In the world of programming, Collections are a powerful feature that helps us store and manage data in a structured and organized manner. C# provides two types of Collections:Â
- Generic and
- Non-Generic Collection Classes.
Both have their own set of advantages and disadvantages. This article will discuss the advantages and disadvantages of Non-Generic Collection Classes in C#.
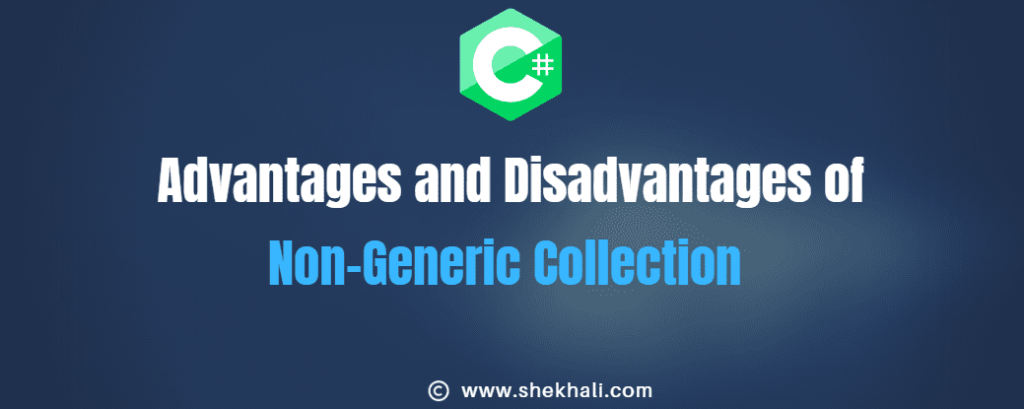
Table of Contents
- 1 Advantages and Disadvantages of Non-Generic Collection in C#
- 2 Comparison Table: Generic vs Non-Generic Collection in C#.
- 3 Conclusion:
- 4 FAQs
- 4.1 Q: What is a Non-Generic Collection Class in C#?
- 4.2 What are some examples of Non-Generic Collection Classes in C#?
- 4.3 Q: What are the advantages of using Non-Generic Collection Classes in C#?
- 4.4 Q: What are the disadvantages of using Non-Generic Collection Classes in C#?
- 4.5 Q: Which Collection Class should you use in C#?
- 4.6 Related
Advantages and Disadvantages of Non-Generic Collection in C#
Non-Generic Collection Classes are a type of Collection Classes in C# that can store any data, be it an integer, string, or even an object. These classes are useful in scenarios where the type of data that needs to be stored is not known beforehand. However, they also have their drawbacks.
01. Advantages of using Non-Generic Collection Classes in C#:
Non-Generic Collection Classes provide the following advantages:
- They can store any data, which makes them versatile.
- They are easy to use and don’t require specifying the data type.
- They are backward compatible with older versions of C#.
- We can use them in scenarios where the type of data that needs to be stored is not known beforehand.
Example 1:
Let’s take an example of storing data in an ArrayList
, a type of Non-Generic Collection Class.
ArrayList arrList = new ArrayList();
arrList.Add(1);
arrList.Add("DotNet");
arrList.Add(new Object());
In the above example, we can see that we are storing an integer, a string, and an object in the ArrayList
. It is possible because the ArrayList
is a Non-Generic Collection Class and can store any data type.
02. Disadvantages of using Non-Generic Collection Classes in C#:
Non-Generic Collection Classes have the following disadvantages:
- They are not type-safe, which means there are chances of run-time errors.
- They are slower in performance as compared to Generic Collection Classes.
- They require manual type-casting when retrieving data, which is unmanageable.
- They don’t provide compile-time type checking, which can lead to errors.
Example 2:
Let’s take an example of retrieving data from an ArrayList.
ArrayList arrList = new ArrayList();
arrList.Add(1);
arrList.Add("DotNet");
arrList.Add(new Object());
int firstElement = (int)arrList[0];
string secondElement = (string)arrList[1];
Object thirdElement = arrList[2];
In the above example, we are retrieving data from the ArrayList. We need to manually type-cast the data into its original type, which can be unmanageable and prone to errors.
Comparison Table: Generic vs Non-Generic Collection in C#.
Let’s compare the Generic and Non-Generic Collection Classes in C# using the following table:
Feature | Generic Collection | Non-Generic Collection |
---|---|---|
Type-Safe: | Yes | No |
Performance: | Faster | Slower |
Compile-time Checking: | Yes | No |
Backward Compatibility: | No | Yes |
Non-Generic Collection Classes are a set of Collection Classes in C# that can store any data, be it an integer, string, or object. Let’s discuss some of the popular Non-Generic Collection Classes:
- ArrayList: ArrayList is a dynamic array that can store any data type. It automatically grows in size as more elements are added to it. However, it is not type-safe and requires manual type-casting when retrieving data.
- Stack: A stack is a collection of elements that follows the Last-In-First-Out (LIFO) principle. It can store any data and provides methods such as Push() and Pop() for adding and removing elements from the stack.
- Queue: A queue is a collection of elements that follows the First-In-First-Out (FIFO) principle. It can store any data and provides methods such as Enqueue() and Dequeue() for adding and removing elements from the queue.
Conclusion:
C# contains generic and non-generic collections, which are available in System.Collections.Generic
 and System.Collections
namespace, respectively.
In conclusion, Non-Generic Collection Classes in C# provide flexibility in storing data of any type. However, they also come with disadvantages, such as being slower in performance and not being type-safe.
Understanding these advantages and disadvantages is important before choosing the appropriate Collection Class for your project.
References MSDN:Â Collections (C#)
FAQs
Q: What is a Non-Generic Collection Class in C#?
A Non-Generic Collection Class in C# is a collection class that can store any type of data. It does not require specifying the data type during declaration and can store a mixture of different types of data.
What are some examples of Non-Generic Collection Classes in C#?
Some examples of Non-Generic Collection Classes in C# are ArrayList
, Stack, Queue, SortedList
, and Hashtable
.
Q: What are the advantages of using Non-Generic Collection Classes in C#?
The advantages of using Non-Generic Collection Classes in C# are that they provide flexibility in storing different types of data and are easy to use.
Q: What are the disadvantages of using Non-Generic Collection Classes in C#?
The disadvantages of using Non-Generic Collection Classes in C# are that they are not type-safe, which means they require manual type-casting when retrieving data. They can be slower in performance compared to Generic Collection Classes.
Q: Which Collection Class should you use in C#?
The choice of Collection Class depends on the specific requirements of your project. If you need to store data of a specific type, it’s better to use Generic Collection Classes.
However, if you need flexibility in storing different types of data, Non-Generic Collection Classes may be a better option.
You Might Like:
- C# Stack
- C# Queue Class
- C# Collection
- C# Array Vs List
- C# Dictionary with Examples
- C# Hashtable vs Dictionary
- C# List Class With Examples
- Generics in C# with Examples
- Generic Delegates in C# With Examples
- C# Struct vs Class
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025