A Hashtable, Dictionary, and HashSet are all data structures that store and retrieve data based on keys. However, there are some important differences between them.
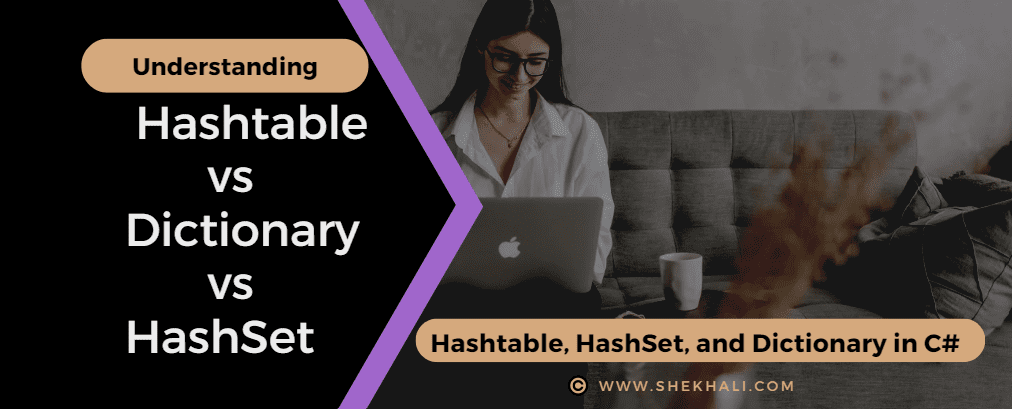
Following are the differences between a Hashtable
, Dictionary
, and HashSet
in C#
- A
Hashtable
is a collection that stores key-value pairs in a hash table, using the key as the hash value. It is thread-safe and allows null values, but it does not allow null keys and does not allow duplicate keys. It is not type-safe and has a slower performance compared to Dictionary. Hashtable is available in .NET 1.0 and above. It is slower and less efficient than the other two options, and it is not recommended for use in new code. - A
Dictionary
is a generic collection that stores key-value pairs in a hash table, using the key as the hash value. It is not thread-safe and does not allow null keys or null values. It does not allow duplicate keys.Dictionary
is available in .NET 2.0 and above. It is type-safe, faster, and more efficient than a hashtable, and it is the recommended choice for new code. - A
HashSet
is a generic collection that stores a set of values in a hash table. It does not allow null values but does allow null elements. It does not allow duplicate elements. It is thread-safe and type-safe.HashSet
has faster performance compared toDictionary
.HashSet
is available in .NET 3.5 and above. - If you need to store key-value pairs and need thread safety,
Hashtable
is a good choice. However, keep in mind that it is not type-safe and has a slower performance compared toDictionary
. - If you need to store key-value pairs and do not need thread safety,
Dictionary
is a good choice. It is type-safe and has faster performance compared toHashtable
. - If you need to store a set of values and do not need to store key-value pairs,
HashSet
is a good choice. It is thread-safe and type-safe and has faster performance compared toDictionary
.
It’s important to choose the right data structure for your specific needs, as each data structure has its own strengths and limitations.
Table of Contents
C# Hashtable vs Dictionary vs HashSet – Comparision
Following is a comparison of Hashtable
, Dictionary
, and HashSet
in C#
Comparison | Hashtable | Dictionary | HashSet |
---|---|---|---|
Data Structure | Hashtable | Hashtable | Hashtable |
Key | Object | TKey | T |
Value | Object | TValue | n/a |
Thread Safety | Yes | No | No |
Null Keys | No | No | Yes |
Null Values | Yes | Yes | No |
Duplicate Keys | No | No | No |
Inheritance | Yes | No | Yes |
Type Safety | No | Yes | Yes |
Performance | Slower | Faster | Faster |
Available in .NET | 1.0 | 2.0 | 3.5 |
C# HashSet<T>
A HashSet
is a collection of unique items in C#. It is a data structure that provides fast lookups and adds/removes items. It is implemented using a hash table, which is a data structure that maps keys to values using a hash function.
HashSet is a good choice for storing only unique elements as It won’t accept duplicate entries. It is a generic collection and is available in System.Collections.Generic
namespace.
Example:
Following is an example of how to use a HashSet
in C#:
using System;
using System.Collections.Generic;
namespace HashSetExample
{
class Program
{
static void Main(string[] args)
{
// Creating a new HashSet
HashSet<int> hashset = new HashSet<int>();
// Add some elements to the set
hashset.Add(1);
hashset.Add(2);
hashset.Add(3);
// The set contains only unique items, so adding a duplicate will replace the existing one
hashset.Add(3);
// Check if the set contains a specific item
if (hashset.Contains(2))
{
Console.WriteLine("The set contains 3.");
}
// Iterate over the items in the set
foreach (int item in hashset)
{
Console.WriteLine(item);
}
// Remove an item from the set
hashset.Remove(3);
Console.WriteLine("After removing 3, the set contains:");
foreach (int item in hashset)
{
Console.WriteLine(item);
}
Console.ReadKey();
}
}
}
Output:
The output of the above program would be:
The HashSet contains 3.
1
2
3
After removing 3, the set contains:
1
2
C# Dictionary<T>
The Dictionary
class in C# is a generic data structure that stores key-value pairs, similar to a hashtable. It is found in the System.Collections.Generic
namespace and provides a more efficient and type-safe alternative to the Hashtable
class.
Learn more about Dictionary here: Dictionary<T> in C# with examples
Example:
Following is an example of how to use the Dictionary
class in C#:
using System;
using System.Collections.Generic;
namespace DictionaryClassExample
{
class Program
{
static void Main(string[] args)
{
// Create a new dictionary
Dictionary<string, int> dictionary = new Dictionary<string, int>();
// Adding some key-value pairs to the dictionary
dictionary.Add("key1", 100);
dictionary.Add("key2", 200);
dictionary.Add("key3", 300);
dictionary.Add("key4", 400);
// Get the number of key-value pairs in the dictionary
int count = dictionary.Count;
Console.WriteLine($"Number of key-value pairs: {count}");
// Get the keys in the dictionary
ICollection<string> keys = dictionary.Keys;
Console.WriteLine("*** Keys ***");
foreach (var key in keys)
{
Console.WriteLine(key);
}
// Get the values in the dictionary
ICollection<int> values = dictionary.Values;
Console.WriteLine("*** Values ***");
foreach (var value in values)
{
Console.WriteLine(value);
}
// Check if the dictionary contains a specific key
bool containsKey = dictionary.ContainsKey("key4");
Console.WriteLine($"Contains key 'key4': {containsKey}");
// Check if the dictionary contains a specific value
bool containsValue = dictionary.ContainsValue(300);
Console.WriteLine($"Contains value 300: {containsValue}");
// Iterate over the key-value pairs in the dictionary
Console.WriteLine("Key-value pairs:");
foreach (var entry in dictionary)
{
string key = entry.Key;
int value = entry.Value;
Console.WriteLine($"Key: {key}, Value: {value}");
}
Console.ReadKey();
}
}
}
Output:
Number of key-value pairs: 4
*** Keys ***
key1
key2
key3
key4
*** Values ***
100
200
300
400
Contains key 'key4': True
Contains value 300: True
Key-value pairs:
Key: key1, Value: 100
Key: key2, Value: 200
Key: key3, Value: 300
Key: key4, Value: 400
C# Hashtable
In C#, a Hashtable is a collection that stores key-value pairs. It is similar to a dictionary, but it is not type-safe, which means you can store keys and values of different types in the same Hashtable.
Learn more about Hashtable here: C# Hashtable with examples
Example:
Following is an example of how to create and use a Hashtable in C#:
using System;
using System.Collections;
namespace DictionaryClassExample
{
class Program
{
static void Main(string[] args)
{
Hashtable hashtable = new Hashtable();
// Adding some key-value pairs to the hashtable
hashtable.Add("Key1", "Value1");
hashtable.Add("Key2", 200);
hashtable.Add("Key3", true);
// Getting the value associated with a key
string value1 = (string)hashtable["Key1"];
int value2 = (int)hashtable["Key2"];
bool value3 = (bool)hashtable["Key3"];
Console.WriteLine($"Key1: {value1}, Key2: {value2}, Key3: {value3}");
// foreach loop to Iterate through the hashtable
foreach (DictionaryEntry entry in hashtable)
{
Console.WriteLine($"Key: {entry.Key}, Value: {entry.Value}");
}
// Check if the hashtable contains a key
if (hashtable.ContainsKey("Key1"))
{
Console.WriteLine("The hashtable contains the key 'Key1'");
}
// Remove a key-value pair from the hashtable
hashtable.Remove("Key2");
Console.ReadKey();
}
}
}
In the above code example, we created a new Hashtable and added three key-value pairs. We then retrieve the values associated with the keys, iterate through the Hashtable, check if it contains a specific key, and remove a key-value pair.
Output:
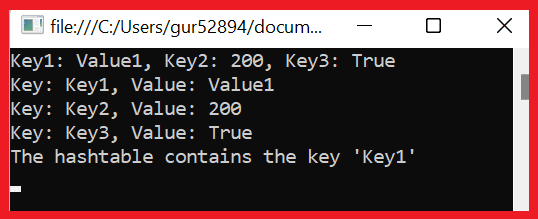
Summary:
In summary, if you need to store key-value pairs and retrieve values based on keys, you should use a dictionary. If you need to store a set of values and check for the existence of a value quickly, you should use a hash set. If you are working with legacy code that uses a hashtable, you may need to continue using it, but for new code, you should use a dictionary or a hash set instead.
FAQ:
Following are some common questions about HashSet, Hashtable, and Dictionary in C#:
Q: When should you use a HashSet in C#?
A: You should use a hash set in C# when you need to store a large number of values and need to check for the presence of a value quickly. Hash sets are useful for scenarios where you need to store a set of values and perform operations such as adding, removing, and checking for the presence of values in the set.
Q: When should you use a dictionary in C#?
A: You should use a dictionary in C# when you need to store key-value pairs and retrieve values based on keys. Dictionaries are faster and more efficient than hashtables, and they allow you to specify the types of keys and values they store. They are the recommended choice for new code when you need to store and retrieve data based on keys.
Q: When should you use a hashtable in C#?
A: You should generally not use a hashtable in C# for new code. Hashtables are slower and less efficient than dictionaries, and they are considered obsolete. If you are working with legacy code that uses a hashtable, you may need to continue using it, but for new code, you should use a dictionary or a HashSet instead.
Here are some recommended articles on collections:
- C# Queue with examples
- C# Stack
- C# Dictionary
- C# Hashtable
- Collections in C#
- Array vs List
- Array vs ArrayList in C#
- C# List with examples
- C# Arrays
Refernces: MSDN-Hashtable, HashSet
If you enjoyed this post, please share it with your friends or leave a comment below.
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024