the order of elements, frequently insert or delete elements, or require straightforward iteration through the collection.
Q3: In which scenarios should I use Dictionary in C#?
You should use a Dictionary in C# when you need to access elements quickly based on a key. Dictionaries are highly efficient for search operations.
They are ideal for scenarios where you require fast key-based lookups, such as implementing a cache, managing configuration settings, or building efficient data retrieval systems.
Q4: Is it possible to iterate through a Dictionary in C#?
Yes, you can iterate through a Dictionary using foreach
loops for both keys and values.
However, the order in which the elements are iterated may be different from the order in which they were added.
Q5 : What are the time complexities for insertion and deletion operations in Lists and Dictionaries?
Lists are more suitable for insertions and deletions, with an average time complexity of O(1) for insertions and O(n) for deletions.Â
Dictionaries, while allowing these operations, are less efficient, with an average case of O(1) and a worst-case scenario of O(n).
References: MSDN-Dictionary Class, List in C#
Related Articles:
- C# Hashtable vs Dictionary
- C# Array vs List
- C# ArrayList vs List
- C# List class
- C# Dictionary with examples
- Garbage Collection in C#: Managing Memory Efficiently
- C# Stack Class With Push And Pop Examples
- Generic Delegates in C# With Examples
- Static vs Singleton in C#: Understanding the Key Differences
- C# Tutorial
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024