In this article, we will delve into System.IO
namespace in C# and understand the classes it provides to perform various input and output operations in a C# program. This article will cover all the essential topics of System.IO
namespace, including the classes, methods, and properties, along with code examples to illustrate their use.
This article aims to provide a comprehensive understanding of System.IO
namespace and help you in your journey of mastering C# programming.
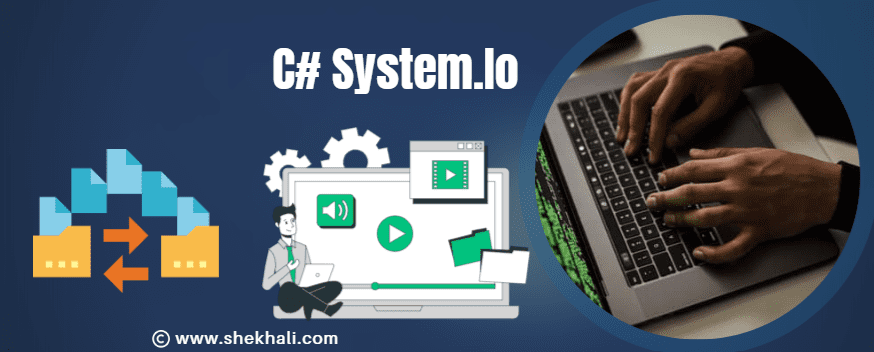
Table of Contents
I. System.IO Namespace:
The System.IO namespace is part of the .NET framework and provides various classes to perform input and output operations in a C# program. This namespace contains classes for reading and writing to files, manipulating directories and files, working with streams, and more. System.IO
namespace is essential for most C# programs that require file and directory operations.
II. File and Directory Operations:
File and directory operations are critical components of many C# applications. The System.IO namespace provides several classes for performing file and directory operations, including the following:
- File Class: This class provides methods for creating, copying, deleting, moving, and opening files.
- Directory Class: This class provides methods for creating, deleting, and enumerating directories.
- FileInfo Class: This class provides methods for manipulating files, such as copying and moving files.
- DirectoryInfo Class: This class provides methods for manipulating directories, such as creating and deleting directories.
Example code for performing file operations:
using System;
using System.IO;
namespace FileOperations
{
class Program
{
static void Main(string[] args)
{
// Creating a file
File.Create("test.txt");
// Copying a file
File.Copy("test.txt", "test_copy.txt", true);
// Moving a file
File.Move("test.txt", "test_move.txt");
// Deleting a file
File.Delete("test_copy.txt");
// Creating a directory
Directory.CreateDirectory("test_dir");
// Deleting a directory
Directory.Delete("test_dir");
}
}
}
III. Streams:
Streams are a fundamental concept in C# for performing input and output operations. The System.IO namespace provides several stream classes for reading and writing data. The most commonly used stream classes are:
- MemoryStream: This class provides a stream for reading and writing to memory.
- FileStream: This class provides a stream for reading from and writing to files.
- NetworkStream: This class provides a stream for reading from and writing to network connections.
- BufferedStream: This class provides a stream for reading from and writing to a buffered stream.
Example:
Example code for reading from a stream:
using System;
using System.IO;
namespace StreamOperations
{
class Program
{
static void Main(string[] args)
{
// Reading from a file stream
using (FileStream fs = new FileStream("test.txt", FileMode.Open, FileAccess.Read))
{
using (StreamReader reader = new StreamReader(fs))
{
string line;
while ((line = reader.ReadLine()) != null)
{
Console.WriteLine(line);
}
}
}
}
}
}
To read more about stream, please refer to this article: StreamReader and StreamWriter in C# with examples
IV. File I/O Exceptions:
It is essential to handle exceptions that may occur when performing file and directory operations. The System.IO namespace provides several exceptions that can be thrown, including:
- FileNotFoundException: This exception is thrown when a file cannot be found.
- DirectoryNotFoundException: This exception is thrown when a directory cannot be found.
- IOException: This exception is thrown when an I/O error occurs.
Example code for handling file I/O exceptions:
using System;
using System.IO;
namespace ExceptionHandling
{
class Program
{
static void Main(string[] args)
{
try
{
// Reading from a file that doesn't exist
using (StreamReader reader = new StreamReader("non_existent_file.txt"))
{
string line;
while ((line = reader.ReadLine()) != null)
{
Console.WriteLine(line);
}
}
}
catch (FileNotFoundException ex)
{
Console.WriteLine("File not found: " + ex.Message);
}
catch (IOException ex)
{
Console.WriteLine("I/O Error: " + ex.Message);
}
}
}
}
V. Text I/O:
Text I/O is a common operation in C#, and the System.IO namespace provides several classes for performing text I/O, including:
- StreamReader: This class provides methods for reading text from a stream.
- StreamWriter: This class provides methods for writing text to a stream.
- StringReader: This class provides methods for reading text from a string.
- StringWriter: This class provides methods for writing text to a string.
Example code for reading and writing text in C#:
using System;
using System.IO;
namespace TextIO
{
class Program
{
static void Main(string[] args)
{
// Writing text to a file
using (StreamWriter writer = new StreamWriter("test.txt"))
{
writer.WriteLine("Hello World");
writer.WriteLine("This is a test.");
}
// Reading text from a file
using (StreamReader reader = new StreamReader("test.txt"))
{
string line;
while ((line = reader.ReadLine()) != null)
{
Console.WriteLine(line);
}
}
}
}
}
VI. Binary I/O:
Binary I/O is an essential operation in C#, and the System.IO namespace provides several classes for performing binary I/O, including:
- BinaryReader: This class provides methods for reading binary data from a stream.
- BinaryWriter: This class provides methods for writing binary data to a stream.
The following is the sample code example for reading and writing binary data:
using System;
using System.IO;
namespace BinaryIO
{
class Program
{
static void Main(string[] args)
{
// Writing binary data to a file
using (BinaryWriter writer = new BinaryWriter(new FileStream("binary.bin", FileMode.Create)))
{
writer.Write(123456);
writer.Write(3.14159265358979);
writer.Write("Hello World");
}
// Reading binary data from a file
using (BinaryReader reader = new BinaryReader(new FileStream("binary.bin", FileMode.Open)))
{
int i = reader.ReadInt32();
double d = reader.ReadDouble();
string s = reader.ReadString();
Console.WriteLine("Integer: " + i);
Console.WriteLine("Double: " + d);
Console.WriteLine("String: " + s);
}
}
}
}
Class | Description |
---|---|
File: | Provides static methods for the creation, copying, deletion, moving, and opening of a single file. |
FileInfo: | Represents a file and provides instance methods for the creation, copying, deletion, moving, and opening of a single file. |
Directory: | Provides static methods for creating, deleting, and enumerating directories. |
DirectoryInfo: | Represents a directory and provides instance methods for creating, deleting, and enumerating directories. |
Path: | Provides methods for processing directory and file path strings. |
FileStream: | Represents a stream for reading from and writing to a file. |
StreamReader: | This represents a reader that can read a sequence of characters from a file or a stream. |
StreamWriter: | Represents a writer that can write a sequence of characters to a file or a stream. |
BinaryWriter: | Represents a writer that can write primitive data types in binary to a stream. |
These classes provide a comprehensive set of tools for performing I/O operations and allow you to easily read and write text and binary data, perform file and directory operations, and handle exceptions that may occur during I/O operations. Whether a beginner or an experienced developer, these classes provide the necessary functionality to work with files and directories in C#.
Example:
This code demonstrates how to use the File class to create a file, the Directory class to create a directory, the StreamReader class to read text data from a file, and the BinaryWriter class to write binary data to a file. The code also shows how to use the using statement to automatically close the file and release any associated resources when you’re done with them.
using System;
using System.IO;
namespace SystemIOExample
{
class Program
{
static void Main(string[] args)
{
// Use the File class to create a file
string filePath = @"C:\example.txt";
File.WriteAllText(filePath, "Hello, World!");
Console.WriteLine($"File created at: {filePath}");
// Use the Directory class to create a directory
string directoryPath = @"C:\exampleDirectory";
Directory.CreateDirectory(directoryPath);
Console.WriteLine($"Directory created at: {directoryPath}");
// Use the StreamReader class to read text data from a file
using (StreamReader reader = new StreamReader(filePath))
{
string content = reader.ReadToEnd();
Console.WriteLine($"File content: {content}");
}
// Use the BinaryWriter class to write binary data to a file
using (BinaryWriter writer = new BinaryWriter(File.Open(filePath, FileMode.Append)))
{
writer.Write(123456);
Console.WriteLine("Binary data written to file.");
}
Console.ReadLine();
}
}
}
Output:
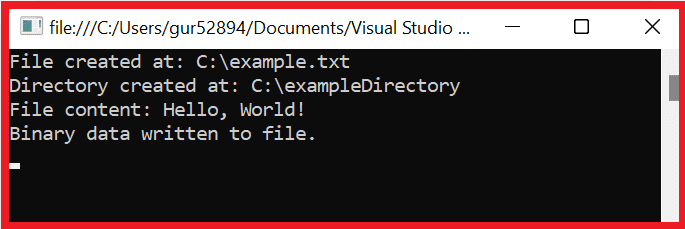
System.IO delegates in C#
- FileSystemEventHandler: Represents the method that will handle a change in the file system, such as the creation, deletion, or modification of a file or directory.
- RenamedEventHandler: Represents the method that will handle the renaming of a file or directory.
- ErrorEventHandler: This represents the method that will handle errors during file system operations.
- WaitForChangedResult: Represents the result of a file system change wait operation.
- FileSystemWatcher: Monitors the file system for changes and raises events when a file or directory is created, deleted, modified, or renamed.
- Directory: Provides static methods for creating, deleting, and enumerating directories.
- DirectoryInfo: Represents a directory and provides instance methods for creating, deleting, and enumerating directories.
These delegates provide a way to handle file system events and monitor changes in the file system, which can be helpful in applications that need to keep track of changes to the files and directories. Additionally, the Directory and DirectoryInfo
classes provide methods for working with directories, making it easier to manage the file system in C#.
References: MSDN-System.IO Namespace
FAQs
Q: What is the System.IO namespace in C#?
The System.IO
namespace in C# provides a comprehensive set of classes for performing I/O operations, including file and directory operations, text and binary I/O, and exception handling.
Q: What are some of the key System.IO classes in C#?Â
Some important System.IO
classes in C# are: FileInfo
, Directory
, DirectoryInfo
, Path
, FileStream
, StreamReader
, StreamWriter
, BinaryReader
, and BinaryWriter
classes.
Q: Why do you use the File class in C#?
The File class in C# provides static methods for performing operations on a single file, such as creating, copying, deleting, moving, and opening a file.
Q: How do you read and write text data in C# using the StreamReader and StreamWriter classes?Â
The StreamReader and StreamWriter classes in C# provide methods for reading and writing text data to and from a file or a stream.
To read text data using the StreamReader class, you would create a StreamReader object and call its ReadLine
method to read each lines of the file.
To write text data using the StreamWriter class, you would create a StreamWriter object and call its WriteLine
method to write each line of text to the file.
You might also like:
- StreamReader and StreamWriter in C# with examples
- Properties in C#
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- URL vs URI: The Ultimate Guide to Understanding the difference between URL and URI
- C# Struct vs Class |Top 15+ Differences Between C# Struct and Class
- 10 Difference between interface and abstract class In C#
- C# Queue with examples
- Web API vs web service: Top 10+ Differences between web API and web service in C#
- C# Polymorphism: Different types of polymorphism in C# with examples
- C# Stack
- Understanding the Difference between == and Equals() Method in C#
- Difference between SortedList and SortedDictionary in C#
- C# Hashtable vs Dictionary vs HashSet
Please share this post and let us know what you think in the comments.
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024